DevOps Made Simple: A Beginner’s Guide to Understanding Microservices and Their Role in DevOps
Introduction In the fast-evolving world of software development, organizations are shifting from traditional monolithic architectures to microservices. But what exactly are microservices, and why are they crucial for DevOps? Microservices break down large applications into smaller, independent services, making software development faster, scalable, and more resilient. In this blog, we will explore microservices, their importance in DevOps, real-world applications, common pitfalls, and best practices to follow. What Are Microservices? Microservices, also known as the microservices architecture, is a design approach where an application is structured as a collection of small, loosely coupled services. Each service performs a specific function and communicates with others via APIs. Key Characteristics of Microservices: Independence: Each service runs independently and can be developed, deployed, and scaled separately. Resilience: If one service fails, it doesn’t bring down the entire application. Technology Agnostic: Different services can be built using different programming languages and frameworks. Easy Maintenance: Developers can update individual services without affecting the whole system. Monolithic vs. Microservices Architecture Feature Monolithic Architecture Microservices Architecture Scalability Hard to scale Easily scalable Deployment Requires redeploying the entire app Deploy services independently Resilience Single point of failure Fault-tolerant Technology Flexibility Uses a single tech stack Multiple technologies supported How Microservices Fit into DevOps DevOps focuses on continuous integration and continuous delivery (CI/CD), automation, and faster software releases. Microservices align perfectly with these principles: CI/CD & Automation: Since microservices are independent, teams can continuously integrate and deploy changes without downtime. Containerization: Tools like Docker and Kubernetes simplify microservices deployment and orchestration. Scalability: Microservices enable dynamic scaling, optimizing resources efficiently. Monitoring & Logging: Tools like Prometheus, Grafana, and ELK stack help monitor microservices health. Real-World Applications of Microservices Many tech giants use microservices to enhance their services: 1. Netflix Netflix adopted microservices to handle millions of users. Every function—streaming, user authentication, recommendations—operates as an independent microservice. 2. Amazon Amazon transformed from a monolithic structure to microservices to improve its e-commerce platform’s scalability and efficiency. 3. Uber Uber’s ride-booking system utilizes microservices to manage pricing, driver-rider matching, notifications, and payments. Step-by-Step Guide: Deploying a Simple Microservices Application Prerequisites: Basic knowledge of Docker and Kubernetes Installed Docker and Kubernetes (Minikube for local testing) Step 1: Create Two Simple Microservices (Node.js Example) Service 1: User API const express = require('express'); const app = express(); app.get('/user', (req, res) => { res.json({ name: 'Yash', role: 'DevOps Engineer' }); }); app.listen(3000, () => console.log('User API running on port 3000')); Service 2: Product API const express = require('express'); const app = express(); app.get('/product', (req, res) => { res.json({ product: 'Laptop', price: 75000 }); }); app.listen(4000, () => console.log('Product API running on port 4000')); Step 2: Dockerizing the Microservices Create a Dockerfile for each service: FROM node:14 WORKDIR /app COPY package.json . RUN npm install COPY . . CMD ["node", "index.js"] EXPOSE 3000 Build and run the containers: docker build -t user-api . docker run -p 3000:3000 user-api Step 3: Deploying to Kubernetes Create a Kubernetes Deployment YAML file: apiVersion: apps/v1 kind: Deployment metadata: name: user-api spec: replicas: 2 selector: matchLabels: app: user-api template: metadata: labels: app: user-api spec: containers: - name: user-api image: user-api:latest ports: - containerPort: 3000 Apply the deployment: kubectl apply -f user-api-deployment.yaml Common Mistakes & Best Practices Common Mistakes: Tightly Coupled Services: If microservices depend too much on each other, they lose their independent nature. Lack of Monitoring: Without proper observability, debugging becomes difficult. Incorrect API Versioning: Changes in APIs should be backward-compatible to avoid breaking dependent services. Best Practices: Use API Gateways: Manage requests efficiently using API gateways like Kong or Nginx. Automate Deployment: Use
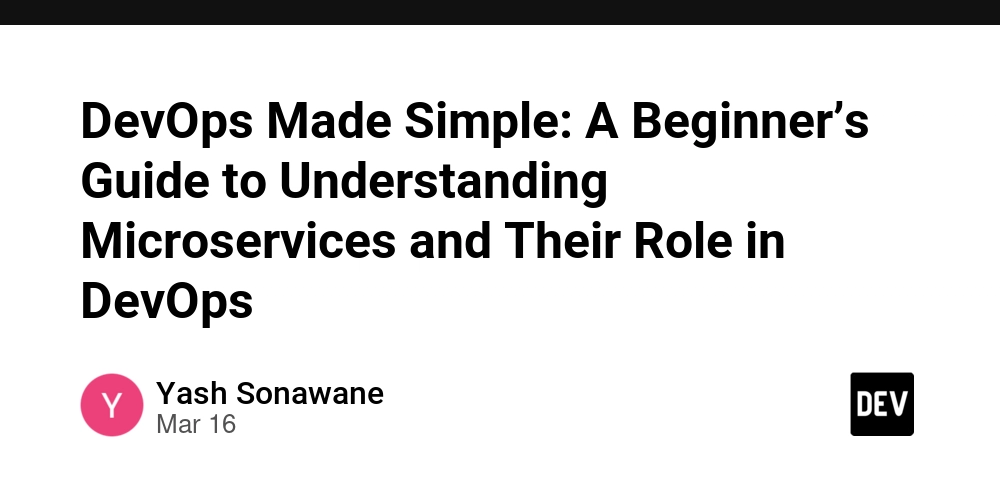
Introduction
In the fast-evolving world of software development, organizations are shifting from traditional monolithic architectures to microservices. But what exactly are microservices, and why are they crucial for DevOps?
Microservices break down large applications into smaller, independent services, making software development faster, scalable, and more resilient. In this blog, we will explore microservices, their importance in DevOps, real-world applications, common pitfalls, and best practices to follow.
What Are Microservices?
Microservices, also known as the microservices architecture, is a design approach where an application is structured as a collection of small, loosely coupled services. Each service performs a specific function and communicates with others via APIs.
Key Characteristics of Microservices:
- Independence: Each service runs independently and can be developed, deployed, and scaled separately.
- Resilience: If one service fails, it doesn’t bring down the entire application.
- Technology Agnostic: Different services can be built using different programming languages and frameworks.
- Easy Maintenance: Developers can update individual services without affecting the whole system.
Monolithic vs. Microservices Architecture
Feature | Monolithic Architecture | Microservices Architecture |
---|---|---|
Scalability | Hard to scale | Easily scalable |
Deployment | Requires redeploying the entire app | Deploy services independently |
Resilience | Single point of failure | Fault-tolerant |
Technology Flexibility | Uses a single tech stack | Multiple technologies supported |
How Microservices Fit into DevOps
DevOps focuses on continuous integration and continuous delivery (CI/CD), automation, and faster software releases. Microservices align perfectly with these principles:
- CI/CD & Automation: Since microservices are independent, teams can continuously integrate and deploy changes without downtime.
- Containerization: Tools like Docker and Kubernetes simplify microservices deployment and orchestration.
- Scalability: Microservices enable dynamic scaling, optimizing resources efficiently.
- Monitoring & Logging: Tools like Prometheus, Grafana, and ELK stack help monitor microservices health.
Real-World Applications of Microservices
Many tech giants use microservices to enhance their services:
1. Netflix
Netflix adopted microservices to handle millions of users. Every function—streaming, user authentication, recommendations—operates as an independent microservice.
2. Amazon
Amazon transformed from a monolithic structure to microservices to improve its e-commerce platform’s scalability and efficiency.
3. Uber
Uber’s ride-booking system utilizes microservices to manage pricing, driver-rider matching, notifications, and payments.
Step-by-Step Guide: Deploying a Simple Microservices Application
Prerequisites:
- Basic knowledge of Docker and Kubernetes
- Installed Docker and Kubernetes (Minikube for local testing)
Step 1: Create Two Simple Microservices (Node.js Example)
Service 1: User API
const express = require('express');
const app = express();
app.get('/user', (req, res) => {
res.json({ name: 'Yash', role: 'DevOps Engineer' });
});
app.listen(3000, () => console.log('User API running on port 3000'));
Service 2: Product API
const express = require('express');
const app = express();
app.get('/product', (req, res) => {
res.json({ product: 'Laptop', price: 75000 });
});
app.listen(4000, () => console.log('Product API running on port 4000'));
Step 2: Dockerizing the Microservices
Create a Dockerfile
for each service:
FROM node:14
WORKDIR /app
COPY package.json .
RUN npm install
COPY . .
CMD ["node", "index.js"]
EXPOSE 3000
Build and run the containers:
docker build -t user-api .
docker run -p 3000:3000 user-api
Step 3: Deploying to Kubernetes
Create a Kubernetes Deployment YAML file:
apiVersion: apps/v1
kind: Deployment
metadata:
name: user-api
spec:
replicas: 2
selector:
matchLabels:
app: user-api
template:
metadata:
labels:
app: user-api
spec:
containers:
- name: user-api
image: user-api:latest
ports:
- containerPort: 3000
Apply the deployment:
kubectl apply -f user-api-deployment.yaml
Common Mistakes & Best Practices
Common Mistakes:
- Tightly Coupled Services: If microservices depend too much on each other, they lose their independent nature.
- Lack of Monitoring: Without proper observability, debugging becomes difficult.
- Incorrect API Versioning: Changes in APIs should be backward-compatible to avoid breaking dependent services.
Best Practices:
- Use API Gateways: Manage requests efficiently using API gateways like Kong or Nginx.
- Automate Deployment: Use CI/CD tools like Jenkins, GitHub Actions, or ArgoCD.
- Ensure Security: Implement OAuth, JWT, and other security measures.
- Optimize Database Strategy: Choose the right database (SQL vs. NoSQL) based on service needs.
Conclusion
Microservices play a vital role in modern DevOps, enabling fast, scalable, and efficient software development. By breaking applications into smaller services, teams can deploy updates faster, improve resilience, and scale effectively.
Are you working on a microservices project? Share your experience in the comments! If you’re new, start experimenting with simple microservices and explore tools like Docker, Kubernetes, and CI/CD pipelines.