MVVM Directory Structure for Point of Sale (POS) System
Here’s a standard and scalable directory structure for a Point of Sale (POS) system using MVVM architecture in Swift, with clean separation of concerns and real-world modularity: POSApp/ │ ├── App/ │ ├── AppDelegate.swift │ ├── SceneDelegate.swift │ └── Environment/ │ └── AppEnvironment.swift │ ├── Coordinators/ │ ├── AppCoordinator.swift │ └── Modules/ │ ├── AuthCoordinator.swift │ ├── ProductCoordinator.swift │ ├── CartCoordinator.swift │ └── CheckoutCoordinator.swift │ ├── Modules/ │ ├── Auth/ │ │ ├── View/ │ │ ├── ViewModel/ │ │ ├── Model/ │ │ └── Service/ │ │ │ ├── Product/ │ │ ├── View/ │ │ ├── ViewModel/ │ │ ├── Model/ │ │ └── Service/ │ │ │ ├── Cart/ │ │ ├── View/ │ │ ├── ViewModel/ │ │ ├── Model/ │ │ └── Service/ │ │ │ ├── Checkout/ │ │ ├── View/ │ │ ├── ViewModel/ │ │ ├── Model/ │ │ └── Service/ │ │ │ └── Dashboard/ ← For reporting/sales summary │ ├── View/ │ ├── ViewModel/ │ ├── Model/ │ └── Service/ │ ├── Services/ │ ├── API/ │ │ ├── APIClient.swift │ │ └── Endpoints.swift │ ├── Persistence/ │ │ ├── CoreDataStack.swift │ │ └── LocalStorage.swift │ └── Payment/ │ └── QRPaymentService.swift │ ├── Helpers/ │ ├── FormatterHelper.swift │ ├── Validator.swift │ └── ImagePickerHelper.swift │ ├── Components/ │ ├── Common/ │ │ ├── POSButton.swift │ │ └── POSLabel.swift │ └── ReusableViews/ │ └── ProductCardView.swift │ ├── Resources/ │ ├── Assets.xcassets │ ├── Localizable.strings │ └── Fonts/ │ ├── Extensions/ │ ├── UIView+Ext.swift │ ├── String+Ext.swift │ └── UIColor+Ext.swift │ ├── Bindings/ │ └── ReactiveBinder.swift (for RxSwift, Combine, or custom bindings) │ ├── Middlewares/ │ └── AuthMiddleware.swift │ ├── Protocols/ │ └── Coordinatable.swift │ ├── Utils/ │ └── Logger.swift │ └── Tests/ ├── Unit/ └── UI/ Key Principles: Modularized per feature: Each Module/ has its own MVC elements. MVVM: Clear separation of View, ViewModel, and Model. Coordinator Pattern: Clean navigation handling. Scalable: Easy to expand for inventory, employee management, reporting, etc.
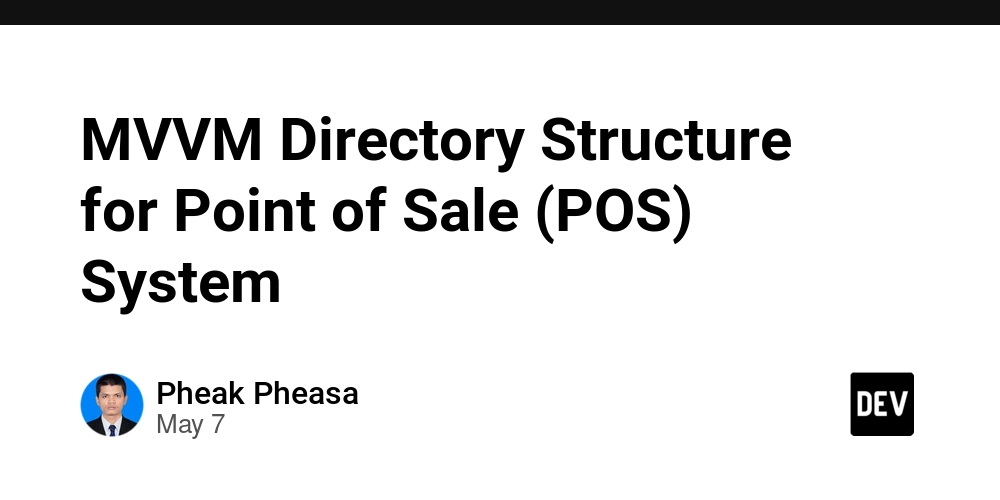
Here’s a standard and scalable directory structure for a Point of Sale (POS) system using MVVM architecture in Swift, with clean separation of concerns and real-world modularity:
POSApp/
│
├── App/
│ ├── AppDelegate.swift
│ ├── SceneDelegate.swift
│ └── Environment/
│ └── AppEnvironment.swift
│
├── Coordinators/
│ ├── AppCoordinator.swift
│ └── Modules/
│ ├── AuthCoordinator.swift
│ ├── ProductCoordinator.swift
│ ├── CartCoordinator.swift
│ └── CheckoutCoordinator.swift
│
├── Modules/
│ ├── Auth/
│ │ ├── View/
│ │ ├── ViewModel/
│ │ ├── Model/
│ │ └── Service/
│ │
│ ├── Product/
│ │ ├── View/
│ │ ├── ViewModel/
│ │ ├── Model/
│ │ └── Service/
│ │
│ ├── Cart/
│ │ ├── View/
│ │ ├── ViewModel/
│ │ ├── Model/
│ │ └── Service/
│ │
│ ├── Checkout/
│ │ ├── View/
│ │ ├── ViewModel/
│ │ ├── Model/
│ │ └── Service/
│ │
│ └── Dashboard/ ← For reporting/sales summary
│ ├── View/
│ ├── ViewModel/
│ ├── Model/
│ └── Service/
│
├── Services/
│ ├── API/
│ │ ├── APIClient.swift
│ │ └── Endpoints.swift
│ ├── Persistence/
│ │ ├── CoreDataStack.swift
│ │ └── LocalStorage.swift
│ └── Payment/
│ └── QRPaymentService.swift
│
├── Helpers/
│ ├── FormatterHelper.swift
│ ├── Validator.swift
│ └── ImagePickerHelper.swift
│
├── Components/
│ ├── Common/
│ │ ├── POSButton.swift
│ │ └── POSLabel.swift
│ └── ReusableViews/
│ └── ProductCardView.swift
│
├── Resources/
│ ├── Assets.xcassets
│ ├── Localizable.strings
│ └── Fonts/
│
├── Extensions/
│ ├── UIView+Ext.swift
│ ├── String+Ext.swift
│ └── UIColor+Ext.swift
│
├── Bindings/
│ └── ReactiveBinder.swift (for RxSwift, Combine, or custom bindings)
│
├── Middlewares/
│ └── AuthMiddleware.swift
│
├── Protocols/
│ └── Coordinatable.swift
│
├── Utils/
│ └── Logger.swift
│
└── Tests/
├── Unit/
└── UI/
Key Principles:
- Modularized per feature: Each Module/ has its own MVC elements.
- MVVM: Clear separation of View, ViewModel, and Model.
- Coordinator Pattern: Clean navigation handling.
- Scalable: Easy to expand for inventory, employee management, reporting, etc.