Top 5 JavaScript Unit Testing Frameworks
Unit testing is a fundamental practice in software development that focuses on verifying the functionality of small, isolated units of code, typically individual functions or methods. In the context of JavaScript, unit testing frameworks are tools designed to make it easier for developers to write and run tests on their JavaScript code to ensure that each unit performs as expected. Unit tests are essential for maintaining code quality, catching bugs early, and providing documentation for the expected behavior of your functions. By automating the testing process, JavaScript unit testing frameworks can save time, improve collaboration, and boost confidence when making changes to the codebase. This article introduces you to some of the most popular JavaScript unit testing frameworks, explaining their features, how they work, and why you should consider using them in your projects. Why Use JavaScript Unit Testing Frameworks? Unit testing frameworks provide a structured way to write and execute tests. They bring several benefits: Error Detection: Unit tests catch errors early by testing individual pieces of functionality, helping developers identify problems quickly. Improved Code Quality: Automated tests encourage cleaner, more modular code, as it needs to be structured in a way that is testable. Faster Development: With tests in place, developers can make changes to code without the fear of breaking existing functionality, speeding up the development process. Documentation: Unit tests serve as documentation for the expected behavior of code, making it easier for new developers to understand how specific functions should behave. Refactoring Confidence: Unit testing provides confidence when refactoring code. As long as the tests pass, developers know that the refactor didn’t break any functionality. Popular JavaScript Unit Testing Frameworks Here’s an introduction to some of the most widely used JavaScript unit testing frameworks: 1. Jest Jest is one of the most popular JavaScript testing frameworks. Originally created by Facebook, it has become the default testing tool for React applications. However, Jest can be used for testing any JavaScript project and is highly favored for its simplicity, speed, and powerful features. Key Features: Zero Configuration: Jest requires no configuration to get started, making it easy for new developers to integrate it into their projects. Snapshot Testing: Jest allows for snapshot testing, where the output of a component or function is saved and compared over time to catch unintended changes. Built-in Mocking: Jest comes with built-in functions to mock dependencies and isolate tests, such as jest.fn() for mock functions and jest.mock() for mocking modules. Code Coverage: Jest provides built-in code coverage reports, so you can track how much of your code is covered by tests. Parallel Test Execution: Jest runs tests in parallel, which helps to speed up test execution. Best For: Unit tests, integration tests, and React component testing. 2. Mocha Mocha is a feature-rich JavaScript testing framework that is commonly used in Node.js environments. It is known for its flexibility and supports a wide range of assertion libraries, reporters, and plugins. Key Features: Flexible Assertion Libraries: Mocha allows you to use various assertion libraries such as Chai, Expect.js, or Should.js. Asynchronous Testing: Mocha supports testing asynchronous code, which is useful for testing code that interacts with databases, APIs, or external services. Before/After Hooks: Mocha provides lifecycle hooks (before, beforeEach, afterEach, and after) to set up and clean up test environments before and after each test or test suite. Customizable: Mocha is highly customizable, offering various ways to run tests and output results. Best For: Unit tests, Node.js applications, and testing asynchronous code. 3. Jasmine Jasmine is a behavior-driven development (BDD) testing framework for JavaScript. It provides a rich syntax for writing human-readable tests and comes with built-in support for spies, mocks, and stubs. Key Features: BDD Syntax: Jasmine uses a natural language syntax for writing tests. The syntax reads like plain English, making tests easy to understand. No Dependencies: Jasmine works out of the box without the need for additional assertion libraries or plugins. Spies, Mocks, and Stubs: Jasmine has built-in support for spies (to track function calls), mocks (to simulate dependencies), and stubs (to replace functions with controlled behavior). Asynchronous Testing: Jasmine supports asynchronous testing using done() callbacks or returning promises. Best For: Behavior-driven testing and projects that require a BDD approach. 4. Ava Ava is a minimalistic JavaScript test runner that is known for its speed and simplicity. It runs tests concurrently, which makes it faster than some other frameworks, especially for large test
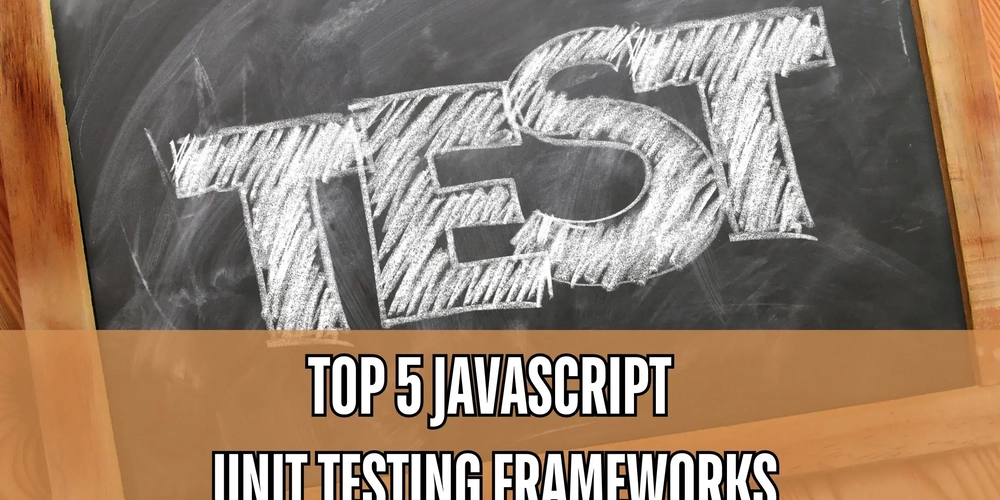
Unit testing is a fundamental practice in software development that focuses on verifying the functionality of small, isolated units of code, typically individual functions or methods. In the context of JavaScript, unit testing frameworks are tools designed to make it easier for developers to write and run tests on their JavaScript code to ensure that each unit performs as expected.
Unit tests are essential for maintaining code quality, catching bugs early, and providing documentation for the expected behavior of your functions. By automating the testing process, JavaScript unit testing frameworks can save time, improve collaboration, and boost confidence when making changes to the codebase.
This article introduces you to some of the most popular JavaScript unit testing frameworks, explaining their features, how they work, and why you should consider using them in your projects.
Why Use JavaScript Unit Testing Frameworks?
Unit testing frameworks provide a structured way to write and execute tests. They bring several benefits:
Error Detection: Unit tests catch errors early by testing individual pieces of functionality, helping developers identify problems quickly.
Improved Code Quality: Automated tests encourage cleaner, more modular code, as it needs to be structured in a way that is testable.
Faster Development: With tests in place, developers can make changes to code without the fear of breaking existing functionality, speeding up the development process.
Documentation: Unit tests serve as documentation for the expected behavior of code, making it easier for new developers to understand how specific functions should behave.
Refactoring Confidence: Unit testing provides confidence when refactoring code. As long as the tests pass, developers know that the refactor didn’t break any functionality.
Popular JavaScript Unit Testing Frameworks
Here’s an introduction to some of the most widely used JavaScript unit testing frameworks:
1. Jest
Jest is one of the most popular JavaScript testing frameworks. Originally created by Facebook, it has become the default testing tool for React applications. However, Jest can be used for testing any JavaScript project and is highly favored for its simplicity, speed, and powerful features.
Key Features:
Zero Configuration: Jest requires no configuration to get started, making it easy for new developers to integrate it into their projects.
Snapshot Testing: Jest allows for snapshot testing, where the output of a component or function is saved and compared over time to catch unintended changes.
Built-in Mocking: Jest comes with built-in functions to mock dependencies and isolate tests, such as jest.fn() for mock functions and jest.mock() for mocking modules.
Code Coverage: Jest provides built-in code coverage reports, so you can track how much of your code is covered by tests.
Parallel Test Execution: Jest runs tests in parallel, which helps to speed up test execution.
Best For: Unit tests, integration tests, and React component testing.
2. Mocha
Mocha is a feature-rich JavaScript testing framework that is commonly used in Node.js environments. It is known for its flexibility and supports a wide range of assertion libraries, reporters, and plugins.
Key Features:
Flexible Assertion Libraries: Mocha allows you to use various assertion libraries such as Chai, Expect.js, or Should.js.
Asynchronous Testing: Mocha supports testing asynchronous code, which is useful for testing code that interacts with databases, APIs, or external services.
Before/After Hooks: Mocha provides lifecycle hooks (before, beforeEach, afterEach, and after) to set up and clean up test environments before and after each test or test suite.
Customizable: Mocha is highly customizable, offering various ways to run tests and output results.
Best For: Unit tests, Node.js applications, and testing asynchronous code.
3. Jasmine
Jasmine is a behavior-driven development (BDD) testing framework for JavaScript. It provides a rich syntax for writing human-readable tests and comes with built-in support for spies, mocks, and stubs.
Key Features:
BDD Syntax: Jasmine uses a natural language syntax for writing tests. The syntax reads like plain English, making tests easy to understand.
No Dependencies: Jasmine works out of the box without the need for additional assertion libraries or plugins.
Spies, Mocks, and Stubs: Jasmine has built-in support for spies (to track function calls), mocks (to simulate dependencies), and stubs (to replace functions with controlled behavior).
Asynchronous Testing: Jasmine supports asynchronous testing using done() callbacks or returning promises.
Best For: Behavior-driven testing and projects that require a BDD approach.
4. Ava
Ava is a minimalistic JavaScript test runner that is known for its speed and simplicity. It runs tests concurrently, which makes it faster than some other frameworks, especially for large test suites.
Key Features:
Concurrent Testing: Ava runs tests in parallel, which speeds up test execution.
Built-in Assertions: Ava comes with built-in assertion functions, which simplifies the setup process.
Promise-Based: Ava is designed to work with async/await and promises, making it ideal for testing asynchronous code.
No Global Variables: Ava runs tests in isolated environments, preventing tests from interfering with each other.
Best For: Fast, minimalistic testing of JavaScript and Node.js applications.
5. QUnit
QUnit is a powerful JavaScript testing framework primarily used for testing code written for the jQuery ecosystem, though it can be used for any JavaScript codebase. It is known for its simplicity and clear, actionable feedback.
Key Features:
Simple and Lightweight: QUnit is a very lightweight framework, which makes it easy to get started.
Asynchronous Testing: QUnit supports asynchronous testing with promises and callbacks.
Test Suites: Tests are organized into suites, making it easy to group related tests together.
Plugin Support: It has a set of plugins for additional functionality, such as mocking or running tests in a browser environment.
Best For: jQuery projects, small JavaScript applications, or if you need a minimal framework.
Choosing the Right Unit Testing Framework
Choosing the right unit testing framework depends on various factors, such as the nature of your project, the tools you’re already using, and the type of tests you need to perform. Here’s a quick guide to help:
For React or modern JavaScript apps: Jest is a top choice due to its zero configuration, built-in mocking, and snapshot testing.
For Node.js applications or flexible setups: Mocha provides great flexibility and can be paired with many assertion libraries like Chai.
For behavior-driven development: Jasmine is ideal, especially if you’re writing tests that are highly descriptive.
For minimal and fast testing: Ava offers fast test execution and minimal setup.
For simple or jQuery-based projects: QUnit is lightweight and great for small projects.
JavaScript unit testing frameworks play an essential role in ensuring the reliability and maintainability of your codebase. By using these frameworks, you can automate the testing process, catch bugs early, and ensure that your JavaScript code works as expected. Whether you’re building a small project or a large-scale application, incorporating unit tests into your development workflow will help improve the quality of your code and reduce the chances of issues arising in production.
The most popular JavaScript unit testing frameworks — Jest, Mocha, Jasmine, Ava, and QUnit — each have their strengths and are suited for different types of projects. Choose the one that aligns with your project needs and start writing automated tests to ensure your code stays functional and maintainable.
You can learn more about “UNIT TESTING” in my Medium blog: https://medium.com/@CodingAdventure