Latency and Throughput: Optimizing Application Performance
When discussing latency and throughput, they are often framed in the context of networking. However, these metrics are just as critical when evaluating application and server performance. In applications, latency measures how quickly a request is processed and responded to, while throughput reflects how many requests or transactions a system can handle within a given timeframe. Optimizing both is essential to ensure fast, efficient operations, especially in high-demand environments. Understanding how these metrics apply to applications—and how to balance them—can drastically improve user experience and system scalability. Latency Definition: Latency is the time it takes for a request to be processed and a response to be returned. It refers to the delay between a user action (like clicking a button) and the system’s response (like loading a page). It is usually measured in milliseconds (ms). Key Factors: Processing Time: The time the server spends executing a request, such as querying databases, performing computations, or communicating with other services. Resource Contention: High CPU, memory, or I/O usage increases latency as tasks compete for shared resources. Application Architecture: Service-oriented designs like microservices may introduce additional latency, as multiple services might need to communicate to fulfill a request. Garbage Collection: Managed runtime environments (e.g., Java, .NET) may pause for garbage collection, temporarily increasing latency. Impact: Low latency is crucial for smooth user interactions, especially in real-time or interactive applications. High latency causes delays, slow page loads, and degraded user experience. Throughput Definition: Throughput refers to the number of requests or transactions a system can handle over time, typically measured in requests per second (RPS) or transactions per second (TPS). Key Factors: Concurrency: The server’s ability to handle multiple requests at the same time, influenced by thread management, asynchronous processing, and non-blocking I/O. Hardware Capacity: More powerful hardware (e.g., more CPU cores, faster memory) enables higher throughput. Database Performance: Slow or inefficient database queries can become a bottleneck, limiting throughput. I/O Bound Operations: Disk and network operations, such as file reads or external API calls, can slow throughput if not optimized. Impact: High throughput is critical for systems with many users or high transaction volumes. Low throughput results in bottlenecks, limiting the system’s ability to scale effectively. Balancing Latency and Throughput Trade-offs: Optimizing for low latency may require dedicating more resources to each request, reducing the system’s capacity to handle large numbers of requests. Focusing on high throughput by handling many concurrent requests can sometimes increase individual request latency, as tasks may be queued or processed more slowly. Performance Tuning CPU-Heavy Tasks: Refactor code with complex computations or inefficient algorithms. Using parallel processing or more efficient data structures can lower CPU usage, improving both response times and capacity. I/O Bound Operations: Convert blocking I/O calls, such as database queries or file reads, to asynchronous or batched processes to reduce wait times and increase throughput. Memory Leaks: Address memory leaks by ensuring proper resource management, such as using object pooling or lazy initialization. These techniques help avoid excessive memory consumption, which can degrade performance. Minimize Lock Contention: In multi-threaded environments, refactor to reduce lock contention, allowing more requests to be processed concurrently without bottlenecks. Choosing the Right Garbage Collection: Use the appropriate garbage collection (GC) algorithm for your application. For instance, switching to a low-latency GC like G1 or ZGC in Java can help reduce pauses during memory cleanup, improving both latency and throughput in high-demand applications.
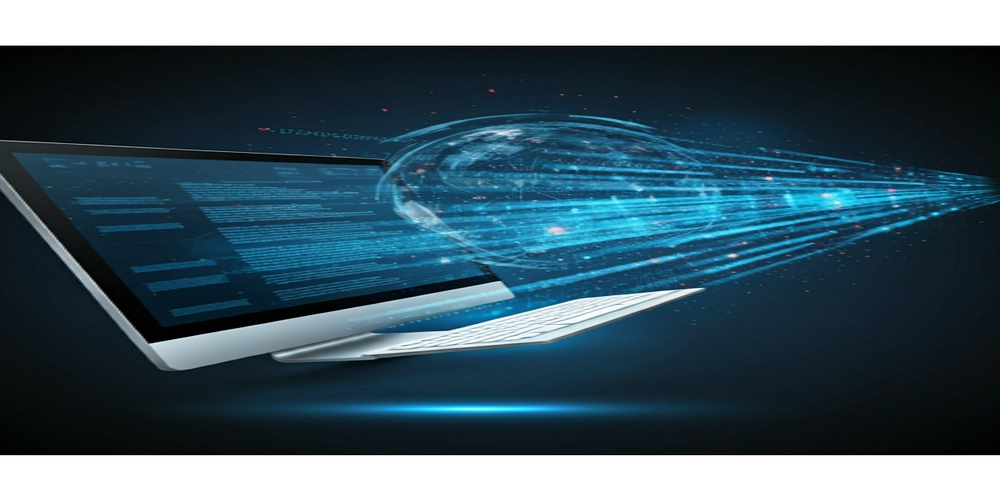
When discussing latency and throughput, they are often framed in the context of networking. However, these metrics are just as critical when evaluating application and server performance. In applications, latency measures how quickly a request is processed and responded to, while throughput reflects how many requests or transactions a system can handle within a given timeframe. Optimizing both is essential to ensure fast, efficient operations, especially in high-demand environments. Understanding how these metrics apply to applications—and how to balance them—can drastically improve user experience and system scalability.
Latency
Definition: Latency is the time it takes for a request to be processed and a response to be returned. It refers to the delay between a user action (like clicking a button) and the system’s response (like loading a page). It is usually measured in milliseconds (ms).
Key Factors:
- Processing Time: The time the server spends executing a request, such as querying databases, performing computations, or communicating with other services.
- Resource Contention: High CPU, memory, or I/O usage increases latency as tasks compete for shared resources.
- Application Architecture: Service-oriented designs like microservices may introduce additional latency, as multiple services might need to communicate to fulfill a request.
- Garbage Collection: Managed runtime environments (e.g., Java, .NET) may pause for garbage collection, temporarily increasing latency.
Impact: Low latency is crucial for smooth user interactions, especially in real-time or interactive applications. High latency causes delays, slow page loads, and degraded user experience.
Throughput
Definition: Throughput refers to the number of requests or transactions a system can handle over time, typically measured in requests per second (RPS) or transactions per second (TPS).
Key Factors:
- Concurrency: The server’s ability to handle multiple requests at the same time, influenced by thread management, asynchronous processing, and non-blocking I/O.
- Hardware Capacity: More powerful hardware (e.g., more CPU cores, faster memory) enables higher throughput. Database Performance: Slow or inefficient database queries can become a bottleneck, limiting throughput.
- I/O Bound Operations: Disk and network operations, such as file reads or external API calls, can slow throughput if not optimized.
Impact: High throughput is critical for systems with many users or high transaction volumes. Low throughput results in bottlenecks, limiting the system’s ability to scale effectively.
Balancing Latency and Throughput
Trade-offs:
Optimizing for low latency may require dedicating more resources to each request, reducing the system’s capacity to handle large numbers of requests.
Focusing on high throughput by handling many concurrent requests can sometimes increase individual request latency, as tasks may be queued or processed more slowly.
Performance Tuning
- CPU-Heavy Tasks: Refactor code with complex computations or inefficient algorithms. Using parallel processing or more efficient data structures can lower CPU usage, improving both response times and capacity.
- I/O Bound Operations: Convert blocking I/O calls, such as database queries or file reads, to asynchronous or batched processes to reduce wait times and increase throughput.
- Memory Leaks: Address memory leaks by ensuring proper resource management, such as using object pooling or lazy initialization. These techniques help avoid excessive memory consumption, which can degrade performance.
- Minimize Lock Contention: In multi-threaded environments, refactor to reduce lock contention, allowing more requests to be processed concurrently without bottlenecks.
- Choosing the Right Garbage Collection: Use the appropriate garbage collection (GC) algorithm for your application. For instance, switching to a low-latency GC like G1 or ZGC in Java can help reduce pauses during memory cleanup, improving both latency and throughput in high-demand applications.