Java Exception Error Enumerations Anti-pattern
Over the years I've many times seen folks enumerate error codes for exceptions in Java. I generally have felt this leads to more tedious code and does not provide value. For example, there will be an enum like this ErrorType, often times with a numeric errorCode, which I find additionally egregious. package so.example; public enum ErrorType { INVALID_DATA_FORMAT(1000), MISSING_REQUIRED_FIELD(1010), MODIFY_DISABLED(2020), DUPLICATE_MERCHANT(2050), UNKNOWN_RESELLER(2051), XML_PARSING_FAILURE(2999), SYSTEM_ERROR(3000), AND_HUNDREDS_MORE_LIKE_THIS(4444); private final int errorCode; ErrorType(final int errorCode) { this.errorCode = errorCode; } public int getErrorCode() { return errorCode; } } Then there will be an Exception subclass which requires this enum as a construction parameter, like package so.example; public class SoExampleException extends GenericExampleException { public SoExampleException(ErrorType subtype, String message) { super(subtype, message); } public SoExampleException(ErrorType subtype, Exception causedBy) { super(subtype, causedBy); } } with code calling it like private void validate(final Request requestBody) throws SoExampleException { if (StringUtils.isBlank(requestBody.getSomeNumber())) { throw new SoExampleException(ErrorType.MISSING_REQUIRED_FIELD, "someNumber cannot be empty or null"); } } To me it feels like a type of anti-pattern because Every time some new exception condition is dreamed up, then the enumeration has to be updated. It implies callers should explicitly deal with each possible type, which makes the client exception handling tedious. To me, an enumeration should really only be used for a small set of well known values, like "North, East, South, West" or "Red, Green, Blue". Having exceptions mapped to that implies that we know exactly, forever, the small set of things that can go wrong, which feels pretentious. And the errorCode seems even more unnecessary considering this is an enumeration with a built-in ordinal. Am I off base here? Is there a good reason for this type of pattern?
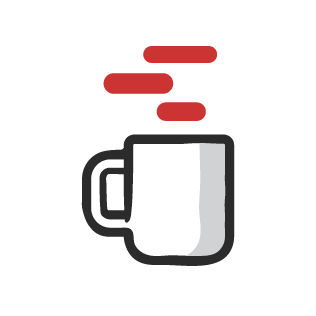
Over the years I've many times seen folks enumerate error codes for exceptions in Java. I generally have felt this leads to more tedious code and does not provide value.
For example, there will be an enum
like this ErrorType
, often times with a numeric errorCode
, which I find additionally egregious.
package so.example;
public enum ErrorType {
INVALID_DATA_FORMAT(1000),
MISSING_REQUIRED_FIELD(1010),
MODIFY_DISABLED(2020),
DUPLICATE_MERCHANT(2050),
UNKNOWN_RESELLER(2051),
XML_PARSING_FAILURE(2999),
SYSTEM_ERROR(3000),
AND_HUNDREDS_MORE_LIKE_THIS(4444);
private final int errorCode;
ErrorType(final int errorCode) {
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
}
Then there will be an Exception
subclass which requires this enum
as a construction parameter, like
package so.example;
public class SoExampleException extends GenericExampleException {
public SoExampleException(ErrorType subtype, String message) {
super(subtype, message);
}
public SoExampleException(ErrorType subtype, Exception causedBy) {
super(subtype, causedBy);
}
}
with code calling it like
private void validate(final Request requestBody) throws SoExampleException {
if (StringUtils.isBlank(requestBody.getSomeNumber())) {
throw new SoExampleException(ErrorType.MISSING_REQUIRED_FIELD, "someNumber cannot be empty or null");
}
}
To me it feels like a type of anti-pattern because
- Every time some new exception condition is dreamed up, then the enumeration has to be updated.
- It implies callers should explicitly deal with each possible type, which makes the client exception handling tedious.
- To me, an enumeration should really only be used for a small set of well known values, like "North, East, South, West" or "Red, Green, Blue". Having exceptions mapped to that implies that we know exactly, forever, the small set of things that can go wrong, which feels pretentious.
And the errorCode
seems even more unnecessary considering this is an enumeration with a built-in ordinal
.
Am I off base here? Is there a good reason for this type of pattern?