VueJS - JavaScript Framework
What is Vue? Vue is a JavaScript framework for building UIs. It builds on top of standard HTML, CSS, and JavaScript. Single-File Components In most build-tool-enabled Vue projects, we author Vue components using an HTML-like file format called Single-File Component (also known as *.vue files, abbreviated as SFC). A Vue SFC, as the name suggests, encapsulates the component's logic (JavaScript), template (HTML), and styles (CSS) in a single file. Here's the previous example, written in SFC format: import { ref } from 'vue' const count = ref(0) Count is: {{ count }} button { font-weight: bold; } API Styles Vue components can be authored in two different API styles: Options API and Composition API. Options API With Options API, we define a component's logic using an object of options such as data, methods, and mounted. Properties defined by options are exposed on this inside functions, which points to the component instance: export default { // Properties returned from data() become reactive state // and will be exposed on `this`. data() { return { count: 0 } }, // Methods are functions that mutate state and trigger updates. // They can be bound as event handlers in templates. methods: { increment() { this.count++ } }, // Lifecycle hooks are called at different stages // of a component's lifecycle. // This function will be called when the component is mounted. mounted() { console.log(`The initial count is ${this.count}.`) } } Count is: {{ count }} Composition API With Composition API, we define a component's logic using imported API functions. Here is the same component, with the exact same template, but using Composition API instead: import { ref, onMounted } from 'vue' // reactive state const count = ref(0) // functions that mutate state and trigger updates function increment() { count.value++ } // lifecycle hooks onMounted(() => { console.log(`The initial count is ${count.value}.`) }) Count is: {{ count }} *Which to choose? For learning purpose: Go with the style that looks easier to understand to you. Again, most of the core concepts are shared between the two styles. You can always pick up the other style later. For production use: Go with Options API if you are not using build tools, or plan to use Vue primarily in low-complexity scenarios, e.g. progressive enhancement. Go with Composition API + Single-File Components if you plan to build full applications with Vue.*
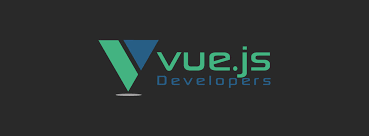
What is Vue?
Vue is a JavaScript framework for building UIs. It builds on top of standard HTML, CSS, and JavaScript.
Single-File Components
In most build-tool-enabled Vue projects, we author Vue components using an HTML-like file format called Single-File Component (also known as *.vue files, abbreviated as SFC). A Vue SFC, as the name suggests, encapsulates the component's logic (JavaScript), template (HTML), and styles (CSS) in a single file. Here's the previous example, written in SFC format:
API Styles
Vue components can be authored in two different API styles: Options API and Composition API.
Options API
With Options API, we define a component's logic using an object of options such as data, methods, and mounted. Properties defined by options are exposed on this inside functions, which points to the component instance:
Composition API
With Composition API, we define a component's logic using imported API functions.
Here is the same component, with the exact same template, but using Composition API instead:
*Which to choose?
For learning purpose:
Go with the style that looks easier to understand to you. Again, most of the core concepts are shared between the two styles. You can always pick up the other style later.-
For production use:
- Go with Options API if you are not using build tools, or plan to use Vue primarily in low-complexity scenarios, e.g. progressive enhancement.
- Go with Composition API + Single-File Components if you plan to build full applications with Vue.*