Understanding Storage Systems in React
Introduction React applications must often store and manage data efficiently to provide a seamless user experience. This storage can be temporary (in-memory), persistent (saved across sessions), or external (server-side storage). In this article, we'll explore different storage systems in React, their use cases, and how to implement them. 1. In-Memory State Management In-memory storage refers to data stored within a component's state or in global state management tools like React Context, Redux, or Zustand. This data is lost when the page refreshes. Example using React State: import { useState } from "react"; function Counter() { const [count, setCount] = useState(0); return ( Count: {count} setCount(count + 1)}>Increase ); } When to Use When data needs to be temporary (e.g., form inputs, UI state) When working with component-specific data 2. Local Storage Local Storage is a browser-based storage system that persists data across sessions. It can store up to 5MB of data per domain and is synchronous. Example: function App() { const [name, setName] = useState(localStorage.getItem("name") || ""); const saveName = () => { localStorage.setItem("name", name); }; return ( setName(e.target.value)} /> Save ); } When to Use Storing small amounts of persistent data (e.g., user preferences) Storing non-sensitive data that needs to persist across page reloads 3. Session Storage Session Storage is similar to Local Storage but only persists while the browser tab is open. Example: sessionStorage.setItem("sessionKey", "Hello World"); console.log(sessionStorage.getItem("sessionKey")); // Outputs: Hello World When to Use Temporary storage needed only while the session is active (e.g., form drafts, authentication states during a single session) 4. Cookies Cookies store small amounts of data that can be sent along with HTTP requests, making them useful for authentication. Example using JavaScript: document.cookie = "username=John; expires=Fri, 31 Dec 2025 23:59:59 GMT; path=/"; console.log(document.cookie); When to Use Storing authentication tokens Handling cross-session user preferences 5. IndexedDB IndexedDB is a low-level NoSQL database built into browsers, allowing efficient storage of large amounts of structured data. Example using IndexedDB (with idb library): import { openDB } from 'idb'; const dbPromise = openDB('myDatabase', 1, { upgrade(db) { db.createObjectStore('store'); }, }); async function saveData(key, value) { const db = await dbPromise; await db.put('store', value, key); } When to Use Storing large datasets (e.g., offline applications, complex data structures) 6. Server-Side Storage For large-scale applications, storing data on a backend (e.g., Firebase, PostgreSQL, MongoDB) is essential. Fetching data from an API is a common approach. Example using Fetch API: fetch("https://api.example.com/data") .then(response => response.json()) .then(data => console.log(data)); When to Use Storing user-generated content (e.g., posts, messages) Managing shared or sensitive data Choosing the Right Storage Option Conclusion Understanding the different storage systems in React is crucial for building efficient applications. Choosing the right method depends on factors such as data persistence, security, and storage capacity. By leveraging these storage options effectively, developers can create robust and user-friendly React applications.
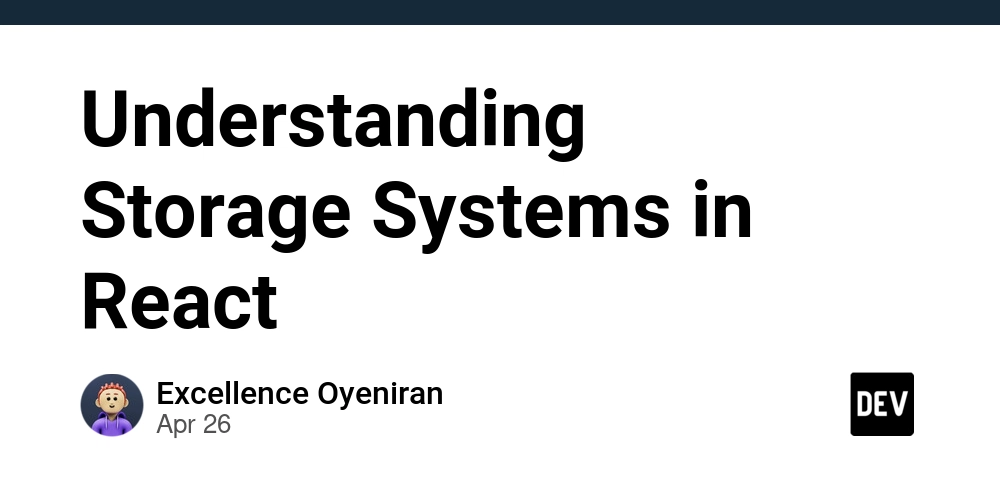
Introduction
React applications must often store and manage data efficiently to provide a seamless user experience. This storage can be temporary (in-memory), persistent (saved across sessions), or external (server-side storage). In this article, we'll explore different storage systems in React, their use cases, and how to implement them.
1. In-Memory State Management
In-memory storage refers to data stored within a component's state or in global state management tools like React Context, Redux, or Zustand. This data is lost when the page refreshes.
Example using React State:
import { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
return (
Count: {count}
);
}
When to Use
- When data needs to be temporary (e.g., form inputs, UI state)
- When working with component-specific data
2. Local Storage
Local Storage is a browser-based storage system that persists data across sessions. It can store up to 5MB of data per domain and is synchronous.
Example:
function App() {
const [name, setName] = useState(localStorage.getItem("name") || "");
const saveName = () => {
localStorage.setItem("name", name);
};
return (
setName(e.target.value)}
/>
);
}
When to Use
- Storing small amounts of persistent data (e.g., user preferences)
- Storing non-sensitive data that needs to persist across page reloads
3. Session Storage
Session Storage is similar to Local Storage but only persists while the browser tab is open.
Example:
sessionStorage.setItem("sessionKey", "Hello World");
console.log(sessionStorage.getItem("sessionKey")); // Outputs: Hello World
When to Use
- Temporary storage needed only while the session is active (e.g., form drafts, authentication states during a single session)
4. Cookies
Cookies store small amounts of data that can be sent along with HTTP requests, making them useful for authentication.
Example using JavaScript:
document.cookie = "username=John; expires=Fri, 31 Dec 2025 23:59:59 GMT; path=/";
console.log(document.cookie);
When to Use
- Storing authentication tokens
- Handling cross-session user preferences
5. IndexedDB
IndexedDB is a low-level NoSQL database built into browsers, allowing efficient storage of large amounts of structured data.
Example using IndexedDB (with idb library):
import { openDB } from 'idb';
const dbPromise = openDB('myDatabase', 1, {
upgrade(db) {
db.createObjectStore('store');
},
});
async function saveData(key, value) {
const db = await dbPromise;
await db.put('store', value, key);
}
When to Use
- Storing large datasets (e.g., offline applications, complex data structures)
6. Server-Side Storage
For large-scale applications, storing data on a backend (e.g., Firebase, PostgreSQL, MongoDB) is essential. Fetching data from an API is a common approach.
Example using Fetch API:
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log(data));
When to Use
- Storing user-generated content (e.g., posts, messages)
- Managing shared or sensitive data
- Choosing the Right Storage Option
Conclusion
Understanding the different storage systems in React is crucial for building efficient applications. Choosing the right method depends on factors such as data persistence, security, and storage capacity. By leveraging these storage options effectively, developers can create robust and user-friendly React applications.