Understanding Higher Order Components in React: A Comprehensive Guide
Understanding Higher Order Components in React In the evolving landscape of React development, design patterns play a crucial role in creating maintainable, scalable applications. Among these patterns, Higher Order Components (HOCs) stand out as one of the most powerful and elegant solutions for component logic reuse. While modern React has introduced hooks as an alternative approach, HOCs remain relevant and valuable in many scenarios. What Are Higher Order Components? At its core, a Higher Order Component is not a component itself but a function that takes a component and returns a new enhanced component. The concept is inspired by higher-order functions in functional programming—functions that operate on other functions by taking them as arguments or returning them. The basic structure of a HOC follows this pattern: function withFeature(WrappedComponent) { return function EnhancedComponent(props) { // Additional logic here return ; }; } This pattern allows you to abstract component logic, state manipulation, and prop transformations into reusable functions that can be applied to different components. The Theoretical Foundation of HOCs HOCs embody several fundamental principles of software development and React philosophy: 1. Composition Over Inheritance React favors composition over inheritance for building component hierarchies. HOCs extend this philosophy to behavior sharing. Instead of creating complex inheritance trees, HOCs compose behavior by wrapping components with additional functionality. 2. Single Responsibility Principle Each HOC should focus on a single aspect of functionality. This adherence to the single responsibility principle makes HOCs easier to understand, test, and maintain. For example, one HOC might handle data fetching, while another manages authentication, and a third provides theming. 3. Pure Function Concept Ideally, HOCs should operate as pure functions—given the same input (component and props), they should always produce the same output (enhanced component) without side effects. This makes their behavior predictable and easier to reason about. 4. Separation of Concerns HOCs enable clear separation between UI rendering logic and cross-cutting concerns like data fetching, state management, and authentication. This separation results in more focused components that are easier to develop and maintain. Anatomy of a Higher Order Component Let's examine the essential aspects of HOCs: The Wrapper Pattern The most common HOC implementation uses the wrapper pattern, where the HOC returns a new component that renders the wrapped component with additional props: function withDataFetching(WrappedComponent, dataSource) { return function WithDataFetching(props) { const [data, setData] = useState([]); const [isLoading, setIsLoading] = useState(true); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await fetch(dataSource); const result = await response.json(); setData(result); setIsLoading(false); } catch (err) { setError(err); setIsLoading(false); } }; fetchData(); }, []); return ( ); }; } This HOC abstracts away the data fetching logic, allowing multiple components to reuse this behavior without code duplication. Prop Manipulation HOCs can add, modify, or filter props before passing them to the wrapped component: function withUser(WrappedComponent) { return function WithUser(props) { const user = getCurrentUser(); // Hypothetical function to get current user // Add the user to the props passed to the wrapped component return ; }; } Render Hijacking HOCs can control what gets rendered by the enhanced component, enabling conditional rendering based on various factors: function withAuth(WrappedComponent) { return function WithAuth(props) { const isAuthenticated = checkAuthStatus(); // Hypothetical auth check if (!isAuthenticated) { return ; } return ; }; } HOCs in Practice: Common Use Cases Let's explore some common scenarios where HOCs prove particularly useful: Authentication and Authorization HOCs excel at protecting routes or components that require authentication: function withAuth(WrappedComponent, requiredRole = null) { return function WithAuth(props) { const { user, isLoggedIn } = useAuth(); // Hypothetical auth hook if (!isLoggedIn) { return ; } if (requiredRole && !user.roles.includes(requiredRole)) { return ; } return ; }; } // Usage const ProtectedDashboard = withAuth(Dashboard); const AdminPanel = withAuth(AdminSettings, 'admin'); Cross-Cutting Concerns HOCs are ideal
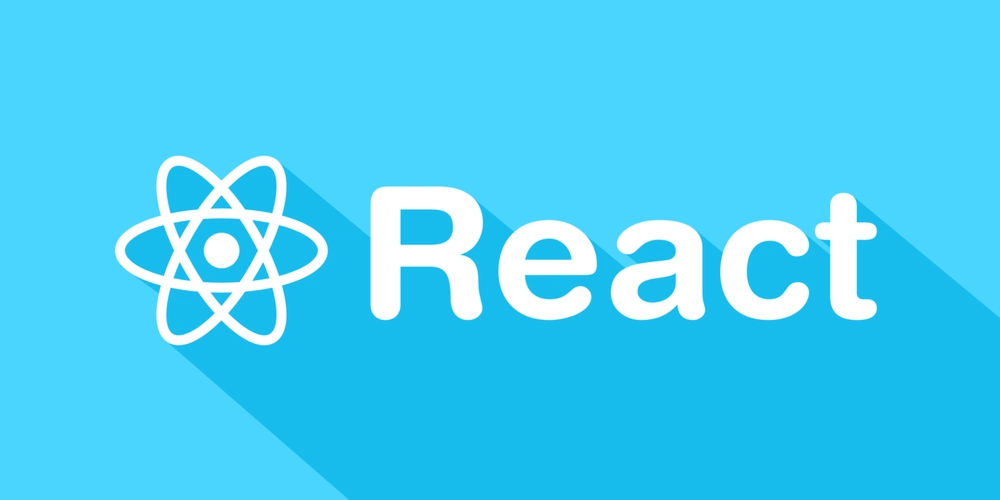
Understanding Higher Order Components in React
In the evolving landscape of React development, design patterns play a crucial role in creating maintainable, scalable applications. Among these patterns, Higher Order Components (HOCs) stand out as one of the most powerful and elegant solutions for component logic reuse. While modern React has introduced hooks as an alternative approach, HOCs remain relevant and valuable in many scenarios.
What Are Higher Order Components?
At its core, a Higher Order Component is not a component itself but a function that takes a component and returns a new enhanced component. The concept is inspired by higher-order functions in functional programming—functions that operate on other functions by taking them as arguments or returning them.
The basic structure of a HOC follows this pattern:
function withFeature(WrappedComponent) {
return function EnhancedComponent(props) {
// Additional logic here
return <WrappedComponent {...props} additionalProp={value} />;
};
}
This pattern allows you to abstract component logic, state manipulation, and prop transformations into reusable functions that can be applied to different components.
The Theoretical Foundation of HOCs
HOCs embody several fundamental principles of software development and React philosophy:
1. Composition Over Inheritance
React favors composition over inheritance for building component hierarchies. HOCs extend this philosophy to behavior sharing. Instead of creating complex inheritance trees, HOCs compose behavior by wrapping components with additional functionality.
2. Single Responsibility Principle
Each HOC should focus on a single aspect of functionality. This adherence to the single responsibility principle makes HOCs easier to understand, test, and maintain. For example, one HOC might handle data fetching, while another manages authentication, and a third provides theming.
3. Pure Function Concept
Ideally, HOCs should operate as pure functions—given the same input (component and props), they should always produce the same output (enhanced component) without side effects. This makes their behavior predictable and easier to reason about.
4. Separation of Concerns
HOCs enable clear separation between UI rendering logic and cross-cutting concerns like data fetching, state management, and authentication. This separation results in more focused components that are easier to develop and maintain.
Anatomy of a Higher Order Component
Let's examine the essential aspects of HOCs:
The Wrapper Pattern
The most common HOC implementation uses the wrapper pattern, where the HOC returns a new component that renders the wrapped component with additional props:
function withDataFetching(WrappedComponent, dataSource) {
return function WithDataFetching(props) {
const [data, setData] = useState([]);
const [isLoading, setIsLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(dataSource);
const result = await response.json();
setData(result);
setIsLoading(false);
} catch (err) {
setError(err);
setIsLoading(false);
}
};
fetchData();
}, []);
return (
<WrappedComponent
{...props}
data={data}
isLoading={isLoading}
error={error}
/>
);
};
}
This HOC abstracts away the data fetching logic, allowing multiple components to reuse this behavior without code duplication.
Prop Manipulation
HOCs can add, modify, or filter props before passing them to the wrapped component:
function withUser(WrappedComponent) {
return function WithUser(props) {
const user = getCurrentUser(); // Hypothetical function to get current user
// Add the user to the props passed to the wrapped component
return <WrappedComponent {...props} user={user} />;
};
}
Render Hijacking
HOCs can control what gets rendered by the enhanced component, enabling conditional rendering based on various factors:
function withAuth(WrappedComponent) {
return function WithAuth(props) {
const isAuthenticated = checkAuthStatus(); // Hypothetical auth check
if (!isAuthenticated) {
return <LoginPrompt />;
}
return <WrappedComponent {...props} />;
};
}
HOCs in Practice: Common Use Cases
Let's explore some common scenarios where HOCs prove particularly useful:
Authentication and Authorization
HOCs excel at protecting routes or components that require authentication:
function withAuth(WrappedComponent, requiredRole = null) {
return function WithAuth(props) {
const { user, isLoggedIn } = useAuth(); // Hypothetical auth hook
if (!isLoggedIn) {
return <Redirect to="/login" />;
}
if (requiredRole && !user.roles.includes(requiredRole)) {
return <AccessDenied />;
}
return <WrappedComponent {...props} />;
};
}
// Usage
const ProtectedDashboard = withAuth(Dashboard);
const AdminPanel = withAuth(AdminSettings, 'admin');
Cross-Cutting Concerns
HOCs are ideal for handling aspects that cut across multiple components, such as logging, analytics, or error boundaries:
function withErrorHandling(WrappedComponent) {
// Note: Error boundaries must be class components as of React 16.14
// This is one case where we still need to use a class component
// We'll create a functional HOC that uses a class component internally
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false, error: null };
}
static getDerivedStateFromError(error) {
return { hasError: true, error };
}
componentDidCatch(error, info) {
logErrorToService(error, info);
}
render() {
if (this.state.hasError) {
return <ErrorDisplay error={this.state.error} />;
}
return this.props.children;
}
}
// The HOC itself is a functional component
return function WithErrorHandling(props) {
return (
<ErrorBoundary>
<WrappedComponent {...props} />
ErrorBoundary>
);
};
}
The Philosophical Underpinnings of HOCs
Understanding HOCs at a deeper level involves appreciating several key concepts:
Inversion of Control
HOCs implement the inversion of control principle by reversing the traditional component relationship. Instead of components defining their own behaviors, HOCs provide behaviors to components. This inversion allows for more flexible and reusable code.
Declarative vs. Imperative Programming
HOCs align with React's declarative programming model. They declare what additional capabilities a component should have, rather than imperatively describing how to achieve those capabilities step by step.
Composition Chains
HOCs can be composed together to create chains of behaviors. Each HOC in the chain adds a specific capability, following the Unix philosophy of "do one thing and do it well":
// Compose multiple HOCs
const EnhancedComponent = withAuth(
withTheme(
withLogging(BaseComponent)
)
);
// Or using a compose utility for cleaner code
const enhance = compose(withAuth, withTheme, withLogging);
const EnhancedComponent = enhance(BaseComponent);
Best Practices and Common Pitfalls
To effectively use HOCs, keep these best practices in mind:
Pass Unrelated Props Through
Always pass through props that the HOC doesn't specifically need to modify:
function withFeature(WrappedComponent) {
return function(props) {
// Add feature-specific props
const featureProps = { feature: 'value' };
// Pass through all original props
return <WrappedComponent {...props} {...featureProps} />;
};
}
Use Descriptive Names
Adopt a naming convention like "with" prefix for HOCs and ensure proper display names for debugging:
function withFeature(WrappedComponent) {
const WithFeature = (props) => {
return <WrappedComponent {...props} feature="value" />;
};
// Set a descriptive display name
WithFeature.displayName = `WithFeature(${
WrappedComponent.displayName || WrappedComponent.name || 'Component'
})`;
return WithFeature;
}
Avoid Using HOCs Inside Render Methods
Creating HOCs inside render methods causes component remounts on every render:
//