How to Troubleshoot ASAN Logging Issues in C Daemons
Introduction When developing a Linux daemon utilizing AddressSanitizer (ASAN) for debugging memory issues, encountering problems with ASAN logging can be frustrating. You seem to be facing an unusual problem where ASAN fails to log crashes when detect_leaks=0, yet it works perfectly when this option is enabled. Let’s explore this issue in detail. Understanding ASAN and LeakSanitizer AddressSanitizer (ASAN) is a memory error detector that helps identify issues like buffer overflows, use-after-free errors, and memory leaks. LeakSanitizer (LSAN) is a tool that works in conjunction with ASAN to find memory leaks, but it can introduce additional overhead. The logging issues you’re experiencing might stem from the interplay of ASAN and LSAN options. Specifically, setting detect_leaks=0 should ideally prevent LSAN from running, but it appears to cause side effects on ASAN's logging capability. Let's dive into a step-by-step troubleshooting process. Potential Reasons for Logging Issues Configuration Conflicts: The ASAN options might be conflicting. ASAN requires specific environment setups for logging errors correctly. Setting or omitting certain flags could lead to unexpected behavior. Core Dumps Handling: Core dumps indicate your program crashed and may provide insights into memory usage at the time of the crash. Depending on how Linux handles these dumps, ASAN might not operate as expected without proper configurations. Library Dependencies: Ensure that the ASAN libraries are correctly installed and compatible with your version of the Linux system. Incompatibilities or missing libraries may lead to logging failures. Step-by-Step Solution To resolve your ASAN logging issues, follow these steps: Step 1: Review Your ASAN Environment Variables Your environment variables look mostly fine. However, consider modifying them slightly to find the right balance for your daemon. For example: Environment=ASAN_OPTIONS=abort_on_error=1:log_path=%h/.local/share/grd_asan/as_%p.log:symbolize=1:halt_on_error=0:disable_coredump=0:unmap_shadow_on_exit=1:detect_leaks=1 After making the changes, restart your daemon and monitor the log output. Step 2: Check Core Dump Settings Examine your system's core dump settings to ensure it correctly generates dumps. You can configure them using: ulimit -c unlimited By running this command in the shell where your daemon starts, you can ensure that core dumps are generated without restrictions. Step 3: Re-enable LeakSanitizer Although it may seem counterintuitive, re-enabling detect_leaks=1 might give you more information. Do so by adjusting your ASAN_OPTIONS, as shown in Step 1. Monitoring the logs with LS enabled can give insights that ASAN might be missing otherwise. Step 4: Debugging the Backtrace Your backtrace highlights that ASAN attempts to log the issue correctly. Investigate the specific lines in your codebase that correlate with the logged crash site. You might be dealing with a premature program exit or a race condition. Examine the critical sections of your daemon for memory-related issues and paths that lead to aborts. Step 5: Add Debugging Statements In addition to ASAN, you can add logging statements in your code to gather more detail when potential crashes occur. For example: #include void problematic_function() { printf("Entering problematic_function\n"); // Your code that may cause issues } This will help you trace execution flow leading up to the crash. Final Thoughts By following these steps, you should be able to mitigate the logging issues with ASAN in your Linux daemon. Remember that debugging tools like ASAN and LSAN can be complex, so don't hesitate to reach out to community forums or documentation for extra support. Frequently Asked Questions (FAQ) Q1: Why does ASAN not log if detect_leaks is turned off? A1: This may happen due to internal ASAN mechanisms relying on LeakSanitizer for some logging functionalities. Turning it back on can sometimes help provide better insights. Q2: Are there any specific flags for better ASAN logging? A2: Aside from the logging options you’ve set, adding log_path is essential. Additionally, ensuring that your daemon has permissions to write to the specified logging directory is crucial. Q3: Can I run ASAN without core dumps? A3: While it is possible, core dumps provide valuable information during debugging. Consider using both ASAN and core dumps for a comprehensive analysis.
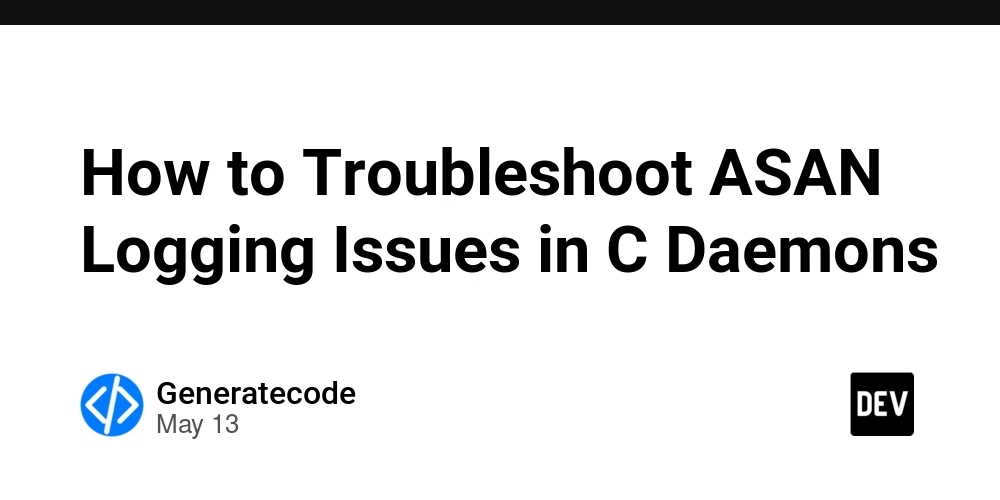
Introduction
When developing a Linux daemon utilizing AddressSanitizer (ASAN) for debugging memory issues, encountering problems with ASAN logging can be frustrating. You seem to be facing an unusual problem where ASAN fails to log crashes when detect_leaks=0
, yet it works perfectly when this option is enabled. Let’s explore this issue in detail.
Understanding ASAN and LeakSanitizer
AddressSanitizer (ASAN) is a memory error detector that helps identify issues like buffer overflows, use-after-free errors, and memory leaks. LeakSanitizer (LSAN) is a tool that works in conjunction with ASAN to find memory leaks, but it can introduce additional overhead.
The logging issues you’re experiencing might stem from the interplay of ASAN and LSAN options. Specifically, setting detect_leaks=0
should ideally prevent LSAN from running, but it appears to cause side effects on ASAN's logging capability. Let's dive into a step-by-step troubleshooting process.
Potential Reasons for Logging Issues
-
Configuration Conflicts: The ASAN options might be conflicting. ASAN requires specific environment setups for logging errors correctly. Setting or omitting certain flags could lead to unexpected behavior.
-
Core Dumps Handling: Core dumps indicate your program crashed and may provide insights into memory usage at the time of the crash. Depending on how Linux handles these dumps, ASAN might not operate as expected without proper configurations.
-
Library Dependencies: Ensure that the ASAN libraries are correctly installed and compatible with your version of the Linux system. Incompatibilities or missing libraries may lead to logging failures.
Step-by-Step Solution
To resolve your ASAN logging issues, follow these steps:
Step 1: Review Your ASAN Environment Variables
Your environment variables look mostly fine. However, consider modifying them slightly to find the right balance for your daemon. For example:
Environment=ASAN_OPTIONS=abort_on_error=1:log_path=%h/.local/share/grd_asan/as_%p.log:symbolize=1:halt_on_error=0:disable_coredump=0:unmap_shadow_on_exit=1:detect_leaks=1
After making the changes, restart your daemon and monitor the log output.
Step 2: Check Core Dump Settings
Examine your system's core dump settings to ensure it correctly generates dumps. You can configure them using:
ulimit -c unlimited
By running this command in the shell where your daemon starts, you can ensure that core dumps are generated without restrictions.
Step 3: Re-enable LeakSanitizer
Although it may seem counterintuitive, re-enabling detect_leaks=1
might give you more information. Do so by adjusting your ASAN_OPTIONS
, as shown in Step 1. Monitoring the logs with LS enabled can give insights that ASAN might be missing otherwise.
Step 4: Debugging the Backtrace
Your backtrace highlights that ASAN attempts to log the issue correctly. Investigate the specific lines in your codebase that correlate with the logged crash site. You might be dealing with a premature program exit or a race condition. Examine the critical sections of your daemon for memory-related issues and paths that lead to aborts.
Step 5: Add Debugging Statements
In addition to ASAN, you can add logging statements in your code to gather more detail when potential crashes occur. For example:
#include
void problematic_function() {
printf("Entering problematic_function\n");
// Your code that may cause issues
}
This will help you trace execution flow leading up to the crash.
Final Thoughts
By following these steps, you should be able to mitigate the logging issues with ASAN in your Linux daemon. Remember that debugging tools like ASAN and LSAN can be complex, so don't hesitate to reach out to community forums or documentation for extra support.
Frequently Asked Questions (FAQ)
Q1: Why does ASAN not log if detect_leaks
is turned off?
A1: This may happen due to internal ASAN mechanisms relying on LeakSanitizer for some logging functionalities. Turning it back on can sometimes help provide better insights.
Q2: Are there any specific flags for better ASAN logging?
A2: Aside from the logging options you’ve set, adding log_path
is essential. Additionally, ensuring that your daemon has permissions to write to the specified logging directory is crucial.
Q3: Can I run ASAN without core dumps?
A3: While it is possible, core dumps provide valuable information during debugging. Consider using both ASAN and core dumps for a comprehensive analysis.