How to Read Data from Redis Slave Nodes Using Java?
Reading data from slave nodes in Redis is an essential part of building scalable applications. If you’re working with a Redis cluster, it’s important to understand how to connect and retrieve data specifically from slave nodes, as they can help to offload read operations, improve performance, and ensure high availability. Understanding Redis Master-Slave Architecture Redis operates on a master-slave architecture where one primary master node handles write operations and one or more slave nodes replicate the data from the master and handle read requests. This separation of read and write responsibilities allows for improved load balancing and redundancy, making your application more resilient. Benefits of Using Slave Nodes Improved Read Performance: Distributing read traffic across multiple slaves reduces the load on the master node, enhancing response times. High Availability: In case the master fails, one of the slaves can take over with minimal downtime. Data Redundancy: Multiple copies of your data provide safety against data loss. Prerequisites Before we jump into the code, ensure that you have the following set up: Java Development Kit (JDK) installed. A Redis server set up with master and slave nodes. Jedis library for Java, which you can add via Maven or Gradle. Maven Dependency for Jedis If you're using Maven, include the following dependency in your pom.xml: redis.clients jedis 4.0.1 Example Code: Reading from Redis Slave Nodes Now let’s look at a sample Java code that demonstrates how to read data from a Redis slave node using Jedis: import redis.clients.jedis.Jedis; public class RedisSlaveReader { public static void main(String[] args) { // Replace with your slave node's IP and port String slaveHost = "127.0.0.1"; int slavePort = 6380; // Create a Jedis instance to connect to the slave node try (Jedis jedis = new Jedis(slaveHost, slavePort)) { // Check if the connection is successful System.out.println("Connected to Redis slave node."); // Fetch data from the slave node String value = jedis.get("yourKey"); if (value != null) { System.out.println("Value for 'yourKey': " + value); } else { System.out.println("Key 'yourKey' does not exist in the slave."); } } catch (Exception e) { e.printStackTrace(); } } } Code Breakdown Connecting to the Slave Node: In the code, we create a Jedis instance and connect to the slave node's IP and port. Fetching Data: We use the .get() method to retrieve the value associated with a specific key. If the key doesn't exist, a message is displayed. Error Handling: Utilizing a try-catch block ensures that any exceptions are caught, helping with debugging. Common Issues When Reading from Redis Slaves When connecting to Redis slave nodes, you might encounter a few common issues: Connection Refused: Ensure the slave node is running and accessible through the specified IP and port. Data Consistency: Remember that slave nodes might not always have the latest data due to replication lag. How to Troubleshoot Check Redis Logs: Look for any errors in the Redis server logs that might indicate issues with the slave node. Test Connection: Use redis-cli to ensure you can connect to the slave node and query data correctly. Monitor Replicas: Use the INFO replication command in Redis CLI to check the state of your nodes. Frequently Asked Questions (FAQ) Can I write to a Redis slave node? No, you cannot write to a slave node. All write operations must be directed to the master node. How does replication lag impact reads? Replication lag occurs when the slave nodes take time to copy data from the master. During this period, reads from slaves may return stale data. Can I use slaves for caching? Yes, using slaves for caching can help offload read requests, but ensure you are aware of potential data consistency issues due to replication delays. In conclusion, reading data from slave nodes in Redis using Java can greatly enhance your application's performance and availability. By using the Jedis library, it’s simple to connect and retrieve data efficiently. Follow the best practices outlined in this article to ensure you effectively utilize Redis’s slave nodes.
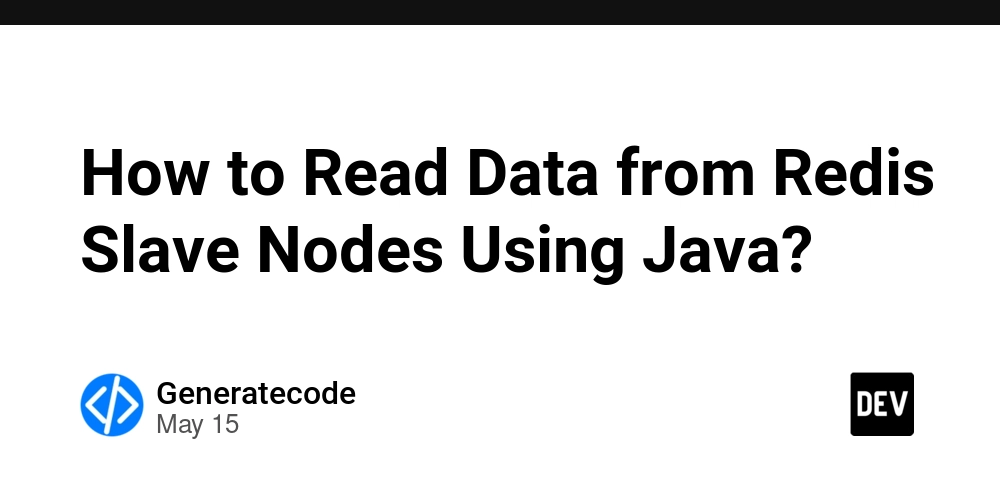
Reading data from slave nodes in Redis is an essential part of building scalable applications. If you’re working with a Redis cluster, it’s important to understand how to connect and retrieve data specifically from slave nodes, as they can help to offload read operations, improve performance, and ensure high availability.
Understanding Redis Master-Slave Architecture
Redis operates on a master-slave architecture where one primary master node handles write operations and one or more slave nodes replicate the data from the master and handle read requests. This separation of read and write responsibilities allows for improved load balancing and redundancy, making your application more resilient.
Benefits of Using Slave Nodes
- Improved Read Performance: Distributing read traffic across multiple slaves reduces the load on the master node, enhancing response times.
- High Availability: In case the master fails, one of the slaves can take over with minimal downtime.
- Data Redundancy: Multiple copies of your data provide safety against data loss.
Prerequisites
Before we jump into the code, ensure that you have the following set up:
- Java Development Kit (JDK) installed.
- A Redis server set up with master and slave nodes.
- Jedis library for Java, which you can add via Maven or Gradle.
Maven Dependency for Jedis
If you're using Maven, include the following dependency in your pom.xml
:
redis.clients
jedis
4.0.1
Example Code: Reading from Redis Slave Nodes
Now let’s look at a sample Java code that demonstrates how to read data from a Redis slave node using Jedis:
import redis.clients.jedis.Jedis;
public class RedisSlaveReader {
public static void main(String[] args) {
// Replace with your slave node's IP and port
String slaveHost = "127.0.0.1";
int slavePort = 6380;
// Create a Jedis instance to connect to the slave node
try (Jedis jedis = new Jedis(slaveHost, slavePort)) {
// Check if the connection is successful
System.out.println("Connected to Redis slave node.");
// Fetch data from the slave node
String value = jedis.get("yourKey");
if (value != null) {
System.out.println("Value for 'yourKey': " + value);
} else {
System.out.println("Key 'yourKey' does not exist in the slave.");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Code Breakdown
- Connecting to the Slave Node: In the code, we create a Jedis instance and connect to the slave node's IP and port.
-
Fetching Data: We use the
.get()
method to retrieve the value associated with a specific key. If the key doesn't exist, a message is displayed. - Error Handling: Utilizing a try-catch block ensures that any exceptions are caught, helping with debugging.
Common Issues When Reading from Redis Slaves
When connecting to Redis slave nodes, you might encounter a few common issues:
- Connection Refused: Ensure the slave node is running and accessible through the specified IP and port.
- Data Consistency: Remember that slave nodes might not always have the latest data due to replication lag.
How to Troubleshoot
- Check Redis Logs: Look for any errors in the Redis server logs that might indicate issues with the slave node.
-
Test Connection: Use
redis-cli
to ensure you can connect to the slave node and query data correctly. -
Monitor Replicas: Use the
INFO replication
command in Redis CLI to check the state of your nodes.
Frequently Asked Questions (FAQ)
Can I write to a Redis slave node?
No, you cannot write to a slave node. All write operations must be directed to the master node.
How does replication lag impact reads?
Replication lag occurs when the slave nodes take time to copy data from the master. During this period, reads from slaves may return stale data.
Can I use slaves for caching?
Yes, using slaves for caching can help offload read requests, but ensure you are aware of potential data consistency issues due to replication delays.
In conclusion, reading data from slave nodes in Redis using Java can greatly enhance your application's performance and availability. By using the Jedis library, it’s simple to connect and retrieve data efficiently. Follow the best practices outlined in this article to ensure you effectively utilize Redis’s slave nodes.