How to Declare Anonymous Types in C# Without Instances?
Introduction In C#, anonymous types are useful for encapsulating a set of read-only properties into a single object without explicitly defining a class. However, developers often seek better ways to declare these types without relying on creating instances, which can lead to less readable and maintainable code. This article explores various methods and optimizes the solution for anonymous types in C# while avoiding hacky implementations. Understanding Anonymous Types in C# Anonymous types in C# allow you to create simple objects without creating a separate class definition. However, creating instances as seen in your initial example can appear convoluted and detracts from clarity and maintainability. The issue arises when developers need a type to be used across different scopes without repeatedly instantiating it or using complex encoding or data structures. Streamlined Approach to Using Anonymous Types To enhance the readability and effective use of anonymous types within hash sets and similar data structures, consider defining your properties explicitly. Here’s a refined approach: Defining a Class for Clarity Instead of anonymous types, if you find yourself needing to use a specific structure in multiple places, consider crafting a dedicated class. This method minimizes confusion and makes your code easier to maintain. public class Person { public string FirstName { get; set; } public string LastName { get; set; } } Using the Person class ensures that you have a well-defined and clear structure to work with: Implementing HashSet with Defined Class Now you can use the Person class efficiently: private IEnumerable _people; public IEnumerable People() { HashSet hashSet; using (var scope = new Scope()) { hashSet = new HashSet { new Person { FirstName = "Boaty", LastName = "McBoatface" } }; } using (var anotherScope = new AnotherScope()) { return _people.Where(x => hashSet.Contains(new Person { FirstName = x.Nombre, LastName = x.Apellido })); } } Benefits of Using Defined Classes Readability: Code becomes clearer when using well-defined classes over hacky anonymous types. Reusability: Classes can be instantiated and passed around, making them more flexible than anonymous types. Type Safety: Using classes maintains stronger type checks during compile time. Frequently Asked Questions Why should I avoid anonymous types for larger applications? Anonymous types lose their contextual clarity as size and complexity increase. Regular classes provide a structured approach, improving maintainability. Can I use anonymous types in LINQ queries? Yes, you can efficiently use anonymous types in LINQ; however, for complex scenarios, well-defined classes are preferred. Are anonymous types suitable for multi-threaded applications? Using anonymous types can lead to challenges in thread safety and debugging; standard classes minimize these risks significantly. Conclusion Although anonymous types in C# offer a quick solution for many scenarios, they may not always provide the best path forward when clarity and maintainability matter. By defining your properties using standard classes, you gain readability and enhance the organization of your code. In cases where scopes and access to properties are crucial, this approach proves invaluable. By refining how we approach anonymous types, we can ensure our codebase remains adaptable and clear.
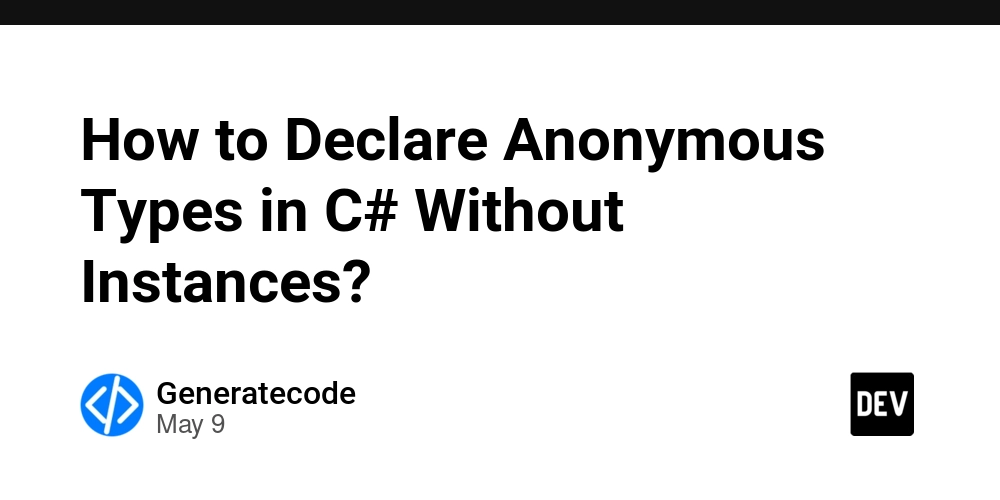
Introduction
In C#, anonymous types are useful for encapsulating a set of read-only properties into a single object without explicitly defining a class. However, developers often seek better ways to declare these types without relying on creating instances, which can lead to less readable and maintainable code. This article explores various methods and optimizes the solution for anonymous types in C# while avoiding hacky implementations.
Understanding Anonymous Types in C#
Anonymous types in C# allow you to create simple objects without creating a separate class definition. However, creating instances as seen in your initial example can appear convoluted and detracts from clarity and maintainability. The issue arises when developers need a type to be used across different scopes without repeatedly instantiating it or using complex encoding or data structures.
Streamlined Approach to Using Anonymous Types
To enhance the readability and effective use of anonymous types within hash sets and similar data structures, consider defining your properties explicitly. Here’s a refined approach:
Defining a Class for Clarity
Instead of anonymous types, if you find yourself needing to use a specific structure in multiple places, consider crafting a dedicated class. This method minimizes confusion and makes your code easier to maintain.
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
}
Using the Person
class ensures that you have a well-defined and clear structure to work with:
Implementing HashSet with Defined Class
Now you can use the Person
class efficiently:
private IEnumerable _people;
public IEnumerable People()
{
HashSet hashSet;
using (var scope = new Scope())
{
hashSet = new HashSet
{
new Person { FirstName = "Boaty", LastName = "McBoatface" }
};
}
using (var anotherScope = new AnotherScope())
{
return _people.Where(x => hashSet.Contains(new Person { FirstName = x.Nombre, LastName = x.Apellido }));
}
}
Benefits of Using Defined Classes
- Readability: Code becomes clearer when using well-defined classes over hacky anonymous types.
- Reusability: Classes can be instantiated and passed around, making them more flexible than anonymous types.
- Type Safety: Using classes maintains stronger type checks during compile time.
Frequently Asked Questions
Why should I avoid anonymous types for larger applications?
Anonymous types lose their contextual clarity as size and complexity increase. Regular classes provide a structured approach, improving maintainability.
Can I use anonymous types in LINQ queries?
Yes, you can efficiently use anonymous types in LINQ; however, for complex scenarios, well-defined classes are preferred.
Are anonymous types suitable for multi-threaded applications?
Using anonymous types can lead to challenges in thread safety and debugging; standard classes minimize these risks significantly.
Conclusion
Although anonymous types in C# offer a quick solution for many scenarios, they may not always provide the best path forward when clarity and maintainability matter. By defining your properties using standard classes, you gain readability and enhance the organization of your code. In cases where scopes and access to properties are crucial, this approach proves invaluable. By refining how we approach anonymous types, we can ensure our codebase remains adaptable and clear.