How Can I Handle Excessive Validator Errors in Angular Forms?
Introduction Handling form validations effectively is a common requirement in Angular applications, especially when using Material Design components like . When a user encounters multiple validation errors on a form input, it can sometimes lead to a chaotic user experience, particularly when the error messages overflow and overlap other UI elements. This article explores how to manage excessive validator messages gracefully and cleanly, giving user-friendly feedback without cluttering the interface. Understanding the Problem When displaying validator errors, the typical approach involves listing all the error messages directly below the input field. In scenarios with multiple errors, this can lead to overflowing content that disrupts the layout and makes it difficult for users to focus on the input field. The challenge lies in maintaining readability while still providing access to all the necessary error information. One effective solution would be to limit the visible error messages and provide a summary. This way, users can still understand that there are issues with their input without being overwhelmed by messages. Step-by-Step Solution In this section, we will outline how to implement a summary display for error messages using Angular's capabilities and improve visibility and legibility. Let's dive into the code. Adjusting the Template Below is a revised version of your existing Angular template that adds functionality for displaying a summarized error message: {{ control.label | translate }} Errors ({{ getErrorCount(control.formControl.errors) }}) {{ FORM_ERRORS[errorKey] | translate }} Break Down of the Solution Count Errors: The method getErrorCount(errors) counts the number of error keys in the form control. Summarize Errors: We check if the error count exceeds 2. If so, we display a summarized message, such as 'Errors (2)'. Otherwise, we list all error messages as before. Conditional Rendering: Angular's ng-container and ng-template are used for conditional rendering, enhancing performance and readability. Example Method Implementation You can implement the getErrorCount method in your component's TypeScript file: getErrorCount(errors: { [key: string]: any }): number { return Object.keys(errors).length; } Making Error Messages More Opaque To adjust the appearance of mat-error messages, you can add CSS to make the messages more readable. A slightly opaque background can help distinguish between the error messages and the input field: .mat-error { background-color: rgba(255, 0, 0, 0.1); /* light red background */ padding: 5px; border-radius: 4px; } Conclusion By summarizing excessive error messages and enhancing their visibility with a slight opacity, you can provide a clean and user-friendly experience for form validations in Angular applications. Doing so not only improves user interaction but also helps maintain clarity in the user interface. Educate your users effectively by informing them when issues arise while avoiding overwhelming them with numerous messages. Frequently Asked Questions Q: How do I ensure accessibility with summary messages? A: Always ensure that tooltips or hover effects used to expand the error messages are also keyboard navigable. Use ARIA live regions if applicable to announce changes dynamically. Q: What if I have different types of form inputs? A: You can apply similar logic across all input types; simply modify the switch cases accordingly. The logic remains the same, just adapt to different validation messages as needed. By implementing these strategies, your Angular form inputs can remain clean, clear, and user-friendly, regardless of the number of validation errors encountered.
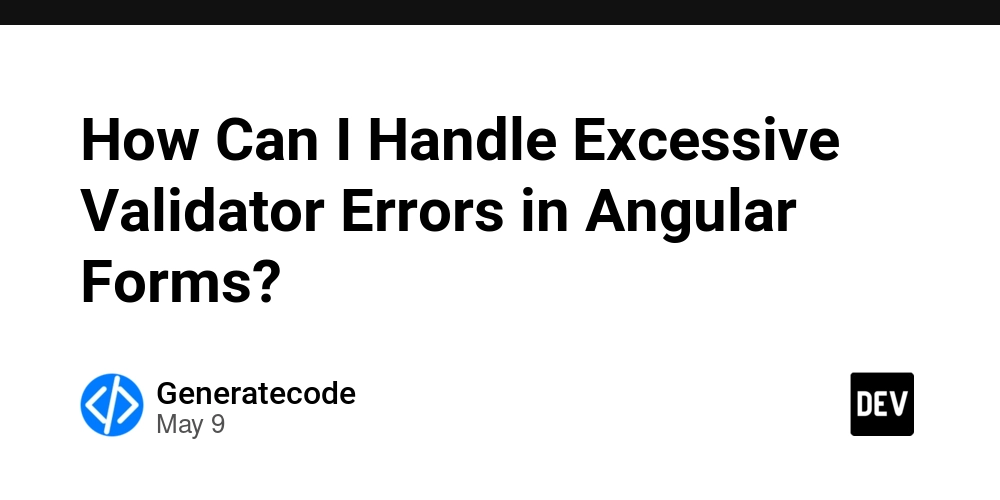
Introduction
Handling form validations effectively is a common requirement in Angular applications, especially when using Material Design components like
. When a user encounters multiple validation errors on a form input, it can sometimes lead to a chaotic user experience, particularly when the error messages overflow and overlap other UI elements. This article explores how to manage excessive validator messages gracefully and cleanly, giving user-friendly feedback without cluttering the interface.
Understanding the Problem
When displaying validator errors, the typical approach involves listing all the error messages directly below the input field. In scenarios with multiple errors, this can lead to overflowing content that disrupts the layout and makes it difficult for users to focus on the input field. The challenge lies in maintaining readability while still providing access to all the necessary error information.
One effective solution would be to limit the visible error messages and provide a summary. This way, users can still understand that there are issues with their input without being overwhelmed by messages.
Step-by-Step Solution
In this section, we will outline how to implement a summary display for error messages using Angular's capabilities and improve visibility and legibility. Let's dive into the code.
Adjusting the Template
Below is a revised version of your existing Angular template that adds functionality for displaying a summarized error message:
{{ control.label | translate }}
2; else showAllErrors">
Errors ({{ getErrorCount(control.formControl.errors) }})
{{ FORM_ERRORS[errorKey] | translate }}
Break Down of the Solution
-
Count Errors: The method
getErrorCount(errors)
counts the number of error keys in the form control. - Summarize Errors: We check if the error count exceeds 2. If so, we display a summarized message, such as 'Errors (2)'. Otherwise, we list all error messages as before.
-
Conditional Rendering: Angular's
ng-container
andng-template
are used for conditional rendering, enhancing performance and readability.
Example Method Implementation
You can implement the getErrorCount
method in your component's TypeScript file:
getErrorCount(errors: { [key: string]: any }): number {
return Object.keys(errors).length;
}
Making Error Messages More Opaque
To adjust the appearance of mat-error
messages, you can add CSS to make the messages more readable. A slightly opaque background can help distinguish between the error messages and the input field:
.mat-error {
background-color: rgba(255, 0, 0, 0.1); /* light red background */
padding: 5px;
border-radius: 4px;
}
Conclusion
By summarizing excessive error messages and enhancing their visibility with a slight opacity, you can provide a clean and user-friendly experience for form validations in Angular applications. Doing so not only improves user interaction but also helps maintain clarity in the user interface. Educate your users effectively by informing them when issues arise while avoiding overwhelming them with numerous messages.
Frequently Asked Questions
Q: How do I ensure accessibility with summary messages?
A: Always ensure that tooltips or hover effects used to expand the error messages are also keyboard navigable. Use ARIA live regions if applicable to announce changes dynamically.
Q: What if I have different types of form inputs?
A: You can apply similar logic across all input types; simply modify the switch cases accordingly. The logic remains the same, just adapt to different validation messages as needed.
By implementing these strategies, your Angular form inputs can remain clean, clear, and user-friendly, regardless of the number of validation errors encountered.