Gamepad API for Game Controller Integration
Comprehensive Guide to the Gamepad API for Game Controller Integration Table of Contents Introduction Historical Context Technical Overview of the Gamepad API 3.1. Gamepad API Specification 3.2. Types of Gamepad Input Devices Core Functionality of the Gamepad API 4.1. Gamepad Properties 4.2. Polling Gamepad States Advanced Code Examples 5.1. Complex Scenarios 5.2. Handling Multiple Gamepad Inputs Edge Cases and Advanced Implementations 6.1. Custom Gamepad Vibration 6.2. Non-Standard Controller Support Comparative Analysis with Alternative Approaches Real-World Use Cases 8.1. Gaming Industry Applications 8.2. Non-Gaming Applications Performance Considerations and Optimization Strategies 9.1. Reducing Latency 9.2. Memory Optimization Potential Pitfalls and Advanced Debugging Techniques Conclusion and Further Resources References 1. Introduction The Gamepad API is a JavaScript interface that provides a way for developers to access and manage game controller inputs within web applications. This allows for the creation of immersive and dynamic gaming experiences directly from web browsers. This guide aims to serve as a definitive resource for developers, especially senior developers, providing a deep dive into the Gamepad API's intricacies, performance considerations, and best practices. 2. Historical Context The emergence of the Gamepad API can be traced back to the growing need for interactive web applications that could leverage hardware peripherals, like game controllers. As HTML5 evolved and modern browsers started supporting new standards, the availability of robust APIs to access game controllers became an essential requirement, particularly with the rise of browser-based gaming and applications. Key milestones include: 2011: The Web Hypertext Application Technology Working Group (WHATWG) began discussing the need for a unified way to handle gamepad input. 2016: The Gamepad API became a working draft in the W3C's proposed specifications. 2018: Major browser vendors like Chrome, Firefox, and Edge implemented support for the Gamepad API, making it a viable option for developers across platforms. 3. Technical Overview of the Gamepad API 3.1. Gamepad API Specification The Gamepad API is designed to expose the state of gamepads to JavaScript in a way that allows developers to determine the current status of connected gamepad devices, such as buttons pressed, axes moved, and the capabilities of the device itself. 3.2. Types of Gamepad Input Devices There are various types of controllers that can be used, each with specific attributes: Xbox Controllers PlayStation Controllers Generic Controllers with varying configurations While the API standardizes interactions, each controller type may have unique button mappings. 4. Core Functionality of the Gamepad API 4.1. Gamepad Properties The API revolves around the Gamepad interface, which exposes various properties: id - Provides a unique identifier for the gamepad. buttons - An array of button states, each button is an object with properties like pressed, value, and touched. axes - An array representing joystick positions. 4.2. Polling Gamepad States Polling is central to detecting inputs in real-time: function updateGamepadStatus() { const gamepads = navigator.getGamepads(); for (let i = 0; i { if (button.pressed) { console.log(`Button ${index} is pressed`); } }); } } requestAnimationFrame(updateGamepadStatus); // Continually poll for gamepad state } window.addEventListener("gamepadconnected", (event) => { console.log(`Gamepad connected at index ${event.gamepad.index}: ${event.gamepad.id}`); updateGamepadStatus(); }); 5. Advanced Code Examples 5.1. Complex Scenarios Let's explore a more complex implementation that integrates multiple game controllers for a multiplayer game scenario. const gamepads = {}; function updateGamepads() { const connectedGamepads = navigator.getGamepads(); for (const gamepad of connectedGamepads) { if (gamepad) { gamepads[gamepad.index] = gamepad; console.log(`Gamepad ${gamepad.index} connected: ${gamepad.id}`); } else { delete gamepads[gamepad.index]; } } // Game logic based on gamepad states Object.values(gamepads).forEach((gamepad) => { handleGamepadInput(gamepad); }); requestAnimationFrame(updateGamepads); } function handleGamepadInput(gamepad) { // Example: Move and shoot actions const moveAxisX = gamepad.axes[0]; const moveAxisY = gamepad.axes[1]; if (Math.abs(moveAxisX) > 0.1 || Math.abs(moveAxisY) > 0.1) { console.log(`Moving player ${gamepad.index}: X=${moveAxisX}, Y=${moveAxisY}`); } if (gamepad.buttons[0
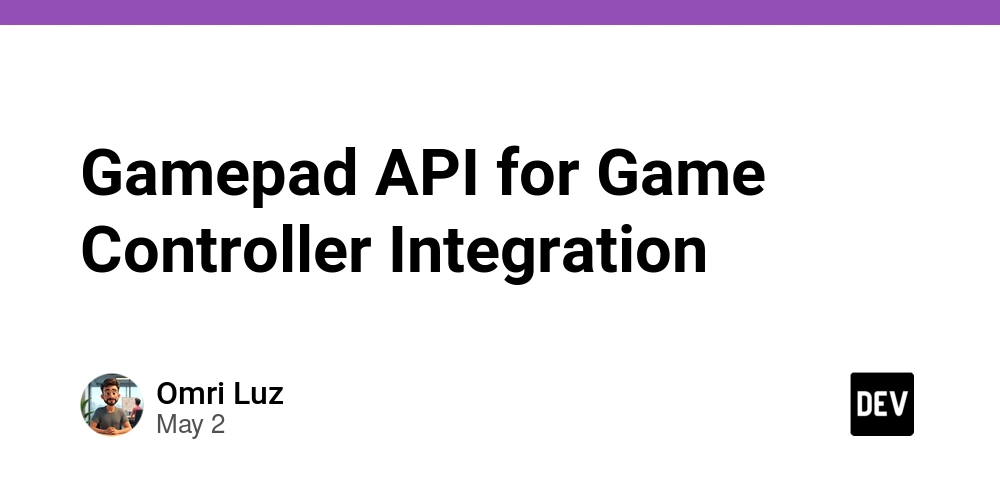
Comprehensive Guide to the Gamepad API for Game Controller Integration
Table of Contents
- Introduction
- Historical Context
-
Technical Overview of the Gamepad API
- 3.1. Gamepad API Specification
- 3.2. Types of Gamepad Input Devices
-
Core Functionality of the Gamepad API
- 4.1. Gamepad Properties
- 4.2. Polling Gamepad States
-
Advanced Code Examples
- 5.1. Complex Scenarios
- 5.2. Handling Multiple Gamepad Inputs
-
Edge Cases and Advanced Implementations
- 6.1. Custom Gamepad Vibration
- 6.2. Non-Standard Controller Support
- Comparative Analysis with Alternative Approaches
-
Real-World Use Cases
- 8.1. Gaming Industry Applications
- 8.2. Non-Gaming Applications
-
Performance Considerations and Optimization Strategies
- 9.1. Reducing Latency
- 9.2. Memory Optimization
- Potential Pitfalls and Advanced Debugging Techniques
- Conclusion and Further Resources
- References
1. Introduction
The Gamepad API is a JavaScript interface that provides a way for developers to access and manage game controller inputs within web applications. This allows for the creation of immersive and dynamic gaming experiences directly from web browsers. This guide aims to serve as a definitive resource for developers, especially senior developers, providing a deep dive into the Gamepad API's intricacies, performance considerations, and best practices.
2. Historical Context
The emergence of the Gamepad API can be traced back to the growing need for interactive web applications that could leverage hardware peripherals, like game controllers. As HTML5 evolved and modern browsers started supporting new standards, the availability of robust APIs to access game controllers became an essential requirement, particularly with the rise of browser-based gaming and applications.
Key milestones include:
- 2011: The Web Hypertext Application Technology Working Group (WHATWG) began discussing the need for a unified way to handle gamepad input.
- 2016: The Gamepad API became a working draft in the W3C's proposed specifications.
- 2018: Major browser vendors like Chrome, Firefox, and Edge implemented support for the Gamepad API, making it a viable option for developers across platforms.
3. Technical Overview of the Gamepad API
3.1. Gamepad API Specification
The Gamepad API is designed to expose the state of gamepads to JavaScript in a way that allows developers to determine the current status of connected gamepad devices, such as buttons pressed, axes moved, and the capabilities of the device itself.
3.2. Types of Gamepad Input Devices
There are various types of controllers that can be used, each with specific attributes:
- Xbox Controllers
- PlayStation Controllers
- Generic Controllers with varying configurations
While the API standardizes interactions, each controller type may have unique button mappings.
4. Core Functionality of the Gamepad API
4.1. Gamepad Properties
The API revolves around the Gamepad
interface, which exposes various properties:
-
id
- Provides a unique identifier for the gamepad. -
buttons
- An array of button states, each button is an object with properties likepressed
,value
, andtouched
. -
axes
- An array representing joystick positions.
4.2. Polling Gamepad States
Polling is central to detecting inputs in real-time:
function updateGamepadStatus() {
const gamepads = navigator.getGamepads();
for (let i = 0; i < gamepads.length; i++) {
const gamepad = gamepads[i];
if (gamepad) {
console.log(`Gamepad ${gamepad.index} connected. ID: ${gamepad.id}`);
gamepad.buttons.forEach((button, index) => {
if (button.pressed) {
console.log(`Button ${index} is pressed`);
}
});
}
}
requestAnimationFrame(updateGamepadStatus); // Continually poll for gamepad state
}
window.addEventListener("gamepadconnected", (event) => {
console.log(`Gamepad connected at index ${event.gamepad.index}: ${event.gamepad.id}`);
updateGamepadStatus();
});
5. Advanced Code Examples
5.1. Complex Scenarios
Let's explore a more complex implementation that integrates multiple game controllers for a multiplayer game scenario.
const gamepads = {};
function updateGamepads() {
const connectedGamepads = navigator.getGamepads();
for (const gamepad of connectedGamepads) {
if (gamepad) {
gamepads[gamepad.index] = gamepad;
console.log(`Gamepad ${gamepad.index} connected: ${gamepad.id}`);
} else {
delete gamepads[gamepad.index];
}
}
// Game logic based on gamepad states
Object.values(gamepads).forEach((gamepad) => {
handleGamepadInput(gamepad);
});
requestAnimationFrame(updateGamepads);
}
function handleGamepadInput(gamepad) {
// Example: Move and shoot actions
const moveAxisX = gamepad.axes[0];
const moveAxisY = gamepad.axes[1];
if (Math.abs(moveAxisX) > 0.1 || Math.abs(moveAxisY) > 0.1) {
console.log(`Moving player ${gamepad.index}: X=${moveAxisX}, Y=${moveAxisY}`);
}
if (gamepad.buttons[0].pressed) {
console.log(`Shooting with Gamepad ${gamepad.index}`);
}
}
window.addEventListener("gamepadconnected", updateGamepads);
5.2. Handling Multiple Gamepad Inputs
You might need to manage inputs from an arbitrary number of controllers, particularly when making more complex games. Here's an advanced example involving multiple gamepad handling.
const playerActions = {};
function processGamepadInput(gamepad) {
playerActions[gamepad.index] = playerActions[gamepad.index] || {};
gamepad.buttons.forEach((button, index) => {
if (button.pressed && !playerActions[gamepad.index][index]) {
playerActions[gamepad.index][index] = true;
console.log(`Player ${gamepad.index} pressed button ${index}`);
} else if (!button.pressed && playerActions[gamepad.index][index]) {
playerActions[gamepad.index][index] = false;
console.log(`Player ${gamepad.index} released button ${index}`);
}
});
}
function updateConnections() {
const gamepads = navigator.getGamepads();
for (const gamepad of gamepads) {
if (gamepad) {
processGamepadInput(gamepad);
}
}
requestAnimationFrame(updateConnections);
}
window.addEventListener("gamepadconnected", updateConnections);
6. Edge Cases and Advanced Implementations
6.1. Custom Gamepad Vibration
Apart from basic input, advanced features such as controller vibration may also be implemented via the Gamepad API when supported.
// Assuming gamepad has vibration capabilities
function vibrateController(gamepad) {
if (gamepad.vibrationActuator) {
gamepad.vibrationActuator.playEffect("dual-rumble", {
startDelay: 0,
duration: 1000,
strongMagnitude: 1.0,
weakMagnitude: 0.5,
});
}
}
function checkButtonAndVibrate(gamepad) {
if (gamepad.buttons[0].pressed) {
vibrateController(gamepad);
}
}
6.2. Non-Standard Controller Support
The Gamepad API depends on the gamepad’s compatibility with the modern standards. Developers may encounter controllers that do not adhere to common conventions (e.g., Steam controllers, or specialized hardware). To combat this, developers can create custom mappings and adapt input reading dynamically based on detected gamepad capabilities.
const customMapping = {
1: "A", // map to some custom action
2: "B", // map to some custom action
};
function handleNonStandardInputs(gamepad) {
gamepad.buttons.forEach((button, index) => {
if (button.pressed) {
const action = customMapping[index];
if (action) {
console.log(`Executing ${action} action via custom mapping for button ${index}`);
// Trigger custom action here
}
}
});
}
7. Comparative Analysis with Alternative Approaches
Before the Gamepad API's inception, developers relied heavily on external libraries, Flash, or custom plugins to handle gamepad input. Each method had severe limitations, such as:
- Flash: Has been deprecated and is not supported on most modern devices.
- External Libraries: Often lack standardization and require additional overhead to manage updates.
Advantages of Gamepad API
- Directly supported by major browsers.
- Standardized API functionality robust enough to support a variety of controllers.
- Simplifies the input management, reducing cross-browser compatibility issues.
8. Real-World Use Cases
8.1. Gaming Industry Applications
Major gaming platforms like Sky: Children of the Light and WebGL-based games (e.g., Krunker.io) leverage the Gamepad API for a seamless multi-controller experience.
8.2. Non-Gaming Applications
Gamepad API applications extend beyond gaming, enabling accessibility solutions through custom controls for disabled users or multimedia applications where a gamepad can serve as an alternative input device.
9. Performance Considerations and Optimization Strategies
9.1. Reducing Latency
Latency can significantly impact user experience. To minimize latency:
- Limit the frequency of state polling without compromising responsiveness.
- Optimize the requestAnimationFrame loops to avoid unnecessary re-renders or processing.
9.2. Memory Optimization
Keep allocated resources in check:
- Deallocate references to unused gamepad states.
- Use WeakMaps for storage if tracking limited information about controllers.
10. Potential Pitfalls and Advanced Debugging Techniques
Debugging Gamepad Inputs:
- Expect discrepancies in button indices across different gamepads. Create a mapping for each device or handle an array.
- Monitor connectivity changes and ensure deactivation of handlers for unresponsive gamepads.
- Use console logging extensively to visualize the button states being registered.
Handling Gamepad Disconnects:
window.addEventListener("gamepaddisconnected", (event) => {
console.log(`Gamepad ${event.gamepad.index} disconnected.`);
});
11. Conclusion and Further Resources
In conclusion, the Gamepad API provides a powerful and sophisticated means for game controller integration and interaction in web applications. The API continues to evolve, so keeping abreast of the latest developments and best practices is crucial for developers.
Further Resources:
12. References
- MDN Web Docs. Gamepad API.
- W3C Gamepad API Working Draft.
- Various community resources and articles on advanced game development techniques.
This guide seeks to balance complexity with accessibility, delivering a thorough exploration of the Gamepad API and its place in modern web development, helping seasoned developers bolster their expertise in this valuable area.