Day-16: Java Variables: Comprehensive Documentation
1). Variables Definition: Variables are containers that store data values in memory during program execution. 2). Classification of Variables A. Based on Data Type 1). Primitive Data Types Data Type Default Value Size (bits) Range/Example byte 0 8 -128 to 127 short 0 16 -32,768 to 32,767 int 0 32 -2³¹ to 2³¹-1 long 0L 64 -2⁶³ to 2⁶³-1 float 0.0f 32 3.4e-038 to 3.4e+038 double 0.0d 64 1.7e-308 to 1.7e+308 char '\u0000' 16 'A', '1', '$' boolean false 1 (JVM-dependent) true or false 2). Non-Primitive (Reference) Data Types Data Type Default Value Example String null "Hello" Arrays null int[] arr = {1, 2, 3}; Classes null Tourist tourist = new Tourist(); 3). Variable Scope A. Local Variables Declared inside methods/constructors/blocks. No default value (must be initialized before use). Lifetime: Exists only while the method executes. Example: public void calculate() { int localVar = 10; // Local variable (must be initialized) System.out.println(localVar); } B. Global Variables (Fields) Declared inside a class, outside methods. Have default values (if not initialized). Two types: Static (static keyword) → Class-level (shared). Non-Static (no keyword) → Instance-level (object-specific). Example: public class Tourist { static int globalStaticVar = 1000; // Static (class-level) int globalNonStaticVar = 500; // Non-static (instance-level) } 4). Default Values Variable Type Default Value Global (Static/Non-Static) Yes (e.g., 0, null) Local No (Compiler Error if Uninitialized) Interview Question: ❓ "Do local variables have default values?" ➡ No! Only global variables get default values. 5). Variable Naming Conflicts Case 1): Local Variable Shadows Global Variable If a local variable has the same name as a global variable, the local variable takes precedence. Example: public class Tourist { static int amount = 100000; // Global static public void havingDinner() { int amount = 2000; // Local variable (shadows global) System.out.println(amount); // Prints 2000 (local) } public static void main(String[] args) { System.out.println(Tourist.amount); // 100000 (global) Tourist t = new Tourist(); t.havingDinner(); // 2000 (local) } } Case 2): Accessing Global Variable Inside Method (Using this for Non-Static) public class Example { int value = 100; // Non-static global public void printValue() { int value = 50; // Local variable System.out.println(value); // 50 (local) System.out.println(this.value); // 100 (global) } } 6). Best Practices ✔ Use meaningful names (e.g., userAge instead of a). ✔ Initialize variables before use (especially local). ✔ Avoid shadowing unless necessary (can reduce readability). ✔ Use static for class-wide constants (e.g., static final double PI = 3.14;). Summary Table Feature Local Variables Global Variables Scope Inside method/block Class-level Default Value ❌ No (must initialize) ✔ Yes Lifetime Method execution Entire program (static) / Object lifetime (non-static) Shadowing Can override global Can be accessed via ClassName.staticVar or this.nonStaticVar This documentation covers variables in Java comprehensively, including data types, scope, naming conflicts, and best practices. Note: The formatting and structure were optimized for clarity with AI assistance. --------------------------- End of the Blog -------------------------------
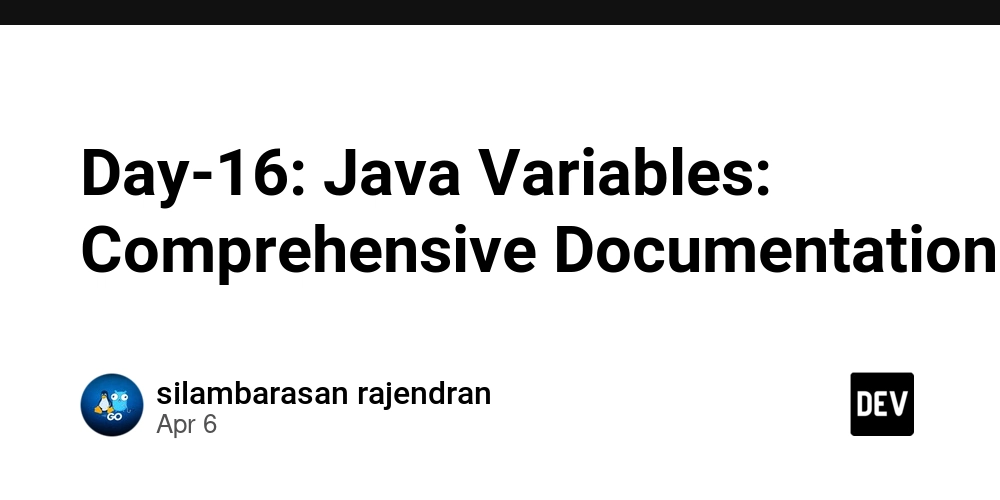
1). Variables Definition:
Variables are containers that store data values in memory during program execution.
2). Classification of Variables
A. Based on Data Type
1). Primitive Data Types
Data Type Default Value Size (bits) Range/Example
byte 0 8 -128 to 127
short 0 16 -32,768 to 32,767
int 0 32 -2³¹ to 2³¹-1
long 0L 64 -2⁶³ to 2⁶³-1
float 0.0f 32 3.4e-038 to 3.4e+038
double 0.0d 64 1.7e-308 to 1.7e+308
char '\u0000' 16 'A', '1', '$'
boolean false 1 (JVM-dependent) true or false
2). Non-Primitive (Reference) Data Types
Data Type Default Value Example
String null "Hello"
Arrays null int[] arr = {1, 2, 3};
Classes null Tourist tourist = new Tourist();
3). Variable Scope
A. Local Variables
Declared inside methods/constructors/blocks.
No default value (must be initialized before use).
Lifetime: Exists only while the method executes.
Example:
public void calculate() {
int localVar = 10; // Local variable (must be initialized)
System.out.println(localVar);
}
B. Global Variables (Fields)
Declared inside a class, outside methods.
Have default values (if not initialized).
Two types:
Static (static keyword) → Class-level (shared).
Non-Static (no keyword) → Instance-level (object-specific).
Example:
public class Tourist {
static int globalStaticVar = 1000; // Static (class-level)
int globalNonStaticVar = 500; // Non-static (instance-level)
}
4). Default Values
Variable Type Default Value
Global (Static/Non-Static) Yes (e.g., 0, null)
Local No (Compiler Error if Uninitialized)
Interview Question:
❓ "Do local variables have default values?"
➡ No! Only global variables get default values.
5). Variable Naming Conflicts
Case 1): Local Variable Shadows Global Variable
If a local variable has the same name as a global variable, the local variable takes precedence.
Example:
public class Tourist {
static int amount = 100000; // Global static
public void havingDinner() {
int amount = 2000; // Local variable (shadows global)
System.out.println(amount); // Prints 2000 (local)
}
public static void main(String[] args) {
System.out.println(Tourist.amount); // 100000 (global)
Tourist t = new Tourist();
t.havingDinner(); // 2000 (local)
}
}
Case 2): Accessing Global Variable Inside Method (Using this for Non-Static)
public class Example {
int value = 100; // Non-static global
public void printValue() {
int value = 50; // Local variable
System.out.println(value); // 50 (local)
System.out.println(this.value); // 100 (global)
}
}
6). Best Practices
✔ Use meaningful names (e.g., userAge instead of a).
✔ Initialize variables before use (especially local).
✔ Avoid shadowing unless necessary (can reduce readability).
✔ Use static for class-wide constants (e.g., static final double PI = 3.14;).
Summary Table
Feature Local Variables Global Variables
Scope Inside method/block Class-level
Default Value ❌ No (must initialize) ✔ Yes
Lifetime Method execution Entire program (static) / Object lifetime (non-static)
Shadowing Can override global Can be accessed via ClassName.staticVar or this.nonStaticVar
This documentation covers variables in Java comprehensively, including data types, scope, naming conflicts, and best practices.
Note: The formatting and structure were optimized for clarity with AI assistance.
--------------------------- End of the Blog -------------------------------