CSS Houdini API for Extending CSS Capabilities
CSS Houdini API: Extending CSS Capabilities The CSS Houdini API represents a paradigm shift in how developers can interact with and extend the capabilities of CSS. Conceived as a way to bridge the gap between traditional styling methods and the evolving needs of the web development landscape, Houdini allows developers to write custom CSS features that can be used alongside existing ones. This article offers a comprehensive exploration of the CSS Houdini API, blending historical context, technical nuances, code examples, and practical applications, making it an invaluable resource for senior developers seeking to master this innovative tool. Historical Context The journey toward CSS Houdini began with the need for more powerful styling capabilities in web development. Traditional CSS, while robust, has its limitations—especially when tackling dynamic layouts and complex animations. Developers often resorted to JavaScript solutions that could introduce inefficiencies and complexity. Recognizing this, the CSS Working Group began discussions around a proposal in 2015, leading to the CSS Houdini initiative. Houdini is not merely a library or framework but a set of APIs that enable developers to implement custom CSS features directly in the browser's rendering engine. It provides a mechanism that allows JavaScript to interact with the CSS Object Model (CSSOM), thus enabling developers to style their applications in new ways. Understanding the Houdini API The Houdini API includes several key components, each aimed at enhancing different aspects of CSS. These components are: Paint API: Allows developers to create custom paint worklets. Layout API: Enables the creation of new layout algorithms. Animation API: Facilitates the creation of custom animations. Typed OM: Provides a more efficient way to read and write styles to the CSSOM without the overhead of traditional methods. Paint API The Paint API allows the creation of custom paint effects that can be applied as part of the background or border of an element. This is done using a worklet script to define properties and methods for the rendering engine. Example: Custom Checkerboard Pattern Let’s create a simple checkerboard pattern using the Paint API: if ('paintWorklet' in CSS) { CSS.paintWorklet.addModule('checkerboard_paint.js'); } // checkerboard_paint.js registerPaint('checkerboard', class { static get inputProperties() { return ['--checker-color1', '--checker-color2']; } paint(ctx, size, properties) { const { width, height } = size; const color1 = properties.get('--checker-color1').toString(); const color2 = properties.get('--checker-color2').toString(); const boxSize = 20; for (let y = 0; y { const x = (index % cols) * childWidth; const y = Math.floor(index / cols) * childHeight; child.place(x, y, childWidth, childHeight); }); } }); Usage in CSS: .container { layout: custom-grid; --columns: 3; --rows: 2; } This example defines a custom grid layout where the number of columns and rows can be dynamically set via CSS variables, demonstrating the flexibility of the Layout API. Real-World Use Cases CSS Houdini offers exciting possibilities across various industries: Creative Agencies: Many agencies utilize Houdini’s Paint API for producing unique effects, such as dynamic backgrounds and text effects for websites. Game Development: Games utilize Houdini layouts to create responsive UIs that adapt seamlessly to varying screen sizes and resolutions. Design Systems: Building reusable components that maintain consistent styles while allowing for custom behaviors and aesthetics can benefit from Houdini. Performance Considerations Despite the power offered by Houdini, developers must also consider performance implications. The following strategies can help ensure optimal performance: Minimize Repaints: When applying custom styles, aim to minimize repaints by leveraging the will-change property judiciously. This can help the browser prepare optimally for upcoming changes. Batch Worklet Execution: When designing worklets, ensure that they process changes in batches to reduce rendering overhead. Profiling Tools: Utilize tools like Chrome DevTools to monitor paint performance, ensuring that custom worklets do not introduce significant delays. Edge Cases and Advanced Implementation While the Houdini API is powerful, it is crucial to be aware of potential pitfalls: Fallbacks: Always consider scenarios where support for Houdini features may not exist. Implement fallback styles for non-supporting browsers. Non-blocking Nature: Worklets are non-blocking, which means that user interaction might continue while rendering happens in the background. Proper synchronizations or state management in JavaScript may be necessary to keep UI updates in sync. Advanced Debugging Techniqu
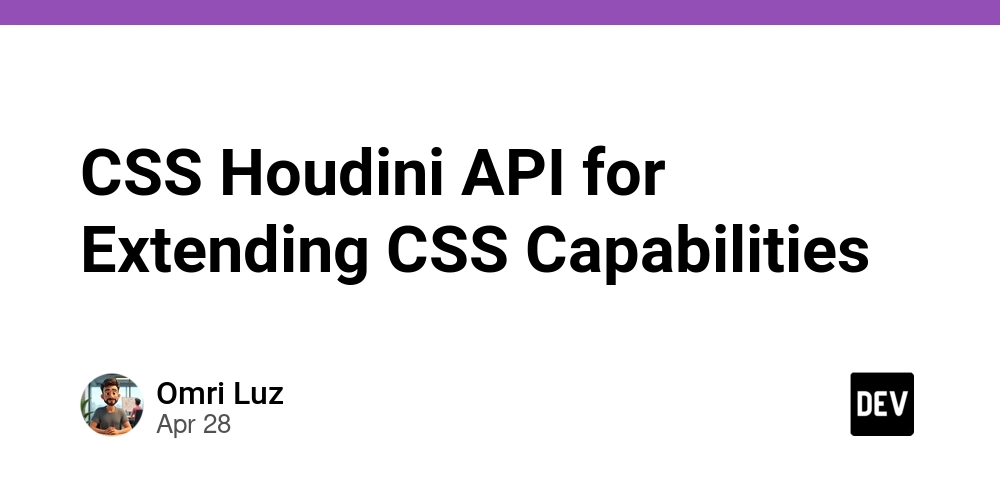
CSS Houdini API: Extending CSS Capabilities
The CSS Houdini API represents a paradigm shift in how developers can interact with and extend the capabilities of CSS. Conceived as a way to bridge the gap between traditional styling methods and the evolving needs of the web development landscape, Houdini allows developers to write custom CSS features that can be used alongside existing ones. This article offers a comprehensive exploration of the CSS Houdini API, blending historical context, technical nuances, code examples, and practical applications, making it an invaluable resource for senior developers seeking to master this innovative tool.
Historical Context
The journey toward CSS Houdini began with the need for more powerful styling capabilities in web development. Traditional CSS, while robust, has its limitations—especially when tackling dynamic layouts and complex animations. Developers often resorted to JavaScript solutions that could introduce inefficiencies and complexity. Recognizing this, the CSS Working Group began discussions around a proposal in 2015, leading to the CSS Houdini initiative.
Houdini is not merely a library or framework but a set of APIs that enable developers to implement custom CSS features directly in the browser's rendering engine. It provides a mechanism that allows JavaScript to interact with the CSS Object Model (CSSOM), thus enabling developers to style their applications in new ways.
Understanding the Houdini API
The Houdini API includes several key components, each aimed at enhancing different aspects of CSS. These components are:
- Paint API: Allows developers to create custom paint worklets.
- Layout API: Enables the creation of new layout algorithms.
- Animation API: Facilitates the creation of custom animations.
- Typed OM: Provides a more efficient way to read and write styles to the CSSOM without the overhead of traditional methods.
Paint API
The Paint API allows the creation of custom paint effects that can be applied as part of the background or border of an element. This is done using a worklet script to define properties and methods for the rendering engine.
Example: Custom Checkerboard Pattern
Let’s create a simple checkerboard pattern using the Paint API:
if ('paintWorklet' in CSS) {
CSS.paintWorklet.addModule('checkerboard_paint.js');
}
// checkerboard_paint.js
registerPaint('checkerboard', class {
static get inputProperties() {
return ['--checker-color1', '--checker-color2'];
}
paint(ctx, size, properties) {
const { width, height } = size;
const color1 = properties.get('--checker-color1').toString();
const color2 = properties.get('--checker-color2').toString();
const boxSize = 20;
for (let y = 0; y < height; y += boxSize) {
for (let x = 0; x < width; x += boxSize) {
ctx.fillStyle = (Math.floor(x / boxSize) + Math.floor(y / boxSize)) % 2 === 0 ? color1 : color2;
ctx.fillRect(x, y, boxSize, boxSize);
}
}
}
});
Usage in CSS:
.element {
background: paint(checkerboard);
--checker-color1: red;
--checker-color2: blue;
}
This code illustrates a custom paint worklet that generates a checkerboard pattern with colors defined in CSS variables.
Layout API
The Layout API enables developers to create new layout models that can be invoked in their CSS. This is particularly important when dealing with unique or complex layouts that require more flexibility than traditional CSS offers.
Example: Custom Grid Layout
if ('layoutWorklet' in CSS) {
CSS.layoutWorklet.addModule('custom_grid_layout.js');
}
// custom_grid_layout.js
registerLayout('custom-grid', class {
static get inputProperties() {
return ['--columns', '--rows'];
}
async layout(children, bounds, styles) {
const cols = parseInt(styles.get('--columns'));
const rows = parseInt(styles.get('--rows'));
const childWidth = bounds.width / cols;
const childHeight = bounds.height / rows;
children.forEach((child, index) => {
const x = (index % cols) * childWidth;
const y = Math.floor(index / cols) * childHeight;
child.place(x, y, childWidth, childHeight);
});
}
});
Usage in CSS:
.container {
layout: custom-grid;
--columns: 3;
--rows: 2;
}
This example defines a custom grid layout where the number of columns and rows can be dynamically set via CSS variables, demonstrating the flexibility of the Layout API.
Real-World Use Cases
CSS Houdini offers exciting possibilities across various industries:
- Creative Agencies: Many agencies utilize Houdini’s Paint API for producing unique effects, such as dynamic backgrounds and text effects for websites.
- Game Development: Games utilize Houdini layouts to create responsive UIs that adapt seamlessly to varying screen sizes and resolutions.
- Design Systems: Building reusable components that maintain consistent styles while allowing for custom behaviors and aesthetics can benefit from Houdini.
Performance Considerations
Despite the power offered by Houdini, developers must also consider performance implications. The following strategies can help ensure optimal performance:
Minimize Repaints: When applying custom styles, aim to minimize repaints by leveraging the
will-change
property judiciously. This can help the browser prepare optimally for upcoming changes.Batch Worklet Execution: When designing worklets, ensure that they process changes in batches to reduce rendering overhead.
Profiling Tools: Utilize tools like Chrome DevTools to monitor paint performance, ensuring that custom worklets do not introduce significant delays.
Edge Cases and Advanced Implementation
While the Houdini API is powerful, it is crucial to be aware of potential pitfalls:
- Fallbacks: Always consider scenarios where support for Houdini features may not exist. Implement fallback styles for non-supporting browsers.
- Non-blocking Nature: Worklets are non-blocking, which means that user interaction might continue while rendering happens in the background. Proper synchronizations or state management in JavaScript may be necessary to keep UI updates in sync.
Advanced Debugging Techniques
When developing Houdini worklets, debugging can be nuanced. Here are several strategies:
Console Logging in Worklets: While worklets run in a worker context, you can post messages to the main thread for debugging purposes.
Using Chrome DevTools: Chrome's worklet debug capabilities can be leveraged by enabling "Enable CSS Custom Properties" in DevTools settings.
Inspecting Worklet States: Use the
getComputedStyle()
method to check custom properties or state conditions after rendering.
Comparison with Alternative Approaches
Before Houdini, developers relied heavily on JavaScript solutions that manipulated the DOM to achieve custom layouts and effects. While libraries like GSAP, Anime.js, and CSS pre-processors (Sass, LESS) provided powerful tools, they typically did not integrate as seamlessly into the rendering engine as Houdini does.
Houdini streamlines the process by allowing developers to define styles that behave like native CSS. Moreover, it offloads rendering tasks from the main thread into a more efficient context, improving performance.
Conclusion
The CSS Houdini API provides a powerful, flexible, and modern means of leveraging CSS for front-end development. By allowing developers to create custom styles, layouts, and animation effects directly tied to CSS, it reduces the reliance on JavaScript while promoting better performance. While it is essential to navigate potential pitfalls and consider performance implications, the practical applications and creative possibilities are vast.
With the combination of innovative potential and the ability to optimize user experience, Houdini is poised to become an integral tool in the arsenal of forward-thinking web developers. For further reading and in-depth technical specifications, refer to the CSS Houdini official documentation and follow ongoing discussions in the CSS Working Group.
As always, continuous exploration and experimentation will be key to mastering the Houdini API and harnessing its power to reshape how we think about CSS.