A Deep Dive into Node.js Logging Tools
Leapcell: The Best of Serverless Web Hosting In-depth Analysis and Comparison of Node.js Logging Tools In the field of software engineering, logging is an indispensable and crucial component. By meticulously recording various events during the operation of an application, logs provide developers with valuable insights, enabling them to gain a deep understanding of how the code actually executes. This helps in debugging, performance optimization, and problem troubleshooting. This article will focus on several mainstream logging tools in the Node.js environment, including Bunyan, Winston, Pino, Morgan, and npmlog, and conduct a detailed analysis and comparison of their features, usage methods, as well as advantages and disadvantages. Core Elements of Logging When dealing with application logs, understanding log levels is a fundamental and critical aspect. Log levels are a standard for classifying entries in a log file according to their urgency and display method. Each log message corresponds to a specific log level, which reflects the importance and urgency of the message. In Node.js, the most common log levels include: ERROR: Indicates a serious problem that must be addressed immediately. Usually, such errors cause some functions of the application to fail to operate properly, but the application may still continue to run. For example, when a database connection fails and data cannot be stored properly, an ERROR-level log should be recorded. INFO: Used to record routine events that have occurred. This type of information is usually used to show the normal running status and progress of the application. For instance, events like a user successfully logging into the system or an order being successfully created can be logged at the INFO level. DEBUG: Contains detailed information that is only useful during the debugging phase and may have less value in a production environment. For example, during development, recording information such as function parameter passing and variable value changes helps developers locate code logic issues. WARN: Indicates a potentially harmful situation. Although it does not currently cause a serious error, it may lead to problems in the future. For example, when system resources are approaching the critical value or there are incompatible settings in the configuration file, a WARN-level log should be recorded. FATAL: Indicates an extremely serious error event that is expected to cause the application to terminate immediately. Situations such as system memory exhaustion or the crash of a critical process require recording at the FATAL level. Detailed Explanation of Node.js Logging Libraries Bunyan Bunyan is a popular logging tool in the Node.js ecosystem. It is a simple and efficient JSON logging library that also provides a nice-looking CLI view of the logs. Different log levels are distinguished by different colors, making it easy for developers to quickly identify and view them. As of April 28, 2025, it has 7.2k stars and 522 issues on GitHub. Installation Execute the following command in the terminal to install Bunyan: npm i bunyan Usage Create a file named test.js and import the Bunyan library: const bunyan = require('bunyan'); Use the createLogger method to define a logger instance: var log = bunyan.createLogger({ name: 'leapcell-test', stream: process.stdout }); Use the logger to record data: log.info('hi'); After running the test.js file, you will get output similar to the following in the console: {"name":"leapcell-test","hostname":"your_hostname","pid":xxxx,"level":30,"msg":"hi","time":"2025-04-28Txx:xx:xx.xxxZ","v":0} It can be seen that the logs generated by Bunyan are in JSON format, and each log contains key information such as the time when the log occurred. In practical applications, these logs are usually stored in a file for subsequent reference and analysis. Advantages Multi-runtime Support: In addition to Node.js, it also supports multiple runtime environments such as Webpack, Browserify, and NW.js. Serialization Mechanism: It has the concept of serialization and can convert JavaScript objects into JSON-serializable objects. A specific logger instance can map log record field names to serialization functions through serializer. Sub-log Function: Developers can create dedicated loggers for sub-components of the application and include additional binding fields in the log records, which is convenient for detailed log management of specific modules. Winston Winston is one of the top logging libraries in the Node.js field. With its large and active community and rich features, it has received 23.5k stars and 1.6k issues on GitHub. Through the decoupling of logging functions and a large number of configuration options, it achieves a high degree of extensibility and flexibility, allowing developers to easi
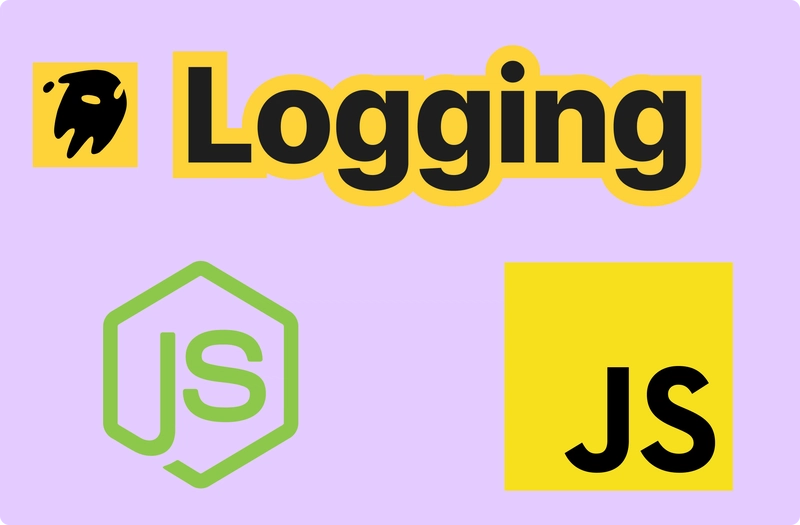
Leapcell: The Best of Serverless Web Hosting
In-depth Analysis and Comparison of Node.js Logging Tools
In the field of software engineering, logging is an indispensable and crucial component. By meticulously recording various events during the operation of an application, logs provide developers with valuable insights, enabling them to gain a deep understanding of how the code actually executes. This helps in debugging, performance optimization, and problem troubleshooting. This article will focus on several mainstream logging tools in the Node.js environment, including Bunyan, Winston, Pino, Morgan, and npmlog, and conduct a detailed analysis and comparison of their features, usage methods, as well as advantages and disadvantages.
Core Elements of Logging
When dealing with application logs, understanding log levels is a fundamental and critical aspect. Log levels are a standard for classifying entries in a log file according to their urgency and display method. Each log message corresponds to a specific log level, which reflects the importance and urgency of the message. In Node.js, the most common log levels include:
- ERROR: Indicates a serious problem that must be addressed immediately. Usually, such errors cause some functions of the application to fail to operate properly, but the application may still continue to run. For example, when a database connection fails and data cannot be stored properly, an ERROR-level log should be recorded.
- INFO: Used to record routine events that have occurred. This type of information is usually used to show the normal running status and progress of the application. For instance, events like a user successfully logging into the system or an order being successfully created can be logged at the INFO level.
- DEBUG: Contains detailed information that is only useful during the debugging phase and may have less value in a production environment. For example, during development, recording information such as function parameter passing and variable value changes helps developers locate code logic issues.
- WARN: Indicates a potentially harmful situation. Although it does not currently cause a serious error, it may lead to problems in the future. For example, when system resources are approaching the critical value or there are incompatible settings in the configuration file, a WARN-level log should be recorded.
- FATAL: Indicates an extremely serious error event that is expected to cause the application to terminate immediately. Situations such as system memory exhaustion or the crash of a critical process require recording at the FATAL level.
Detailed Explanation of Node.js Logging Libraries
Bunyan
Bunyan is a popular logging tool in the Node.js ecosystem. It is a simple and efficient JSON logging library that also provides a nice-looking CLI view of the logs. Different log levels are distinguished by different colors, making it easy for developers to quickly identify and view them. As of April 28, 2025, it has 7.2k stars and 522 issues on GitHub.
Installation
Execute the following command in the terminal to install Bunyan:
npm i bunyan
Usage
- Create a file named
test.js
and import the Bunyan library:
const bunyan = require('bunyan');
- Use the
createLogger
method to define a logger instance:
var log = bunyan.createLogger({
name: 'leapcell-test',
stream: process.stdout
});
- Use the logger to record data:
log.info('hi');
After running the test.js
file, you will get output similar to the following in the console:
{"name":"leapcell-test","hostname":"your_hostname","pid":xxxx,"level":30,"msg":"hi","time":"2025-04-28Txx:xx:xx.xxxZ","v":0}
It can be seen that the logs generated by Bunyan are in JSON format, and each log contains key information such as the time when the log occurred. In practical applications, these logs are usually stored in a file for subsequent reference and analysis.
Advantages
- Multi-runtime Support: In addition to Node.js, it also supports multiple runtime environments such as Webpack, Browserify, and NW.js.
-
Serialization Mechanism: It has the concept of serialization and can convert JavaScript objects into JSON-serializable objects. A specific logger instance can map log record field names to serialization functions through
serializer
. - Sub-log Function: Developers can create dedicated loggers for sub-components of the application and include additional binding fields in the log records, which is convenient for detailed log management of specific modules.
Winston
Winston is one of the top logging libraries in the Node.js field. With its large and active community and rich features, it has received 23.5k stars and 1.6k issues on GitHub. Through the decoupling of logging functions and a large number of configuration options, it achieves a high degree of extensibility and flexibility, allowing developers to easily meet various complex logging requirements.
Installation
Execute the following command in the terminal to install Winston:
npm i winston
Usage
- Import the Winston library in the root file and create a logger instance:
const winston = require('winston');
const logger = winston.createLogger({});
- Make rich configurations through the
createLogger
method, such as setting the log level, format, and meta description:
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'leapcell-test' },
});
- You can specify writing logs to files through the
transports
array:
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'leapcell-test' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' }),
],
});
The above configuration means that all error-level logs are written to the error.log
file, and other logs are written to the combined.log
file. In addition, you can also choose to display logs only in the console by adding winston.transports.Console()
to the transports
array.
Advantages
- Cloud Log Integration: It supports sending logs to cloud logging services such as logz.io and AWS Cloudwatch. Compared with traditional static file storage or console recording, it provides more powerful log management and analysis capabilities.
- Convenient Tracing: Each log contains a timestamp and log level, which makes it convenient for developers to quickly trace and locate problems according to specific situations.
Pino
Pino is known for its "extremely low overhead" and is an efficient Node.js logger. It achieves fast logging by minimizing resource consumption. As time goes by, the recorded information continues to increase, but it will not have a significant impact on the application performance. For example, it will not cause a large reduction in the number of requests per second. As of April 28, 2025, it has 15.3k stars and 530 issues on GitHub.
Installation
Run the following command in the terminal to install Pino:
npm i pino
Usage
Using Pino is very simple and straightforward. You just need to import the library and initialize it:
const logger = require('pino')();
logger.info('hello world');
After running the above script, you will get output similar to the following in the console:
{"level":30,"time":xxxxxxx,"msg":"hello world","pid":xxxx,"hostname":"your_hostname"}
The output log data contains the log level, recording time, actual log information, process ID, and hostname.
Usage in Express.js
- Install the
pino-http
package:
npm install pino-http
- Use it in an Express.js application:
const express = require('express');
const pino = require('pino-http');
const app = express();
const pinoInstance = pino();
app.use(pinoInstance);
app.post('/do-stuff', (req, res) => {
req.log.info('Something done');
res.send('Say hello to Pino');
});
app.listen(5500);
Advantages
-
Formatting Tool: It provides the
pino-pretty
module, which is a formatter based on Newline Delimited JSON (NDJSON). NDJSON is an efficient structured data storage and streaming format that can process records one by one.pino-pretty
will perform additional formatting on the log data according to the log level, timestamp, and other information, making the logs more readable. You can install it globally vianpm install -g pino-pretty
and then run the application using thenode app.js | pino-pretty
command to view the logs in a formatted form.
Morgan
Morgan is a Node.js library specifically designed for recording HTTP requests. It is usually integrated as a middleware into web frameworks such as Express.js to achieve tracking of all HTTP requests. Unlike other general-purpose logging tools, it focuses on HTTP request logging. Currently, it has 8k stars and 485 issues on GitHub.
Installation
Execute the following command in the terminal to install Morgan:
npm i morgan
Usage
- Import the Morgan library and add it as an Express.js middleware:
var morgan = require('morgan');
app.use(morgan('dev'));
Here, dev
is one of the log formats of Morgan. Morgan has implemented a total of five log formats:
- combined: Uses the standard Apache combined log output format.
- common: Also uses the standard Apache combined log output format.
- dev: A concise output format that displays the response status in different colors, suitable for the development environment.
- short: Includes the response time and simplifies the logs by default.
- tiny: Uses a minimized output format.
- You can choose different formats for configuration according to your needs:
app.use(morgan('combined'));
app.use(morgan('common'));
app.use(morgan('dev'));
app.use(morgan('short'));
app.use(morgan('tiny'));
Advantages
It provides a variety of predefined log formats. Developers can quickly select the appropriate format according to the project requirements and usage scenarios without writing complex configuration code, which greatly saves development time.
npmlog
npmlog is the logging tool officially used by npm. Similar to other Node.js logging libraries, it supports custom log levels, colored output, and allows developers to set personalized colors for different log levels. It has 458 stars and 55 issues on GitHub.
Installation
Run the following command in the terminal to install npmlog:
npm i npmlog
Usage
- Create a test file and import the npmlog package:
const log = require('npmlog');
log.info('hello leapcell', 'Hello from logrocket', {'message': 'test'});
The log.info
method can accept up to three parameters, which are the log prefix, the actual log information, and the additional data.
Advantages
It has a rich set of methods, such as setting the log header, title style, and defining log levels, which can simplify log-related operations during development and improve development efficiency.
Statistical Comparison of Node.js Logging Libraries
Library Name | GitHub Stars | GitHub Issue Count | Main Features and Applicable Scenarios |
---|---|---|---|
Bunyan | 7.2k | 522 | JSON-formatted logs, multi-runtime support, suitable for projects with high requirements for log structuring and cross-environment usage |
Winston | 23.5k | 1.6k | Highly extensible and flexible, supports cloud logging services, suitable for complex applications and scenarios with diverse log management requirements |
Pino | 15.3k | 530 | Low overhead, suitable for Node.js applications with extremely high performance requirements and large log volumes |
Morgan | 8k | 485 | Focuses on HTTP request logging, suitable for web applications and API services, convenient for request tracking and analysis |
npmlog | 458 | 55 | The official npm logging tool, suitable for npm-related projects and scenarios with simple logging function requirements |
Conclusion
The rich logging tools in the Node.js ecosystem provide developers with a variety of choices, making it easy to implement logging functions in actual projects. However, choosing the right logging library is crucial. It is necessary to comprehensively consider factors such as the specific requirements of the application, performance requirements, deployment environment, and the complexity of log management. Developers should select a logging tool that can accurately record the required data, is easy to analyze and troubleshoot problems, and at the same time does not have too much impact on the application performance, so as to provide strong support for the stable operation and continuous optimization of the project.
Leapcell: The Best of Serverless Web Hosting
Finally, I recommend a platform that is most suitable for deploying Node.js services: Leapcell