Why is Visual Studio Code Warning About OpenCV Version?
In this article, we'll explore a common warning encountered in Visual Studio Code when attempting to print the version of installed packages, specifically OpenCV. Many developers face similar issues while running scripts to check versions of libraries, such as PyTorch, NumPy, and OpenCV. Let's dive into why this warning appears and how you can resolve it or suppress it effectively. Understanding the Warning from Visual Studio Code When you run the script provided: ## --------------------------------------------- ## Installed package version information ## --------------------------------------------- ## Importing modules import torch import torchvision import numpy as np import cv2 ## Printing version information for each package print(f'torch v.{torch.__version__}') print(f'torchvision v.{torchvision.__version__}') print(f'numpy v.{np.__version__}') print(f'cv2 v.{cv2.__version__}') You might receive the warning: "Module 'cv2' has no 'version' member". This behavior can be perplexing. So, why does it happen? What's Causing the Warning? The warning originates from type-checking tools like mypy and the language server used in Visual Studio Code. These tools analyze your code and try to verify if the attributes you are trying to access on a module actually exist. In your case, "cv2" does not have an official __version__ attribute defined in its module. Consequently, the static analysis performed by the IDE leads to this warning. Here are a few reasons why the warning appears: Missing Type Definition: The type stubs for OpenCV may not define the __version__ member, which is why the IDE can't detect it. Dynamic Nature of Python: Python is a dynamically typed language, and some package properties are set at runtime rather than defined statically. Conda Packages: The way OpenCV was installed (via Conda) might also impact how the library was packaged and what metadata is available for type checking. Solutions for the Warning If you want to suppress the warning or find a workaround, here are some step-by-step solutions you can follow: 1. Ignore the Warning You can configure your IDE to ignore specific warnings related to missing attributes. In Visual Studio Code, you may want to add the following in the settings.json file: "python.linting.mypyEnabled": false, This will disable mypy type checking, but take note that it will hide all mypy warnings, not just the one related to OpenCV. 2. Use a Try-Except Block A more controlled method to handle potential missing attributes is using a try-except block: try: print(f'cv2 v.{cv2.__version__}') except AttributeError: print('cv2 version information not available') This way you can prevent your program from crashing if the attribute does not exist, and you avoid the warning in a clean manner. 3. Manual Check of Version If you prefer not to modify your code structure too much, consider checking for the version in a more manual way: import cv2 cv2_version = 'Unknown' # Default value try: cv2_version = cv2.__version__ except AttributeError: pass print(f'cv2 v.{cv2_version}') This will help you print 'Unknown' if the version is not accessible, ensuring your script can run smoothly without warnings. Frequently Asked Questions (FAQ) Q1: What is the purpose of __version__? A1: The __version__ attribute is typically used by Python packages to specify the version of the library currently in use. This helps developers ensure that they are using the correct version for their code. Q2: Is this warning a serious issue? A2: No, it’s mostly a minor issue. The warning doesn’t affect the functionality of your script as long as the packages work properly; it’s simply an alert from the type checker. Q3: How can I check OpenCV version without __version__? A3: You can check the version of OpenCV using alternative methods, such as using cv2.getBuildInformation() to view detailed build information about OpenCV. Conclusion In summary, the warning appearing in Visual Studio Code about cv2 not having a __version__ member is due to the limitations of type-checking tools that analyze your code. You can suppress the warning or handle it gracefully using try-except statements or default values. Understanding these aspects will help you work more efficiently and promote better coding practices. Remember, while these tools are useful, they sometimes have limitations that you need to navigate around. Happy coding!
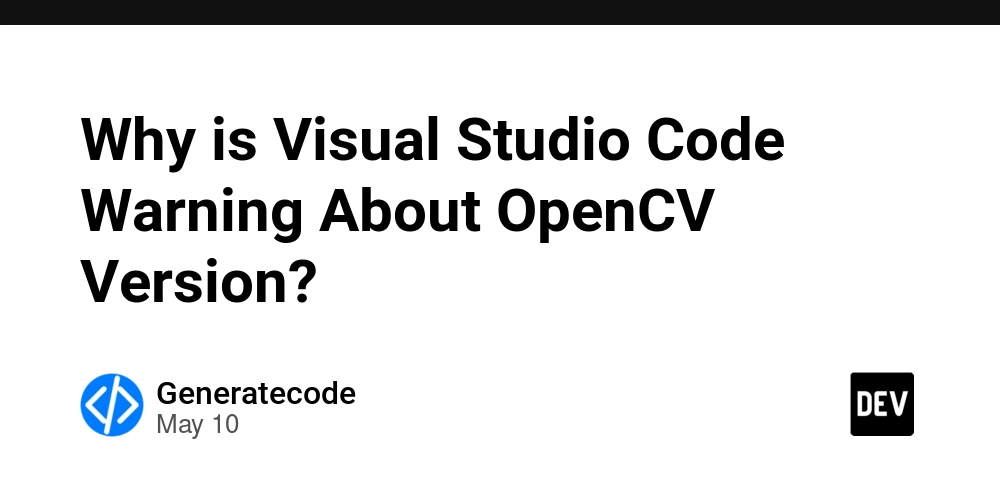
In this article, we'll explore a common warning encountered in Visual Studio Code when attempting to print the version of installed packages, specifically OpenCV. Many developers face similar issues while running scripts to check versions of libraries, such as PyTorch, NumPy, and OpenCV. Let's dive into why this warning appears and how you can resolve it or suppress it effectively.
Understanding the Warning from Visual Studio Code
When you run the script provided:
## ---------------------------------------------
## Installed package version information
## ---------------------------------------------
## Importing modules
import torch
import torchvision
import numpy as np
import cv2
## Printing version information for each package
print(f'torch v.{torch.__version__}')
print(f'torchvision v.{torchvision.__version__}')
print(f'numpy v.{np.__version__}')
print(f'cv2 v.{cv2.__version__}')
You might receive the warning: "Module 'cv2' has no 'version' member". This behavior can be perplexing. So, why does it happen?
What's Causing the Warning?
The warning originates from type-checking tools like mypy and the language server used in Visual Studio Code. These tools analyze your code and try to verify if the attributes you are trying to access on a module actually exist. In your case, "cv2" does not have an official __version__
attribute defined in its module. Consequently, the static analysis performed by the IDE leads to this warning.
Here are a few reasons why the warning appears:
-
Missing Type Definition: The type stubs for OpenCV may not define the
__version__
member, which is why the IDE can't detect it. - Dynamic Nature of Python: Python is a dynamically typed language, and some package properties are set at runtime rather than defined statically.
- Conda Packages: The way OpenCV was installed (via Conda) might also impact how the library was packaged and what metadata is available for type checking.
Solutions for the Warning
If you want to suppress the warning or find a workaround, here are some step-by-step solutions you can follow:
1. Ignore the Warning
You can configure your IDE to ignore specific warnings related to missing attributes. In Visual Studio Code, you may want to add the following in the settings.json
file:
"python.linting.mypyEnabled": false,
This will disable mypy type checking, but take note that it will hide all mypy warnings, not just the one related to OpenCV.
2. Use a Try-Except Block
A more controlled method to handle potential missing attributes is using a try-except block:
try:
print(f'cv2 v.{cv2.__version__}')
except AttributeError:
print('cv2 version information not available')
This way you can prevent your program from crashing if the attribute does not exist, and you avoid the warning in a clean manner.
3. Manual Check of Version
If you prefer not to modify your code structure too much, consider checking for the version in a more manual way:
import cv2
cv2_version = 'Unknown' # Default value
try:
cv2_version = cv2.__version__
except AttributeError:
pass
print(f'cv2 v.{cv2_version}')
This will help you print 'Unknown' if the version is not accessible, ensuring your script can run smoothly without warnings.
Frequently Asked Questions (FAQ)
Q1: What is the purpose of __version__
?
A1: The __version__
attribute is typically used by Python packages to specify the version of the library currently in use. This helps developers ensure that they are using the correct version for their code.
Q2: Is this warning a serious issue?
A2: No, it’s mostly a minor issue. The warning doesn’t affect the functionality of your script as long as the packages work properly; it’s simply an alert from the type checker.
Q3: How can I check OpenCV version without __version__
?
A3: You can check the version of OpenCV using alternative methods, such as using cv2.getBuildInformation()
to view detailed build information about OpenCV.
Conclusion
In summary, the warning appearing in Visual Studio Code about cv2
not having a __version__
member is due to the limitations of type-checking tools that analyze your code. You can suppress the warning or handle it gracefully using try-except statements or default values. Understanding these aspects will help you work more efficiently and promote better coding practices. Remember, while these tools are useful, they sometimes have limitations that you need to navigate around. Happy coding!