Vector Dimensions: Handle with Care!
"Dimensions aren't just numbers — they're the room your ideas get to breathe in." Not All Vectors Are Created Equal So you’ve got an embedding. It’s a list of floats — like [0.23, -0.56, 1.12, ...]. Great. But have you ever paused to ask: how long should that list be? That’s where vector dimensions come in. When we say an embedding is 768 or 1536 or 4096-dimensional, we’re talking about how much "space" a model gives to represent meaning. But more isn’t always better. Let's walk through why. Dimensionality = Expressiveness Imagine describing a photo using just three words. Now imagine using 300. The more dimensions you give a model, the more subtle features it can encode: Word meaning Syntax Emotion Domain specificity Each added dimension is like giving your AI another brushstroke — but there's a catch. Why Not Just Use 10,000 Dimensions? Because more dimensions mean: Slower computation (harder to search, store, and scale) Risk of overfitting (the model gets too good at memorizing noise) Curse of dimensionality (distance metrics break down) You’re not just increasing resolution — you’re adding baggage. So… How Many Dimensions Do We Really Need? There’s no magic number, but here’s a rough guide: 128–384: Light-weight models, fast retrieval, low cost 768–1024: Common for BERT-like models (balanced for NLP tasks) 1536–4096: Used in OpenAI, Cohere, and other LLM-grade embeddings >4096: Niche — only if you're encoding very rich data Real-World Tradeoffs If you’re: Running semantic search at scale → smaller dimensions = faster index + cheaper storage Doing domain-specific RAG → medium dimensions give better nuance Building open-ended chatbots → higher dimensions help retain subtle context Your use case defines your dimensional sweet spot. Why We Reduce Dimensions Higher dimensions increase the chance that two vectors look equally close. This is because as dimensionality increases, data points tend to become equidistant from each other — a phenomenon known as the curse of dimensionality. This hurts algorithms like cosine similarity or Euclidean distance because the difference in distances between relevant and irrelevant vectors becomes less distinguishable. When everything is almost the same distance apart, it's harder to find the "closest" match with confidence. The signal gets muddy. That’s why dimensionality reduction techniques like PCA or SVD are sometimes used to bring embeddings back down to Earth. But how does that work in practice? How PCA Helps PCA (Principal Component Analysis) is a mathematical technique that finds the most important directions in your data. Instead of treating all 1536 dimensions equally, PCA asks: "Which axes capture the biggest variance across samples?" Then it projects your data onto those axes, keeping only the top few. It’s like distilling the essence of each vector while leaving out less useful noise. This is especially helpful when you want to: Visualize high-dimensional data (in 2D or 3D) Speed up similarity searches Understand the structure of your embedding space Let’s look at a quick example to make this concrete: from sklearn.decomposition import PCA import numpy as np # Simulate two 1536-dimensional sentence embeddings vecs = np.random.rand(2, 1536) # Reduce to 2D to visualize or understand structure pca = PCA(n_components=2) reduced = pca.fit_transform(vecs) print("Original shape:", vecs.shape) print("Reduced shape:", reduced.shape) print("Reduced vectors:", reduced) This is a toy case with just two embeddings, but it shows how high-dimensional data can be squeezed into a smaller, more interpretable form — perfect for debugging, visualization, or fast lookup. In Summary Vector dimensions control how much nuance your embedding can carry. More dimensions = more power, but also more complexity. Pick the smallest size that preserves meaning for your task. "A 4096-d vector doesn’t mean it’s four times better than 1024. It just means it speaks in paragraphs, not sentences."
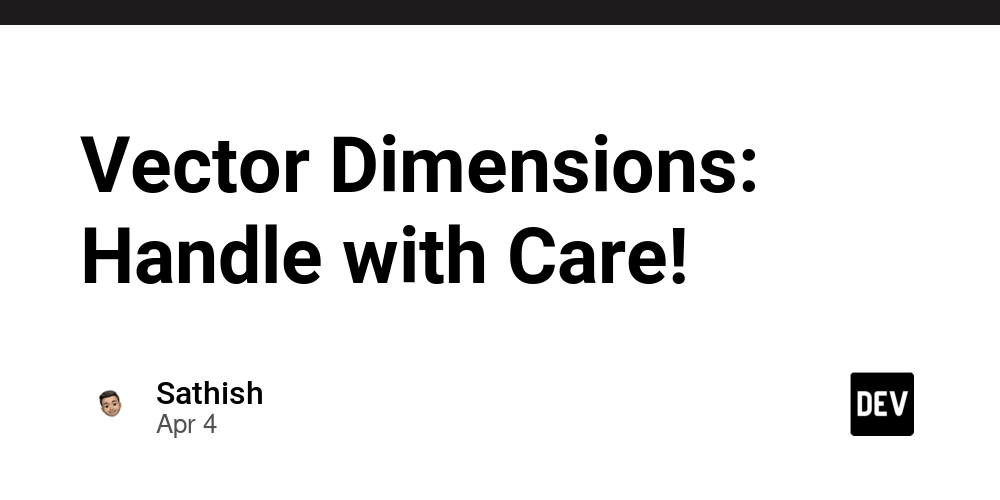
"Dimensions aren't just numbers — they're the room your ideas get to breathe in."
Not All Vectors Are Created Equal
So you’ve got an embedding. It’s a list of floats — like [0.23, -0.56, 1.12, ...]
. Great. But have you ever paused to ask: how long should that list be?
That’s where vector dimensions come in. When we say an embedding is 768 or 1536 or 4096-dimensional, we’re talking about how much "space" a model gives to represent meaning.
But more isn’t always better. Let's walk through why.
Dimensionality = Expressiveness
Imagine describing a photo using just three words. Now imagine using 300.
The more dimensions you give a model, the more subtle features it can encode:
- Word meaning
- Syntax
- Emotion
- Domain specificity
Each added dimension is like giving your AI another brushstroke — but there's a catch.
Why Not Just Use 10,000 Dimensions?
Because more dimensions mean:
- Slower computation (harder to search, store, and scale)
- Risk of overfitting (the model gets too good at memorizing noise)
- Curse of dimensionality (distance metrics break down)
You’re not just increasing resolution — you’re adding baggage.
So… How Many Dimensions Do We Really Need?
There’s no magic number, but here’s a rough guide:
- 128–384: Light-weight models, fast retrieval, low cost
- 768–1024: Common for BERT-like models (balanced for NLP tasks)
- 1536–4096: Used in OpenAI, Cohere, and other LLM-grade embeddings
- >4096: Niche — only if you're encoding very rich data
Real-World Tradeoffs
If you’re:
- Running semantic search at scale → smaller dimensions = faster index + cheaper storage
- Doing domain-specific RAG → medium dimensions give better nuance
- Building open-ended chatbots → higher dimensions help retain subtle context
Your use case defines your dimensional sweet spot.
Why We Reduce Dimensions
Higher dimensions increase the chance that two vectors look equally close. This is because as dimensionality increases, data points tend to become equidistant from each other — a phenomenon known as the curse of dimensionality.
This hurts algorithms like cosine similarity or Euclidean distance because the difference in distances between relevant and irrelevant vectors becomes less distinguishable. When everything is almost the same distance apart, it's harder to find the "closest" match with confidence. The signal gets muddy.
That’s why dimensionality reduction techniques like PCA or SVD are sometimes used to bring embeddings back down to Earth.
But how does that work in practice?
How PCA Helps
PCA (Principal Component Analysis) is a mathematical technique that finds the most important directions in your data.
Instead of treating all 1536 dimensions equally, PCA asks:
"Which axes capture the biggest variance across samples?"
Then it projects your data onto those axes, keeping only the top few. It’s like distilling the essence of each vector while leaving out less useful noise.
This is especially helpful when you want to:
- Visualize high-dimensional data (in 2D or 3D)
- Speed up similarity searches
- Understand the structure of your embedding space
Let’s look at a quick example to make this concrete:
from sklearn.decomposition import PCA
import numpy as np
# Simulate two 1536-dimensional sentence embeddings
vecs = np.random.rand(2, 1536)
# Reduce to 2D to visualize or understand structure
pca = PCA(n_components=2)
reduced = pca.fit_transform(vecs)
print("Original shape:", vecs.shape)
print("Reduced shape:", reduced.shape)
print("Reduced vectors:", reduced)
This is a toy case with just two embeddings, but it shows how high-dimensional data can be squeezed into a smaller, more interpretable form — perfect for debugging, visualization, or fast lookup.
In Summary
- Vector dimensions control how much nuance your embedding can carry.
- More dimensions = more power, but also more complexity.
- Pick the smallest size that preserves meaning for your task.
"A 4096-d vector doesn’t mean it’s four times better than 1024. It just means it speaks in paragraphs, not sentences."