useState Overload? How useReducer Can Make Your React State Management Easier
Introduction If you’ve been building React applications for a while, you’ve likely reached for useState as your go-to state management tool. It’s simple, intuitive, and works great for basic scenarios. But what happens when your component’s state logic grows complex? Nested useState calls, intertwined updates, and messy handlers can quickly turn your clean code into a tangled mess. That’s where useReducer comes in—a more scalable and maintainable alternative for managing complex state. In this article, we’ll explore when and why you should consider switching from useState to useReducer. 1. The Problem with useState in Complex Scenarios ✅ useState works well for: Simple, independent state values (e.g., isLoading, inputValue) Straightforward updates (e.g., setCount(count + 1)) ❌ But it falls short when: Multiple state updates depend on each other State transitions involve complex logic State becomes deeply nested or interconnected Example of useState Struggles: const [form, setForm] = useState({ username: '', email: '', password: '', errors: {}, isSubmitting: false, }); const handleSubmit = async () => { setForm(prev => ({ ...prev, isSubmitting: true })); try { const response = await submitForm(form); setForm(prev => ({ ...prev, success: true, errors: {} })); } catch (error) { setForm(prev => ({ ...prev, errors: error.response.data })); } finally { setForm(prev => ({ ...prev, isSubmitting: false })); } }; This gets messy fast—imagine adding validation, reset logic, or more fields! 2. How useReducer Solves This Inspired by Redux but much simpler, useReducer centralizes state logic in a reducer function, making updates predictable and easier to debug. Basic Structure of useReducer const [state, dispatch] = useReducer(reducer, initialState); Refactoring the Form with useReducer Instead of spreading and updating the state manually, we can handle state changes more efficiently: const initialState = { username: '', email: '', password: '', errors: {}, isSubmitting: false, success: false, }; function formReducer(state, action) { switch (action.type) { case 'FIELD_CHANGE': return { ...state, [action.field]: action.value }; case 'SUBMIT_START': return { ...state, isSubmitting: true, errors: {} }; case 'SUBMIT_SUCCESS': return { ...state, isSubmitting: false, success: true }; case 'SUBMIT_FAILURE': return { ...state, isSubmitting: false, errors: action.errors }; case 'RESET_FORM': return initialState; default: return state; } } // Usage in component const [state, dispatch] = useReducer(formReducer, initialState); const handleSubmit = async () => { dispatch({ type: 'SUBMIT_START' }); try { await submitForm(state); dispatch({ type: 'SUBMIT_SUCCESS' }); } catch (error) { dispatch({ type: 'SUBMIT_FAILURE', errors: error.response.data }); } }; Benefits of useReducer: ✔ Clear separation of state logic ✔ Easier debugging (state transitions are explicit and logged) ✔ Better scalability for complex state(managing complex state with ease) 3. When Should You Actually Use useReducer? Not every component needs useReducer. Here’s when it shines:
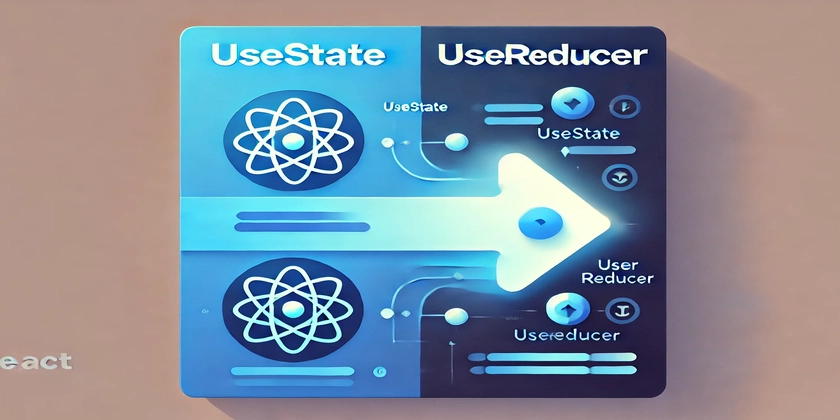
Introduction
If you’ve been building React applications for a while, you’ve likely reached for useState
as your go-to state management tool. It’s simple, intuitive, and works great for basic scenarios. But what happens when your component’s state logic grows complex? Nested useState
calls, intertwined updates, and messy handlers can quickly turn your clean code into a tangled mess.
That’s where useReducer
comes in—a more scalable and maintainable alternative for managing complex state. In this article, we’ll explore when and why you should consider switching from useState
to useReducer
.
1. The Problem with useState in Complex Scenarios
✅ useState works well for:
- Simple, independent state values (e.g.,
isLoading
,inputValue
) - Straightforward updates (e.g.,
setCount(count + 1)
)
❌ But it falls short when:
- Multiple state updates depend on each other
- State transitions involve complex logic
- State becomes deeply nested or interconnected
Example of useState Struggles:
const [form, setForm] = useState({
username: '',
email: '',
password: '',
errors: {},
isSubmitting: false,
});
const handleSubmit = async () => {
setForm(prev => ({ ...prev, isSubmitting: true }));
try {
const response = await submitForm(form);
setForm(prev => ({ ...prev, success: true, errors: {} }));
} catch (error) {
setForm(prev => ({ ...prev, errors: error.response.data }));
} finally {
setForm(prev => ({ ...prev, isSubmitting: false }));
}
};
This gets messy fast—imagine adding validation, reset logic, or more fields!
2. How useReducer Solves This
Inspired by Redux but much simpler, useReducer
centralizes state logic in a reducer function, making updates predictable and easier to debug.
Basic Structure of useReducer
const [state, dispatch] = useReducer(reducer, initialState);
Refactoring the Form with useReducer
Instead of spreading and updating the state manually, we can handle state changes more efficiently:
const initialState = {
username: '',
email: '',
password: '',
errors: {},
isSubmitting: false,
success: false,
};
function formReducer(state, action) {
switch (action.type) {
case 'FIELD_CHANGE':
return { ...state, [action.field]: action.value };
case 'SUBMIT_START':
return { ...state, isSubmitting: true, errors: {} };
case 'SUBMIT_SUCCESS':
return { ...state, isSubmitting: false, success: true };
case 'SUBMIT_FAILURE':
return { ...state, isSubmitting: false, errors: action.errors };
case 'RESET_FORM':
return initialState;
default:
return state;
}
}
// Usage in component
const [state, dispatch] = useReducer(formReducer, initialState);
const handleSubmit = async () => {
dispatch({ type: 'SUBMIT_START' });
try {
await submitForm(state);
dispatch({ type: 'SUBMIT_SUCCESS' });
} catch (error) {
dispatch({ type: 'SUBMIT_FAILURE', errors: error.response.data });
}
};
Benefits of useReducer:
✔ Clear separation of state logic
✔ Easier debugging (state transitions are explicit and logged)
✔ Better scalability for complex state(managing complex state with ease)
3. When Should You Actually Use useReducer?
Not every component needs useReducer
. Here’s when it shines: