Tutorial 8: Creating and Customizing Buttons, Labels, and Text Fields
In this tutorial, we’ll build a fun and interactive “Random Joke Generator” app. The app will generate a random joke every time the user presses a Button, display it on a Label, and allow users to enter their name in a Text Field for a more personalized experience. App Overview Our app will: Have a Text Field where users can enter their name. Display a button labeled "Tell Me a Joke!". Upon pressing the button, the app will display a random joke on a Label, and address the user by name if they have entered one. What You’ll Need Xcode installed on your macOS system Basic knowledge of Swift and iOS development Step 1: Setting up the Xcode Project Open Xcode and create a new project. Choose the App template and click Next. Name your project RandomJokeGenerator and set the interface to Storyboard and the language to Swift. Click Next and save your project. Step 2: Design the UI Open the Main.storyboard file. Drag the following components from the Object Library to the View Controller: Text Field for entering the user’s name. Button labeled “Tell Me a Joke!”. Label to display the random joke. Arrange them neatly in the view. Place the Text Field at the top, the Button below it, and the Label beneath the Button. Customize their properties: Text Field: Set the placeholder text to "Enter your name". Button: Change the title to “Tell Me a Joke!”. Label: Set the initial text to "Your joke will appear here!" and center the text. Step 3: Link UI Components to Code Open the Assistant Editor in Xcode to view both the storyboard and the code. Ctrl-drag the Text Field, Button, and Label from the storyboard to the ViewController.swift file to create IBOutlet and IBAction connections. For the Text Field, create an IBOutlet named nameTextField. For the Label, create an IBOutlet named jokeLabel. For the Button, create an IBAction method named tellMeAJokePressed. Your code should now have the following connections: @IBOutlet weak var nameTextField: UITextField! @IBOutlet weak var jokeLabel: UILabel! @IBAction func tellMeAJokePressed(_ sender: UIButton) { // We'll write the action code here in the next step } Step 4: Writing the Code Now let’s implement the action when the button is pressed. The app should take the text entered in the Text Field, check if it’s not empty, and then display a random joke on the Label. If the user has entered their name, we’ll personalize the joke. Here’s a list of jokes to pick from: let jokes = [ "Why don't skeletons fight each other? They don't have the guts!", "I told my computer I needed a break, and now it won’t stop sending me Kit-Kats.", "Why don’t eggs tell jokes? They’d crack each other up.", "Why did the coffee file a police report? It got mugged!", "I told my wife she was drawing her eyebrows too high. She looked surprised!" ] Now let’s implement the functionality in the tellMeAJokePressed method: @IBAction func tellMeAJokePressed(_ sender: UIButton) { // Get the user's name if available let userName = nameTextField.text ?? "" // Generate a random joke let randomJoke = jokes.randomElement()! // Personalize the joke if the user has entered their name if !userName.isEmpty { jokeLabel.text = "\(userName), here’s your joke: \(randomJoke)" } else { jokeLabel.text = "Here’s your joke: \(randomJoke)" } } Step 5: Customize UI Elements Let’s make our app more fun and engaging by customizing the Button, Label, and Text Field. Button Customization: Set the Button's background color to orange (for a cheerful look). Change the title color to white and make the button text bold. Add rounded corners for a smooth, modern appearance. tellMeAJokeButton.layer.cornerRadius = 10 tellMeAJokeButton.backgroundColor = .orange tellMeAJokeButton.setTitleColor(.white, for: .normal) tellMeAJokeButton.titleLabel?.font = UIFont.boldSystemFont(ofSize: 16) Label Customization: Set the Label's text color to dark blue to make the jokes stand out. Increase the font size and weight to make the text more prominent. jokeLabel.textColor = .darkBlue jokeLabel.font = UIFont.systemFont(ofSize: 20, weight: .medium) Text Field Customization: Add some padding inside the Text Field for better user experience. Set a border around the Text Field to make it visually distinct. nameTextField.layer.borderColor = UIColor.gray.cgColor nameTextField.layer.borderWidth = 1 nameTextField.layer.cornerRadius = 5 nameTextField.paddingLeft = 10 Step 6: Run Your App Choose the simulator device (e.g., iPhone 13) from the Xcode toolbar. Press Cmd + R to build and run your app. In the simulator, enter your name in the Text Field, press the Tell Me a Joke! button, and see a random joke personalized with your name. If no name is entered, the joke will simply appear without personaliz
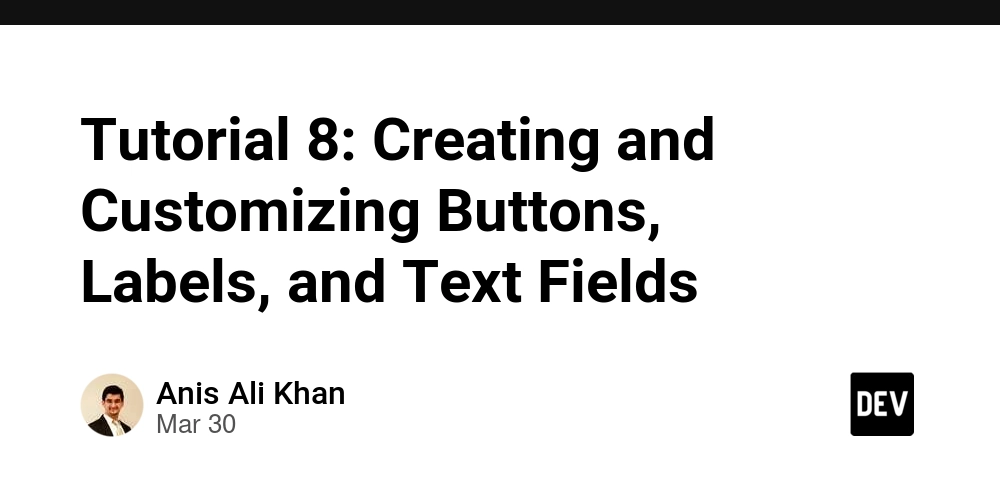
In this tutorial, we’ll build a fun and interactive “Random Joke Generator” app. The app will generate a random joke every time the user presses a Button, display it on a Label, and allow users to enter their name in a Text Field for a more personalized experience.
App Overview
Our app will:
- Have a Text Field where users can enter their name.
- Display a button labeled "Tell Me a Joke!".
- Upon pressing the button, the app will display a random joke on a Label, and address the user by name if they have entered one.
What You’ll Need
- Xcode installed on your macOS system
- Basic knowledge of Swift and iOS development
Step 1: Setting up the Xcode Project
- Open Xcode and create a new project.
- Choose the App template and click Next.
- Name your project RandomJokeGenerator and set the interface to Storyboard and the language to Swift.
- Click Next and save your project.
Step 2: Design the UI
- Open the Main.storyboard file.
- Drag the following components from the Object Library to the View Controller:
- Text Field for entering the user’s name.
- Button labeled “Tell Me a Joke!”.
- Label to display the random joke.
- Arrange them neatly in the view. Place the Text Field at the top, the Button below it, and the Label beneath the Button.
- Customize their properties:
- Text Field: Set the placeholder text to "Enter your name".
- Button: Change the title to “Tell Me a Joke!”.
- Label: Set the initial text to "Your joke will appear here!" and center the text.
Step 3: Link UI Components to Code
- Open the Assistant Editor in Xcode to view both the storyboard and the code.
-
Ctrl-drag the Text Field, Button, and Label from the storyboard to the
ViewController.swift
file to create IBOutlet and IBAction connections.- For the Text Field, create an IBOutlet named
nameTextField
. - For the Label, create an IBOutlet named
jokeLabel
. - For the Button, create an IBAction method named
tellMeAJokePressed
.
- For the Text Field, create an IBOutlet named
Your code should now have the following connections:
@IBOutlet weak var nameTextField: UITextField!
@IBOutlet weak var jokeLabel: UILabel!
@IBAction func tellMeAJokePressed(_ sender: UIButton) {
// We'll write the action code here in the next step
}
Step 4: Writing the Code
Now let’s implement the action when the button is pressed. The app should take the text entered in the Text Field, check if it’s not empty, and then display a random joke on the Label. If the user has entered their name, we’ll personalize the joke.
Here’s a list of jokes to pick from:
let jokes = [
"Why don't skeletons fight each other? They don't have the guts!",
"I told my computer I needed a break, and now it won’t stop sending me Kit-Kats.",
"Why don’t eggs tell jokes? They’d crack each other up.",
"Why did the coffee file a police report? It got mugged!",
"I told my wife she was drawing her eyebrows too high. She looked surprised!"
]
Now let’s implement the functionality in the tellMeAJokePressed
method:
@IBAction func tellMeAJokePressed(_ sender: UIButton) {
// Get the user's name if available
let userName = nameTextField.text ?? ""
// Generate a random joke
let randomJoke = jokes.randomElement()!
// Personalize the joke if the user has entered their name
if !userName.isEmpty {
jokeLabel.text = "\(userName), here’s your joke: \(randomJoke)"
} else {
jokeLabel.text = "Here’s your joke: \(randomJoke)"
}
}
Step 5: Customize UI Elements
Let’s make our app more fun and engaging by customizing the Button, Label, and Text Field.
-
Button Customization:
- Set the Button's background color to orange (for a cheerful look).
- Change the title color to white and make the button text bold.
- Add rounded corners for a smooth, modern appearance.
tellMeAJokeButton.layer.cornerRadius = 10
tellMeAJokeButton.backgroundColor = .orange
tellMeAJokeButton.setTitleColor(.white, for: .normal)
tellMeAJokeButton.titleLabel?.font = UIFont.boldSystemFont(ofSize: 16)
-
Label Customization:
- Set the Label's text color to dark blue to make the jokes stand out.
- Increase the font size and weight to make the text more prominent.
jokeLabel.textColor = .darkBlue
jokeLabel.font = UIFont.systemFont(ofSize: 20, weight: .medium)
-
Text Field Customization:
- Add some padding inside the Text Field for better user experience.
- Set a border around the Text Field to make it visually distinct.
nameTextField.layer.borderColor = UIColor.gray.cgColor
nameTextField.layer.borderWidth = 1
nameTextField.layer.cornerRadius = 5
nameTextField.paddingLeft = 10
Step 6: Run Your App
- Choose the simulator device (e.g., iPhone 13) from the Xcode toolbar.
- Press Cmd + R to build and run your app.
- In the simulator, enter your name in the Text Field, press the Tell Me a Joke! button, and see a random joke personalized with your name.
- If no name is entered, the joke will simply appear without personalization.
Conclusion
You've successfully built a Random Joke Generator app with Buttons, Labels, and Text Fields! Not only did you learn how to implement basic UI components, but you also personalized the experience by adding a fun twist to the app. You can extend the app further by adding more jokes, images, or animations for even more interactivity!
This app is a great starting point for creating fun and engaging apps that interact with the user in creative ways.