Turbocharge Dev Velocity: Modern Tooling & DX in the Node.js Ecosystem
Developer Experience (DX) is no longer just a buzzword—it's a competitive advantage. As the Node.js ecosystem matures and development cycles shorten, teams are seeking ways to ship high-quality software faster. But how do you actually speed up a Node.js workflow without sacrificing maintainability or developer happiness? Let’s walk through a progressive journey of modern tooling—tools and practices that, when combined thoughtfully, create a seamless, productive development experience. 1. Begin with Types: The Foundation of Confidence Let’s be honest—JavaScript is powerful, but it’s also prone to runtime surprises. That's where TypeScript comes in. If you're not already using it, ask yourself: Wouldn’t it be better to catch bugs while typing code instead of during production outages? Now, once you're on TypeScript, how can we level it up? Zod: Why define types in one place and validations in another? Zod lets you define your schema and get both validation and static types—one source of truth. It's declarative, concise, and inferable. tRPC: No more duplicating API types between backend and frontend. With tRPC, your frontend gets full type inference directly from your server. It’s TypeScript on steroids for APIs. tsup: Need to ship a package or microservice? tsup builds your TypeScript with blazing speed and zero-config. When was the last time your build tool just worked? These tools set the tone for a high-trust, low-friction coding experience. 2. Layer in Testing: Shift-Left with Zero-Config Confidence Types are great, but they don’t replace tests. Once you have static safety, it’s time to verify logic. But wait—does your test setup feel like more work than value? Vitest: Familiar like Jest, but faster and Vite-native. Imagine instant feedback, snapshot testing, and great DX—all without spending hours on configuration. Bun: This JavaScript runtime is incredibly fast and includes a built-in test runner. If you haven’t tried it yet, just ask yourself: What if testing wasn’t a chore? By removing the pain of testing setup, you create space for writing better, more thorough tests. 3. Standardize Environments: Eliminate “Works on My Machine” Even with great types and tests, what happens when the dev environment differs across your team? Dev Containers: Define your environment in code. One Dockerfile to rule them all. Whether you're onboarding a junior dev or switching machines, your project setup is now one Reopen in Container away. GitHub Codespaces: Need cloud-powered dev environments? Codespaces boot your project in the cloud, pre-configured and ready to code. Ever wondered how it would feel to onboard in minutes instead of days? These tools bring predictability and portability to your dev environments. 4. Supercharge with Smart Assistance: Pair with the Machines By now, you’ve laid the foundation: types, tests, consistent environments. But what about speed? Where can you squeeze more out of every keystroke? GitHub Copilot: It’s not just autocomplete—it’s a pair programmer on standby. Copilot suggests code, tests, even documentation. Use it with discernment, and it’ll boost your output dramatically. AI Test Writers & Linters: Tools like Codeium and Testim are now generating meaningful test cases and catching anti-patterns. Ask yourself: What if your IDE could nudge you before the code review? The future is collaborative—between humans and AI. Don’t ignore the shift. Real-World Reflection Let’s pause. Imagine a team using TypeScript + tRPC for tight API contracts, Vitest for blazing-fast tests, and Codespaces for shared environments. Every new team member is productive within an hour. Bugs are caught at the type level or during fast CI runs. AI handles boilerplate. Velocity isn’t just a metric—it’s visible in the team’s energy. This isn’t a dream stack. It’s happening today. Related Series You Might Like If you’re interested in taking these concepts even further: Code the Core: DDD with Node.js & NestJSLearn how to structure complex Node.js applications using Domain-Driven Design. Real-Time with Node.js: WebSocketsDive into real-world WebSocket patterns using native Node.js clients and the ws library. Conclusion Modern tooling is not about chasing trends—it’s about removing bottlenecks. By progressing from types, to tests, to consistent environments, and then to AI-enhanced development, you create a compound effect that accelerates every aspect of building with Node.js. So next time you're stuck in a sluggish dev flow, ask yourself: Which layer of my workflow needs a boost? Chances are, the tools are already here—waiting to be adopted.
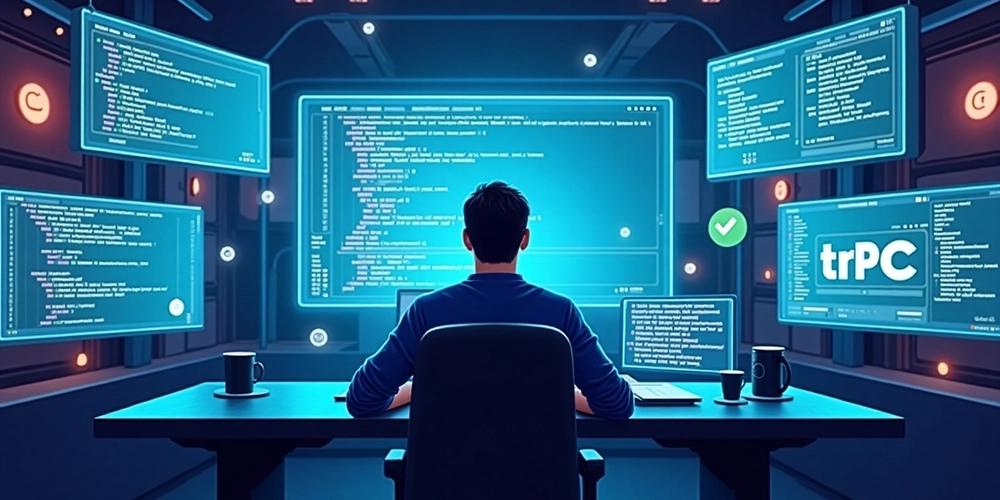
Developer Experience (DX) is no longer just a buzzword—it's a competitive advantage. As the Node.js ecosystem matures and development cycles shorten, teams are seeking ways to ship high-quality software faster. But how do you actually speed up a Node.js workflow without sacrificing maintainability or developer happiness?
Let’s walk through a progressive journey of modern tooling—tools and practices that, when combined thoughtfully, create a seamless, productive development experience.
1. Begin with Types: The Foundation of Confidence
Let’s be honest—JavaScript is powerful, but it’s also prone to runtime surprises. That's where TypeScript comes in. If you're not already using it, ask yourself: Wouldn’t it be better to catch bugs while typing code instead of during production outages?
Now, once you're on TypeScript, how can we level it up?
- Zod: Why define types in one place and validations in another? Zod lets you define your schema and get both validation and static types—one source of truth. It's declarative, concise, and inferable.
- tRPC: No more duplicating API types between backend and frontend. With tRPC, your frontend gets full type inference directly from your server. It’s TypeScript on steroids for APIs.
- tsup: Need to ship a package or microservice? tsup builds your TypeScript with blazing speed and zero-config. When was the last time your build tool just worked?
These tools set the tone for a high-trust, low-friction coding experience.
2. Layer in Testing: Shift-Left with Zero-Config Confidence
Types are great, but they don’t replace tests. Once you have static safety, it’s time to verify logic. But wait—does your test setup feel like more work than value?
- Vitest: Familiar like Jest, but faster and Vite-native. Imagine instant feedback, snapshot testing, and great DX—all without spending hours on configuration.
- Bun: This JavaScript runtime is incredibly fast and includes a built-in test runner. If you haven’t tried it yet, just ask yourself: What if testing wasn’t a chore?
By removing the pain of testing setup, you create space for writing better, more thorough tests.
3. Standardize Environments: Eliminate “Works on My Machine”
Even with great types and tests, what happens when the dev environment differs across your team?
- Dev Containers: Define your environment in code. One Dockerfile to rule them all. Whether you're onboarding a junior dev or switching machines, your project setup is now one Reopen in Container away.
- GitHub Codespaces: Need cloud-powered dev environments? Codespaces boot your project in the cloud, pre-configured and ready to code. Ever wondered how it would feel to onboard in minutes instead of days?
These tools bring predictability and portability to your dev environments.
4. Supercharge with Smart Assistance: Pair with the Machines
By now, you’ve laid the foundation: types, tests, consistent environments. But what about speed? Where can you squeeze more out of every keystroke?
- GitHub Copilot: It’s not just autocomplete—it’s a pair programmer on standby. Copilot suggests code, tests, even documentation. Use it with discernment, and it’ll boost your output dramatically.
- AI Test Writers & Linters: Tools like Codeium and Testim are now generating meaningful test cases and catching anti-patterns. Ask yourself: What if your IDE could nudge you before the code review?
The future is collaborative—between humans and AI. Don’t ignore the shift.
Real-World Reflection
Let’s pause. Imagine a team using TypeScript + tRPC for tight API contracts, Vitest for blazing-fast tests, and Codespaces for shared environments. Every new team member is productive within an hour. Bugs are caught at the type level or during fast CI runs. AI handles boilerplate. Velocity isn’t just a metric—it’s visible in the team’s energy.
This isn’t a dream stack. It’s happening today.
Related Series You Might Like
If you’re interested in taking these concepts even further:
- Code the Core: DDD with Node.js & NestJSLearn how to structure complex Node.js applications using Domain-Driven Design.
- Real-Time with Node.js: WebSocketsDive into real-world WebSocket patterns using native Node.js clients and the ws library.
Conclusion
Modern tooling is not about chasing trends—it’s about removing bottlenecks.
By progressing from types, to tests, to consistent environments, and then to AI-enhanced development, you create a compound effect that accelerates every aspect of building with Node.js.
So next time you're stuck in a sluggish dev flow, ask yourself: Which layer of my workflow needs a boost? Chances are, the tools are already here—waiting to be adopted.