The Basics of PHP for Web Development
The Basics of PHP for Web Development PHP (Hypertext Preprocessor) is one of the most widely used server-side scripting languages for web development. It powers over 77% of all websites with a known server-side programming language, including major platforms like WordPress, Facebook (initially), and Wikipedia. If you're looking to dive into web development or enhance your programming skills, PHP is an excellent place to start. In this article, we’ll cover the basics of PHP, its key features, and how you can use it to build dynamic websites. And if you're looking to monetize your web programming skills, consider exploring opportunities like MillionFormula. What is PHP? PHP is an open-source scripting language designed for web development but also used as a general-purpose programming language. It is embedded within HTML and executed on the server, generating dynamic content for the client’s browser. PHP is known for its simplicity, flexibility, and seamless integration with databases like MySQL, making it a go-to choice for developers. Why Use PHP? Ease of Learning: PHP has a gentle learning curve, making it beginner-friendly. Its syntax is similar to C and Java, so if you’re familiar with those languages, you’ll pick up PHP quickly. Cross-Platform Compatibility: PHP runs on various platforms (Windows, Linux, macOS) and supports almost all web servers (Apache, IIS, etc.). Database Integration: PHP works seamlessly with databases like MySQL, PostgreSQL, and MongoDB, enabling you to build data-driven applications. Large Community: With a massive developer community, PHP has extensive documentation, tutorials, and frameworks like Laravel and Symfony. Cost-Effective: Being open-source, PHP is free to use, reducing development costs. Setting Up PHP To start coding in PHP, you’ll need a local development environment. Tools like XAMPP or WAMP provide a complete stack, including PHP, Apache (web server), and MySQL (database). Once installed, create a .php file (e.g., index.php) in your web server’s root directory. Here’s a simple "Hello, World!" example: php Copy Save the file and open it in your browser via http://localhost/index.php. You’ll see "Hello, World!" displayed. PHP Syntax Basics PHP code is enclosed within tags. Here are some fundamental concepts: Variables Variables in PHP start with a $ sign. They are loosely typed, meaning you don’t need to declare their data type explicitly. php Copy Conditional Statements PHP supports if, else, and switch statements for decision-making. php Copy Loops PHP provides for, while, and foreach loops for repetitive tasks. php Copy Functions Functions allow you to encapsulate reusable code. php Copy Working with Forms PHP is commonly used to handle form data. Here’s an example of a simple HTML form and PHP script to process it: HTML Form: html Copy Name: Email: Run HTML PHP Script (process.php): php Copy Database Integration with MySQL PHP’s integration with MySQL is one of its strongest features. Here’s how you can connect to a MySQL database and fetch data: php Copy PHP Frameworks While PHP is powerful on its own, frameworks like Laravel, Symfony, and CodeIgniter simplify development by providing built-in tools for routing, authentication, and database management. Monetizing Your PHP Skills Once you’ve mastered PHP, you can leverage your skills to build websites, web applications, or even freelance. If you’re looking for a structured way to monetize your programming expertise, check out MillionFormula. It’s a great platform to explore opportunities and turn your coding skills into a profitable venture. Conclusion PHP remains a cornerstone of web development due to its simplicity, versatility, and robust community support. Whether you’re building a small personal blog or a large-scale e-commerce platform, PHP has the tools and resources to help you succeed. Start experimenting with the basics, explore frameworks, and consider how you can turn your skills into a career. Happy coding!
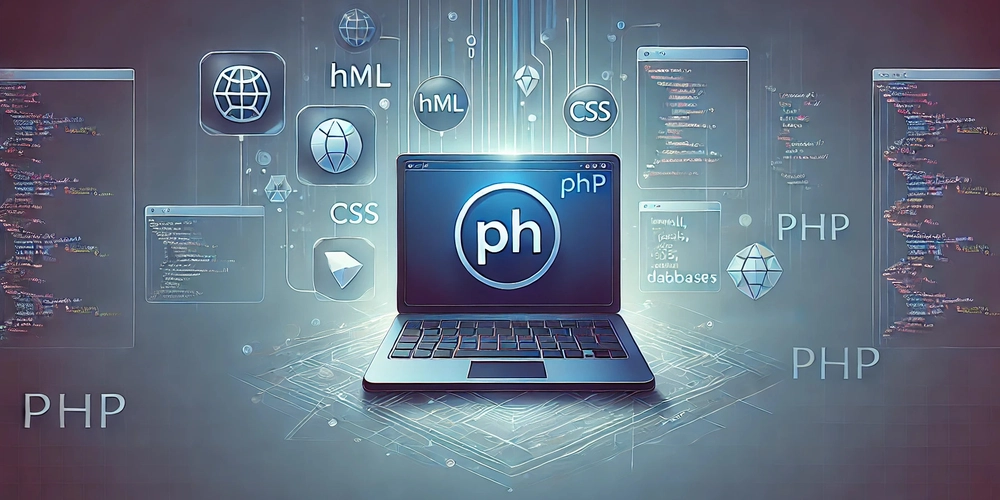
The Basics of PHP for Web Development
PHP (Hypertext Preprocessor) is one of the most widely used server-side scripting languages for web development. It powers over 77% of all websites with a known server-side programming language, including major platforms like WordPress, Facebook (initially), and Wikipedia. If you're looking to dive into web development or enhance your programming skills, PHP is an excellent place to start. In this article, we’ll cover the basics of PHP, its key features, and how you can use it to build dynamic websites. And if you're looking to monetize your web programming skills, consider exploring opportunities like MillionFormula.
What is PHP?
PHP is an open-source scripting language designed for web development but also used as a general-purpose programming language. It is embedded within HTML and executed on the server, generating dynamic content for the client’s browser. PHP is known for its simplicity, flexibility, and seamless integration with databases like MySQL, making it a go-to choice for developers.
Why Use PHP?
- Ease of Learning: PHP has a gentle learning curve, making it beginner-friendly. Its syntax is similar to C and Java, so if you’re familiar with those languages, you’ll pick up PHP quickly.
- Cross-Platform Compatibility: PHP runs on various platforms (Windows, Linux, macOS) and supports almost all web servers (Apache, IIS, etc.).
- Database Integration: PHP works seamlessly with databases like MySQL, PostgreSQL, and MongoDB, enabling you to build data-driven applications.
- Large Community: With a massive developer community, PHP has extensive documentation, tutorials, and frameworks like Laravel and Symfony.
- Cost-Effective: Being open-source, PHP is free to use, reducing development costs.
Setting Up PHP
To start coding in PHP, you’ll need a local development environment. Tools like XAMPP or WAMP provide a complete stack, including PHP, Apache (web server), and MySQL (database).
Once installed, create a .php
file (e.g., index.php
) in your web server’s root directory. Here’s a simple "Hello, World!" example:
php
Copy
echo "Hello, World!";
?>
Save the file and open it in your browser via
http://localhost/index.php
. You’ll see "Hello, World!" displayed.
PHP Syntax Basics
PHP code is enclosed within tags. Here are some fundamental concepts:
Variables
Variables in PHP start with a $
sign. They are loosely typed, meaning you don’t need to declare their data type explicitly.
php
Copy
$name = "John Doe";
$age = 25;
echo "My name is $name and I am $age years old.";
?>
Conditional Statements
PHP supports if
, else
, and switch
statements for decision-making.
php
Copy
$score = 85;
if ($score >= 90) {
echo "Excellent!";
} elseif ($score >= 70) {
echo "Good job!";
} else {
echo "Keep trying!";
}
?>
Loops
PHP provides for
, while
, and foreach
loops for repetitive tasks.
php
Copy
for ($i = 1; $i <= 5; $i++) {
echo "Iteration $i
";
}
?>
Functions
Functions allow you to encapsulate reusable code.
php
Copy
function greet($name) {
return "Hello, $name!";
}
echo greet("Alice");
?>
Working with Forms
PHP is commonly used to handle form data. Here’s an example of a simple HTML form and PHP script to process it:
HTML Form:
html
Copy
<form action="process.php" method="post"> Name: <input type="text" name="name"> Email: <input type="email" name="email"> <input type="submit" value="Submit"> form>Run HTML
PHP Script (process.php): php Copy
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = $_POST['name'];
$email = $_POST['email'];
echo "Hello, $name! Your email is $email.";
}
?>
Database Integration with MySQL
PHP’s integration with MySQL is one of its strongest features. Here’s how you can connect to a MySQL database and fetch data:
php
Copy
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myDatabase";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Fetch data
$sql = "SELECT id, name FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"]. " - Name: " . $row["name"]. "
";
}
} else {
echo "0 results";
}
$conn->close();
?>
PHP Frameworks
While PHP is powerful on its own, frameworks like Laravel, Symfony, and CodeIgniter simplify development by providing built-in tools for routing, authentication, and database management.
Monetizing Your PHP Skills
Once you’ve mastered PHP, you can leverage your skills to build websites, web applications, or even freelance. If you’re looking for a structured way to monetize your programming expertise, check out MillionFormula. It’s a great platform to explore opportunities and turn your coding skills into a profitable venture.
Conclusion
PHP remains a cornerstone of web development due to its simplicity, versatility, and robust community support. Whether you’re building a small personal blog or a large-scale e-commerce platform, PHP has the tools and resources to help you succeed. Start experimenting with the basics, explore frameworks, and consider how you can turn your skills into a career. Happy coding!