Simulating GCash's Luck Load Game with Minimal Ruby Code
If you've ever participated in a lottery, raffle, or lucky draw, you might have wondered how many possible outcomes exist. In Ruby, three powerful methods—product, combination, and sample—make calculating and generating these possibilities simple. In this post, we'll explore how to use these methods to simulate games like GCash Lucky Load and the Super Lotto 6/49 lottery. By the end, you'll understand how to efficiently model scenarios like selecting 6 unique pairs from a set of options or picking 6 numbers out of 49 for a lottery. Understanding product, combination, and sample product: Generating All Possible Combinations The product method in Ruby creates all possible pairings (or combinations) of elements from multiple arrays. This is particularly useful when combining two distinct sets of options, such as colors and icons. For example, given an array of colors and an array of icons, product generates every possible color-icon pair: colors = %w[Green Orange Red Purple Teal] icons = %w[Crown Diamond Gift Thumb Ticket Trophy] combinations = colors.product(icons) puts combinations.inspect #=> [["Green", "Crown"], ["Green", "Diamond"], ...] This results in 30 unique color-icon pairs (5 × 6). combination: Selecting Unique Groups Once you've generated all possible pairs, combination(n) allows you to create unique groups of n items where the order doesn’t matter. This is perfect for simulating draws where only a subset of items is chosen. draws = combinations.combination(6).to_a puts "Total possible 6-draw combinations: #{draws.size}" #=> Total possible 6-draw combinations: 593,775 sample: Simulating Random Draws The sample method is useful for randomly selecting a single winning draw, simulating a real-world lottery or prize selection: winning_draw = combinations.combination(6).to_a.sample puts "Winning Draw: #{winning_draw.inspect}" #=> [["Orange", "Gift"], ["Red", "Crown"], ...] Now that we understand these methods, let’s apply them to simulate a GCash Lucky Load game. Simulating GCash's Lucky Load Game Step 1: Define the Available Choices We set up two arrays: one for colors and another for icons. colors = %w[Green Orange Red Purple Teal] icons = %w[Crown Diamond Gift Thumb Ticket Trophy] Step 2: Generate All Possible Color-Icon Combinations Using product, we generate every possible pairing: all_pairs = colors.product(icons) puts "Total unique color-icon pairs: #{all_pairs.size}" #=> Total unique color-icon pairs: 30 Step 3: Simulate a 6-Pair Lucky Draw To simulate a game round, we randomly select 6 unique pairs from the 30 available. draws = all_pairs.combination(6).to_a winning_draw = draws.sample puts "Winning combination: #{winning_draw.inspect}" Step 4: Display the Winning Draw in a Grid For better visualization, we format the output: col_width = 8 puts " " * col_width + colors.map { |c| c.center(col_width) }.join icons.each do |icon| row = icon.ljust(col_width) colors.each do |color| mark = winning_draw.include?([color, icon]) ? 'x' : 'o' row += mark.center(col_width) end puts row end puts "\nWinning selection:" winning_draw.each { |color, icon| puts "x #{color}-#{icon}" } This outputs a clear representation of the winning draw. Green Orange Red Purple Teal Crown o o o o o Diamond o x o o x Gift o o o x o Thumb o x o o o Ticket o o x x o Trophy o o o o o Winning selection: x Orange-Diamond x Orange-Thumb x Red-Ticket x Purple-Gift x Purple-Ticket x Teal-Diamond Applying This to Other Games These methods extend beyond just color-icon games. Here are some additional applications: Lotto (Pick 6 out of 49 Numbers) Ever wondered about your odds in a standard Super Lotto 6/49 game? With 13,983,816 possible combinations, here’s how to generate a winning draw: numbers = (1..49).to_a winning_draw = numbers.combination(6).to_a.sample puts "Winning Lotto Draw: #{winning_draw.inspect}" Fantasy Sports Drafts Use combination to simulate drafting teams of players from a pool. Card Games Generate random hands by combining cards from a deck. Prize Draws Ensure fairness by randomly selecting winners from a list of participants. Why Use product, combination, and sample? Ruby’s product, combination, and sample methods offer efficient ways to generate, analyze, and randomly select from large sets of possibilities. Whether you're designing a game, running a raffle, or calculating probabilities, these methods allow you to: Quickly calculate total possible outcomes. Simulate random draws or selections. Test different scenarios
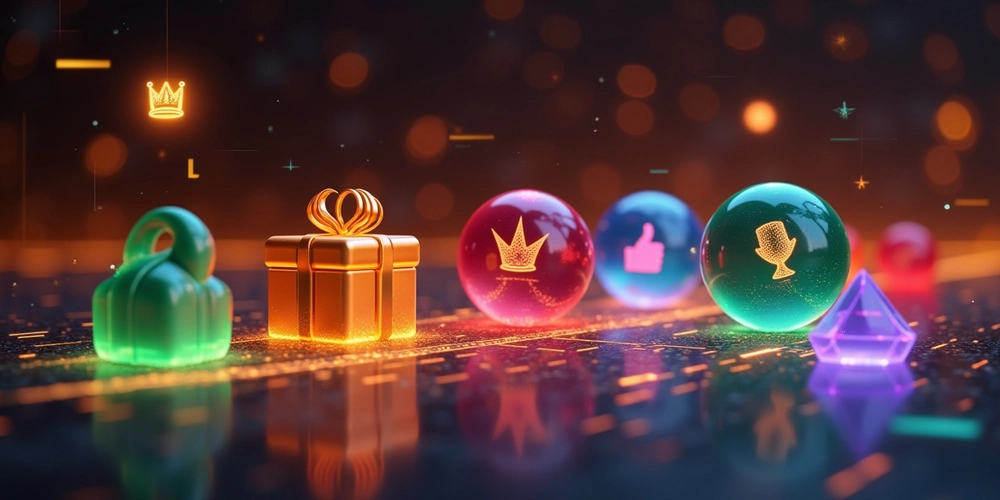
If you've ever participated in a lottery, raffle, or lucky draw, you might have wondered how many possible outcomes exist. In Ruby, three powerful methods—product
, combination
, and sample
—make calculating and generating these possibilities simple. In this post, we'll explore how to use these methods to simulate games like GCash Lucky Load and the Super Lotto 6/49 lottery. By the end, you'll understand how to efficiently model scenarios like selecting 6 unique pairs from a set of options or picking 6 numbers out of 49 for a lottery.
Understanding product
, combination
, and sample
product
: Generating All Possible Combinations
The product
method in Ruby creates all possible pairings (or combinations) of elements from multiple arrays. This is particularly useful when combining two distinct sets of options, such as colors and icons.
For example, given an array of colors and an array of icons, product
generates every possible color-icon pair:
colors = %w[Green Orange Red Purple Teal]
icons = %w[Crown Diamond Gift Thumb Ticket Trophy]
combinations = colors.product(icons)
puts combinations.inspect
#=> [["Green", "Crown"], ["Green", "Diamond"], ...]
This results in 30 unique color-icon pairs (5 × 6).
combination
: Selecting Unique Groups
Once you've generated all possible pairs, combination(n)
allows you to create unique groups of n
items where the order doesn’t matter. This is perfect for simulating draws where only a subset of items is chosen.
draws = combinations.combination(6).to_a
puts "Total possible 6-draw combinations: #{draws.size}"
#=> Total possible 6-draw combinations: 593,775
sample
: Simulating Random Draws
The sample
method is useful for randomly selecting a single winning draw, simulating a real-world lottery or prize selection:
winning_draw = combinations.combination(6).to_a.sample
puts "Winning Draw: #{winning_draw.inspect}"
#=> [["Orange", "Gift"], ["Red", "Crown"], ...]
Now that we understand these methods, let’s apply them to simulate a GCash Lucky Load game.
Simulating GCash's Lucky Load Game
Step 1: Define the Available Choices
We set up two arrays: one for colors and another for icons.
colors = %w[Green Orange Red Purple Teal]
icons = %w[Crown Diamond Gift Thumb Ticket Trophy]
Step 2: Generate All Possible Color-Icon Combinations
Using product
, we generate every possible pairing:
all_pairs = colors.product(icons)
puts "Total unique color-icon pairs: #{all_pairs.size}"
#=> Total unique color-icon pairs: 30
Step 3: Simulate a 6-Pair Lucky Draw
To simulate a game round, we randomly select 6 unique pairs from the 30 available.
draws = all_pairs.combination(6).to_a
winning_draw = draws.sample
puts "Winning combination: #{winning_draw.inspect}"
Step 4: Display the Winning Draw in a Grid
For better visualization, we format the output:
col_width = 8
puts " " * col_width + colors.map { |c| c.center(col_width) }.join
icons.each do |icon|
row = icon.ljust(col_width)
colors.each do |color|
mark = winning_draw.include?([color, icon]) ? 'x' : 'o'
row += mark.center(col_width)
end
puts row
end
puts "\nWinning selection:"
winning_draw.each { |color, icon| puts "x #{color}-#{icon}" }
This outputs a clear representation of the winning draw.
Green Orange Red Purple Teal
Crown o o o o o
Diamond o x o o x
Gift o o o x o
Thumb o x o o o
Ticket o o x x o
Trophy o o o o o
Winning selection:
x Orange-Diamond
x Orange-Thumb
x Red-Ticket
x Purple-Gift
x Purple-Ticket
x Teal-Diamond
Applying This to Other Games
These methods extend beyond just color-icon games. Here are some additional applications:
Lotto (Pick 6 out of 49 Numbers)
Ever wondered about your odds in a standard Super Lotto 6/49 game? With 13,983,816 possible combinations, here’s how to generate a winning draw:
numbers = (1..49).to_a
winning_draw = numbers.combination(6).to_a.sample
puts "Winning Lotto Draw: #{winning_draw.inspect}"
Fantasy Sports Drafts
Use combination
to simulate drafting teams of players from a pool.
Card Games
Generate random hands by combining cards from a deck.
Prize Draws
Ensure fairness by randomly selecting winners from a list of participants.
Why Use product
, combination
, and sample
?
Ruby’s product
, combination
, and sample
methods offer efficient ways to generate, analyze, and randomly select from large sets of possibilities. Whether you're designing a game, running a raffle, or calculating probabilities, these methods allow you to:
- Quickly calculate total possible outcomes.
- Simulate random draws or selections.
- Test different scenarios without manually listing all possibilities.
Conclusion
Ruby’s product
and combination
methods are powerful tools for simulating real-world games and scenarios. By combining them with sample
, you can efficiently generate all possible outcomes and calculate probabilities. Whether you're designing a lucky draw, organizing a raffle, or building a card game, these methods make the process straightforward and fun.
Try experimenting with different sets and numbers to see how the odds change—and let us know what creative applications you come up with!
Final Notes:
- The example above uses a 6-pair draw, but you can adjust this based on your game’s rules.
- For larger datasets, consider using lazy evaluation (
lazy
) to optimize memory usage.
Happy coding!