8 Key Principles for Effective RESTful APIs in Node.js
Building a RESTful API in Node.js is easy, but building an effective one? That’s a different challenge. Whether you're developing an API for an enterprise application or a simple SaaS product, designing it with best practices in mind ensures performance, security, and maintainability. 1. Follow RESTful Resource Naming Conventions A good RESTful API starts with clear and consistent resource naming. Your API should use nouns instead of verbs when defining endpoints. Bad Example: POST /createUser GET /getUserData PUT /updateUserProfile DELETE /removeUser Good Example: POST /users GET /users/{id} PUT /users/{id} DELETE /users/{id} Each resource represents a real-world entity (users, products, orders, etc.), and HTTP methods define the operations. GET → Retrieve data POST → Create new data PUT → Update existing data DELETE → Remove data Sticking to these conventions makes APIs predictable and easier to use. 2. Use Proper HTTP Status Codes Many APIs return a 200 OK for everything, which is a bad practice. HTTP status codes should communicate what happened clearly. Common HTTP Status Codes: 200 OK → Successful GET request 201 Created → Successful POST request 204 No Content → Successful DELETE request with no response body 400 Bad Request → Invalid input from client 401 Unauthorized → Authentication failure 403 Forbidden → Authorization failure 404 Not Found → Resource doesn’t exist 500 Internal Server Error → Unexpected server-side issue Example Response: { "error": "User not found", "statusCode": 404 } This makes debugging easier for developers and improves API usability. 3. Implement Authentication and Authorization Security is non-negotiable in APIs. You don’t want unauthorized users accessing sensitive data. Use authentication and authorization mechanisms properly. Authentication Options: JWT (JSON Web Token) – Ideal for stateless authentication OAuth 2.0 – Great for third-party integrations API Keys – Simple but less secure Example: Protecting Routes with JWT in Express const jwt = require("jsonwebtoken"); const authenticateToken = (req, res, next) => { const token = req.header("Authorization")?.split(" ")[1]; if (!token) return res.status(401).json({ error: "Access Denied" }); jwt.verify(token, process.env.JWT_SECRET, (err, user) => { if (err) return res.status(403).json({ error: "Invalid Token" }); req.user = user; next(); }); }; // Protect routes app.get("/dashboard", authenticateToken, (req, res) => { res.json({ message: "Welcome to Dashboard!" }); }); Here, the authenticateToken middleware ensures only authenticated users can access the /dashboard route. 4. Rate Limiting to Prevent Abuse APIs can be abused by bots, scrapers, or brute-force attackers. Rate limiting prevents excessive requests from a single user/IP within a short time. Using express-rate-limit: const rateLimit = require("express-rate-limit"); const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // Limit each IP to 100 requests per window message: "Too many requests, please try again later.", }); app.use("/api/", limiter); This ensures a user cannot spam your API with excessive requests. 5. Proper Pagination for Large Datasets When dealing with large data (users, products, orders), returning all records at once is inefficient. Instead, implement pagination. Example: Paginated Users API app.get("/users", async (req, res) => { const { page = 1, limit = 10 } = req.query; const users = await User.find() .skip((page - 1) * limit) .limit(Number(limit)); res.json(users); }); Pagination Query Parameters: GET /users?page=2&limit=10 Returns page 2 with 10 users per page. 6. Use API Versioning APIs evolve over time. If you introduce breaking changes, older clients might break. The solution? API versioning. Methods of API Versioning: URL versioning (Most common) GET /v1/users GET /v2/users Header-based versioning GET /users Headers: X-API-Version: v2 Example in Express.js: app.use("/v1", require("./routes/v1")); app.use("/v2", require("./routes/v2")); This ensures older clients can still use the API without breaking. 7. Enable CORS for Cross-Domain Access APIs often serve frontend applications, and without CORS (Cross-Origin Resource Sharing), requests from different origins will be blocked. Enable CORS in Express.js: const cors = require("cors"); app.use(cors({ origin: "https://yourfrontend.com", methods: "GET,POST,PUT,DELETE", credentials: true, })); This ensures only trusted origins can call your API. 8. Structured and Meaningful Error Handling APIs should return clear error messages so developers can debug issues
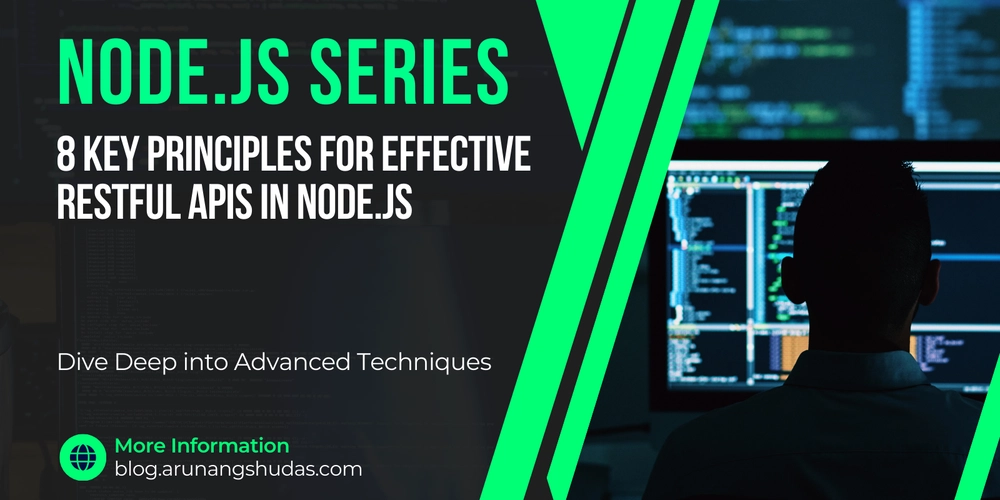
Building a RESTful API in Node.js is easy, but building an effective one? That’s a different challenge. Whether you're developing an API for an enterprise application or a simple SaaS product, designing it with best practices in mind ensures performance, security, and maintainability.
1. Follow RESTful Resource Naming Conventions
A good RESTful API starts with clear and consistent resource naming. Your API should use nouns instead of verbs when defining endpoints.
Bad Example:
POST /createUser
GET /getUserData
PUT /updateUserProfile
DELETE /removeUser
Good Example:
POST /users
GET /users/{id}
PUT /users/{id}
DELETE /users/{id}
Each resource represents a real-world entity (users
, products
, orders
, etc.), and HTTP methods define the operations.
- GET → Retrieve data
- POST → Create new data
- PUT → Update existing data
- DELETE → Remove data
Sticking to these conventions makes APIs predictable and easier to use.
2. Use Proper HTTP Status Codes
Many APIs return a 200 OK for everything, which is a bad practice. HTTP status codes should communicate what happened clearly.
Common HTTP Status Codes:
- 200 OK → Successful GET request
- 201 Created → Successful POST request
- 204 No Content → Successful DELETE request with no response body
- 400 Bad Request → Invalid input from client
- 401 Unauthorized → Authentication failure
- 403 Forbidden → Authorization failure
- 404 Not Found → Resource doesn’t exist
- 500 Internal Server Error → Unexpected server-side issue
Example Response:
{
"error": "User not found",
"statusCode": 404
}
This makes debugging easier for developers and improves API usability.
3. Implement Authentication and Authorization
Security is non-negotiable in APIs. You don’t want unauthorized users accessing sensitive data. Use authentication and authorization mechanisms properly.
Authentication Options:
- JWT (JSON Web Token) – Ideal for stateless authentication
- OAuth 2.0 – Great for third-party integrations
- API Keys – Simple but less secure
Example: Protecting Routes with JWT in Express
const jwt = require("jsonwebtoken");
const authenticateToken = (req, res, next) => {
const token = req.header("Authorization")?.split(" ")[1];
if (!token) return res.status(401).json({ error: "Access Denied" });
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) return res.status(403).json({ error: "Invalid Token" });
req.user = user;
next();
});
};
// Protect routes
app.get("/dashboard", authenticateToken, (req, res) => {
res.json({ message: "Welcome to Dashboard!" });
});
Here, the authenticateToken middleware ensures only authenticated users can access the /dashboard
route.
4. Rate Limiting to Prevent Abuse
APIs can be abused by bots, scrapers, or brute-force attackers. Rate limiting prevents excessive requests from a single user/IP within a short time.
Using express-rate-limit
:
const rateLimit = require("express-rate-limit");
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per window
message: "Too many requests, please try again later.",
});
app.use("/api/", limiter);
This ensures a user cannot spam your API with excessive requests.
5. Proper Pagination for Large Datasets
When dealing with large data (users, products, orders), returning all records at once is inefficient. Instead, implement pagination.
Example: Paginated Users API
app.get("/users", async (req, res) => {
const { page = 1, limit = 10 } = req.query;
const users = await User.find()
.skip((page - 1) * limit)
.limit(Number(limit));
res.json(users);
});
Pagination Query Parameters:
GET /users?page=2&limit=10
Returns page 2 with 10 users per page.
6. Use API Versioning
APIs evolve over time. If you introduce breaking changes, older clients might break. The solution? API versioning.
Methods of API Versioning:
- URL versioning (Most common)
GET /v1/users
GET /v2/users
- Header-based versioning
GET /users
Headers: X-API-Version: v2
Example in Express.js:
app.use("/v1", require("./routes/v1"));
app.use("/v2", require("./routes/v2"));
This ensures older clients can still use the API without breaking.
7. Enable CORS for Cross-Domain Access
APIs often serve frontend applications, and without CORS (Cross-Origin Resource Sharing), requests from different origins will be blocked.
Enable CORS in Express.js:
const cors = require("cors");
app.use(cors({
origin: "https://yourfrontend.com",
methods: "GET,POST,PUT,DELETE",
credentials: true,
}));
This ensures only trusted origins can call your API.
8. Structured and Meaningful Error Handling
APIs should return clear error messages so developers can debug issues quickly.
Bad Example:
{
"error": "Something went wrong"
}
Good Example:
{
"error": "Invalid email format",
"field": "email",
"statusCode": 400
}
Centralized Error Handling in Express.js:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(err.statusCode || 500).json({
error: err.message || "Internal Server Error",
});
});
Now, all errors will be handled gracefully.
Quick Recap:
→ Follow proper RESTful resource naming
→ Use HTTP status codes correctly
→ Implement authentication & authorization
→ Prevent abuse with rate limiting
→ Use pagination for large datasets
→ Implement API versioning
→ Enable CORS for cross-domain access
→ Provide structured error handling
You may also like:
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin