Simplify Video Frame Rate Adjustment in Rust: A Practical Guide for Developers
Introduction Adjusting video frame rates (FPS) is a common task in video processing, whether to ensure smooth playback or meet specific platform requirements. For instance, converting a 60 FPS video to 30 FPS might be necessary for compatibility or efficiency. While the FFmpeg command-line tool (ffmpeg -i input.mp4 -r 30 output.mp4) is a popular choice, integrating this functionality directly into Rust projects can offer more control and automation. In Rust, calling FFmpeg’s C libraries via FFI (Foreign Function Interface) provides access to its full capabilities, though it can be complex and time-consuming. For simpler tasks like frame rate adjustments, ez-ffmpeg offers a more straightforward approach. Built on top of FFI, it provides a user-friendly interface that streamlines common video processing tasks, making it a practical option for developers looking to avoid the intricacies of direct FFI calls. FFI vs. ez-ffmpeg: Choosing the Right Tool The Challenges of FFI FFI allows direct interaction with FFmpeg’s C libraries, offering maximum flexibility: Full Control: Access every feature of FFmpeg, ideal for advanced use cases. Complexity: Requires managing structs, pointers, and memory manually, which can lead to verbose and error-prone code. Steep Learning Curve: Developers need a solid understanding of FFmpeg’s low-level API. For example, adjusting frame rates via FFI involves configuring AVCodecContext and AVFrame, where mistakes can easily lead to issues. How ez-ffmpeg Helps ez-ffmpeg is designed to simplify common tasks without replacing FFI entirely: Ease of Use: Its API mirrors FFmpeg command-line syntax, making it intuitive for those familiar with the tool. Safety: Handles memory management automatically, reducing risks. Focus: Lets developers concentrate on application logic rather than FFmpeg internals. While FFI is better for intricate, custom workflows, ez-ffmpeg shines in scenarios where simplicity and speed are priorities, such as basic frame rate adjustments. Get Started: Adjust Video Frame Rates in Rust Let’s say you need to convert a 60 FPS video to 30 FPS. Here’s how ez-ffmpeg can help. 1. Install FFmpeg macOS: brew install ffmpeg Windows: vcpkg install ffmpeg # Set the VCPKG_ROOT environment variable if using vcpkg for the first time 2. Add the Rust Dependency In your Cargo.toml: [dependencies] ez-ffmpeg = "*" 3. Code Examples Below are two ways to adjust frame rates using ez-ffmpeg: Method 1: Set Frame Rate via Output use ez_ffmpeg::{FfmpegContext, Output}; use ffmpeg_sys_next::AVRational; fn main() -> Result { FfmpegContext::builder() .input("test.mp4") // Input file .output(Output::from("output.mp4").set_framerate(AVRational { num: 30, den: 1 })) // Set to 30 FPS .build()? .start()? .wait()?; Ok(()) } Method 2: Use a Filter to Set Frame Rate use ez_ffmpeg::{FfmpegContext, Output}; fn main() -> Result { FfmpegContext::builder() .input("test.mp4") // Input file .filter_desc("fps=30") // Use "fps" filter to set 30 FPS .output(Output::from("output.mp4")) // Output file .build()? .start()? .wait()?; Ok(()) } When to Use Each Method Method 1: Straightforward for basic frame rate changes. Best when that’s the only adjustment needed. Method 2: More flexible, allowing frame rate changes alongside other filters (e.g., resizing). Ideal for combined operations. Both methods produce a 30 FPS output.mp4 from test.mp4. Conclusion For Rust developers working with video, ez-ffmpeg provides a convenient way to handle tasks like frame rate adjustments. It builds on FFI to offer a simpler, safer interface for common operations, complementing the power of direct FFmpeg calls. While it’s not a solution for every scenario, it can be a valuable tool in your toolkit, especially when ease of use is a priority.
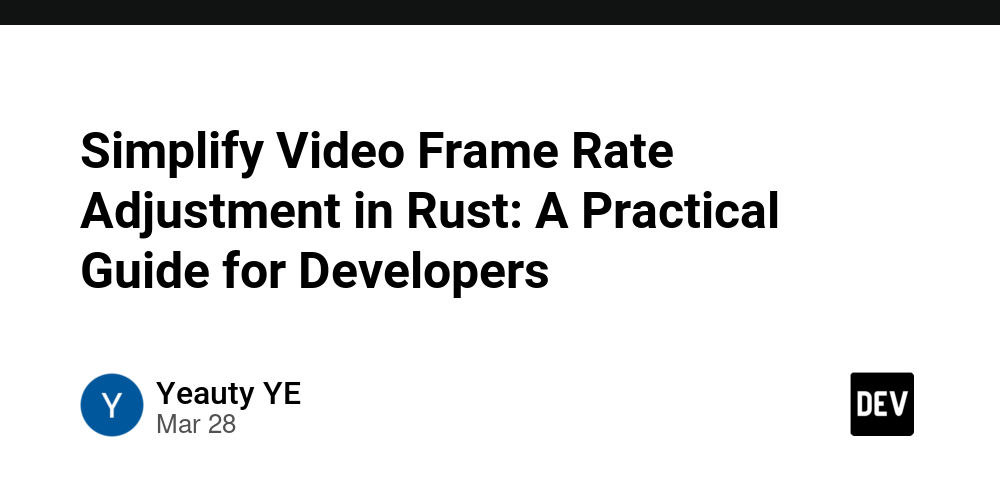
Introduction
Adjusting video frame rates (FPS) is a common task in video processing, whether to ensure smooth playback or meet specific platform requirements. For instance, converting a 60 FPS video to 30 FPS might be necessary for compatibility or efficiency. While the FFmpeg command-line tool (ffmpeg -i input.mp4 -r 30 output.mp4
) is a popular choice, integrating this functionality directly into Rust projects can offer more control and automation.
In Rust, calling FFmpeg’s C libraries via FFI (Foreign Function Interface) provides access to its full capabilities, though it can be complex and time-consuming. For simpler tasks like frame rate adjustments, ez-ffmpeg
offers a more straightforward approach. Built on top of FFI, it provides a user-friendly interface that streamlines common video processing tasks, making it a practical option for developers looking to avoid the intricacies of direct FFI calls.
FFI vs. ez-ffmpeg: Choosing the Right Tool
The Challenges of FFI
FFI allows direct interaction with FFmpeg’s C libraries, offering maximum flexibility:
- Full Control: Access every feature of FFmpeg, ideal for advanced use cases.
- Complexity: Requires managing structs, pointers, and memory manually, which can lead to verbose and error-prone code.
- Steep Learning Curve: Developers need a solid understanding of FFmpeg’s low-level API.
For example, adjusting frame rates via FFI involves configuring AVCodecContext
and AVFrame
, where mistakes can easily lead to issues.
How ez-ffmpeg Helps
ez-ffmpeg
is designed to simplify common tasks without replacing FFI entirely:
- Ease of Use: Its API mirrors FFmpeg command-line syntax, making it intuitive for those familiar with the tool.
- Safety: Handles memory management automatically, reducing risks.
- Focus: Lets developers concentrate on application logic rather than FFmpeg internals.
While FFI is better for intricate, custom workflows, ez-ffmpeg
shines in scenarios where simplicity and speed are priorities, such as basic frame rate adjustments.
Get Started: Adjust Video Frame Rates in Rust
Let’s say you need to convert a 60 FPS video to 30 FPS. Here’s how ez-ffmpeg
can help.
1. Install FFmpeg
macOS:
brew install ffmpeg
Windows:
vcpkg install ffmpeg
# Set the VCPKG_ROOT environment variable if using vcpkg for the first time
2. Add the Rust Dependency
In your Cargo.toml
:
[dependencies]
ez-ffmpeg = "*"
3. Code Examples
Below are two ways to adjust frame rates using ez-ffmpeg
:
Method 1: Set Frame Rate via Output
use ez_ffmpeg::{FfmpegContext, Output};
use ffmpeg_sys_next::AVRational;
fn main() -> Result<(), Box<dyn std::error::Error>> {
FfmpegContext::builder()
.input("test.mp4") // Input file
.output(Output::from("output.mp4").set_framerate(AVRational { num: 30, den: 1 })) // Set to 30 FPS
.build()?
.start()?
.wait()?;
Ok(())
}
Method 2: Use a Filter to Set Frame Rate
use ez_ffmpeg::{FfmpegContext, Output};
fn main() -> Result<(), Box<dyn std::error::Error>> {
FfmpegContext::builder()
.input("test.mp4") // Input file
.filter_desc("fps=30") // Use "fps" filter to set 30 FPS
.output(Output::from("output.mp4")) // Output file
.build()?
.start()?
.wait()?;
Ok(())
}
When to Use Each Method
- Method 1: Straightforward for basic frame rate changes. Best when that’s the only adjustment needed.
- Method 2: More flexible, allowing frame rate changes alongside other filters (e.g., resizing). Ideal for combined operations.
Both methods produce a 30 FPS output.mp4
from test.mp4
.
Conclusion
For Rust developers working with video, ez-ffmpeg
provides a convenient way to handle tasks like frame rate adjustments. It builds on FFI to offer a simpler, safer interface for common operations, complementing the power of direct FFmpeg calls. While it’s not a solution for every scenario, it can be a valuable tool in your toolkit, especially when ease of use is a priority.