PostgreSQL CASE: Conditional Logic for Powerful SQL Queries
PostgreSQL’s CASE statement allows you to add conditional logic to SQL queries, helping developers return different values based on specific conditions. It’s a key feature for efficient data handling directly within SQL. Example Usage Simple CASE: SELECT product_name, CASE product_category WHEN 'Electronics' THEN 'High-Tech' WHEN 'Clothing' THEN 'Fashion' ELSE 'Other' END AS category_group FROM products; Searched CASE: SELECT order_id, order_quantity, CASE WHEN order_quantity > 100 THEN 'Large' WHEN order_quantity > 50 THEN 'Medium' ELSE 'Small' END AS order_size FROM orders; Use Cases 1.Data Categorization: The CASE statement can categorize customers based on their total purchases. SELECT customer_name, CASE WHEN total_purchases > 1000 THEN 'VIP' WHEN total_purchases > 500 THEN 'Preferred' ELSE 'Regular' END AS customer_category FROM customers; 2.Handling NULL Values: You can use CASE to replace NULL values with a default value, ensuring cleaner results. SELECT product_name, price, CASE WHEN price IS NULL THEN '0' ELSE price END AS formatted_price FROM products; 3.Custom Sorting: CASE also allows custom sorting of rows based on specific rules. For instance, sorting employees by their job title: SELECT employee_name, job_title FROM employees ORDER BY CASE job_title WHEN 'Manager' THEN 1 WHEN 'Supervisor' THEN 2 ELSE 3 END; Best Practices Order conditions logically: Start with the most specific condition to prevent false matches. Comment your code: Always explain complex logic for better readability. Test your queries: Validate with different data to ensure it works as expected. FAQ What is the difference between simple and searched CASE statements? A simple CASE compares one expression to several values, while a searched CASE evaluates multiple conditions independently. Can I use CASE with WHERE or UPDATE clauses? -Yes, CASE can be used in SELECT, WHERE, and UPDATE clauses to conditionally modify or filter data. Conclusion The CASE statement in PostgreSQL is an indispensable tool for simplifying conditional logic within SQL queries. It enables flexible, efficient data manipulation. To learn more, visit PostgreSQL CASE: A Comprehensive Guide.
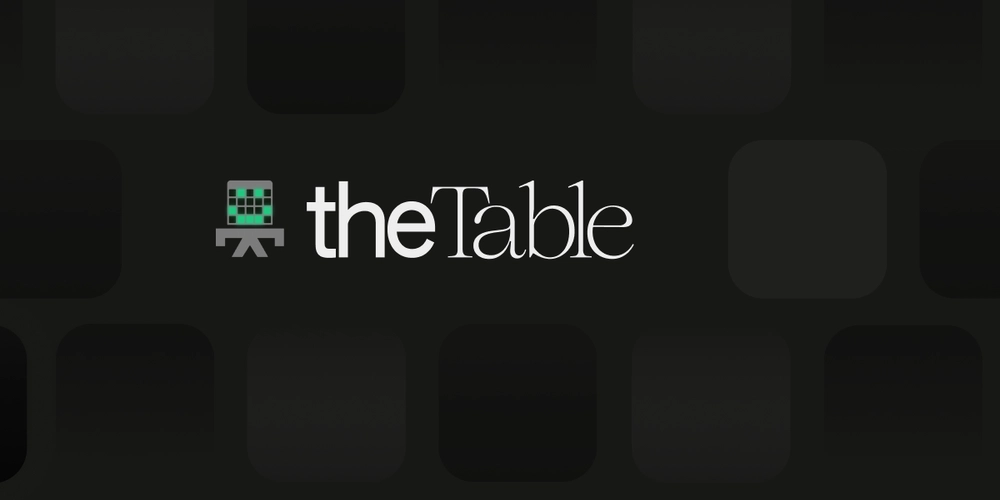
PostgreSQL’s CASE statement allows you to add conditional logic to SQL queries, helping developers return different values based on specific conditions. It’s a key feature for efficient data handling directly within SQL.
Example Usage
Simple CASE:
SELECT product_name,
CASE product_category
WHEN 'Electronics' THEN 'High-Tech'
WHEN 'Clothing' THEN 'Fashion'
ELSE 'Other'
END AS category_group
FROM products;
Searched CASE:
SELECT order_id, order_quantity,
CASE
WHEN order_quantity > 100 THEN 'Large'
WHEN order_quantity > 50 THEN 'Medium'
ELSE 'Small'
END AS order_size
FROM orders;
Use Cases
1.Data Categorization:
The CASE statement can categorize customers based on their total purchases.
SELECT customer_name,
CASE
WHEN total_purchases > 1000 THEN 'VIP'
WHEN total_purchases > 500 THEN 'Preferred'
ELSE 'Regular'
END AS customer_category
FROM customers;
2.Handling NULL Values:
You can use CASE to replace NULL values with a default value, ensuring cleaner results.
SELECT product_name, price,
CASE
WHEN price IS NULL THEN '0'
ELSE price
END AS formatted_price
FROM products;
3.Custom Sorting:
CASE also allows custom sorting of rows based on specific rules. For instance, sorting employees by their job title:
SELECT employee_name, job_title
FROM employees
ORDER BY
CASE job_title
WHEN 'Manager' THEN 1
WHEN 'Supervisor' THEN 2
ELSE 3
END;
Best Practices
- Order conditions logically: Start with the most specific condition to prevent false matches.
- Comment your code: Always explain complex logic for better readability.
- Test your queries: Validate with different data to ensure it works as expected.
FAQ
What is the difference between simple and searched CASE statements?
- A simple CASE compares one expression to several values, while a searched CASE evaluates multiple conditions independently.
Can I use CASE with WHERE or UPDATE clauses?
-Yes, CASE can be used in SELECT, WHERE, and UPDATE clauses to conditionally modify or filter data.
Conclusion
The CASE statement in PostgreSQL is an indispensable tool for simplifying conditional logic within SQL queries. It enables flexible, efficient data manipulation. To learn more, visit PostgreSQL CASE: A Comprehensive Guide.