Implementing Rate Limiting and Throttling in .NET 8: Safeguard Your Backend Services
In today's post, we’re diving into rate limiting and throttling in .NET 8, a crucial technique to protect your backend services from overload. With the ever-increasing number of client requests, it's essential to incorporate mechanisms that will control traffic and ensure your ASP.NET 8 applications remain robust and responsive. Table of Contents Introduction Understanding Rate Limiting and Throttling Built-in Rate Limiting in .NET 8 Coding Example: Implementing Rate Limiting Best Practices and Usage Scenarios Conclusion Introduction As modern applications become more complex and handle increasing volumes of traffic, managing the flow of incoming requests becomes paramount. Rate limiting and throttling are strategies designed to protect services by controlling how often clients can make requests. In .NET 8, leveraging built-in middleware and configuration options can help implement these techniques easily. In this guide, we will: Define rate limiting and throttling and explain why they are essential. Explore .NET 8’s built-in support for implementing these features. Look at real-world code examples. Discuss best practices for configuring your policies. Understanding Rate Limiting and Throttling Rate limiting restricts the number of requests a client can make within a certain time period. This helps prevent spam, DDoS attacks, and ensures that resources are shared equitably among all users. Throttling, on the other hand, manages the speed at which requests are accepted or processed. Key objectives include: Protecting the backend services from being inundated with requests. Maintaining a fair distribution of server resources. Improving application stability and user experience. Built-in Rate Limiting in .NET 8 With .NET 8, the framework now includes built-in middleware to handle rate limiting, making it easier than ever to integrate this functionality into your applications. The middleware allows you to define various policies, such as fixed window, sliding window, and token bucket algorithms. Using these policies, you can customize the behavior for different endpoints or globally, ensuring flexible and powerful traffic management. Coding Example: Implementing Rate Limiting Below is a simple example of how to implement rate limiting in an ASP.NET 8 application using the built-in middleware. using Microsoft.AspNetCore.RateLimiting; using System.Threading.RateLimiting; var builder = WebApplication.CreateBuilder(args); // Add Rate Limiter services with a Fixed Window policy. builder.Services.AddRateLimiter(options => { // Define a fixed window rate limiter policy. options.AddFixedWindowLimiter(policyName: "Fixed", options => { options.PermitLimit = 10; // Maximum requests allowed per window. options.Window = TimeSpan.FromSeconds(10); // Time window. options.QueueLimit = 2; // Max queued requests. options.QueueProcessingOrder = QueueProcessingOrder.OldestFirst; }); }); var app = builder.Build(); // Use the rate limiter middleware. app.UseRateLimiter(); // Example endpoint that will be rate limited. app.MapGet("/", () => "Hello, World!").RequireRateLimiting("Fixed"); app.Run(); Explanation The code above registers a fixed window rate limiter policy, limiting the endpoint to 10 requests every 10 seconds with a small queue buffer. The UseRateLimiter middleware is added to the application pipeline, ensuring that rate limiting rules are enforced on incoming requests. The endpoint / is configured to use the "Fixed" policy, meaning any traffic to this route will be throttled according to the defined rules. Best Practices and Usage Scenarios When implementing rate limiting and throttling in your applications, consider the following best practices: Policy Customization Define different policies for different endpoints. A public API may have stricter limits than internal endpoints. Consider using sliding or token bucket algorithms for smoother request flows where appropriate. Graceful Degradation When limits are exceeded, ensure your application returns informative error responses (e.g., HTTP 429 Too Many Requests) to help clients adjust their behavior. Optionally implement Retry-After headers. Monitoring and Logging Track usage metrics to identify abuse or unusual traffic patterns. Use logging to monitor the performance of rate-limiting policies. Testing Thoroughly test your rate-limiting strategy under simulated load conditions to ensure that legitimate users are not inadvertently penalized. Use tools like Postman or automated tests to emulate various request patterns. Hybrid Approaches Combine rate limiting with other security measures such as IP filtering, authentication, and CDN protections for a comprehensive defense strategy. Conclusion Rate limiting and throttling are
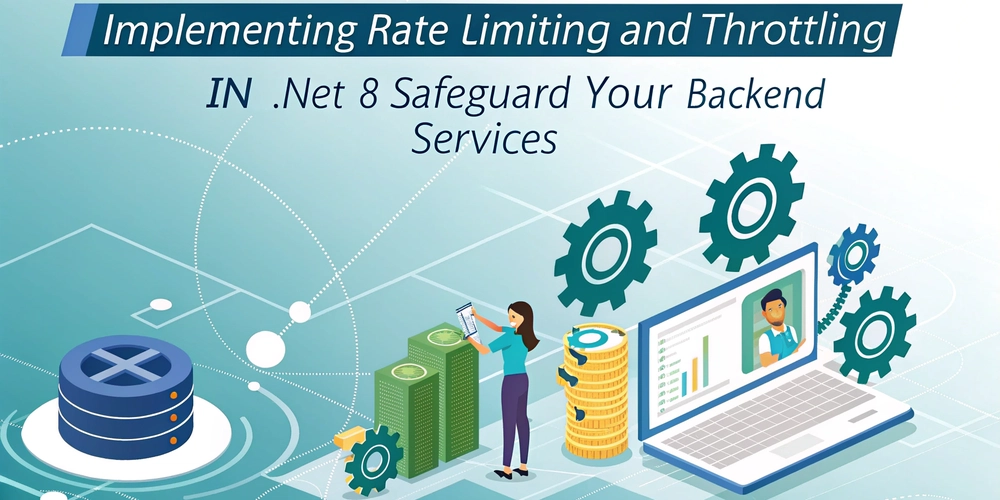
In today's post, we’re diving into rate limiting and throttling in .NET 8, a crucial technique to protect your backend services from overload. With the ever-increasing number of client requests, it's essential to incorporate mechanisms that will control traffic and ensure your ASP.NET 8 applications remain robust and responsive.
Table of Contents
- Introduction
- Understanding Rate Limiting and Throttling
- Built-in Rate Limiting in .NET 8
- Coding Example: Implementing Rate Limiting
- Best Practices and Usage Scenarios
- Conclusion
Introduction
As modern applications become more complex and handle increasing volumes of traffic, managing the flow of incoming requests becomes paramount. Rate limiting and throttling are strategies designed to protect services by controlling how often clients can make requests. In .NET 8, leveraging built-in middleware and configuration options can help implement these techniques easily.
In this guide, we will:
- Define rate limiting and throttling and explain why they are essential.
- Explore .NET 8’s built-in support for implementing these features.
- Look at real-world code examples.
- Discuss best practices for configuring your policies.
Understanding Rate Limiting and Throttling
Rate limiting restricts the number of requests a client can make within a certain time period. This helps prevent spam, DDoS attacks, and ensures that resources are shared equitably among all users. Throttling, on the other hand, manages the speed at which requests are accepted or processed.
Key objectives include:
- Protecting the backend services from being inundated with requests.
- Maintaining a fair distribution of server resources.
- Improving application stability and user experience.
Built-in Rate Limiting in .NET 8
With .NET 8, the framework now includes built-in middleware to handle rate limiting, making it easier than ever to integrate this functionality into your applications. The middleware allows you to define various policies, such as fixed window, sliding window, and token bucket algorithms.
Using these policies, you can customize the behavior for different endpoints or globally, ensuring flexible and powerful traffic management.
Coding Example: Implementing Rate Limiting
Below is a simple example of how to implement rate limiting in an ASP.NET 8 application using the built-in middleware.
using Microsoft.AspNetCore.RateLimiting;
using System.Threading.RateLimiting;
var builder = WebApplication.CreateBuilder(args);
// Add Rate Limiter services with a Fixed Window policy.
builder.Services.AddRateLimiter(options =>
{
// Define a fixed window rate limiter policy.
options.AddFixedWindowLimiter(policyName: "Fixed", options =>
{
options.PermitLimit = 10; // Maximum requests allowed per window.
options.Window = TimeSpan.FromSeconds(10); // Time window.
options.QueueLimit = 2; // Max queued requests.
options.QueueProcessingOrder = QueueProcessingOrder.OldestFirst;
});
});
var app = builder.Build();
// Use the rate limiter middleware.
app.UseRateLimiter();
// Example endpoint that will be rate limited.
app.MapGet("/", () => "Hello, World!").RequireRateLimiting("Fixed");
app.Run();
Explanation
- The code above registers a fixed window rate limiter policy, limiting the endpoint to 10 requests every 10 seconds with a small queue buffer.
- The
UseRateLimiter
middleware is added to the application pipeline, ensuring that rate limiting rules are enforced on incoming requests. - The endpoint
/
is configured to use the "Fixed" policy, meaning any traffic to this route will be throttled according to the defined rules.
Best Practices and Usage Scenarios
When implementing rate limiting and throttling in your applications, consider the following best practices:
Policy Customization
- Define different policies for different endpoints. A public API may have stricter limits than internal endpoints.
- Consider using sliding or token bucket algorithms for smoother request flows where appropriate.
Graceful Degradation
- When limits are exceeded, ensure your application returns informative error responses (e.g., HTTP 429 Too Many Requests) to help clients adjust their behavior.
- Optionally implement
Retry-After
headers.
Monitoring and Logging
- Track usage metrics to identify abuse or unusual traffic patterns.
- Use logging to monitor the performance of rate-limiting policies.
Testing
- Thoroughly test your rate-limiting strategy under simulated load conditions to ensure that legitimate users are not inadvertently penalized.
- Use tools like Postman or automated tests to emulate various request patterns.
Hybrid Approaches
- Combine rate limiting with other security measures such as IP filtering, authentication, and CDN protections for a comprehensive defense strategy.
Conclusion
Rate limiting and throttling are essential features for maintaining the health and performance of your backend services. With .NET 8’s robust, built-in middleware, implementing these strategies has never been easier. By following the techniques and best practices discussed in this article, you can ensure that your ASP.NET applications remain resilient under heavy traffic while protecting your critical resources.
I hope this guide empowers you to take practical steps in safeguarding your services. If you have any questions, suggestions, or would like to share your experiences, feel free to leave a comment below!
Happy coding!