Display PDF files as PNG images in .NET – Efficient and Cloud Hosted
Showcase PDF pages as images within your .NET application using the GroupDocs.Viewer Cloud .NET SDK, enhancing your document rendering processes significantly. The Cloud SDK simplifies the task of displaying PDF files to PNG images—there's no need for Adobe Acrobat or cumbersome desktop libraries. With just a handful of API calls, you can render complete PDF documents into sharp, high-resolution PNG files. This functionality is vital for creating previews, thumbnails, or displaying pages for viewing in lightweight .NET applications. Rendering PDFs into PNGs in .NET enables your users to interact with static content swiftly and dependably. You can develop a document viewer, prepare pages for OCR, or provide visual outputs for PDFs; this C# REST API performs the rendering in the cloud—which reduces server-side dependencies and enhances scalability. Additionally, with support for diverse rendering options, you can manage image quality, page ranges, and more. This .NET Cloud SDK enables developers to create rich document experiences without the added complexity. Eliminate the need for heavy toolkits or licensing issues. Simply connect to the GroupDocs.Viewer REST API, process your files, and obtain clean, ready-to-use PNGs. If you're aiming to programmatically view PDFs as PNGs in C#, explore this detailed tutorial today. using GroupDocs.Viewer.Cloud.Sdk.Api; using GroupDocs.Viewer.Cloud.Sdk.Client; using GroupDocs.Viewer.Cloud.Sdk.Model; using GroupDocs.Viewer.Cloud.Sdk.Model.Requests; class Program { static void Main(string[] args) { // Step 1: Set up API credentials and initialize configuration string MyClientId = "your-client-id"; string MyClientSecret = "your-client-secret"; var configuration = new Configuration(MyClientId, MyClientSecret); // Step 2: Initialize ViewApi for rendering var viewApi = new ViewApi(configuration); // Step 3: Set up view options to render the PDF to PNG var viewOptions = new ViewOptions { FileInfo = new GroupDocs.Viewer.Cloud.Sdk.Model.FileInfo { FilePath = "SampleFiles/source.pdf" }, // Set output format to PNG ViewFormat = ViewOptions.ViewFormatEnum.PNG, RenderOptions = new ImageOptions { Width = 1200, // Set custom width Height = 800 // Set custom height } }; // Step 4: Create and execute the view request to // render the PDF as PNG var viewRequest = new CreateViewRequest(viewOptions); var viewResponse = viewApi.CreateView(viewRequest); } }
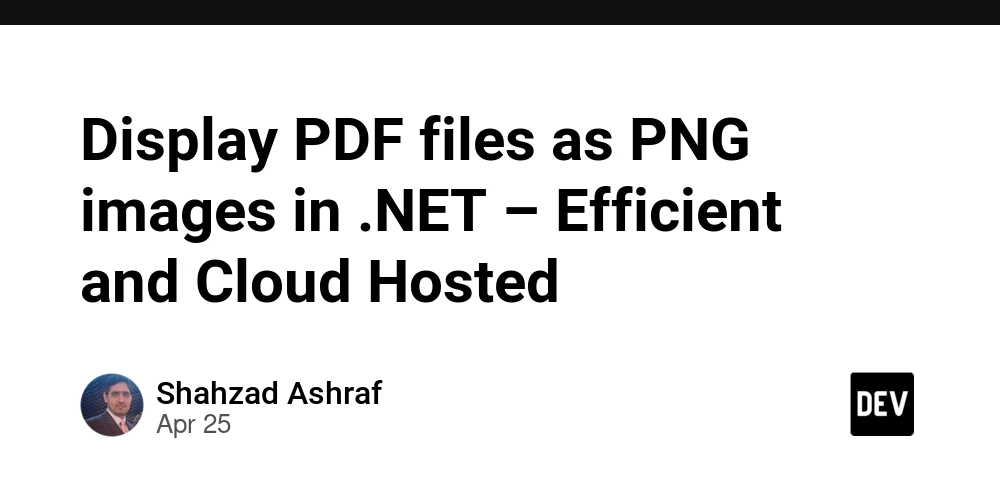
Showcase PDF pages as images within your .NET application using the GroupDocs.Viewer Cloud .NET SDK, enhancing your document rendering processes significantly. The Cloud SDK simplifies the task of displaying PDF files to PNG images—there's no need for Adobe Acrobat or cumbersome desktop libraries. With just a handful of API calls, you can render complete PDF documents into sharp, high-resolution PNG files. This functionality is vital for creating previews, thumbnails, or displaying pages for viewing in lightweight .NET applications.
Rendering PDFs into PNGs in .NET enables your users to interact with static content swiftly and dependably. You can develop a document viewer, prepare pages for OCR, or provide visual outputs for PDFs; this C# REST API performs the rendering in the cloud—which reduces server-side dependencies and enhances scalability. Additionally, with support for diverse rendering options, you can manage image quality, page ranges, and more.
This .NET Cloud SDK enables developers to create rich document experiences without the added complexity. Eliminate the need for heavy toolkits or licensing issues. Simply connect to the GroupDocs.Viewer REST API, process your files, and obtain clean, ready-to-use PNGs. If you're aiming to programmatically view PDFs as PNGs in C#, explore this detailed tutorial today.
using GroupDocs.Viewer.Cloud.Sdk.Api;
using GroupDocs.Viewer.Cloud.Sdk.Client;
using GroupDocs.Viewer.Cloud.Sdk.Model;
using GroupDocs.Viewer.Cloud.Sdk.Model.Requests;
class Program
{
static void Main(string[] args)
{
// Step 1: Set up API credentials and initialize configuration
string MyClientId = "your-client-id";
string MyClientSecret = "your-client-secret";
var configuration = new Configuration(MyClientId, MyClientSecret);
// Step 2: Initialize ViewApi for rendering
var viewApi = new ViewApi(configuration);
// Step 3: Set up view options to render the PDF to PNG
var viewOptions = new ViewOptions
{
FileInfo = new GroupDocs.Viewer.Cloud.Sdk.Model.FileInfo
{
FilePath = "SampleFiles/source.pdf"
},
// Set output format to PNG
ViewFormat = ViewOptions.ViewFormatEnum.PNG,
RenderOptions = new ImageOptions
{
Width = 1200, // Set custom width
Height = 800 // Set custom height
}
};
// Step 4: Create and execute the view request to
// render the PDF as PNG
var viewRequest = new CreateViewRequest(viewOptions);
var viewResponse = viewApi.CreateView(viewRequest);
}
}