DAY : 19 How to use Encapsulation in java
Encapsulation Encapsulation is a Data protection In Java, encapsulation is a core object-oriented programming (OOP) principle that bundles data (variables) and the methods (functions) that operate on that data into a single unit, a class, and restricts direct access to some of an object's components, promoting data hiding and controlled access Access Modifier Encapsulation is achieved through Access modifier 1.Private - within the same class 2.Default - Within the same package 3.Protection - Within the same package, inherited, class 4.Public - Public to the entire project **All methods all called as method Signature* Questions: 1.One can access non private fields and methods of a class (consider class A) from another class (Class B) - True 2.Can we have a Private main method - False 3.All Access Modifier applicable for local variables? - False Class Example: public class Google { private int emp_salary; public static String ho_address = "Mountain View"; public static boolean working = true; private void get_user_details() { int no = 10; System.out.println("All Users Details"); } public void search_Results() { System.out.println("Searching Results"); } } public class User { public static void main(String[] args) { //Method Signature Google googleObj = new Google() ; //System.out.println(googleObj.emp_salary); System.out.println(Google.working); System.out.println(Google.ho_address); //googleObj.get_user_details(); googleObj.search_Results(); User.main(); } public static void main(){ System.out.println("Overloaded Main Method"); } }
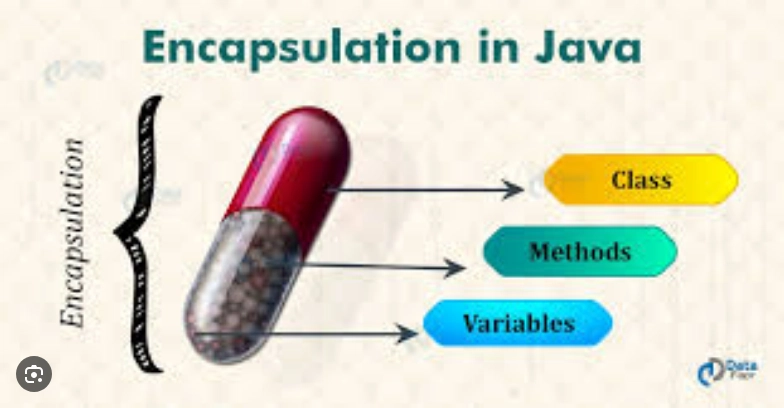
Encapsulation
Encapsulation is a Data protection
In Java, encapsulation is a core object-oriented programming (OOP) principle that bundles data (variables) and the methods (functions) that operate on that data into a single unit, a class, and restricts direct access to some of an object's components, promoting data hiding and controlled access
Access Modifier
Encapsulation is achieved through Access modifier
1.Private - within the same class
2.Default - Within the same package
3.Protection - Within the same package, inherited, class
4.Public - Public to the entire project
**All methods all called as method Signature*
Questions:
1.One can access non private fields and methods of a class (consider class A) from another class (Class B) - True
2.Can we have a Private main method - False
3.All Access Modifier applicable for local variables? - False
Class Example:
public class Google {
private int emp_salary;
public static String ho_address = "Mountain View";
public static boolean working = true;
private void get_user_details() {
int no = 10;
System.out.println("All Users Details");
}
public void search_Results() {
System.out.println("Searching Results");
}
}
public class User {
public static void main(String[] args) { //Method Signature
Google googleObj = new Google() ;
//System.out.println(googleObj.emp_salary);
System.out.println(Google.working);
System.out.println(Google.ho_address);
//googleObj.get_user_details();
googleObj.search_Results();
User.main();
}
public static void main(){
System.out.println("Overloaded Main Method");
}
}