Day 12 Java Learning :
What Is Constructor Overloading? In Java, constructor overloading means defining more than one constructor in a class, each with a different parameter list (different number, order, or types of parameters). At compile time, the Java compiler determines which constructor to call based on the arguments provided. public class Student { private String name; private int age; // No-arg constructor public Student() { this.name = "Unknown"; this.age = 0; } // Single-arg constructor public Student(String name) { this.name = name; this.age = 0; } // Two-arg constructor public Student(String name, int age) { this.name = name; this.age = age; } } Here, Student has three constructors—each “overloaded” with a different signature. Types of Constructor Overloading Different Number of Parameters public MyClass() { … } public MyClass(int a) { … } public MyClass(int a, String b) { … } Different Parameter Types public MyClass(int a, double b) { … } public MyClass(double a, int b) { … } Different Parameter Order public MyClass(String s, int i) { … } public MyClass(int i, String s) { … } Why Use Constructor Overloading? Flexibility in Object Creation Allows clients of your class to supply different levels of detail when creating objects (e.g., minimal info vs. full configuration). Code Clarity and Convenience Instead of setting default values manually after construction, overloaded constructors let you encapsulate defaulting logic. Immutable Classes For immutable objects (where fields are final), constructor overloading is the only way to offer multiple “ways in” without setters. When and Where to Use It Use constructor overloading when: You Have Sensible Defaults When many fields have common default values, provide a no‑arg or partial‑arg constructor that applies those defaults internally. You Want to Offer Multiple Initialization Paths E.g., a Rectangle class might let callers specify width & height, or supply only one side to create a square. Enhancing Readability Different constructors named by their parameter lists make the intent clear at the call site: new Student(); // blank student new Student("Alice"); // name only new Student("Bob", 22); // name + age When Not to Use Constructor Overloading Avoid excessive overloading when: Too Many Combinations If the number of parameters grows and the combinations become unmanageable, switch to the Builder pattern instead. Ambiguity Risks Constructors whose signatures differ only by parameter types that can be converted (e.g., int vs. long) may lead to unexpected overload resolution. Complex Initialization Logic If your initialization involves branching logic or error‑handling that’s too intricate for a constructor, consider static factory methods (public static MyClass of(…)) that can throw checked exceptions or return subclasses. Summary What? Multiple constructors in one class, each with different signatures. Types? Varying parameter count, types, or order. Why? Flexibility, clarity, and support for immutable objects. When to Use? When you need different “entry points” with sensible defaults or clearer calls. When to Avoid? When overloads grow unwieldy, risk ambiguity, or when a Builder/static factory is more appropriate.
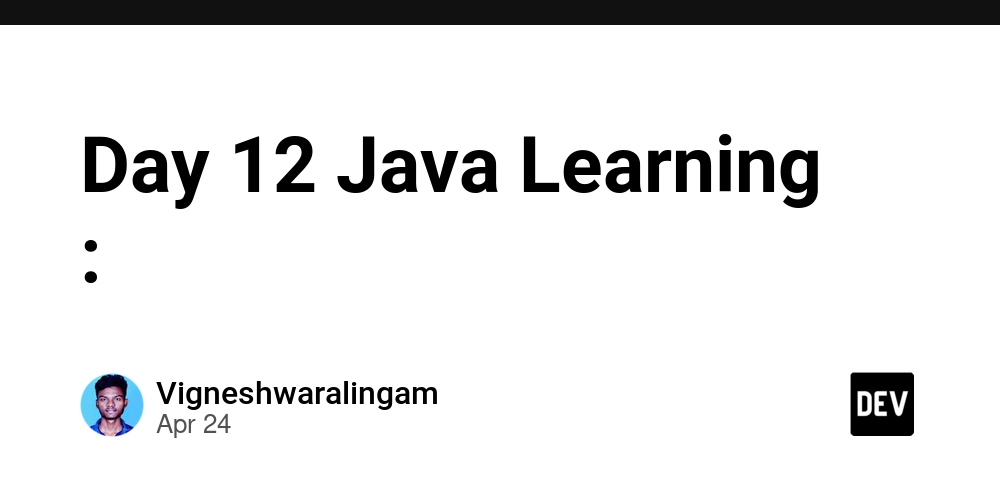
What Is Constructor Overloading?
In Java, constructor overloading means defining more than one constructor in a class, each with a different parameter list (different number, order, or types of parameters). At compile time, the Java compiler determines which constructor to call based on the arguments provided.
public class Student {
private String name;
private int age;
// No-arg constructor
public Student() {
this.name = "Unknown";
this.age = 0;
}
// Single-arg constructor
public Student(String name) {
this.name = name;
this.age = 0;
}
// Two-arg constructor
public Student(String name, int age) {
this.name = name;
this.age = age;
}
}
Here, Student
has three constructors—each “overloaded” with a different signature.
Types of Constructor Overloading
- Different Number of Parameters
public MyClass() { … }
public MyClass(int a) { … }
public MyClass(int a, String b) { … }
- Different Parameter Types
public MyClass(int a, double b) { … }
public MyClass(double a, int b) { … }
- Different Parameter Order
public MyClass(String s, int i) { … }
public MyClass(int i, String s) { … }
Why Use Constructor Overloading?
- Flexibility in Object Creation Allows clients of your class to supply different levels of detail when creating objects (e.g., minimal info vs. full configuration).
- Code Clarity and Convenience Instead of setting default values manually after construction, overloaded constructors let you encapsulate defaulting logic.
-
Immutable Classes
For immutable objects (where fields are
final
), constructor overloading is the only way to offer multiple “ways in” without setters.
When and Where to Use It
Use constructor overloading when:
- You Have Sensible Defaults When many fields have common default values, provide a no‑arg or partial‑arg constructor that applies those defaults internally.
-
You Want to Offer Multiple Initialization Paths
E.g., a
Rectangle
class might let callers specify width & height, or supply only one side to create a square. - Enhancing Readability Different constructors named by their parameter lists make the intent clear at the call site:
new Student(); // blank student
new Student("Alice"); // name only
new Student("Bob", 22); // name + age
When Not to Use Constructor Overloading
Avoid excessive overloading when:
- Too Many Combinations If the number of parameters grows and the combinations become unmanageable, switch to the Builder pattern instead.
-
Ambiguity Risks
Constructors whose signatures differ only by parameter types that can be converted (e.g.,
int
vs.long
) may lead to unexpected overload resolution. -
Complex Initialization Logic
If your initialization involves branching logic or error‑handling that’s too intricate for a constructor, consider static factory methods (
public static MyClass of(…)
) that can throw checked exceptions or return subclasses.
Summary
- What? Multiple constructors in one class, each with different signatures.
- Types? Varying parameter count, types, or order.
- Why? Flexibility, clarity, and support for immutable objects.
- When to Use? When you need different “entry points” with sensible defaults or clearer calls.
- When to Avoid? When overloads grow unwieldy, risk ambiguity, or when a Builder/static factory is more appropriate.