Clean Architecture in .NET: When to Use It and How to Stay Flexible
As a C# developer, you’ve probably heard about Clean Architecture. It’s praised as a best practice for building maintainable, scalable applications. But following it strictly can feel overwhelming, especially when deadlines are tight or the project is small. Let’s explore its core ideas, when to use it, and how to adapt it flexibly. What Are the Key Concepts? Clean Architecture emphasizes separation of concerns by organizing code into layers: Core: Contains business logic (entities, interfaces). Application: Implements use cases (services, DTOs). Infrastructure: Handles external concerns (databases, APIs). Presentation: Manages user interaction (APIs, UI). The dependency rule ensures outer layers depend on inner layers, not the other way around. This is achieved through dependency inversion (e.g., using interfaces in Core and implementations in Infrastructure). Other principles include: Testability: Isolating business logic makes unit testing easier. Decoupling: Changes in one layer (e.g., switching databases) don’t break others. When Does It Feel Overwhelming? Clean Architecture shines in large, complex systems (e.g., enterprise apps) where scalability and long-term maintenance matter. However, it might not be ideal for: Small Projects: A simple CRUD app or prototype doesn’t need four layers. Adding abstractions here slows development. Tight Deadlines: Strict layering can delay delivery if the team isn’t familiar with the pattern. Limited Resources: Maintaining layers requires effort. For small teams, this can become a burden. Flexibility Is Key Clean Architecture is a guideline, not a rule. Adapt it based on your project’s needs: Simplify Layers: Merge Core and Application layers for smaller apps. Use Shared Projects: In .NET, combine Infrastructure and Presentation if they’re tightly related (e.g., a Blazor WASM app). Avoid Over-Engineering: Only introduce abstractions (like repositories or MediatR) when necessary. For simple apps, Entity Framework Core’s DbContext might suffice. Start Simple, Evolve Later: Begin with minimal structure and add layers as the project grows. When to Make Exceptions Prototypes: Focus on speed, not structure. Microservices: Some services might not need full Clean Architecture if they’re small and single-purpose. Legacy Code Integration: When modernizing old systems, apply Clean Architecture incrementally. Final Thoughts Clean Architecture is a powerful tool, but it’s not a one-size-fits-all solution. For C# developers, the goal is to balance best practices with practicality. Ask: Will this abstraction add value, or just complexity? By staying flexible and prioritizing your project’s unique needs, you’ll avoid overwhelm while keeping your codebase clean and adaptable. Remember: Good architecture solves problems, not creates them.
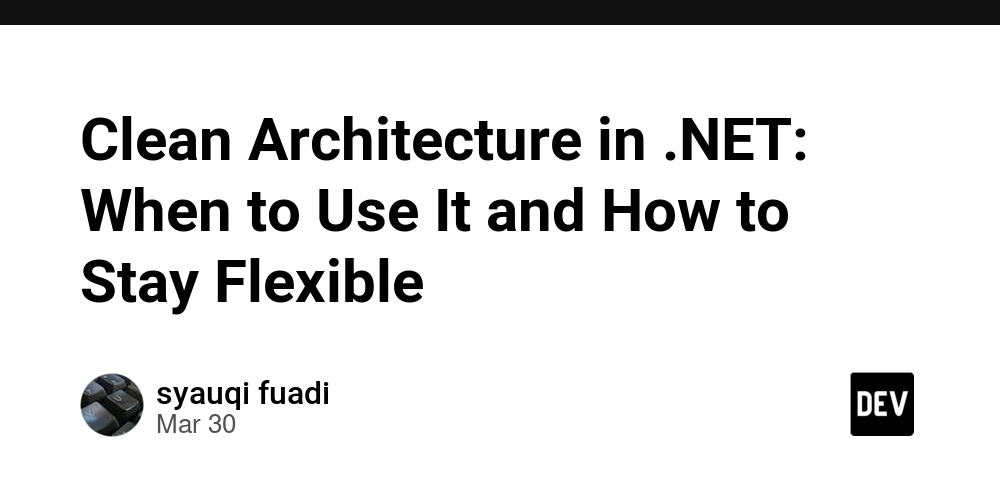
As a C# developer, you’ve probably heard about Clean Architecture. It’s praised as a best practice for building maintainable, scalable applications. But following it strictly can feel overwhelming, especially when deadlines are tight or the project is small. Let’s explore its core ideas, when to use it, and how to adapt it flexibly.
What Are the Key Concepts?
Clean Architecture emphasizes separation of concerns by organizing code into layers:
- Core: Contains business logic (entities, interfaces).
- Application: Implements use cases (services, DTOs).
- Infrastructure: Handles external concerns (databases, APIs).
- Presentation: Manages user interaction (APIs, UI).
The dependency rule ensures outer layers depend on inner layers, not the other way around. This is achieved through dependency inversion (e.g., using interfaces in Core and implementations in Infrastructure).
Other principles include:
- Testability: Isolating business logic makes unit testing easier.
- Decoupling: Changes in one layer (e.g., switching databases) don’t break others.
When Does It Feel Overwhelming?
Clean Architecture shines in large, complex systems (e.g., enterprise apps) where scalability and long-term maintenance matter. However, it might not be ideal for:
- Small Projects: A simple CRUD app or prototype doesn’t need four layers. Adding abstractions here slows development.
- Tight Deadlines: Strict layering can delay delivery if the team isn’t familiar with the pattern.
- Limited Resources: Maintaining layers requires effort. For small teams, this can become a burden.
Flexibility Is Key
Clean Architecture is a guideline, not a rule. Adapt it based on your project’s needs:
- Simplify Layers: Merge Core and Application layers for smaller apps.
- Use Shared Projects: In .NET, combine Infrastructure and Presentation if they’re tightly related (e.g., a Blazor WASM app).
-
Avoid Over-Engineering: Only introduce abstractions (like repositories or MediatR) when necessary. For simple apps, Entity Framework Core’s
DbContext
might suffice. - Start Simple, Evolve Later: Begin with minimal structure and add layers as the project grows.
When to Make Exceptions
- Prototypes: Focus on speed, not structure.
- Microservices: Some services might not need full Clean Architecture if they’re small and single-purpose.
- Legacy Code Integration: When modernizing old systems, apply Clean Architecture incrementally.
Final Thoughts
Clean Architecture is a powerful tool, but it’s not a one-size-fits-all solution. For C# developers, the goal is to balance best practices with practicality. Ask: Will this abstraction add value, or just complexity? By staying flexible and prioritizing your project’s unique needs, you’ll avoid overwhelm while keeping your codebase clean and adaptable.
Remember: Good architecture solves problems, not creates them.