Building an NSFW AI Image Generator with Next.js and Tailwind CSS
Building an NSFW AI Image Generator with Next.js and Tailwind CSS Creating an AI image generator that can handle NSFW (Not Safe For Work) content is an exciting challenge that combines cutting-edge technologies. In this tutorial, we'll walk through building an NSFW AI image generator using Next.js, TypeScript, and Tailwind CSS. We'll cover setting up the project, integrating the UI library, and handling AI-generated images securely. Introduction AI image generators have gained popularity for their ability to create images from textual descriptions. By leveraging frameworks like Next.js and libraries like Tailwind CSS, we can build a responsive and performant web application. In this tutorial, we'll focus on: Setting up a Next.js project with TypeScript Integrating Tailwind CSS for styling Building the user interface for input and image display Implementing the logic to handle AI image generation, including NSFW content Deploying the application Let's get started! Prerequisites Before we begin, make sure you have the following installed: Node.js (>= 14.x) npm or yarn package manager Basic knowledge of React and TypeScript is also recommended. 1. Setting Up the Project with Next.js and TypeScript First, we'll initialize a new Next.js project with TypeScript support. Initialize the Project Run the following command to create a new Next.js app: bash npx create-next-app@latest nsfw-ai-image-generator --typescript This command sets up a new Next.js project named nsfw-ai-image-generator with TypeScript configuration. Project Structure The project structure should look like this: nsfw-ai-image-generator/ ├── pages/ │ ├── _app.tsx │ ├── index.tsx ├── public/ ├── styles/ │ └── globals.css ├── tsconfig.json ├── package.json 2. Integrating Tailwind CSS Next, we'll add Tailwind CSS to style our application. Install Tailwind CSS Run the following command to install Tailwind CSS and its dependencies: bash npm install -D tailwindcss postcss autoprefixer Initialize Tailwind Configuration Generate the Tailwind and PostCSS configuration files: bash npx tailwindcss init -p Configure Tailwind Modify tailwind.config.js to specify the paths to all of your template files: module.exports = { content: [ './pages//*.{js,ts,jsx,tsx}', './components//*.{js,ts,jsx,tsx}', ], theme: { extend: {}, }, plugins: [], } Include Tailwind in CSS Add the Tailwind directives to styles/globals.css: css @tailwind base; @tailwind components; @tailwind utilities; 3. Building the NSFW AI Image Generator UI Now, let's build the user interface for our application. Creating the Input Form Open pages/index.tsx and replace its content with the following: tsx import { useState } from 'react'; const Home = () => { const [prompt, setPrompt] = useState(''); const [imageSrc, setImageSrc] = useState(''); const handleGenerateImage = async () => { // Logic to generate image will go here }; return (
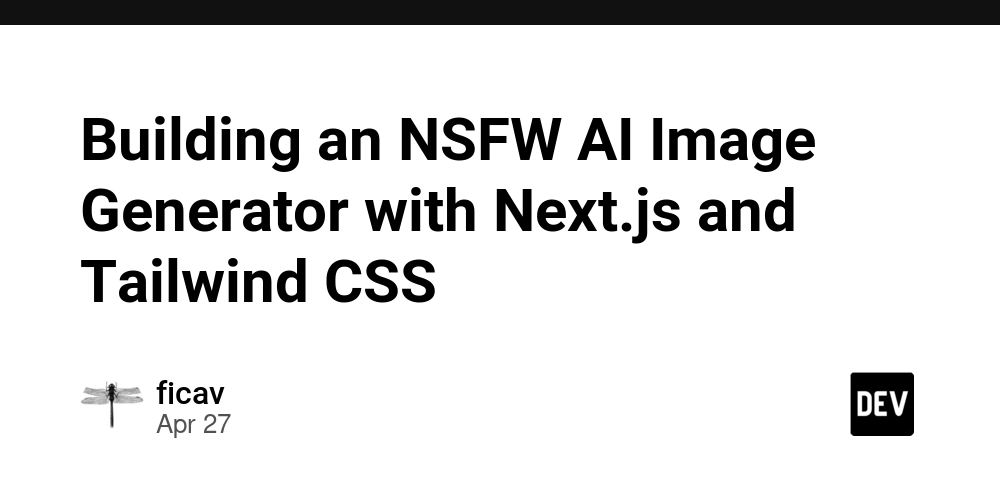
Building an NSFW AI Image Generator with Next.js and Tailwind CSS
Creating an AI image generator that can handle NSFW (Not Safe For Work) content is an exciting challenge that combines cutting-edge technologies. In this tutorial, we'll walk through building an NSFW AI image generator using Next.js, TypeScript, and Tailwind CSS. We'll cover setting up the project, integrating the UI library, and handling AI-generated images securely.
Introduction
AI image generators have gained popularity for their ability to create images from textual descriptions. By leveraging frameworks like Next.js and libraries like Tailwind CSS, we can build a responsive and performant web application. In this tutorial, we'll focus on:
- Setting up a Next.js project with TypeScript
- Integrating Tailwind CSS for styling
- Building the user interface for input and image display
- Implementing the logic to handle AI image generation, including NSFW content
- Deploying the application
Let's get started!
Prerequisites
Before we begin, make sure you have the following installed:
- Node.js (>= 14.x)
- npm or yarn package manager
Basic knowledge of React and TypeScript is also recommended.
1. Setting Up the Project with Next.js and TypeScript
First, we'll initialize a new Next.js project with TypeScript support.
Initialize the Project
Run the following command to create a new Next.js app:
bash
npx create-next-app@latest nsfw-ai-image-generator --typescript
This command sets up a new Next.js project named nsfw-ai-image-generator
with TypeScript configuration.
Project Structure
The project structure should look like this:
nsfw-ai-image-generator/
├── pages/
│ ├── _app.tsx
│ ├── index.tsx
├── public/
├── styles/
│ └── globals.css
├── tsconfig.json
├── package.json
2. Integrating Tailwind CSS
Next, we'll add Tailwind CSS to style our application.
Install Tailwind CSS
Run the following command to install Tailwind CSS and its dependencies:
bash
npm install -D tailwindcss postcss autoprefixer
Initialize Tailwind Configuration
Generate the Tailwind and PostCSS configuration files:
bash
npx tailwindcss init -p
Configure Tailwind
Modify tailwind.config.js
to specify the paths to all of your template files:
module.exports = {
content: [
'./pages//*.{js,ts,jsx,tsx}',
'./components//*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Include Tailwind in CSS
Add the Tailwind directives to styles/globals.css
:
css
@tailwind base;
@tailwind components;
@tailwind utilities;
3. Building the NSFW AI Image Generator UI
Now, let's build the user interface for our application.
Creating the Input Form
Open pages/index.tsx
and replace its content with the following:
tsx
import { useState } from 'react';
const Home = () => {
const [prompt, setPrompt] = useState('');
const [imageSrc, setImageSrc] = useState('');
const handleGenerateImage = async () => {
// Logic to generate image will go here
};
return (