WeakMap and WeakSet: Memory-Efficient Collections
In the ever-evolving landscape of JavaScript, developers are often in pursuit of more sophisticated and memory-efficient ways to manage collections of objects. Enter WeakMap and WeakSet: two types of collections that serve as memory-efficient replacements for their standard counterparts, Map and Set. Not only do they provide all the functionality of these traditional collections, but they also have the added benefit of not preventing garbage collection of their elements. In this article, we’ll dive deeply into what WeakMap and WeakSet are, examine their intricacies, and explore how and when to use them, accompanied by practical code examples. Understanding WeakMap and WeakSet 1. What is WeakMap? A WeakMap is a collection of key-value pairs where the keys are objects and the values can be any data type. The important characteristic of WeakMap is that its keys are held weakly. This means that if there are no other references to a key object, it can be garbage collected, thereby freeing up memory. Creating a WeakMap: const weakMap = new WeakMap(); const objKey = {}; const objValue = 'I am a weak value.'; weakMap.set(objKey, objValue); console.log(weakMap.get(objKey)); // Output: "I am a weak value." 2. What is WeakSet? Similar to WeakMap, a WeakSet is a collection of objects. The primary distinction is that WeakSet only stores objects (as its values) and does not associate them with any values. Like WeakMap, WeakSet also holds its entries weakly. Creating a WeakSet: const weakSet = new WeakSet(); const obj1 = {}; const obj2 = {}; weakSet.add(obj1); console.log(weakSet.has(obj1)); // Output: true console.log(weakSet.has(obj2)); // Output: false Memory Management: The Power of Weakness Both WeakMap and WeakSet offer a significant advantage in terms of memory management due to the weak references they maintain. This allows you to avoid memory leaks—especially helpful when managing objects that are not needed after a certain lifecycle. Practical Use Cases Managing Object References When you have a scenario where you want to associate metadata or additional information with objects, but don't want to stop those objects from being garbage collected, WeakMap shines. const metadata = new WeakMap(); function trackObject(obj, data) { metadata.set(obj, data); } const someObject = {}; trackObject(someObject, { info: 'This object is tracked.' }); console.log(metadata.get(someObject)); // Output: { info: 'This object is tracked.' } Event Listener Management Imagine you are attaching event listeners to an object but don't want to maintain a reference to that object once it goes out of scope. Using WeakMap, you can safely store the event handlers. const eventHandlers = new WeakMap(); function addEventListenerToObject(obj, event, handler) { eventHandlers.set(obj, handler); obj.addEventListener(event, handler); } const button = document.createElement('button'); addEventListenerToObject(button, 'click', () => { console.log('Button clicked!'); }); // Once `button` goes out of scope or is removed, no memory leaks occur. Detailed Implementation Strategies When implementing WeakMap or WeakSet, consider the lifecycle of the objects you are dealing with. Here are some strategies for effective use: Use WeakMap for Object Metadata: Store information about the object that can aid in operations like logging or tracking without holding onto the object itself. Use WeakSet for State Management: Maintain a list of active objects, such as those currently engaged in an operation or those that have registered for an event, making sure not to prevent cleanup. Monitor Performance: Since WeakMap and WeakSet are not enumerable, keep track of reference counts in a separate structure if necessary to understand the use cases better. const myWeakMap = new WeakMap(); const obj = {}; myWeakMap.set(obj, 'data related to obj'); // Expect that `obj` can be garbage collected after this point Key Takeaways Garbage Collection: The primary feature that sets WeakMap and WeakSet apart is their ability to let the garbage collector clean up unreferenced objects, ensuring efficient memory usage. Weak References: Both collections use weak references for storing keys in WeakMap and values in WeakSet. Not Iterable: Unlike traditional Map and Set, WeakMap and WeakSet are not iterable, which means you cannot loop through their contents. Use Cases: They are particularly useful for tracking private data associated with objects or implementing patterns like event delegation without creating memory leaks. Conclusion WeakMap and WeakSet are powerful tools within JavaScript's rich collection of data structures. Their capabilities allow developers to write memory-efficient code by utilizing weak references effectively. As you incorporate these structures into your applications, always co
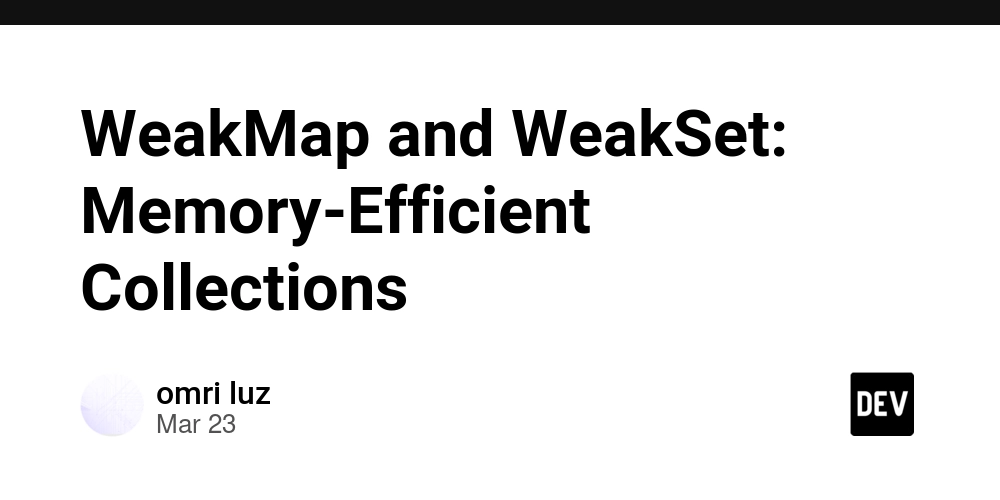
In the ever-evolving landscape of JavaScript, developers are often in pursuit of more sophisticated and memory-efficient ways to manage collections of objects. Enter WeakMap
and WeakSet
: two types of collections that serve as memory-efficient replacements for their standard counterparts, Map
and Set
. Not only do they provide all the functionality of these traditional collections, but they also have the added benefit of not preventing garbage collection of their elements. In this article, we’ll dive deeply into what WeakMap
and WeakSet
are, examine their intricacies, and explore how and when to use them, accompanied by practical code examples.
Understanding WeakMap and WeakSet
1. What is WeakMap?
A WeakMap
is a collection of key-value pairs where the keys are objects and the values can be any data type. The important characteristic of WeakMap
is that its keys are held weakly. This means that if there are no other references to a key object, it can be garbage collected, thereby freeing up memory.
Creating a WeakMap:
const weakMap = new WeakMap();
const objKey = {};
const objValue = 'I am a weak value.';
weakMap.set(objKey, objValue);
console.log(weakMap.get(objKey)); // Output: "I am a weak value."
2. What is WeakSet?
Similar to WeakMap
, a WeakSet
is a collection of objects. The primary distinction is that WeakSet
only stores objects (as its values) and does not associate them with any values. Like WeakMap
, WeakSet
also holds its entries weakly.
Creating a WeakSet:
const weakSet = new WeakSet();
const obj1 = {};
const obj2 = {};
weakSet.add(obj1);
console.log(weakSet.has(obj1)); // Output: true
console.log(weakSet.has(obj2)); // Output: false
Memory Management: The Power of Weakness
Both WeakMap
and WeakSet
offer a significant advantage in terms of memory management due to the weak references they maintain. This allows you to avoid memory leaks—especially helpful when managing objects that are not needed after a certain lifecycle.
Practical Use Cases
Managing Object References
When you have a scenario where you want to associate metadata or additional information with objects, but don't want to stop those objects from being garbage collected, WeakMap
shines.
const metadata = new WeakMap();
function trackObject(obj, data) {
metadata.set(obj, data);
}
const someObject = {};
trackObject(someObject, { info: 'This object is tracked.' });
console.log(metadata.get(someObject)); // Output: { info: 'This object is tracked.' }
Event Listener Management
Imagine you are attaching event listeners to an object but don't want to maintain a reference to that object once it goes out of scope. Using WeakMap
, you can safely store the event handlers.
const eventHandlers = new WeakMap();
function addEventListenerToObject(obj, event, handler) {
eventHandlers.set(obj, handler);
obj.addEventListener(event, handler);
}
const button = document.createElement('button');
addEventListenerToObject(button, 'click', () => {
console.log('Button clicked!');
});
// Once `button` goes out of scope or is removed, no memory leaks occur.
Detailed Implementation Strategies
When implementing WeakMap
or WeakSet
, consider the lifecycle of the objects you are dealing with. Here are some strategies for effective use:
Use WeakMap for Object Metadata: Store information about the object that can aid in operations like logging or tracking without holding onto the object itself.
Use WeakSet for State Management: Maintain a list of active objects, such as those currently engaged in an operation or those that have registered for an event, making sure not to prevent cleanup.
Monitor Performance: Since
WeakMap
andWeakSet
are not enumerable, keep track of reference counts in a separate structure if necessary to understand the use cases better.
const myWeakMap = new WeakMap();
const obj = {};
myWeakMap.set(obj, 'data related to obj');
// Expect that `obj` can be garbage collected after this point
Key Takeaways
-
Garbage Collection: The primary feature that sets
WeakMap
andWeakSet
apart is their ability to let the garbage collector clean up unreferenced objects, ensuring efficient memory usage. -
Weak References: Both collections use weak references for storing keys in
WeakMap
and values inWeakSet
. -
Not Iterable: Unlike traditional
Map
andSet
,WeakMap
andWeakSet
are not iterable, which means you cannot loop through their contents. - Use Cases: They are particularly useful for tracking private data associated with objects or implementing patterns like event delegation without creating memory leaks.
Conclusion
WeakMap
and WeakSet
are powerful tools within JavaScript's rich collection of data structures. Their capabilities allow developers to write memory-efficient code by utilizing weak references effectively. As you incorporate these structures into your applications, always consider the lifecycle of your objects to leverage their full potential without falling into the pit of memory leaks.
Further Reading and Advanced Learning Resources
- JavaScript Info - WeakMap and WeakSet
- ECMAScript Specification - WeakMap
- MDN Web Docs - WeakMap
- MDN Web Docs - WeakSet
By exploring the suggested resources, you'll deepen your understanding of memory management, best practices, and the nuances of these powerful collections in JavaScript. Happy coding!