Using RabbitMQ with Node.js: A Complete Guide
Introduction RabbitMQ is a powerful message broker that enables asynchronous communication between different parts of an application. It supports multiple messaging patterns and is widely used for microservices and distributed systems. In this guide, we will explore how to integrate RabbitMQ with Node.js, covering installation, setup, and a working example. Learn NodeJs by Building Projects Prerequisites Node.js installed (v14+ recommended) RabbitMQ installed on your system Installing RabbitMQ On macOS (using Homebrew) brew install rabbitmq brew services start rabbitmq On Ubuntu/Debian sudo apt update sudo apt install rabbitmq-server sudo systemctl enable rabbitmq-server sudo systemctl start rabbitmq-server On Windows Download and install RabbitMQ from the official website and ensure the service is running. To check if RabbitMQ is running, use: rabbitmqctl status Installing Required Node.js Packages We will use the amqplib package to interact with RabbitMQ in Node.js. npm install amqplib Basic Concepts in RabbitMQ Producer: Sends messages to RabbitMQ. Queue: Stores messages temporarily. Consumer: Receives and processes messages. Exchange: Routes messages to the appropriate queue. Example: Sending and Receiving Messages Step 1: Setting Up a Producer Create a file producer.js: const amqp = require('amqplib'); async function sendMessage() { const connection = await amqp.connect('amqp://localhost'); const channel = await connection.createChannel(); const queue = 'task_queue'; await channel.assertQueue(queue, { durable: true }); const message = 'Hello, RabbitMQ!'; channel.sendToQueue(queue, Buffer.from(message), { persistent: true }); console.log(`Sent: ${message}`); setTimeout(() => { connection.close(); process.exit(0); }, 500); } sendMessage().catch(console.error); Step 2: Setting Up a Consumer Create a file consumer.js: const amqp = require('amqplib'); async function receiveMessage() { const connection = await amqp.connect('amqp://localhost'); const channel = await connection.createChannel(); const queue = 'task_queue'; await channel.assertQueue(queue, { durable: true }); console.log(`Waiting for messages in ${queue}...`); channel.consume(queue, (msg) => { console.log(`Received: ${msg.content.toString()}`); channel.ack(msg); }, { noAck: false }); } receiveMessage().catch(console.error); Running the Example Start the consumer: node consumer.js Start the producer to send a message: node producer.js You should see the consumer receiving and processing the message. Advanced Usage Using Exchanges Instead of sending messages directly to a queue, we can use exchanges to route messages to multiple queues based on routing keys. Direct Exchange: Routes messages based on a specific routing key. Fanout Exchange: Sends messages to all bound queues. Topic Exchange: Uses wildcard patterns for routing keys. Example: channel.assertExchange('logs', 'fanout', { durable: false }); channel.publish('logs', '', Buffer.from('Log message!')); Conclusion RabbitMQ is a robust and flexible message broker that can greatly improve the scalability and resilience of your Node.js applications. By following this guide, you now have a working setup to integrate RabbitMQ in your projects. Try experimenting with different messaging patterns to optimize your architecture! References RabbitMQ Official Documentation amqplib GitHub
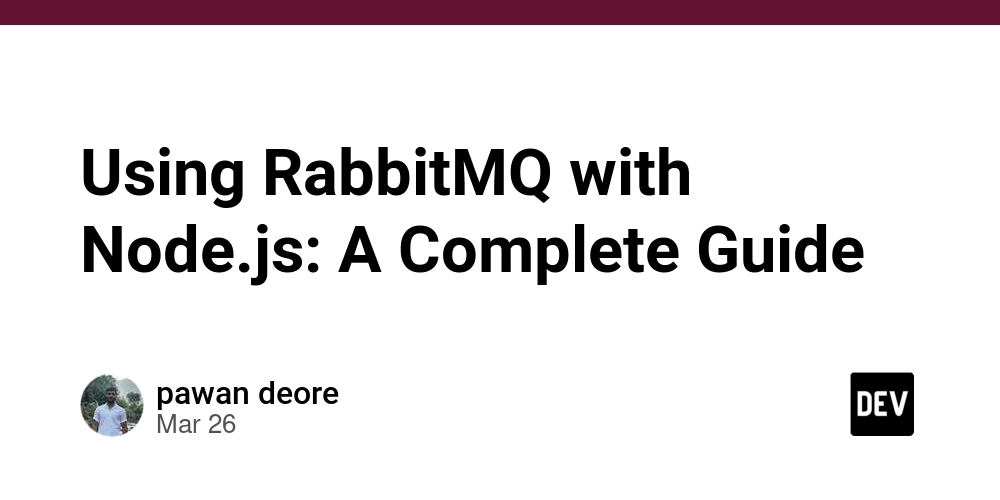
Introduction
RabbitMQ is a powerful message broker that enables asynchronous communication between different parts of an application. It supports multiple messaging patterns and is widely used for microservices and distributed systems.
In this guide, we will explore how to integrate RabbitMQ with Node.js, covering installation, setup, and a working example.
Learn NodeJs by Building Projects
Prerequisites
- Node.js installed (v14+ recommended)
- RabbitMQ installed on your system
Installing RabbitMQ
On macOS (using Homebrew)
brew install rabbitmq
brew services start rabbitmq
On Ubuntu/Debian
sudo apt update
sudo apt install rabbitmq-server
sudo systemctl enable rabbitmq-server
sudo systemctl start rabbitmq-server
On Windows
Download and install RabbitMQ from the official website and ensure the service is running.
To check if RabbitMQ is running, use:
rabbitmqctl status
Installing Required Node.js Packages
We will use the amqplib
package to interact with RabbitMQ in Node.js.
npm install amqplib
Basic Concepts in RabbitMQ
- Producer: Sends messages to RabbitMQ.
- Queue: Stores messages temporarily.
- Consumer: Receives and processes messages.
- Exchange: Routes messages to the appropriate queue.
Example: Sending and Receiving Messages
Step 1: Setting Up a Producer
Create a file producer.js
:
const amqp = require('amqplib');
async function sendMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'task_queue';
await channel.assertQueue(queue, { durable: true });
const message = 'Hello, RabbitMQ!';
channel.sendToQueue(queue, Buffer.from(message), { persistent: true });
console.log(`Sent: ${message}`);
setTimeout(() => {
connection.close();
process.exit(0);
}, 500);
}
sendMessage().catch(console.error);
Step 2: Setting Up a Consumer
Create a file consumer.js
:
const amqp = require('amqplib');
async function receiveMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'task_queue';
await channel.assertQueue(queue, { durable: true });
console.log(`Waiting for messages in ${queue}...`);
channel.consume(queue, (msg) => {
console.log(`Received: ${msg.content.toString()}`);
channel.ack(msg);
}, { noAck: false });
}
receiveMessage().catch(console.error);
Running the Example
- Start the consumer:
node consumer.js
- Start the producer to send a message:
node producer.js
You should see the consumer receiving and processing the message.
Advanced Usage
Using Exchanges
Instead of sending messages directly to a queue, we can use exchanges to route messages to multiple queues based on routing keys.
- Direct Exchange: Routes messages based on a specific routing key.
- Fanout Exchange: Sends messages to all bound queues.
- Topic Exchange: Uses wildcard patterns for routing keys.
Example:
channel.assertExchange('logs', 'fanout', { durable: false });
channel.publish('logs', '', Buffer.from('Log message!'));
Conclusion
RabbitMQ is a robust and flexible message broker that can greatly improve the scalability and resilience of your Node.js applications. By following this guide, you now have a working setup to integrate RabbitMQ in your projects. Try experimenting with different messaging patterns to optimize your architecture!