Understanding the V8 Garbage Collector
Understanding the V8 Garbage Collector Introduction JavaScript, as the programming language of the web, has evolved significantly since its inception in 1995. Core to its continuous evolution is the V8 JavaScript engine, developed by Google, which compiles JavaScript into machine code and optimizes the execution of JavaScript applications. One of the critical components of V8 is its garbage collector. This article aims to provide an exhaustive examination of the V8 garbage collector by exploring its architecture, historical context, implementation details, comparative methodologies, and performance optimizations. Historical and Technical Context Before V8, JavaScript engines primarily employed simple reference counting approaches. Reference counting struggles with circular references and can lead to memory leaks if objects reference each other indefinitely. With the rise of complex web applications and sophisticated JavaScript frameworks, it became apparent that a more efficient method was necessary. V8 was open-sourced in 2008 as part of Google's Chrome project, introducing a modern garbage collection algorithm based on generational garbage collection principles. This design recognizes that most objects are short-lived, with many dying soon after creation. Generational Garbage Collection The generational hypothesis postulates that newer objects are more likely to become unreachable than older objects. The V8 engine thus divides the heap memory into different segments: New Space: This is the nursery area where all newly created objects are stored. Old Space: Objects that survive garbage collection in New Space get promoted here. Large Object Space (LOS): Contains larger objects that are less commonly allocated. This generational approach enables V8 to employ different collection strategies, optimizing memory management significantly. A Brief Look at Algorithms Used V8 employs several algorithms to keep memory in check: Mark-and-Sweep: This is a two-phase algorithm that marks live objects and cleans up those that aren't. Scavenging: A form of semi-space collection where allocated memory is divided into two equal halves. When one half fills up, V8 copies the live objects to the other half and reclaims the memory in the filled half. Compaction: V8 uses this to reduce memory fragmentation; it rearranges allocated objects to be contiguous in memory and thus optimizes space allocation. Detailed V8 Garbage Collector Architecture The architecture of V8's garbage collector is designed to maximize efficiency and minimize latency. Here’s a deeper dive into the primary components: Heap: This is where all objects are stored and comprises several segments mentioned above: New Space and Old Space, along with the Large Object Space. Garbage Collection Process: Scavenge: This is a minor GC that focuses on New Space. If an object survives a certain number of GCs, it is moved to Old Space. Mark Phase: The GC scans the heap for reachable objects, marking them. Sweep Phase: All unmarked objects are removed from the heap. Compaction: This phase may occur during a major GC, reducing memory fragmentation. Customizable Triggers: Developers can trigger garbage collection manually using global.gc(), though this is typically discouraged in performance-critical applications. In-Depth Code Examples Basic Demo of Garbage Collection Let’s explore a simple example demonstrating the GC process: function createObject() { // An object that will be created and removed let obj = {}; console.log('Object created'); return obj; } let reference = createObject(); // The object is immediately created reference = null; // The reference is cleared, eligible for garbage collection // Requesting GC (only works when running with --expose-gc) if (global.gc) { console.log('Running garbage collection...'); global.gc(); // Explicitly requesting garbage collection } Advanced Scenario: Circular References This example demonstrates how the V8 engine handles circular references by using weak references. let objectA = {}; let objectB = {}; objectA.ref = objectB; objectB.ref = objectA; // Creating a circular reference objectA = null; // Both objects still referenced objectB = null; // They become eligible for GC // This won’t remove circular references in older engines In modern V8, these objects will eventually be cleared out during a mark-and-sweep process, illustrating the importance of understanding object lifecycle management explicitly. Real-World Applications The graph below showcases a high-level view of complex applications utilizing V8’s garbage collector for efficient memory management: Node.js: Utilizes V8’s garbage collector for its non-blocking architecture, ensuring that long-running processes do not degrade performance. Angular: Application architected arou
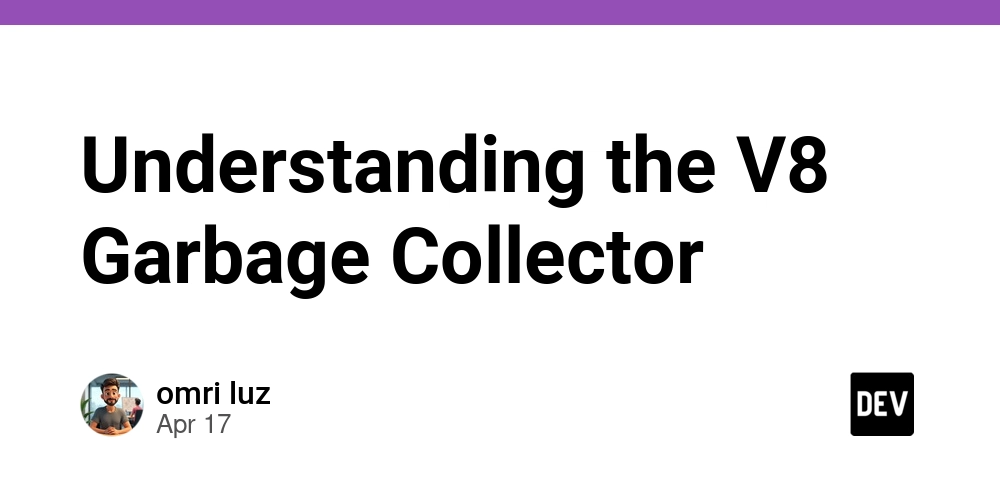
Understanding the V8 Garbage Collector
Introduction
JavaScript, as the programming language of the web, has evolved significantly since its inception in 1995. Core to its continuous evolution is the V8 JavaScript engine, developed by Google, which compiles JavaScript into machine code and optimizes the execution of JavaScript applications. One of the critical components of V8 is its garbage collector. This article aims to provide an exhaustive examination of the V8 garbage collector by exploring its architecture, historical context, implementation details, comparative methodologies, and performance optimizations.
Historical and Technical Context
Before V8, JavaScript engines primarily employed simple reference counting approaches. Reference counting struggles with circular references and can lead to memory leaks if objects reference each other indefinitely. With the rise of complex web applications and sophisticated JavaScript frameworks, it became apparent that a more efficient method was necessary.
V8 was open-sourced in 2008 as part of Google's Chrome project, introducing a modern garbage collection algorithm based on generational garbage collection principles. This design recognizes that most objects are short-lived, with many dying soon after creation.
Generational Garbage Collection
The generational hypothesis postulates that newer objects are more likely to become unreachable than older objects. The V8 engine thus divides the heap memory into different segments:
- New Space: This is the nursery area where all newly created objects are stored.
- Old Space: Objects that survive garbage collection in New Space get promoted here.
- Large Object Space (LOS): Contains larger objects that are less commonly allocated.
This generational approach enables V8 to employ different collection strategies, optimizing memory management significantly.
A Brief Look at Algorithms Used
V8 employs several algorithms to keep memory in check:
- Mark-and-Sweep: This is a two-phase algorithm that marks live objects and cleans up those that aren't.
- Scavenging: A form of semi-space collection where allocated memory is divided into two equal halves. When one half fills up, V8 copies the live objects to the other half and reclaims the memory in the filled half.
- Compaction: V8 uses this to reduce memory fragmentation; it rearranges allocated objects to be contiguous in memory and thus optimizes space allocation.
Detailed V8 Garbage Collector Architecture
The architecture of V8's garbage collector is designed to maximize efficiency and minimize latency. Here’s a deeper dive into the primary components:
Heap: This is where all objects are stored and comprises several segments mentioned above: New Space and Old Space, along with the Large Object Space.
-
Garbage Collection Process:
- Scavenge: This is a minor GC that focuses on New Space. If an object survives a certain number of GCs, it is moved to Old Space.
- Mark Phase: The GC scans the heap for reachable objects, marking them.
- Sweep Phase: All unmarked objects are removed from the heap.
- Compaction: This phase may occur during a major GC, reducing memory fragmentation.
Customizable Triggers: Developers can trigger garbage collection manually using
global.gc()
, though this is typically discouraged in performance-critical applications.
In-Depth Code Examples
Basic Demo of Garbage Collection
Let’s explore a simple example demonstrating the GC process:
function createObject() {
// An object that will be created and removed
let obj = {};
console.log('Object created');
return obj;
}
let reference = createObject(); // The object is immediately created
reference = null; // The reference is cleared, eligible for garbage collection
// Requesting GC (only works when running with --expose-gc)
if (global.gc) {
console.log('Running garbage collection...');
global.gc(); // Explicitly requesting garbage collection
}
Advanced Scenario: Circular References
This example demonstrates how the V8 engine handles circular references by using weak references.
let objectA = {};
let objectB = {};
objectA.ref = objectB;
objectB.ref = objectA; // Creating a circular reference
objectA = null; // Both objects still referenced
objectB = null; // They become eligible for GC
// This won’t remove circular references in older engines
In modern V8, these objects will eventually be cleared out during a mark-and-sweep process, illustrating the importance of understanding object lifecycle management explicitly.
Real-World Applications
The graph below showcases a high-level view of complex applications utilizing V8’s garbage collector for efficient memory management:
- Node.js: Utilizes V8’s garbage collector for its non-blocking architecture, ensuring that long-running processes do not degrade performance.
- Angular: Application architected around the garbage collection capabilities of V8, effectively managing object lifecycles and memory.
- Chrome Extensions: Often must implement best practices surrounding object creation and memory cleanup, relying on V8's sophisticated garbage collector.
Performance Considerations and Optimization Strategies
Garbage collection can introduce latency, particularly during major collection cycles. Here are optimization strategies to enhance performance:
- Object Lifetimes: Keep object scopes as tight as possible to minimize memory usage.
- Immutable Data Structures: Utilizing structures that do not require extensive copying can help reduce the stress on the garbage collector.
- Memory Profiling and Monitoring: Tools like Chrome DevTools can help monitor memory usage and identify leaks.
- Avoid Global Variables: Excessive use of global contexts can lead to longer garbage collection times.
Pitfalls and Debugging Techniques
Certain pitfalls can hinder optimal functionality:
- Memory Leaks: Commonly caused by unintentional global variables, event listeners, or closures holding onto large objects longer than necessary.
- Unreleased References: Watch for unintended references that can prevent garbage collection.
Advanced Debugging Techniques
Debugging the garbage collector requires tools and techniques that provide visibility into the memory usage:
- Heap Snapshots: Create snapshots in Chrome DevTools to analyze memory allocations and identify leaks.
- Timeline Viewers: Identify which parts of the application trigger garbage collection processes. This is useful to optimize performance-sensitive areas.
Advanced Resources
For further exploration, consider the following resources:
- V8's Official Documentation
- Node.js Documentation on Memory Management
- Memory Profiling with Chrome DevTools
Conclusion
The V8 garbage collector is an intricately designed component essential for efficient JavaScript memory management. By understanding its architecture, strategies, and challenges, senior developers can optimize application performance, avoid pitfalls, and leverage the capabilities of the V8 engine effectively. As modern JavaScript applications grow in complexity, mastering the nuances of garbage collection will be paramount for building reliable and maintainable applications.