Understanding REST APIs and Best Practices (With CampusX Implementation)
What is a REST API? A REST API (Representational State Transfer API) is a way for different systems to communicate over HTTP using standard methods like GET, POST, PUT, DELETE. It follows principles that make it scalable, stateless, and easy to integrate. Key Features of REST APIs: Stateless – Each request from a client contains all necessary information, and the server doesn’t store session data. Client-Server Architecture – The client and server operate independently, improving scalability. Resource-Based – Data is treated as resources (e.g., /users, /posts). Use of HTTP Methods – Follows standard methods: GET – Retrieve data POST – Create a resource PUT/PATCH – Update a resource DELETE – Remove a resource JSON as Response Format – Mostly uses JSON for structured data exchange. Stateless Authentication – Uses tokens like JWT or OAuth. Best Practices for Designing REST APIs 1. Use Meaningful Resource Names Instead of /getUserDetails?id=123, use /users/123. 2. Follow HTTP Methods Correctly GET /users → Fetch all users POST /users → Create a new user PUT /users/123 → Update user with ID 123 DELETE /users/123 → Remove user with ID 123 3. Use Status Codes Properly 200 OK → Success 201 Created → Resource Created 400 Bad Request → Invalid Input 401 Unauthorized → Authentication Required 404 Not Found → Resource Doesn’t Exist 500 Internal Server Error → Unexpected Issue 4. Paginate Large Results For large datasets, use pagination: GET /posts?page=2&limit=10 5. Secure APIs with Authentication Use JWT (JSON Web Tokens) for authentication. Implement rate limiting to prevent abuse. Use HTTPS to encrypt communication. 6. Implement Versioning APIs evolve over time, so version them: GET /v1/users GET /v2/users 7. Use Consistent Error Handling Standard error responses help debugging. Example: { "error": { "code": 400, "message": "Invalid input", "details": "Email field is required" } } How REST APIs Are Used in CampusX 1. User Authentication (JWT) CampusX uses JWT-based authentication: POST /register → Registers a new user with profile images. POST /login → Logs in a user, returns JWT token. POST /logout → Logs out a user. POST /refresh-token → Refreshes access token. POST /change-password → Changes the current password. GET /current-user → Fetches authenticated user details. 2. Post Management Users can create and manage posts related to VTU exams, placements, college clubs, etc.: POST / → Creates a new post (Authenticated users only, with image upload). GET / → Fetches all posts. GET /:id → Fetches a specific post. DELETE /:id → Deletes a post (Only by the author). 3. Comments & Engagements Users can engage through comments: POST /:postId/comments → Adds a comment. GET /:postId/comments → Fetches comments. DELETE /:postId/comments/:commentId → Deletes a comment. 4. User Profile & Account Management Users can update their profiles: GET /channel/:user → Fetches a user’s profile. PATCH /update-account → Updates account details. PATCH /update-avatar → Updates profile avatar. PATCH /update-coverimage → Updates cover image. DELETE /delete-account → Deletes a user account. 5. Bookmark & Follow System Users can bookmark posts and follow/unfollow others: POST /:postId → Bookmark a post. DELETE /:bookmarkId → Remove a bookmark. GET / → Get user bookmarks. POST /:userId/follow → Follow a user. POST /:userId/unfollow → Unfollow a user. Conclusion REST APIs power CampusX, making it a scalable and flexible college-specific discussion platform. By following REST API best practices, we ensure clean, maintainable, and secure APIs. Have questions? Drop them in the comments!
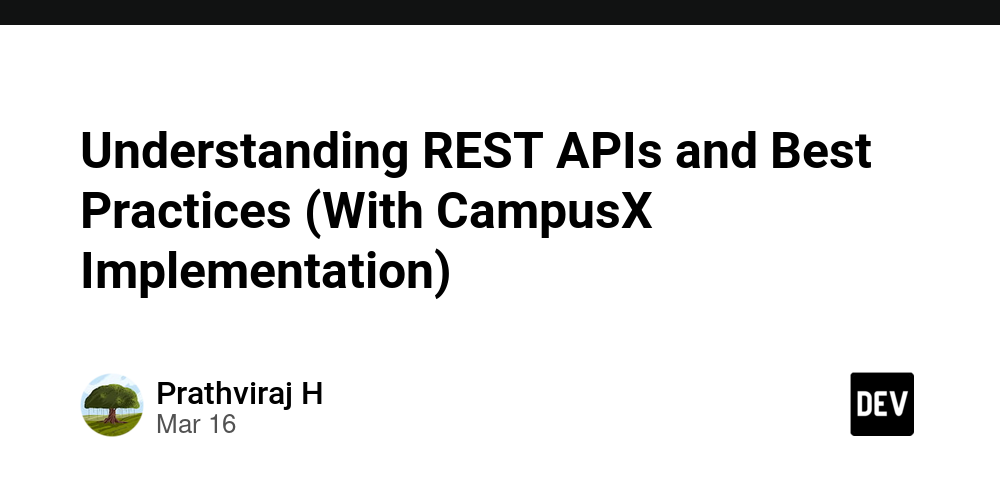
What is a REST API?
A REST API (Representational State Transfer API) is a way for different systems to communicate over HTTP using standard methods like GET, POST, PUT, DELETE. It follows principles that make it scalable, stateless, and easy to integrate.
Key Features of REST APIs:
- Stateless – Each request from a client contains all necessary information, and the server doesn’t store session data.
- Client-Server Architecture – The client and server operate independently, improving scalability.
-
Resource-Based – Data is treated as resources (e.g.,
/users
,/posts
). -
Use of HTTP Methods – Follows standard methods:
-
GET
– Retrieve data -
POST
– Create a resource -
PUT/PATCH
– Update a resource -
DELETE
– Remove a resource
-
- JSON as Response Format – Mostly uses JSON for structured data exchange.
- Stateless Authentication – Uses tokens like JWT or OAuth.
Best Practices for Designing REST APIs
1. Use Meaningful Resource Names
Instead of /getUserDetails?id=123
, use /users/123
.
2. Follow HTTP Methods Correctly
-
GET /users
→ Fetch all users -
POST /users
→ Create a new user -
PUT /users/123
→ Update user with ID 123 -
DELETE /users/123
→ Remove user with ID 123
3. Use Status Codes Properly
-
200 OK
→ Success -
201 Created
→ Resource Created -
400 Bad Request
→ Invalid Input -
401 Unauthorized
→ Authentication Required -
404 Not Found
→ Resource Doesn’t Exist -
500 Internal Server Error
→ Unexpected Issue
4. Paginate Large Results
For large datasets, use pagination:
GET /posts?page=2&limit=10
5. Secure APIs with Authentication
- Use JWT (JSON Web Tokens) for authentication.
- Implement rate limiting to prevent abuse.
- Use HTTPS to encrypt communication.
6. Implement Versioning
APIs evolve over time, so version them:
GET /v1/users
GET /v2/users
7. Use Consistent Error Handling
Standard error responses help debugging. Example:
{
"error": {
"code": 400,
"message": "Invalid input",
"details": "Email field is required"
}
}
How REST APIs Are Used in CampusX
1. User Authentication (JWT)
CampusX uses JWT-based authentication:
-
POST /register
→ Registers a new user with profile images. -
POST /login
→ Logs in a user, returns JWT token. -
POST /logout
→ Logs out a user. -
POST /refresh-token
→ Refreshes access token. -
POST /change-password
→ Changes the current password. -
GET /current-user
→ Fetches authenticated user details.
2. Post Management
Users can create and manage posts related to VTU exams, placements, college clubs, etc.:
-
POST /
→ Creates a new post (Authenticated users only, with image upload). -
GET /
→ Fetches all posts. -
GET /:id
→ Fetches a specific post. -
DELETE /:id
→ Deletes a post (Only by the author).
3. Comments & Engagements
Users can engage through comments:
-
POST /:postId/comments
→ Adds a comment. -
GET /:postId/comments
→ Fetches comments. -
DELETE /:postId/comments/:commentId
→ Deletes a comment.
4. User Profile & Account Management
Users can update their profiles:
-
GET /channel/:user
→ Fetches a user’s profile. -
PATCH /update-account
→ Updates account details. -
PATCH /update-avatar
→ Updates profile avatar. -
PATCH /update-coverimage
→ Updates cover image. -
DELETE /delete-account
→ Deletes a user account.
5. Bookmark & Follow System
Users can bookmark posts and follow/unfollow others:
-
POST /:postId
→ Bookmark a post. -
DELETE /:bookmarkId
→ Remove a bookmark. -
GET /
→ Get user bookmarks. -
POST /:userId/follow
→ Follow a user. -
POST /:userId/unfollow
→ Unfollow a user.
Conclusion
REST APIs power CampusX, making it a scalable and flexible college-specific discussion platform. By following REST API best practices, we ensure clean, maintainable, and secure APIs.
Have questions? Drop them in the comments!