Understanding Flutter Widgets: The Magic Behind the Screen (Without Losing Performance)
So, you've heard it a million times: "Everything in Flutter is a widget!" But what does that actually mean? The Flutter framework hides most of the implementation details from developers. In simple terms, we can say that everything you see on the screen is a widget, but as your app grows and you start facing performance issues with content flickering on screen, you need to understand what's really happening under the hood. Widgets Are Just Blueprints (And They're Immutable) When you see movement on your screen after clicking a button, it's actually due to frames. What you're seeing is like a series of images, and when your device shows these images continuously, your brain perceives it as motion. In reality, your device is displaying different images each second, creating the illusion of movement. This becomes important when we think about performance. Most devices refresh at 60Hz to 120Hz, meaning your Flutter app needs to create a new frame every 8-16ms (1000ms ÷ 120 frames = ~8ms at 120Hz). That's not a lot of time! Think of widgets like blueprints for a house—you can't renovate the blueprint itself; you need to create a new one. Once you create a widget, you can't change its properties because widgets in Flutter are immutable. The Widget Paradox: Immutable Yet Changing Let's dig deeper into our question: what is a widget? When we create a widget, we're actually extending either a StatefulWidget or StatelessWidget. Looking at the source code, we can see: abstract class StatelessWidget extends Widget {...} abstract class StatefulWidget extends Widget {...} “@immutable abstract class Widget {...} Both of these extend from the base Widget class. Now here's where it gets interesting - when we look at the Widget class, we discover that widgets themselves are immutable! This means we can't change a widget's properties after it's created. This raises an important question: if widgets are immutable, how can StatefulWidget change its properties when we call setState()? The answer is surprising: Flutter doesn't actually modify the widget - it creates an entirely new widget and rebuilds the widget tree! Yes, you read that right - it recreates the whole widget tree. "Hold up! Rebuilding the whole tree every time? That sounds terrible for performance!" You're right to panic. But Flutter is smarter than that. After all, the build method is called as often as your device's refresh rate (60-120 times per second). Flutter has to recreate the entire widget tree in just 8ms. If Flutter recreated every widget from scratch every frame, your app would be a slideshow. How does Flutter handle this without performance issues? The Build Method Runs Constantly (But Your Phone Doesn't Explode) To understand how Flutter maintains performance while constantly rebuilding widgets, we need to explore three core concepts - the pillars of Flutter's architecture: Widgets (the "what" to display - Configuration) Elements (the "how" to display it - Reconciliation) RenderObjects (the "where" to paint pixels - Rendering) Because widgets aren't what you see on the screen. They're just configurations. The real magic happens behind the scenes with these three pillars working together seamlessly. RenderObjects: The Actual Painters When we create custom widgets, we extend StatefulWidget or StatelessWidget, and inside those widgets, we use Flutter's built-in widgets like Container and Text. Have you ever wondered how Flutter actually displays these widgets on screen? Let's look at the SizedBox widget as an example. Unlike our custom widgets, SizedBox doesn't extend StatefulWidget or StatelessWidget. Instead, it extends RenderObjectWidget, which has an associated RenderObject property. This RenderObject is what's actually responsible for rendering widgets on screen. RenderObjects are responsible for: Layout (size and position) Painting (drawing pixels on the screen) Every widget in Flutter is somehow related to a RenderObject because RenderObjects know how to display content. When we create a widget, Flutter's build system associates a RenderObject behind the scenes. This RenderObject handles the actual layout and painting on screen. But RenderObjects are expensive to create. So Flutter doesn't rebuild them every frame. Instead, it reuses them. How? That's where Elements come in. If you need a custom widget with special layout and painting requirements, you can extend RenderObjectWidget and implement its RenderObject property so your widget knows exactly how to render on screen using your custom layout and painting logic. Here's another example with the Text widget: void main() { runApp( const MaterialApp( home: Text('Hello, Flutter'), ), ); } I've used two widgets here: MaterialApp (which extends StatefulWidget) and Text (which extends StatelessWidget). But if we look deeper inside the Text widget, we'll see that its build method returns a RichTex
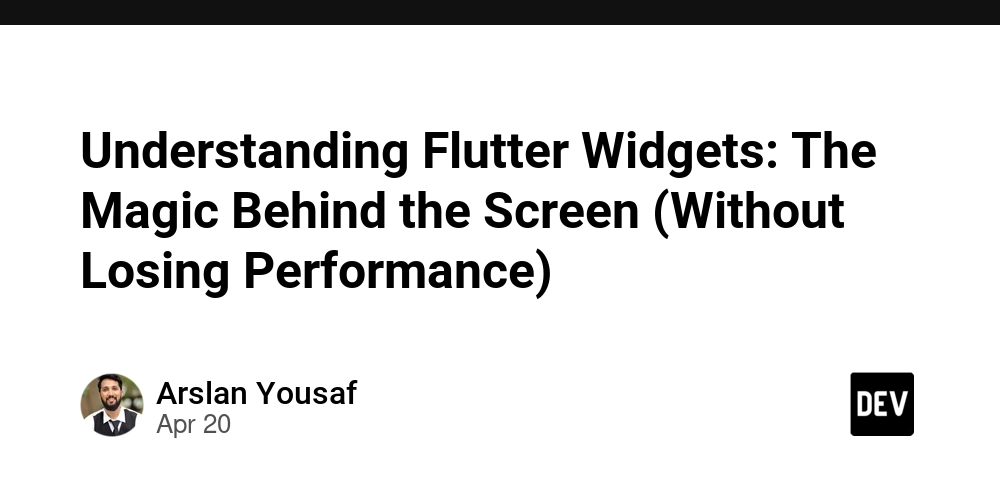
So, you've heard it a million times: "Everything in Flutter is a widget!" But what does that actually mean? The Flutter framework hides most of the implementation details from developers. In simple terms, we can say that everything you see on the screen is a widget, but as your app grows and you start facing performance issues with content flickering on screen, you need to understand what's really happening under the hood.
Widgets Are Just Blueprints (And They're Immutable)
When you see movement on your screen after clicking a button, it's actually due to frames. What you're seeing is like a series of images, and when your device shows these images continuously, your brain perceives it as motion. In reality, your device is displaying different images each second, creating the illusion of movement.
This becomes important when we think about performance. Most devices refresh at 60Hz to 120Hz, meaning your Flutter app needs to create a new frame every 8-16ms (1000ms ÷ 120 frames = ~8ms at 120Hz). That's not a lot of time!
Think of widgets like blueprints for a house—you can't renovate the blueprint itself; you need to create a new one. Once you create a widget, you can't change its properties because widgets in Flutter are immutable.
The Widget Paradox: Immutable Yet Changing
Let's dig deeper into our question: what is a widget? When we create a widget, we're actually extending either a StatefulWidget or StatelessWidget. Looking at the source code, we can see:
abstract class StatelessWidget extends Widget {...}
abstract class StatefulWidget extends Widget {...}
“@immutable
abstract class Widget {...}
Both of these extend from the base Widget class. Now here's where it gets interesting - when we look at the Widget class, we discover that widgets themselves are immutable! This means we can't change a widget's properties after it's created.
This raises an important question: if widgets are immutable, how can StatefulWidget change its properties when we call setState()?
The answer is surprising: Flutter doesn't actually modify the widget - it creates an entirely new widget and rebuilds the widget tree! Yes, you read that right - it recreates the whole widget tree.
"Hold up! Rebuilding the whole tree every time? That sounds terrible for performance!"
You're right to panic. But Flutter is smarter than that. After all, the build method is called as often as your device's refresh rate (60-120 times per second). Flutter has to recreate the entire widget tree in just 8ms. If Flutter recreated every widget from scratch every frame, your app would be a slideshow. How does Flutter handle this without performance issues?
The Build Method Runs Constantly (But Your Phone Doesn't Explode)
To understand how Flutter maintains performance while constantly rebuilding widgets, we need to explore three core concepts - the pillars of Flutter's architecture:
- Widgets (the "what" to display - Configuration)
- Elements (the "how" to display it - Reconciliation)
- RenderObjects (the "where" to paint pixels - Rendering)
Because widgets aren't what you see on the screen. They're just configurations. The real magic happens behind the scenes with these three pillars working together seamlessly.
RenderObjects: The Actual Painters
When we create custom widgets, we extend StatefulWidget or StatelessWidget, and inside those widgets, we use Flutter's built-in widgets like Container and Text. Have you ever wondered how Flutter actually displays these widgets on screen?
Let's look at the SizedBox widget as an example. Unlike our custom widgets, SizedBox doesn't extend StatefulWidget or StatelessWidget. Instead, it extends RenderObjectWidget, which has an associated RenderObject property. This RenderObject is what's actually responsible for rendering widgets on screen.
RenderObjects are responsible for:
- Layout (size and position)
- Painting (drawing pixels on the screen)
Every widget in Flutter is somehow related to a RenderObject because RenderObjects know how to display content. When we create a widget, Flutter's build system associates a RenderObject behind the scenes. This RenderObject handles the actual layout and painting on screen.
But RenderObjects are expensive to create. So Flutter doesn't rebuild them every frame. Instead, it reuses them. How? That's where Elements come in.
If you need a custom widget with special layout and painting requirements, you can extend RenderObjectWidget and implement its RenderObject property so your widget knows exactly how to render on screen using your custom layout and painting logic.
Here's another example with the Text widget:
void main() {
runApp(
const MaterialApp(
home: Text('Hello, Flutter'),
),
);
}
I've used two widgets here: MaterialApp (which extends StatefulWidget) and Text (which extends StatelessWidget). But if we look deeper inside the Text widget, we'll see that its build method returns a RichText widget:
class Text extends StatelessWidget {
@override
Widget build(BuildContext context) {
return RichText(...);
}
}
class RichText extends MultiChildRenderObjectWidget {...}
RichText extends MultiChildRenderObjectWidget, which is a subtype of RenderObjectWidget. So even though we didn't do it explicitly, the last widget in our widget tree extends RenderObjectWidget.
Elements: The Middle Managers
Now we understand that widgets are just configurations and RenderObjects are responsible for displaying widgets on screen. But there's a third pillar of the build system: Elements.
Elements are the glue between widgets and RenderObjects. They do two critical things:
- Hold references to the widget and RenderObject
- Manage updates when the widget changes
RenderObjectWidget has methods like createRenderObject and updateRenderObject. We know that RenderObjects, like widgets, are immutable. So how do they update anything on screen?
When we examine the Widget class, we find this method:
@immutable
abstract class Widget {
Element createElement();
}
This method returns an Element. Elements are responsible for updating widgets because while widgets themselves are immutable, their associated Elements are not. When a widget's properties change and the build method is called, a new widget is created with the changed properties. The Element that's associated with the widget discards the reference to the old widget, connects with the new widget, and tells the associated RenderObject to repaint with the new properties.
Let's go even deeper. When a new Element is created, it associates itself with a widget reference:
abstract class Element {
Widget? _widget;
Element(Widget widget)
: _widget = widget {...}
}
When updates happen, the framework calls the update method of the Element class, which replaces the old widget reference with the new widget reference:
abstract class Element {
void update(covariant Widget newWidget) {
_widget = newWidget;
}
}
When you call setState(), Flutter:
- Creates a new widget with updated properties
- Calls update() on the Element, swapping the old widget for the new one
- The Element tells the RenderObject to reconfigure itself (not recreate!) based on the new widget
Why Rebuilding Widgets Isn't a Disaster
Let's connect the dots and see how Flutter maintains performance despite constantly rebuilding widgets:
- Widgets: Cheap, lightweight configurations. They're immutable blueprints that describe the UI. Rebuilding them is fast.
- Elements: Maintain the state and lifecycle of widgets. They compare old and new widgets—if the type and key are the same, they update the existing RenderObject. If not, they destroy and recreate.
- RenderObjects: Do the actual drawing on screen. They're expensive, but reused whenever possible.
When setState() is called:
- Flutter creates a new widget with updated properties
- The Element updates its reference to point to the new widget
- The Element tells its RenderObject to update based on the new widget's configuration
- Only the parts of the screen that need to be redrawn are updated
This elegant system allows Flutter to maintain high performance even when frequently rebuilding the widget tree. So when your build() method runs 120 times per second, Flutter isn't redrawing the entire screen. It's just updating configurations and letting the Element/RenderObject duo handle the rest efficiently.
When Performance Goes Wrong
If your app lags or flickers, it's usually because:
- Your build() method is doing heavy work (e.g., parsing JSON in a widget)
- Unnecessary repaints: RenderObjects are forced to repaint due to bad widget design
Pro Tip: Use const widgets wherever possible. They help Flutter skip rebuilds.
Understanding these concepts helps you write more performant Flutter apps by being mindful of when and how often you trigger rebuilds, and by using techniques like const constructors and Keys appropriately.
Final Thoughts
Widgets are just the tip of the iceberg. The real heroes are Elements and RenderObjects, which work together to make Flutter fast. So next time someone says, "Everything is a widget!", you can smirk and say, "Yes, but Elements and RenderObjects do the heavy lifting."
Now go build something awesome—and keep an eye on that widget tree!