TS2303: Circular definition of import alias '{0}'
TS2303: Circular definition of import alias '{0}' TypeScript is an open-source programming language developed by Microsoft that builds on top of JavaScript, adding static types (explicitly defined data types such as string, number, boolean, etc.), interfaces, and tools for better, well-structured code. TypeScript is often referred to as a "superset" of JavaScript because it contains everything JavaScript does but adds additional features like static type-checking and advanced tools to reduce bugs in your code. If you're new to TypeScript or want to master it while exploring AI tools, I recommend subscribing to my blog or learning with platforms like GPTeach to accelerate your journey. Now, let's explain the important components first and then dive into the issue at hand, TS2303: Circular definition of import alias '{0}'. What Are Types? In TypeScript, types are a foundational concept. They describe the structure of data within your application, telling the TypeScript compiler what kind of values a variable, function, or object should hold. Here’s an example: let age: number = 25; // 'age' must be a number let name: string = "John"; // 'name' must be a string let isAdmin: boolean = true; // 'isAdmin' must be a boolean Without types, it becomes difficult to verify the behavior of your app at compile time. Types reduce errors by ensuring consistency throughout your code. Why Use Types? Detect errors early at compile time. Make your code easier to read and maintain. Avoid runtime surprises (e.g., mistakenly passing a string where a number is expected). TS2303: Circular definition of import alias '{0}' Now, let’s focus on the main topic: TS2303: Circular definition of import alias '{0}'. This error message indicates a problem related to circular references within your TypeScript project, specifically involving how imports (aliases for modules being imported) are defined. What Does the Error Mean in Human Terms? TypeScript throws TS2303 when it detects a "circular definition" in your imports. This occurs when two or more files depend on each other in a way that creates an infinite loop of references. For example, if Module A depends on Module B, but Module B also depends on Module A, TypeScript gets confused while trying to resolve the dependencies. This cyclical relationship is what triggers the TS2303 error. Code Example That Causes TS2303 Let’s consider the following code structure that leads to TS2303: Circular definition of import alias '{0}'. File: User.ts import { Auth } from "./Auth"; // Assume we also export something here export const User = { isAuthenticated: Auth.checkAuth(), }; File: Auth.ts import { User } from "./User"; export const Auth = { checkAuth: () => `Checking auth for ${User}`, }; In this example: User.ts imports Auth.ts. Auth.ts imports User.ts. This creates a circular relationship where both files are dependent on each other. This cycle prevents TypeScript from resolving the dependencies, resulting in the error TS2303: Circular definition of import alias '{0}'. How To Fix TS2303: Circular definition of import alias '{0}'? When fixing this error, the goal is to break the circular dependency in a way that removes the infinite loop of file imports. Here are three possible solutions: 1. Restructure Your Code One of the simplest ways to solve a circular import problem is to refactor your codebase so the circular relationship no longer exists. Move the common functionality into a separate file. Example Solution: Create a new file SharedTypes.ts (or any common utility file) to define shared types or logic. File: SharedTypes.ts export type CommonType = { name: string; }; Updated Files: User.ts import { CommonType } from "./SharedTypes"; export const User = { name: "John", details: {} as CommonType, }; Auth.ts import { CommonType } from "./SharedTypes"; export const Auth = { checkAuth: () => "Auth Successful", }; By moving shared logic or declarations into a separate file, you avoid mutual dependency and eliminate the error TS2303: Circular definition of import alias '{0}'. 2. Use Lazy Loading Sometimes it is necessary to keep references circular but avoid eager loading (importing everything at the top of the file). By using lazy loading techniques like function references, you can defer execution until the dependent module is actually needed. Example Solution: // User.ts export const User = { isAuthenticated: () => require('./Auth').Auth.checkAuth(), }; In this solution: We use require instead of import, as require resolves dependencies lazily. This effectively delays the reference to Auth until it is actually invoked. Be cautious as this method is less commonly used in TypeScript compared to ES6 modules. 3. Explicitly Split Functionality Across Modules Someti
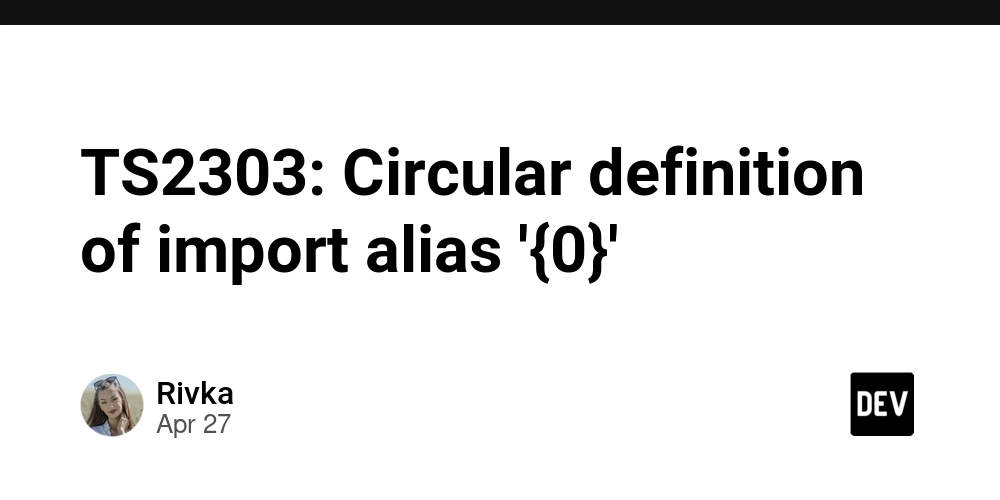
TS2303: Circular definition of import alias '{0}'
TypeScript is an open-source programming language developed by Microsoft that builds on top of JavaScript, adding static types (explicitly defined data types such as string
, number
, boolean
, etc.), interfaces, and tools for better, well-structured code. TypeScript is often referred to as a "superset" of JavaScript because it contains everything JavaScript does but adds additional features like static type-checking and advanced tools to reduce bugs in your code.
If you're new to TypeScript or want to master it while exploring AI tools, I recommend subscribing to my blog or learning with platforms like GPTeach to accelerate your journey.
Now, let's explain the important components first and then dive into the issue at hand, TS2303: Circular definition of import alias '{0}'.
What Are Types?
In TypeScript, types are a foundational concept. They describe the structure of data within your application, telling the TypeScript compiler what kind of values a variable, function, or object should hold. Here’s an example:
let age: number = 25; // 'age' must be a number
let name: string = "John"; // 'name' must be a string
let isAdmin: boolean = true; // 'isAdmin' must be a boolean
Without types, it becomes difficult to verify the behavior of your app at compile time. Types reduce errors by ensuring consistency throughout your code.
Why Use Types?
- Detect errors early at compile time.
- Make your code easier to read and maintain.
- Avoid runtime surprises (e.g., mistakenly passing a string where a number is expected).
TS2303: Circular definition of import alias '{0}'
Now, let’s focus on the main topic: TS2303: Circular definition of import alias '{0}'. This error message indicates a problem related to circular references within your TypeScript project, specifically involving how imports (aliases for modules being imported) are defined.
What Does the Error Mean in Human Terms?
TypeScript throws TS2303 when it detects a "circular definition" in your imports. This occurs when two or more files depend on each other in a way that creates an infinite loop of references. For example, if Module A depends on Module B, but Module B also depends on Module A, TypeScript gets confused while trying to resolve the dependencies. This cyclical relationship is what triggers the TS2303 error.
Code Example That Causes TS2303
Let’s consider the following code structure that leads to TS2303: Circular definition of import alias '{0}'.
File: User.ts
import { Auth } from "./Auth";
// Assume we also export something here
export const User = {
isAuthenticated: Auth.checkAuth(),
};
File: Auth.ts
import { User } from "./User";
export const Auth = {
checkAuth: () => `Checking auth for ${User}`,
};
In this example:
-
User.ts
importsAuth.ts
. -
Auth.ts
importsUser.ts
.
This creates a circular relationship where both files are dependent on each other. This cycle prevents TypeScript from resolving the dependencies, resulting in the error TS2303: Circular definition of import alias '{0}'.
How To Fix TS2303: Circular definition of import alias '{0}'?
When fixing this error, the goal is to break the circular dependency in a way that removes the infinite loop of file imports. Here are three possible solutions:
1. Restructure Your Code
One of the simplest ways to solve a circular import problem is to refactor your codebase so the circular relationship no longer exists. Move the common functionality into a separate file.
Example Solution:
Create a new file SharedTypes.ts
(or any common utility file) to define shared types or logic.
File: SharedTypes.ts
export type CommonType = {
name: string;
};
Updated Files:
User.ts
import { CommonType } from "./SharedTypes";
export const User = {
name: "John",
details: {} as CommonType,
};
Auth.ts
import { CommonType } from "./SharedTypes";
export const Auth = {
checkAuth: () => "Auth Successful",
};
By moving shared logic or declarations into a separate file, you avoid mutual dependency and eliminate the error TS2303: Circular definition of import alias '{0}'.
2. Use Lazy Loading
Sometimes it is necessary to keep references circular but avoid eager loading (importing everything at the top of the file). By using lazy loading techniques like function references, you can defer execution until the dependent module is actually needed.
Example Solution:
// User.ts
export const User = {
isAuthenticated: () => require('./Auth').Auth.checkAuth(),
};
In this solution:
- We use
require
instead ofimport
, asrequire
resolves dependencies lazily. - This effectively delays the reference to
Auth
until it is actually invoked. Be cautious as this method is less commonly used in TypeScript compared to ES6 modules.
3. Explicitly Split Functionality Across Modules
Sometimes circular dependencies point to a deeper design issue. Redesigning and splitting features between modules can solve underlying architectural problems.
Important To Know!
- Circular dependencies are more common in large-scale applications. Refactoring early can help avoid this error altogether.
- Use
--traceResolution
with the TypeScript compiler to debug import resolution and diagnose complex circular reference issues. - While lazy loading techniques like
require
can solve this error temporarily, they should be avoided in most cases as they introduce complexity and may violate ES6 module semantics.
FAQ Section
Q: Is circular dependency unique to TypeScript?
A: No, circular dependencies can occur in any language or framework that supports modules or imports. TypeScript, however, is stricter in catching these issues during compilation.
Q: Can TypeScript always detect circular dependencies?
A: TypeScript won't always detect runtime-based circular dependencies, but it will flag issues related to type definitions during development.
Q: Are there tools to detect circular dependencies?
A: Yes, there are tools like ESLint plugins, dependency graphs, and the depcruise
package that help visualize and resolve circular imports.
Conclusion
Understanding and resolving TS2303: Circular definition of import alias '{0}' requires recognizing how imports create dependencies between files. Circular dependencies can hinder your app's scalability, so solutions like restructuring your code, using lazy loading techniques, or separating shared logic into common utility files are effective in eliminating this error. By being aware of these issues, you can write cleaner and more maintainable TypeScript code.
Want to keep learning about TypeScript? Subscribe to my blog or check out GPTeach to learn how to code smarter with AI-driven tools!