TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions
TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions TypeScript is a strongly typed superset of JavaScript. As a "superset," this means that it builds on top of JavaScript by adding features such as type definitions (to describe the shape or structure of data), interfaces (to define contracts for objects or classes), and enums (to handle named constants). Essentially, TypeScript takes JavaScript to the next level by making your code safer and more predictable. Before diving into the error TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, let’s briefly discuss TypeScript types and why they’re important. A “type” in TypeScript helps developers define what kind of data variables, functions, or objects can accept. For example: let username: string = "Alice"; // `username` must be a string let age: number = 30; // `age` must be a number let isAdmin: boolean = true; // `isAdmin` must be a boolean Using these type annotations ensures fewer bugs in the development process because TypeScript catches type mismatches at compile time, unlike in JavaScript, which can throw unexpected runtime errors. If learning TypeScript excites you, don’t forget to follow my blog or use big AI tools like GPTeach to start your coding journey! What Are Types? To fully understand how to fix issues like TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, let’s deepen our understanding of types. In programming, a type is essentially a blueprint that defines what kind of value a variable or data structure can have. Here’s an example: function add(a: number, b: number): number { return a + b; // Both `a` and `b` must be numbers } Here, we're explicitly telling TypeScript that the arguments a and b should be numbers, and the function itself will return a number. Types make your code more reliable by eliminating guesswork. Understanding TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions This error, TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, generally occurs when TypeScript encounters a problem with how the jsx and jsxs factory functions (React JSX building blocks) are being imported. These factory functions are pivotal for transforming JSX code (the syntax used in React) into JavaScript. What Causes This Error? This issue comes up primarily when: You're using the new React JSX transform introduced in React 17+. Your TypeScript configuration (usually defined in tsconfig.json) is not properly set up to use JSX. Your React version or TypeScript version is mismatched. As an example, let's say you wrote a React component using JSX: const App = () => { return Hello, World!; }; Behind the scenes, TypeScript is supposed to transform that syntax into: jsx("h1", { children: "Hello, World!" }); However, if your TypeScript compiler doesn’t know where to import the jsx (or jsxs) function from, it throws TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions. Solution: Fixing TS1397 Step by Step Here’s how you can solve TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions to get your React project up and running: 1. Update Your TypeScript Configuration First, ensure your tsconfig.json file has the correct compiler options for JSX transformation. Use the jsx option to tell TypeScript how to handle JSX. For the new React 17+ JSX transform, it should look like this: { "compilerOptions": { "jsx": "react-jsx" } } If you are using an older version of React (below 17), set it to "react" instead: { "compilerOptions": { "jsx": "react" } } 2. Check Your React and TypeScript Versions The jsx and jsxs factory functions are only supported in React 17 and above. Ensure you’ve upgraded your React and TypeScript to the latest versions. Run the following commands: npm install react@latest react-dom@latest npm install typescript@latest If you’re stuck with older versions of React for some reason, then use the legacy JSX transform ("jsx": "react"). 3. Install TypeScript Type Definitions When working with React in TypeScript, don’t forget to install the React types. Otherwise, you might encounter issues like TS1397. Install them like this: npm install --save-dev @types/react @types/react-dom These type packages provide TypeScript definitions for React, making it aware of JSX syntax and the jsx/jsxs factory functions. Example: Before and After Fixing TS1397 Before: const App = () => { return Hello, World!; // Error: TS1397 }; After Fix: Update tsconfig.json: { "compilerOptions": { "jsx": "react-jsx" } } Install required dependencies: npm install react react-dom typ
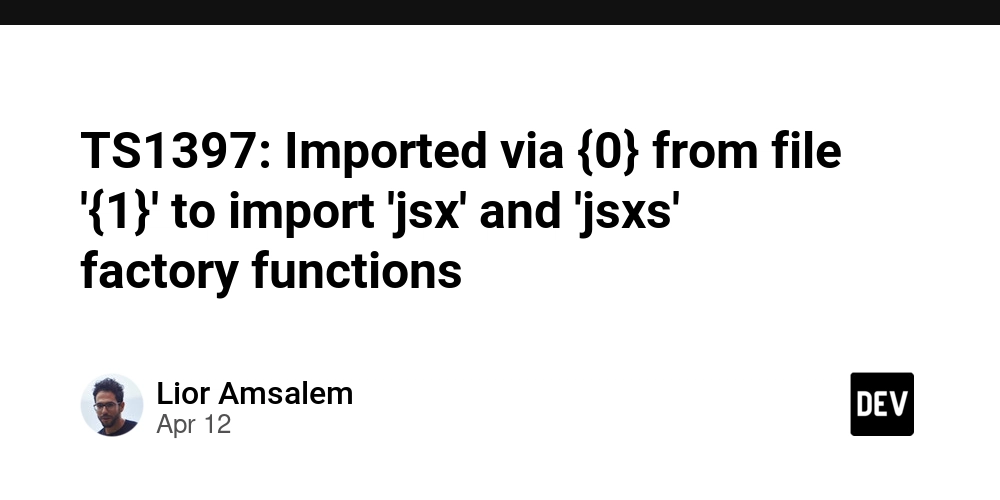
TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions
TypeScript is a strongly typed superset of JavaScript. As a "superset," this means that it builds on top of JavaScript by adding features such as type definitions (to describe the shape or structure of data), interfaces (to define contracts for objects or classes), and enums (to handle named constants). Essentially, TypeScript takes JavaScript to the next level by making your code safer and more predictable.
Before diving into the error TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, let’s briefly discuss TypeScript types and why they’re important. A “type” in TypeScript helps developers define what kind of data variables, functions, or objects can accept. For example:
let username: string = "Alice"; // `username` must be a string
let age: number = 30; // `age` must be a number
let isAdmin: boolean = true; // `isAdmin` must be a boolean
Using these type annotations ensures fewer bugs in the development process because TypeScript catches type mismatches at compile time, unlike in JavaScript, which can throw unexpected runtime errors. If learning TypeScript excites you, don’t forget to follow my blog or use big AI tools like GPTeach to start your coding journey!
What Are Types?
To fully understand how to fix issues like TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, let’s deepen our understanding of types. In programming, a type is essentially a blueprint that defines what kind of value a variable or data structure can have. Here’s an example:
function add(a: number, b: number): number {
return a + b; // Both `a` and `b` must be numbers
}
Here, we're explicitly telling TypeScript that the arguments a
and b
should be numbers, and the function itself will return a number. Types make your code more reliable by eliminating guesswork.
Understanding TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions
This error, TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions, generally occurs when TypeScript encounters a problem with how the jsx
and jsxs
factory functions (React JSX building blocks) are being imported. These factory functions are pivotal for transforming JSX code (the syntax used in React) into JavaScript.
What Causes This Error?
This issue comes up primarily when:
- You're using the new React JSX transform introduced in React 17+.
- Your TypeScript configuration (usually defined in
tsconfig.json
) is not properly set up to use JSX. - Your React version or TypeScript version is mismatched.
As an example, let's say you wrote a React component using JSX:
const App = () => {
return <h1>Hello, World!h1>;
};
Behind the scenes, TypeScript is supposed to transform that syntax into:
jsx("h1", { children: "Hello, World!" });
However, if your TypeScript compiler doesn’t know where to import the jsx
(or jsxs
) function from, it throws TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions.
Solution: Fixing TS1397 Step by Step
Here’s how you can solve TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions to get your React project up and running:
1. Update Your TypeScript Configuration
First, ensure your tsconfig.json
file has the correct compiler options for JSX transformation. Use the jsx
option to tell TypeScript how to handle JSX. For the new React 17+ JSX transform, it should look like this:
{
"compilerOptions": {
"jsx": "react-jsx"
}
}
If you are using an older version of React (below 17), set it to "react"
instead:
{
"compilerOptions": {
"jsx": "react"
}
}
2. Check Your React and TypeScript Versions
The jsx and jsxs factory functions are only supported in React 17 and above. Ensure you’ve upgraded your React and TypeScript to the latest versions. Run the following commands:
npm install react@latest react-dom@latest
npm install typescript@latest
If you’re stuck with older versions of React for some reason, then use the legacy JSX transform ("jsx": "react"
).
3. Install TypeScript Type Definitions
When working with React in TypeScript, don’t forget to install the React types. Otherwise, you might encounter issues like TS1397. Install them like this:
npm install --save-dev @types/react @types/react-dom
These type packages provide TypeScript definitions for React, making it aware of JSX syntax and the jsx
/jsxs
factory functions.
Example: Before and After Fixing TS1397
Before:
const App = () => {
return <h1>Hello, World!h1>; // Error: TS1397
};
After Fix:
Update tsconfig.json
:
{
"compilerOptions": {
"jsx": "react-jsx"
}
}
Install required dependencies:
npm install react react-dom typescript @types/react @types/react-dom
Now, the code compiles without issues.
Important to Know!
- TypeScript 4.1 or later is required to properly utilize the
react-jsx
setting in yourtsconfig.json
. Make sure your project is running at least this version of TypeScript. - The new JSX transform eliminates the need to manually import
React
in every file. For example:
import React from "react"; // No longer needed in React 17+
If you're using React 17+ but still importing React
manually in every file, check if your tsconfig.json
is outdated.
FAQ About TS1397 and TypeScript
Q: Do I always need a tsconfig.json
file?
Yes, the tsconfig.json
file is crucial for any TypeScript project. It tells the TypeScript compiler how to behave and transforms features like JSX correctly.
Q: What are jsx
and jsxs
?
These are factory functions used in JSX transformations. They help convert React components written using JSX syntax (
) into plain JavaScript code.
Hello
Q: How do I know my TypeScript version?
Run the following command in your terminal:
tsc --version
Make sure it’s at least TypeScript 4.1.
Conclusion
The error TS1397: Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions might sound intimidating, but with the right understanding of TypeScript and React, it’s easy to resolve. Make sure to update your tsconfig.json
, React version, and TypeScript dependencies. Following this guide should save you countless hours of debugging!
For more TypeScript tips and guides, join my blog or visit GPTeach.us for interactive AI tutorials.