The Digital Restaurant: Understanding Microservices Through Food Service Analogies
Introduction If you've ever felt lost in the world of microservices architecture, you're not alone. Terms like API Gateway, RabbitMQ, and gRPC can seem overwhelming when you're just starting out. In this post, I'll break down these complex ideas using a simple restaurant menu application as our example – explained so simply that even a 10-year-old could grasp the concepts! What Are Microservices, Anyway? Imagine your restaurant app isn't one big application, but a cafeteria with different people handling specific jobs: Menu Board shows today's food (your menu service) Cashier takes money (order service) Kitchen prepares meals (fulfillment service) Delivery Person brings food to tables (delivery service) Instead of one giant application handling everything, each service focuses on doing one thing really well. But now they need ways to talk to each other! Five Ways Your Menu Service Can Talk to Other Services 1. REST API - Like Asking Questions and Getting Answers How it works: One service asks a specific question, another answers right away. Cashier: "Hey Menu Board, how much is the burger?" Menu Board: "Burgers are $7.99!" In code, it looks like this: # Your menu API endpoint @app.get("/menu/items/{item_id}") def get_menu_item(item_id: str): return {"name": "Burger", "price": 7.99, "available": True} # Another service calling your API response = requests.get("http://menu-service/menu/items/burger-123") price = response.json()["price"] Best used when: You need immediate answers to specific questions. 2. API Gateway - Like a School Principal How it works: Instead of customers needing to know where every service is located, they just talk to one person who directs them to the right place. Customer: "I need to see the menu and place an order." API Gateway: "For the menu, go to Door A. For ordering, go to Door B." In code, it looks like this: # API Gateway configuration routes: - path: /menu/** service: menu-service - path: /orders/** service: order-service - path: /payments/** service: payment-service Best used when: Your restaurant app has many different services that users need to access. 3. RabbitMQ - Like Leaving Notes in Mailboxes How it works: When something important happens, a service writes notes and puts them in other services' mailboxes to read when they're ready. Menu Board updates a price, then leaves notes: "Dear Kitchen and Cashier, the burger is now $8.99, not $7.99." In code, it looks like this: # When updating a menu item def update_menu_item(item_id, new_price): # Update database db.update_item(item_id, price=new_price) # Send a message to anyone who cares rabbit_channel.basic_publish( exchange='menu_events', routing_key='menu.price.updated', body=json.dumps({"item_id": item_id, "new_price": new_price}) ) Best used when: You need to tell other services about changes without waiting for them to respond. 4. Kafka - Like School Announcements How it works: Important information is broadcast over a system where many services can listen, and the messages are saved so even services that were "absent" can catch up later. School Announcement: "Attention everyone! The lunch special today is pizza!" In code, it looks like this: # Publishing events about menu views def record_menu_view(user_id, item_id): kafka_producer.send( 'menu_analytics', key=item_id, value={"user_id": user_id, "item_id": item_id, "timestamp": time.time()} ) Best used when: You have lots of data or events that multiple services need to know about. 5. gRPC - Like a Walkie-Talkie How it works: Services use special, super-fast walkie-talkies that understand each other perfectly, with no misunderstandings. Menu Board: "Kitchen, is the burger available?" Kitchen: "Yes, 15 burgers ready!" In code, it looks like this: // Define the communication contract service MenuService { rpc CheckItemAvailability(ItemRequest) returns (AvailabilityResponse); } message ItemRequest { string item_id = 1; } message AvailabilityResponse { bool available = 1; int32 quantity_remaining = 2; } Best used when: Internal services need to talk very quickly and frequently. What Should Your Menu Service Start With? If you're building your first microservice architecture: Start with REST APIs - They're simple, well-understood, and perfect for basic communication Add an API Gateway when you have more than a couple of services Implement RabbitMQ when you need to notify other services about important changes Save Kafka and gRPC for when your restaurant chain grows to multiple locations with thousands of daily orders! Conclusion Microservices don't have to be intimidating. Think of them like a well-run restaurant where each person has a specific
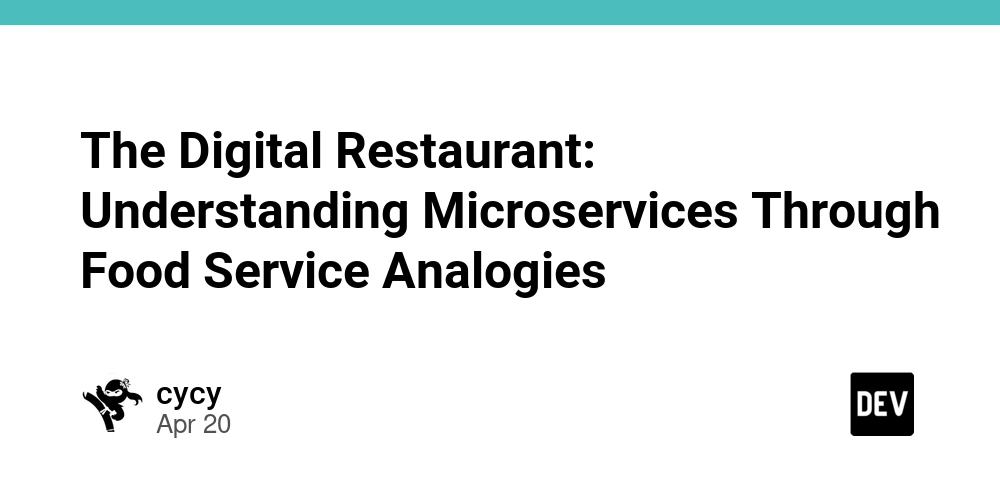
Introduction
If you've ever felt lost in the world of microservices architecture, you're not alone. Terms like API Gateway, RabbitMQ, and gRPC can seem overwhelming when you're just starting out. In this post, I'll break down these complex ideas using a simple restaurant menu application as our example – explained so simply that even a 10-year-old could grasp the concepts!
What Are Microservices, Anyway?
Imagine your restaurant app isn't one big application, but a cafeteria with different people handling specific jobs:
- Menu Board shows today's food (your menu service)
- Cashier takes money (order service)
- Kitchen prepares meals (fulfillment service)
- Delivery Person brings food to tables (delivery service)
Instead of one giant application handling everything, each service focuses on doing one thing really well. But now they need ways to talk to each other!
Five Ways Your Menu Service Can Talk to Other Services
1. REST API - Like Asking Questions and Getting Answers
How it works: One service asks a specific question, another answers right away.
Cashier: "Hey Menu Board, how much is the burger?"
Menu Board: "Burgers are $7.99!"
In code, it looks like this:
# Your menu API endpoint
@app.get("/menu/items/{item_id}")
def get_menu_item(item_id: str):
return {"name": "Burger", "price": 7.99, "available": True}
# Another service calling your API
response = requests.get("http://menu-service/menu/items/burger-123")
price = response.json()["price"]
Best used when: You need immediate answers to specific questions.
2. API Gateway - Like a School Principal
How it works: Instead of customers needing to know where every service is located, they just talk to one person who directs them to the right place.
Customer: "I need to see the menu and place an order."
API Gateway: "For the menu, go to Door A. For ordering, go to Door B."
In code, it looks like this:
# API Gateway configuration
routes:
- path: /menu/**
service: menu-service
- path: /orders/**
service: order-service
- path: /payments/**
service: payment-service
Best used when: Your restaurant app has many different services that users need to access.
3. RabbitMQ - Like Leaving Notes in Mailboxes
How it works: When something important happens, a service writes notes and puts them in other services' mailboxes to read when they're ready.
Menu Board updates a price, then leaves notes:
"Dear Kitchen and Cashier, the burger is now $8.99, not $7.99."
In code, it looks like this:
# When updating a menu item
def update_menu_item(item_id, new_price):
# Update database
db.update_item(item_id, price=new_price)
# Send a message to anyone who cares
rabbit_channel.basic_publish(
exchange='menu_events',
routing_key='menu.price.updated',
body=json.dumps({"item_id": item_id, "new_price": new_price})
)
Best used when: You need to tell other services about changes without waiting for them to respond.
4. Kafka - Like School Announcements
How it works: Important information is broadcast over a system where many services can listen, and the messages are saved so even services that were "absent" can catch up later.
School Announcement: "Attention everyone! The lunch special today is pizza!"
In code, it looks like this:
# Publishing events about menu views
def record_menu_view(user_id, item_id):
kafka_producer.send(
'menu_analytics',
key=item_id,
value={"user_id": user_id, "item_id": item_id, "timestamp": time.time()}
)
Best used when: You have lots of data or events that multiple services need to know about.
5. gRPC - Like a Walkie-Talkie
How it works: Services use special, super-fast walkie-talkies that understand each other perfectly, with no misunderstandings.
Menu Board: "Kitchen, is the burger available?"
Kitchen: "Yes, 15 burgers ready!"
In code, it looks like this:
// Define the communication contract
service MenuService {
rpc CheckItemAvailability(ItemRequest) returns (AvailabilityResponse);
}
message ItemRequest {
string item_id = 1;
}
message AvailabilityResponse {
bool available = 1;
int32 quantity_remaining = 2;
}
Best used when: Internal services need to talk very quickly and frequently.
What Should Your Menu Service Start With?
If you're building your first microservice architecture:
- Start with REST APIs - They're simple, well-understood, and perfect for basic communication
- Add an API Gateway when you have more than a couple of services
- Implement RabbitMQ when you need to notify other services about important changes
Save Kafka and gRPC for when your restaurant chain grows to multiple locations with thousands of daily orders!
Conclusion
Microservices don't have to be intimidating. Think of them like a well-run restaurant where each person has a specific job, and they all need ways to communicate effectively. Whether they're asking direct questions (REST), leaving notes (RabbitMQ), or using walkie-talkies (gRPC), the goal is the same: to coordinate and deliver a great experience to your customers.
Start with the simplest communication methods that meet your needs, and add more sophisticated approaches as your application grows. Your digital menu board might start small today, but with the right communication patterns, it can grow into a full restaurant empire tomorrow!
What communication methods are you using between your microservices? Have you found certain approaches work better for specific scenarios? Share your experiences in the comments below!