Square Every Digit
Instructions: Welcome. In this kata, you are asked to square every digit of a number and concatenate them. For example, if we run 9119 through the function, 811181 will come out, because 92 is 81 and 12 is 1. (81-1-1-81) Example #2: An input of 765 will/should return 493625 because 72 is 49, 62 is 36, and 52 is 25. (49-36-25) Note: The function accepts an integer and returns an integer. Thoughts: I change the number into an array by changing first into a string and split the string. I transform array by using map() function to raise each element of the array to the power of 2 and at the end join them all together. Since i have to return a number at the end I transform the sqDigits from string into number. Solution: function squareDigits(num) { const arr = num.toString().split(""); const sqDigits = arr.map((el) => Math.pow(Number(el), 2)).join(""); return Number(sqDigits); } This is a CodeWars Challenge of 7kyu Rank (https://www.codewars.com/kata/546e2562b03326a88e000020/train/javascript)
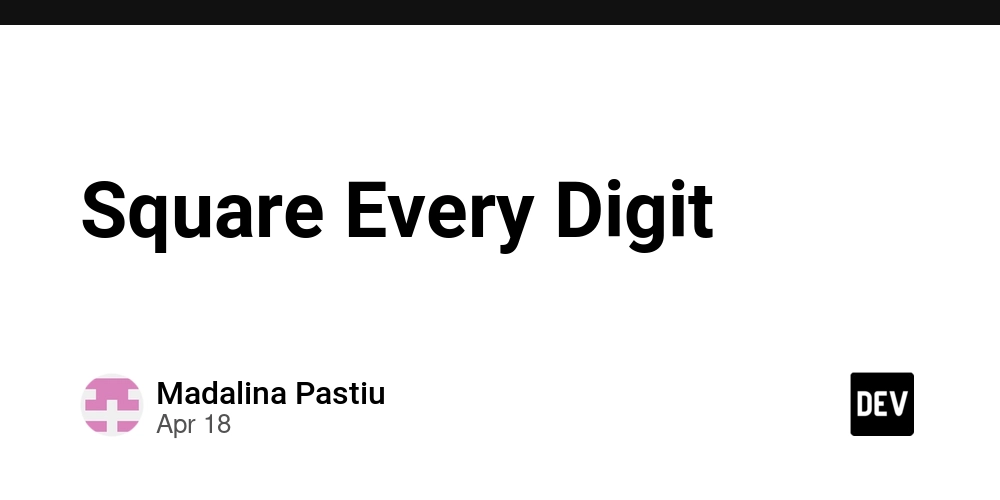
Instructions:
Welcome. In this kata, you are asked to square every digit of a number and concatenate them.
For example, if we run 9119 through the function, 811181 will come out, because 92 is 81 and 12 is 1. (81-1-1-81)
Example #2: An input of 765 will/should return 493625 because 72 is 49, 62 is 36, and 52 is 25. (49-36-25)
Note: The function accepts an integer and returns an integer.
Thoughts:
- I change the number into an array by changing first into a string and split the string.
- I transform array by using map() function to raise each element of the array to the power of 2 and at the end join them all together.
- Since i have to return a number at the end I transform the sqDigits from string into number.
Solution:
function squareDigits(num) {
const arr = num.toString().split("");
const sqDigits = arr.map((el) => Math.pow(Number(el), 2)).join("");
return Number(sqDigits);
}
This is a CodeWars Challenge of 7kyu Rank (https://www.codewars.com/kata/546e2562b03326a88e000020/train/javascript)