Rust Concurrency: When to Use (and Avoid) Async Runtimes
Rust's asynchronous runtimes are extremely useful in a variety of scenarios, especially in high-concurrency and high-performance I/O-intensive applications. Below are some common use cases for Rust async runtimes: Network Programming Web servers and clients: Handling a large number of concurrent connections is a key requirement for web servers. Asynchronous runtimes allow servers to efficiently handle tens of thousands of concurrent requests without creating a separate thread for each connection. Runtimes like Tokio and async-std provide the necessary tools for building high-performance web applications, such as TCP/UDP sockets, HTTP clients, and servers. Real-time communication apps: Chat servers, online game servers, and similar services need to handle many concurrent connections and low-latency message delivery. Async runtimes can efficiently manage these connections and provide fast message routing and processing. Proxy servers and load balancers: These applications need to handle a large volume of concurrent connections and data forwarding. Async runtimes offer high-performance connection management and data transmission capabilities. I/O-Intensive Applications Database drivers and clients: Database operations often involve significant I/O waiting. Async runtimes enable applications to continue executing other tasks while waiting for database responses, improving overall performance. File I/O operations: Applications that process large files or need to access multiple files simultaneously can benefit from async I/O to boost efficiency. Examples include image processing and log analysis. Microservices architecture: In microservices architectures, services usually communicate over the network. Async runtimes can improve communication efficiency and concurrency handling between services. Concurrency and Parallelism Parallel computation: While Rust’s std::thread module can be used for parallel computation, async runtimes are often more effective in I/O-bound scenarios. For instance, they can be used to download multiple files or process multiple network requests in parallel. Task queues and background processing: Async runtimes can be used to implement task queues, allowing time-consuming tasks to be executed asynchronously and avoiding blocking the main thread. For CPU-intensive applications, using an async runtime is typically not the best choice. While Rust async runtimes excel in I/O-heavy applications, they may not bring significant performance improvements in CPU-bound scenarios and could even introduce extra overhead. Why Are Async Runtimes Not Recommended for CPU-Intensive Applications? The primary advantage of async runtimes lies in efficiently handling I/O waits. When a program has to wait for I/O operations (such as network requests or file reads/writes) to complete, an async runtime allows the CPU to perform other tasks in the meantime, thus improving CPU utilization. However, in CPU-intensive applications, the CPU is constantly busy and has no idle time to execute other tasks, meaning the benefits of async runtimes cannot be realized. Async programming introduces additional overhead. It requires maintaining task states, performing context switches, and other operations—all of which come at a cost. In CPU-intensive applications, this overhead may offset or even exceed the potential gains from async programming. Threads are still the preferred choice for CPU-bound tasks. For CPU-intensive workloads, using multithreading is generally more effective. By breaking tasks into multiple subtasks and executing them in parallel across multiple CPU cores, the performance of multi-core processors can be fully leveraged. Rust's std::thread module provides functionality to create and manage threads, making it easy to implement parallel computation. Consider using a thread pool to optimize resource usage. When Should You Consider Using Async Runtimes in CPU-Intensive Applications? Although async runtimes are usually not recommended for purely CPU-bound applications, there are cases where CPU-bound tasks also involve some I/O operations—such as reading configuration files or writing logs. In these cases, using an async runtime may improve the efficiency of those I/O operations. However, it’s important to ensure that I/O operations do not dominate, or the cost may still outweigh the benefits. In addition, if CPU-intensive tasks need to integrate with other async tasks—such as handling network requests—it may make sense to use an async runtime to maintain a unified programming model, which helps organize and maintain code. Although Tokio is primarily optimized for I/O-bound tasks, it also provides mechanisms to handle CPU-bound workloads, preventing them from blocking the Tokio runtime and affecting other tasks. Here are a few ways to run CPU-bound tasks in Tokio: tokio::task::spawn_blocking This is the preferred way to
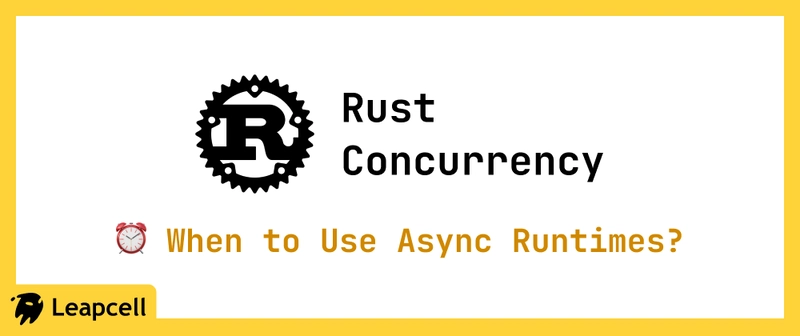
Rust's asynchronous runtimes are extremely useful in a variety of scenarios, especially in high-concurrency and high-performance I/O-intensive applications. Below are some common use cases for Rust async runtimes:
Network Programming
- Web servers and clients: Handling a large number of concurrent connections is a key requirement for web servers. Asynchronous runtimes allow servers to efficiently handle tens of thousands of concurrent requests without creating a separate thread for each connection. Runtimes like Tokio and async-std provide the necessary tools for building high-performance web applications, such as TCP/UDP sockets, HTTP clients, and servers.
- Real-time communication apps: Chat servers, online game servers, and similar services need to handle many concurrent connections and low-latency message delivery. Async runtimes can efficiently manage these connections and provide fast message routing and processing.
- Proxy servers and load balancers: These applications need to handle a large volume of concurrent connections and data forwarding. Async runtimes offer high-performance connection management and data transmission capabilities.
I/O-Intensive Applications
- Database drivers and clients: Database operations often involve significant I/O waiting. Async runtimes enable applications to continue executing other tasks while waiting for database responses, improving overall performance.
- File I/O operations: Applications that process large files or need to access multiple files simultaneously can benefit from async I/O to boost efficiency. Examples include image processing and log analysis.
- Microservices architecture: In microservices architectures, services usually communicate over the network. Async runtimes can improve communication efficiency and concurrency handling between services.
Concurrency and Parallelism
-
Parallel computation: While Rust’s
std::thread
module can be used for parallel computation, async runtimes are often more effective in I/O-bound scenarios. For instance, they can be used to download multiple files or process multiple network requests in parallel. - Task queues and background processing: Async runtimes can be used to implement task queues, allowing time-consuming tasks to be executed asynchronously and avoiding blocking the main thread.
For CPU-intensive applications, using an async runtime is typically not the best choice. While Rust async runtimes excel in I/O-heavy applications, they may not bring significant performance improvements in CPU-bound scenarios and could even introduce extra overhead.
Why Are Async Runtimes Not Recommended for CPU-Intensive Applications?
The primary advantage of async runtimes lies in efficiently handling I/O waits. When a program has to wait for I/O operations (such as network requests or file reads/writes) to complete, an async runtime allows the CPU to perform other tasks in the meantime, thus improving CPU utilization. However, in CPU-intensive applications, the CPU is constantly busy and has no idle time to execute other tasks, meaning the benefits of async runtimes cannot be realized.
Async programming introduces additional overhead. It requires maintaining task states, performing context switches, and other operations—all of which come at a cost. In CPU-intensive applications, this overhead may offset or even exceed the potential gains from async programming.
Threads are still the preferred choice for CPU-bound tasks. For CPU-intensive workloads, using multithreading is generally more effective. By breaking tasks into multiple subtasks and executing them in parallel across multiple CPU cores, the performance of multi-core processors can be fully leveraged. Rust's std::thread
module provides functionality to create and manage threads, making it easy to implement parallel computation. Consider using a thread pool to optimize resource usage.
When Should You Consider Using Async Runtimes in CPU-Intensive Applications?
Although async runtimes are usually not recommended for purely CPU-bound applications, there are cases where CPU-bound tasks also involve some I/O operations—such as reading configuration files or writing logs. In these cases, using an async runtime may improve the efficiency of those I/O operations. However, it’s important to ensure that I/O operations do not dominate, or the cost may still outweigh the benefits.
In addition, if CPU-intensive tasks need to integrate with other async tasks—such as handling network requests—it may make sense to use an async runtime to maintain a unified programming model, which helps organize and maintain code.
Although Tokio is primarily optimized for I/O-bound tasks, it also provides mechanisms to handle CPU-bound workloads, preventing them from blocking the Tokio runtime and affecting other tasks. Here are a few ways to run CPU-bound tasks in Tokio:
tokio::task::spawn_blocking
This is the preferred way to execute CPU-bound tasks in Tokio. The spawn_blocking
function moves the given closure into a dedicated thread pool separate from the Tokio runtime. This ensures that CPU-bound tasks do not block the runtime threads, preserving the performance of I/O-bound tasks.
use tokio::task;
#[tokio::main]
async fn main() {
// Perform some async I/O operations
println!("Starting I/O operation");
tokio::time::sleep(std::time::Duration::from_millis(500)).await;
println!("I/O operation completed");
// Execute CPU-bound task
let result = task::spawn_blocking(move || {
println!("Starting CPU-intensive task");
// Perform some CPU-heavy computation
let mut sum = 0u64;
for i in 0..100000000 {
sum += i;
}
println!("CPU-intensive task completed");
sum
}).await.unwrap();
println!("Computation result: {}", result);
}
In this example, the CPU-intensive computation is placed in the closure passed to spawn_blocking
. Even if this computation takes a long time, it won't block the Tokio runtime, and I/O tasks can still proceed normally.
Using an Independent Thread Pool
Another approach is to use a separate thread pool to execute CPU-intensive tasks. You can use std::thread
or a library like rayon
to create and manage the thread pool. Then, use channels (std::sync::mpsc
or tokio::sync::mpsc
) to transfer data between the Tokio runtime and the thread pool.
use std::thread;
use std::sync::mpsc;
use tokio::runtime::Runtime;
fn main() {
let rt = Runtime::new().unwrap();
let (tx, rx) = mpsc::channel();
rt.block_on(async {
// Perform some async I/O operations
println!("Starting I/O operation");
tokio::time::sleep(std::time::Duration::from_millis(500)).await;
println!("I/O operation completed");
// Send CPU-intensive task to thread pool
let tx_clone = tx.clone();
thread::spawn(move || {
println!("Starting CPU-intensive task");
// Perform some CPU-heavy computation
let mut sum = 0u64;
for i in 0..100000000 {
sum += i;
}
println!("CPU-intensive task completed");
tx_clone.send(sum).unwrap();
});
// Receive result from channel
let result = rx.recv().unwrap();
println!("Computation result: {}", result);
});
}
For simple CPU-bound tasks, spawn_blocking
is the easiest and most convenient approach. For more complex CPU-heavy tasks or scenarios requiring finer control over the thread pool, using a dedicated thread pool may be more appropriate.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ