Rethinking Tech Interviews: Real Skills, Real Projects, No Bullshit
Introduction Let’s be honest — most software engineering interviews suck. They’re filled with algorithmic trivia, abstract whiteboarding, and awkward “culture fit” questions that have nothing to do with the actual job. The result? Interviews that waste time for everyone and still fail to identify great engineers. This blog is for both interviewers and interviewees — whether you’re designing a hiring process or preparing for one. The goal is to make interviews practical, time-efficient, and focused on what truly matters: real-world engineering skills. What follows is a no-nonsense framework based on real projects, meaningful collaboration, and practical coding. It respects your time, cuts the fluff, and gives you real signal — fast. 1. Start with a GitHub (or GitLab) Project Walkthrough What to do: Ask the candidate to walk through a personal or professional project they're proud of. Dive deep into: The why behind the project Their architecture or design choices Real implementation challenges and how they solved them Example prompts: “What tradeoffs did you make here?” “Would you change anything about this design today?” “How did you test this module?” Why it works: You’re evaluating practical experience, ownership, and problem-solving — not memorization. Caveat: Not everyone has public projects. Offer the chance to discuss anonymized work or a written project summary. Don’t penalize candidates without open-source contributions. 2. Ask Role-Relevant Technical Questions (No Trivia) What to do: Ask practical, role-specific questions: Programming fundamentals (e.g., memory management, async flow) SOLID principles or OOP concepts in context Tech relevant to the role (e.g., Docker, message queues, testing frameworks) Operating System concepts Data Structures fundamentals (e.g., Big-O notation) Bad: “What’s the time complexity of a red-black tree insertion?” Good: “Let’s say you’re building an API for file uploads. How would you handle large files?” Why it works: You're checking if they can apply technical concepts to real problems. Caveat: Depth should match the role. Don’t quiz a frontend dev on database locking or a junior dev on system design at scale. 3. Code Review + Refactor: Test How They Think About Bad Code What to do: Provide a small, deliberately messy code sample — 2–3 files, 100–150 lines max. Include: Unclear naming Some logic duplication Mild violations of style/architecture Then ask them to: Review the code and point out what’s wrong Refactor it in a way they believe improves the design Explain why their changes are better Follow-up: Ask: “What test would you write first after this refactor?” to assess how their cleanup impacts testability. What you're testing: Can they identify practical issues? Do they understand real-world maintainability? How do they prioritize: clarity, performance, structure? Can they articulate technical decisions? Why it works: This simulates real work. Engineers constantly read and fix code, not just write from scratch. You see their eye for detail, tradeoffs, and communication ability. Caveats: Tailor to experience: Give simpler code to juniors (naming, formatting), more structural issues to seniors (e.g., poor separation of concerns). Avoid trick questions: Make the code realistically bad — don’t set traps. Timebox it: 30–45 minutes is enough. Don't let it sprawl. 4. Realistic Coding Task — AI Allowed What to do: Assign a real-world problem like: Write a script in your preferred language that fetches data from a public REST API, processes it, and saves the output as a file (any format). Include at least 2 unit tests. Rules: They can use AI tools, docs, or the web — just like real life. They must explain their code in detail. Code must be clean, readable, and minimally documented. What you're evaluating: Can they code in the language they claim? Do they understand the code they copied or referenced? Do they value quality (tests, naming, structure)? Why it works: You’re not testing memory — you’re testing how they work in real-world scenarios, with real tools. Caveat: Be explicit: it's okay to use external help, but they must understand and own every line they submit. Bonus: Soft Skills Appear Naturally You don’t need a separate "culture fit" round. Communication and collaboration show up organically: How they explain their project How they justify a refactor How they handle follow-up questions or critique Just observe: Are they clear? Are they open to feedback? Can they defend ideas without being defensive? That’s culture. Conclusion: A Better Interview Framework A good interview doesn’t need whiteboards or brainteasers. It needs real-world simulation — code, discussion, reasoning, collaboration. What you get: Candidates who can actually do the job A fairer process with less noise Stronger signals in less
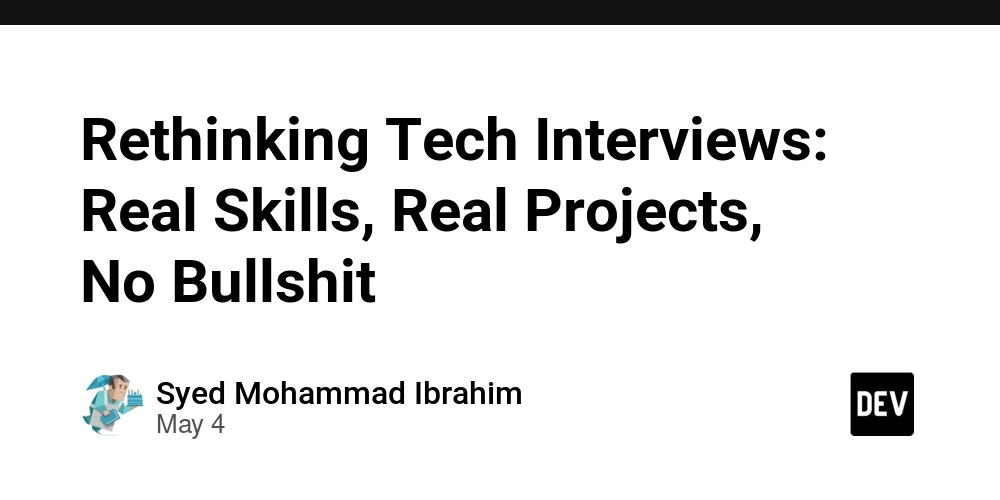
Introduction
Let’s be honest — most software engineering interviews suck.
They’re filled with algorithmic trivia, abstract whiteboarding, and awkward “culture fit” questions that have nothing to do with the actual job. The result? Interviews that waste time for everyone and still fail to identify great engineers.
This blog is for both interviewers and interviewees — whether you’re designing a hiring process or preparing for one. The goal is to make interviews practical, time-efficient, and focused on what truly matters: real-world engineering skills.
What follows is a no-nonsense framework based on real projects, meaningful collaboration, and practical coding. It respects your time, cuts the fluff, and gives you real signal — fast.
1. Start with a GitHub (or GitLab) Project Walkthrough
What to do:
Ask the candidate to walk through a personal or professional project they're proud of. Dive deep into:
- The why behind the project
- Their architecture or design choices
- Real implementation challenges and how they solved them
Example prompts:
- “What tradeoffs did you make here?”
- “Would you change anything about this design today?”
- “How did you test this module?”
Why it works:
You’re evaluating practical experience, ownership, and problem-solving — not memorization.
Caveat:
Not everyone has public projects. Offer the chance to discuss anonymized work or a written project summary. Don’t penalize candidates without open-source contributions.
2. Ask Role-Relevant Technical Questions (No Trivia)
What to do:
Ask practical, role-specific questions:
- Programming fundamentals (e.g., memory management, async flow)
- SOLID principles or OOP concepts in context
- Tech relevant to the role (e.g., Docker, message queues, testing frameworks)
- Operating System concepts
- Data Structures fundamentals (e.g., Big-O notation)
Bad:
“What’s the time complexity of a red-black tree insertion?”
Good:
“Let’s say you’re building an API for file uploads. How would you handle large files?”
Why it works:
You're checking if they can apply technical concepts to real problems.
Caveat:
Depth should match the role. Don’t quiz a frontend dev on database locking or a junior dev on system design at scale.
3. Code Review + Refactor: Test How They Think About Bad Code
What to do:
Provide a small, deliberately messy code sample — 2–3 files, 100–150 lines max. Include:
- Unclear naming
- Some logic duplication
- Mild violations of style/architecture
Then ask them to:
- Review the code and point out what’s wrong
- Refactor it in a way they believe improves the design
- Explain why their changes are better
Follow-up:
Ask: “What test would you write first after this refactor?” to assess how their cleanup impacts testability.
What you're testing:
- Can they identify practical issues?
- Do they understand real-world maintainability?
- How do they prioritize: clarity, performance, structure?
- Can they articulate technical decisions?
Why it works:
This simulates real work. Engineers constantly read and fix code, not just write from scratch. You see their eye for detail, tradeoffs, and communication ability.
Caveats:
- Tailor to experience: Give simpler code to juniors (naming, formatting), more structural issues to seniors (e.g., poor separation of concerns).
- Avoid trick questions: Make the code realistically bad — don’t set traps.
- Timebox it: 30–45 minutes is enough. Don't let it sprawl.
4. Realistic Coding Task — AI Allowed
What to do:
Assign a real-world problem like:
Write a script in your preferred language that fetches data from a public REST API, processes it, and saves the output as a file (any format). Include at least 2 unit tests.
Rules:
- They can use AI tools, docs, or the web — just like real life.
- They must explain their code in detail.
- Code must be clean, readable, and minimally documented.
What you're evaluating:
- Can they code in the language they claim?
- Do they understand the code they copied or referenced?
- Do they value quality (tests, naming, structure)?
Why it works:
You’re not testing memory — you’re testing how they work in real-world scenarios, with real tools.
Caveat:
Be explicit: it's okay to use external help, but they must understand and own every line they submit.
Bonus: Soft Skills Appear Naturally
You don’t need a separate "culture fit" round. Communication and collaboration show up organically:
- How they explain their project
- How they justify a refactor
- How they handle follow-up questions or critique
Just observe:
- Are they clear?
- Are they open to feedback?
- Can they defend ideas without being defensive?
That’s culture.
Conclusion: A Better Interview Framework
A good interview doesn’t need whiteboards or brainteasers. It needs real-world simulation — code, discussion, reasoning, collaboration.
What you get:
- Candidates who can actually do the job
- A fairer process with less noise
- Stronger signals in less time
Let’s stop playing games. Hire engineers like engineers.