REST APIs vs GraphQL: is it one or the other?
GraphQL and REST are popular methods for building APIs that facilitate data transfer across networks. Both approaches emphasize key principles such as statelessness and the clear separation of client and server. They rely on HTTP as the main communication protocol. Both are flexible methods for developing APIs and can easily integrate with various programming languages and applications. As developers began to use APIs more, the need arose to filter and modify responses. Some APIs support synchronous calls, where one output serves as the input for another, while others can operate in parallel by design. This process, called API orchestration, highlights the advantages of GraphQL over REST APIs. But what if you’ve invested considerable engineering hours and money into developing REST APIs for your organization? Is it worth rewriting all of these to implement GraphQL? The good news is that you don’t have to rewrite any of your REST APIs to leverage GraphQL and utilize API Orchestration! Apollo Connectors for REST APIs make it easy and quick to integrate REST APIs into your GraphQL with no complex code to write or compile! To illustrate, consider a REST API endpoint /getOrders/:orderId, where orderId represents an order ID like “12345”. The response to a GET request on /getOrders/12345 would be: { "order_id": "12345", "customer": { "id": "C001", "name": "John Doe", "email": "johndoe@example.com" }, "items": [ { "product_id": "P1001", "name": "Wireless Mouse", "quantity": 2, "price": 25.99 }, { "product_id": "P1002", "name": "Mechanical Keyboard", "quantity": 1, "price": 89.99 } ], "total_price": 141.97, "status": "Shipped", "order_date": "2025-02-25T14:30:00Z" } To integrate this REST API with GraphQL, you only need to define the @source directive in your GraphQL schema like this: extend schema @link( url: "https://specs.apollo.dev/federation/v2.10" import: ["@key", "@requires"] ) @link( url: "https://specs.apollo.dev/connect/v0.1" import: ["@source", "@connect"] ) @source( name: "orders" http: { baseURL: "" }) Then add your Types and Query: type Order { orderId: ID! customer: String! totalItems: Float! totalPrice: Float! status: String! orderDate: String! } type Query { getOrder(order_id: ID!): Order @connect( source: "orders" http: { GET: "/getOrders/{$args.order_id}" } selection: """ orderId: order_id customer:customer.name totalItems: items->size totalPrice: total_price status orderDate: order_date """ ) } The @connect directive in the Query instructs GraphQL to use the “orders” connector. You can also specify a resource path that will be added to the baseUrl defined in the @source declarative statement. The selection field indicates how you want the data to be returned to the client. Here, you can optionally transform and filter the data. To help you build the selection mapping, Apollo provides a free Connectors Mapping Playground, as shown in the figure below. The connection mapper takes an input and can suggest a mapping based on that input. In this example, we are renaming fields and even transforming a field called totalItems to indicate how many order items it contains instead of listing the items themselves (e.g., “totalItems: items->size”). Using the Apollo Sandbox included with the rover CLI, you can run ad-hoc GraphQL queries. In the figure below, we executed the getOrder query by passing “12345”, and the returned data shown is from our REST API. All of this configuration was accomplished without any procedural code, saving time and making it easy to integrate your existing REST APIs into the graph! For more information on Apollo Connectors check out the following: Hear from Apollo GraphQL founder Matt DeBergalis: Apollo Connectors "It's the biggest thing we've ever shipped" - YouTube Learn about all the latest innovations in GraphQL: Livestream Launch: New innovations from Apollo Take a free Apollo Odyssey course on Apollo Connectors: Introducing Apollo Connectors - GraphQL Tutorials Documentation: Get Started with Apollo Connectors and GraphOS Create your Apollo GraphQL Studio for free https://studio.apollographql.com/signup
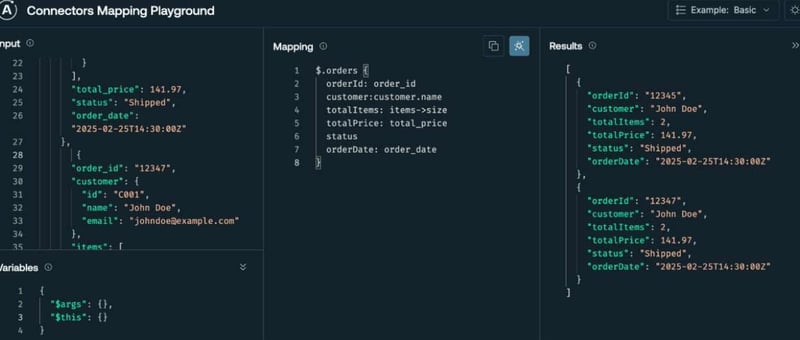
GraphQL and REST are popular methods for building APIs that facilitate data transfer across networks. Both approaches emphasize key principles such as statelessness and the clear separation of client and server. They rely on HTTP as the main communication protocol. Both are flexible methods for developing APIs and can easily integrate with various programming languages and applications. As developers began to use APIs more, the need arose to filter and modify responses. Some APIs support synchronous calls, where one output serves as the input for another, while others can operate in parallel by design. This process, called API orchestration, highlights the advantages of GraphQL over REST APIs.
But what if you’ve invested considerable engineering hours and money into developing REST APIs for your organization? Is it worth rewriting all of these to implement GraphQL?
The good news is that you don’t have to rewrite any of your REST APIs to leverage GraphQL and utilize API Orchestration! Apollo Connectors for REST APIs make it easy and quick to integrate REST APIs into your GraphQL with no complex code to write or compile!
To illustrate, consider a REST API endpoint /getOrders/:orderId
, where orderId represents an order ID like “12345”. The response to a GET request on /getOrders/12345
would be:
{
"order_id": "12345",
"customer": {
"id": "C001",
"name": "John Doe",
"email": "johndoe@example.com"
},
"items": [
{
"product_id": "P1001",
"name": "Wireless Mouse",
"quantity": 2,
"price": 25.99
},
{
"product_id": "P1002",
"name": "Mechanical Keyboard",
"quantity": 1,
"price": 89.99
}
],
"total_price": 141.97,
"status": "Shipped",
"order_date": "2025-02-25T14:30:00Z"
}
To integrate this REST API with GraphQL, you only need to define the @source
directive in your GraphQL schema like this:
extend schema
@link(
url: "https://specs.apollo.dev/federation/v2.10"
import: ["@key", "@requires"]
)
@link(
url: "https://specs.apollo.dev/connect/v0.1"
import: ["@source", "@connect"]
)
@source(
name: "orders"
http: {
baseURL: "<>"
})
Then add your Types and Query:
type Order {
orderId: ID!
customer: String!
totalItems: Float!
totalPrice: Float!
status: String!
orderDate: String!
}
type Query {
getOrder(order_id: ID!): Order
@connect(
source: "orders"
http: { GET: "/getOrders/{$args.order_id}" }
selection: """
orderId: order_id
customer:customer.name
totalItems: items->size
totalPrice: total_price
status
orderDate: order_date
"""
)
}
The @connect
directive in the Query instructs GraphQL to use the “orders” connector. You can also specify a resource path that will be added to the baseUrl defined in the @source
declarative statement. The selection field indicates how you want the data to be returned to the client. Here, you can optionally transform and filter the data. To help you build the selection mapping, Apollo provides a free Connectors Mapping Playground, as shown in the figure below.
The connection mapper takes an input and can suggest a mapping based on that input. In this example, we are renaming fields and even transforming a field called totalItems to indicate how many order items it contains instead of listing the items themselves (e.g., “totalItems: items->size”). Using the Apollo Sandbox included with the rover CLI, you can run ad-hoc GraphQL queries. In the figure below, we executed the getOrder query by passing “12345”, and the returned data shown is from our REST API.
All of this configuration was accomplished without any procedural code, saving time and making it easy to integrate your existing REST APIs into the graph!
For more information on Apollo Connectors check out the following:
Hear from Apollo GraphQL founder Matt DeBergalis: Apollo Connectors "It's the biggest thing we've ever shipped" - YouTube
Learn about all the latest innovations in GraphQL: Livestream Launch: New innovations from Apollo
Take a free Apollo Odyssey course on Apollo Connectors: Introducing Apollo Connectors - GraphQL Tutorials
Documentation: Get Started with Apollo Connectors and GraphOS
Create your Apollo GraphQL Studio for free https://studio.apollographql.com/signup