Read PDF Metadata in C# Using .NET REST API for Better Document Insights
Extracting hidden metadata from PDF files is often a vital task in enterprise settings, particularly for data audits, content classification, and compliance purposes. With the GroupDocs.Metadata Cloud .NET SDK, developers can effortlessly retrieve PDF metadata programmatically using minimal C# code. There's no need for complicated dependencies or manual inspections — just straightforward, reliable access to document properties via a secure .NET REST API. Whether you're developing a digital archiving solution, incorporating document processing features into your web application, or setting up a security mechanism to identify metadata leaks, this SDK facilitates PDF metadata reading in .NET with unmatched precision and efficiency. It enables the extraction of metadata information, creation/modification timestamps, author details, producer information, and more — all in a format that integrates effortlessly with your current C# or ASP.NET applications. This capability for metadata extraction is part of a larger ecosystem powered by the GroupDocs.Metadata Cloud .NET platform. By utilizing this SDK, you gain access to a scalable, cloud-based solution designed for contemporary development environments — all without the hassle of manually handling PDF parsing logic. Explore how to incorporate advanced document insights into your .NET workflows by checking out our extensive tutorial today. The C# code example below assists you in integrating this functionality into your desktop or web applications: using GroupDocs.Metadata.Cloud.Sdk.Api; using GroupDocs.Metadata.Cloud.Sdk.Client; using GroupDocs.Metadata.Cloud.Sdk.Model; using GroupDocs.Metadata.Cloud.Sdk.Model.Requests; class Program { static void Main(string[] args) { // Step 1: Set up API credentials and initialize configuration string MyAppKey = "your-app-key"; string MyAppSecret = "your-app-secret"; var configuration = new Configuration(MyAppKey, MyAppSecret); // Step 2: Initialize Metadata API for extracting metadata var metadataApi = new MetadataApi(configuration); // Step 3: Set up the file info var fileInfo = new GroupDocs.Metadata.Cloud.Sdk.Model.FileInfo { // Path of the source file in the cloud storage FilePath = "SampleFiles/source.pdf" }; // Step 4: Define extraction options var extractOptions = new ExtractOptions { FileInfo = fileInfo, }; // Step 5: Create and execute the request to extract metadata var extractRequest = new ExtractRequest(extractOptions); var response = metadataApi.Extract(extractRequest); // Step 6: Print the extracted PDF metadata var innerPK = response.MetadataTree.InnerPackages; for (int i = 0; i < innerPK.Count; i++) { Console.WriteLine($"\nPackage: {innerPK[i].PackageName}"); var packageProperties = innerPK[i].PackageProperties; for (int j = 0; j < packageProperties.Count; j++) { Console.WriteLine(packageProperties[j].Name + " : " + packageProperties[j].Value); } } } }
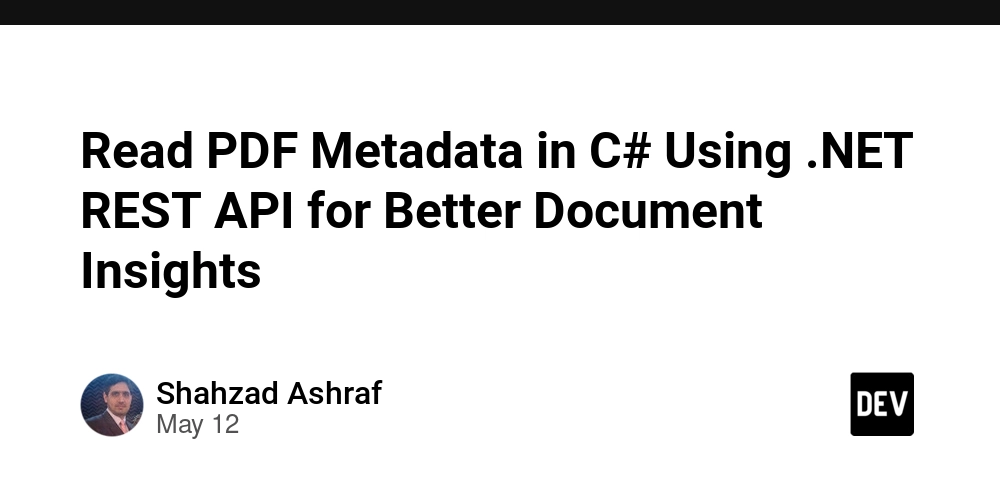
Extracting hidden metadata from PDF files is often a vital task in enterprise settings, particularly for data audits, content classification, and compliance purposes. With the GroupDocs.Metadata Cloud .NET SDK, developers can effortlessly retrieve PDF metadata programmatically using minimal C# code. There's no need for complicated dependencies or manual inspections — just straightforward, reliable access to document properties via a secure .NET REST API.
Whether you're developing a digital archiving solution, incorporating document processing features into your web application, or setting up a security mechanism to identify metadata leaks, this SDK facilitates PDF metadata reading in .NET with unmatched precision and efficiency. It enables the extraction of metadata information, creation/modification timestamps, author details, producer information, and more — all in a format that integrates effortlessly with your current C# or ASP.NET applications.
This capability for metadata extraction is part of a larger ecosystem powered by the GroupDocs.Metadata Cloud .NET platform. By utilizing this SDK, you gain access to a scalable, cloud-based solution designed for contemporary development environments — all without the hassle of manually handling PDF parsing logic. Explore how to incorporate advanced document insights into your .NET workflows by checking out our extensive tutorial today.
The C# code example below assists you in integrating this functionality into your desktop or web applications:
using GroupDocs.Metadata.Cloud.Sdk.Api;
using GroupDocs.Metadata.Cloud.Sdk.Client;
using GroupDocs.Metadata.Cloud.Sdk.Model;
using GroupDocs.Metadata.Cloud.Sdk.Model.Requests;
class Program
{
static void Main(string[] args)
{
// Step 1: Set up API credentials and initialize configuration
string MyAppKey = "your-app-key";
string MyAppSecret = "your-app-secret";
var configuration = new Configuration(MyAppKey, MyAppSecret);
// Step 2: Initialize Metadata API for extracting metadata
var metadataApi = new MetadataApi(configuration);
// Step 3: Set up the file info
var fileInfo = new GroupDocs.Metadata.Cloud.Sdk.Model.FileInfo
{
// Path of the source file in the cloud storage
FilePath = "SampleFiles/source.pdf"
};
// Step 4: Define extraction options
var extractOptions = new ExtractOptions
{
FileInfo = fileInfo,
};
// Step 5: Create and execute the request to extract metadata
var extractRequest = new ExtractRequest(extractOptions);
var response = metadataApi.Extract(extractRequest);
// Step 6: Print the extracted PDF metadata
var innerPK = response.MetadataTree.InnerPackages;
for (int i = 0; i < innerPK.Count; i++)
{
Console.WriteLine($"\nPackage: {innerPK[i].PackageName}");
var packageProperties = innerPK[i].PackageProperties;
for (int j = 0; j < packageProperties.Count; j++)
{
Console.WriteLine(packageProperties[j].Name + " : "
+ packageProperties[j].Value);
}
}
}
}