React Hooks Explained: A Beginner's Guide
** What is a Hook? ** Hooks were added to React in version 16.8. They allow function components to access state and other React features. Hooks allows us to "hook" into React features such as state and lifecycle methods. For example: import React, { useState } from 'react'; const Counter = () => { //useState hook to manage count state const [count, setCount] = useState(0); return ( Count: {count} setCount(count + 1)}>Increase ); }; export default Counter; Explanation: useState(0): Initializes count with 0. setCount(count + 1): Updates count when the button is clicked. This is a simple example of how hooks let function components manage state without needing a class component. Hook Rules There are 3 rules for hooks: Hooks can only be called inside React function components. Hooks can only be called at the top level of a component. Hooks cannot be conditional Let's go into the different react hooks; useState The React useState Hook allows us to track state in a function component. state generally refers to data or properties that need to be tracking in an application. Just like in the example above, it is used to manage local state change, and each time the state changes, the component re-renders with the new value. useEffect
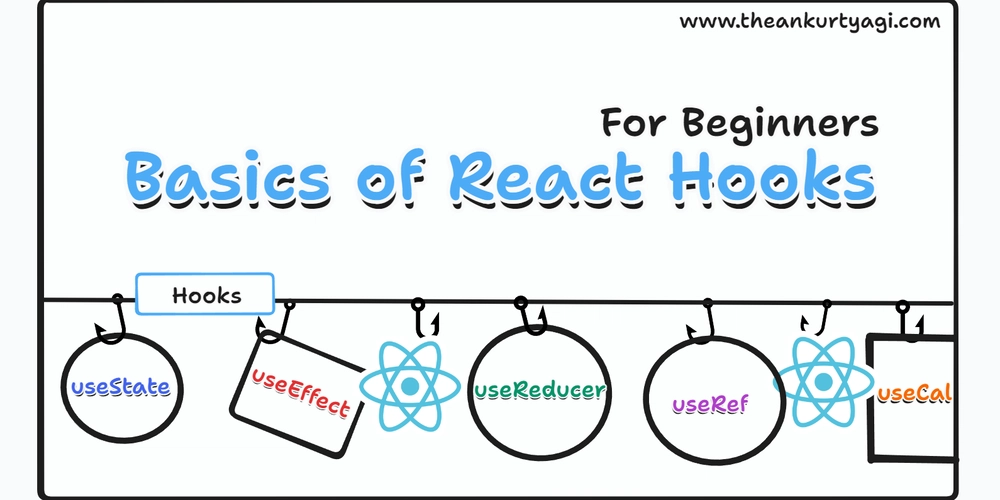
**
What is a Hook?
**
Hooks were added to React in version 16.8. They allow function components to access state and other React features. Hooks allows us to "hook" into React features such as state and lifecycle methods.
For example:
import React, { useState } from 'react';
const Counter = () => {
//useState hook to manage count state
const [count, setCount] = useState(0);
return (
Count: {count}
);
};
export default Counter;
Explanation:
useState(0): Initializes count with 0.
setCount(count + 1): Updates count when the button is clicked.
This is a simple example of how hooks let function components manage state without needing a class component.
Hook Rules
There are 3 rules for hooks:
- Hooks can only be called inside React function components.
- Hooks can only be called at the top level of a component.
- Hooks cannot be conditional
Let's go into the different react hooks;
useState
The React useState Hook allows us to track state in a function component. state generally refers to data or properties that need to be tracking in an application. Just like in the example above, it is used to manage local state change, and each time the state changes, the component re-renders with the new value.