Parsing and Formatting Time Strings 5/10
Working with dates and times is a fundamental part of programming, yet it can be surprisingly tricky. Go takes a unique approach to time formatting and parsing that might seem strange at first but becomes quite elegant once you understand it. In this article, I'll walk you through how Go handles time strings, from formatting to parsing, and cover some common pitfalls you might encounter along the way. How Go formats dates with "2006-01-02 15:04:05" If you're coming from other programming languages, Go's approach to date formatting might throw you off initially. Instead of using format specifiers like %Y-%m-%d or yyyy-MM-dd, Go uses a reference date: January 2, 2006 at 3:04:05 PM MST. const layout = "2006-01-02 15:04:05" now := time.Now() formatted := now.Format(layout) fmt.Println(formatted) // Outputs something like: 2025-03-19 14:23:45 This reference date isn't arbitrary. It's actually a clever mnemonic: 2006 (year), 01 (month), 02 (day), 15 (hour), 04 (minute), 05 (second). Or, numerically: 1, 2, 3, 4, 5, 6, 7 (where 7 corresponds to the time zone, MST or -0700). The beauty of this approach is that your format string looks exactly like the output you want. Want your date to look like "Jan 02, 2006"? That's exactly what you use as your format string. No need to remember that %b means abbreviated month name or that %Y means 4-digit year. The reference time also includes the following components that you can use: Year: 2006 Month: 01 (numeric) or Jan (name) Day: 02 (numeric) or Monday (name) Hour: 15 (24-hour) or 3 (12-hour) Minute: 04 Second: 05 Timezone: MST or -0700 The reference date is also known as the "magic date" or the "magic constant" among Go developers. Once you get used to it, you'll likely find it more intuitive than the format specifiers used in other languages. Formatting time values with Format() Once you understand Go's reference date approach, using the Format() method becomes straightforward. This method takes a layout string as its parameter and returns a formatted string representation of the time value. // Create a time value t := time.Date(2025, time.March, 19, 14, 30, 45, 0, time.UTC) // Basic formatting fmt.Println(t.Format("2006-01-02")) // 2025-03-19 fmt.Println(t.Format("Jan 2, 2006")) // Mar 19, 2025 fmt.Println(t.Format("15:04:05")) // 14:30:45 fmt.Println(t.Format("3:04 PM")) // 2:30 PM You can mix and match the reference date components to create virtually any date format you need. Here are some more examples: // More complex formats fmt.Println(t.Format("Monday, January 2, 2006")) // Wednesday, March 19, 2025 fmt.Println(t.Format("2006/01/02 15:04:05.000")) // 2025/03/19 14:30:45.000 fmt.Println(t.Format("2006-01-02T15:04:05Z07:00")) // 2025-03-19T14:30:45Z Go also provides some predefined layouts in the time package for common formats: fmt.Println(t.Format(time.RFC3339)) // 2025-03-19T14:30:45Z fmt.Println(t.Format(time.RFC822)) // 19 Mar 25 14:30 UTC fmt.Println(t.Format(time.Kitchen)) // 2:30PM fmt.Println(t.Format(time.Stamp)) // Mar 19 14:30:45 The Format() method always returns a string, so it's perfect for situations where you need to display dates to users or include them in logs, JSON responses, or any other text-based output. One thing to keep in mind is that the Format() method uses the time zone information stored in the time value. If your time value doesn't have the correct time zone set, your formatted output might not be what you expect. We'll cover time zones in more detail in a later section. Parsing time strings into time.Time (Parse(), ParseInLocation()) Parsing is the inverse of formatting—it converts string representations of dates and times into time.Time values. Go provides several functions for this purpose, with the two most common being Parse() and ParseInLocation(). Basic Parsing with Parse() The Parse() function takes two parameters: a layout string and the time string to parse. The layout string should follow the same reference date format we discussed earlier. // Parse a date string timeStr := "2025-03-19 14:30:45" layout := "2006-01-02 15:04:05" t, err := time.Parse(layout, timeStr) if err != nil { log.Fatal(err) } fmt.Println(t) // 2025-03-19 14:30:45 +0000 UTC One important detail to note: Parse() returns a time.Time value in UTC by default, unless the input string explicitly specifies a time zone. This is a common source of confusion for newcomers to Go. Parsing with Time Zones using ParseInLocation() If you're parsing a time string that doesn't include time zone information but you know what time zone it should be in, use ParseInLocation(). This function takes an additional *time.Location parameter: // Parse a date string in a specific location (time zone) timeStr := "2025-03-19 14:30:45" layout := "2006-01-02 15:04:05" loc, err := time.LoadLocatio
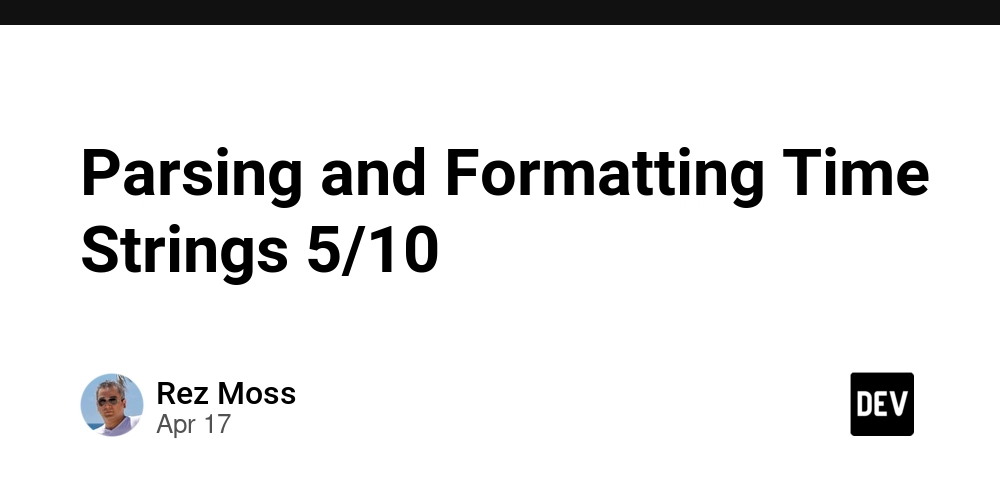
Working with dates and times is a fundamental part of programming, yet it can be surprisingly tricky. Go takes a unique approach to time formatting and parsing that might seem strange at first but becomes quite elegant once you understand it. In this article, I'll walk you through how Go handles time strings, from formatting to parsing, and cover some common pitfalls you might encounter along the way.
How Go formats dates with "2006-01-02 15:04:05"
If you're coming from other programming languages, Go's approach to date formatting might throw you off initially. Instead of using format specifiers like %Y-%m-%d
or yyyy-MM-dd
, Go uses a reference date: January 2, 2006 at 3:04:05 PM MST.
const layout = "2006-01-02 15:04:05"
now := time.Now()
formatted := now.Format(layout)
fmt.Println(formatted) // Outputs something like: 2025-03-19 14:23:45
This reference date isn't arbitrary. It's actually a clever mnemonic: 2006 (year), 01 (month), 02 (day), 15 (hour), 04 (minute), 05 (second). Or, numerically: 1, 2, 3, 4, 5, 6, 7 (where 7 corresponds to the time zone, MST or -0700).
The beauty of this approach is that your format string looks exactly like the output you want. Want your date to look like "Jan 02, 2006"? That's exactly what you use as your format string. No need to remember that %b
means abbreviated month name or that %Y
means 4-digit year.
The reference time also includes the following components that you can use:
Year: 2006
Month: 01 (numeric) or Jan (name)
Day: 02 (numeric) or Monday (name)
Hour: 15 (24-hour) or 3 (12-hour)
Minute: 04
Second: 05
Timezone: MST or -0700
The reference date is also known as the "magic date" or the "magic constant" among Go developers. Once you get used to it, you'll likely find it more intuitive than the format specifiers used in other languages.
Formatting time values with Format()
Once you understand Go's reference date approach, using the Format()
method becomes straightforward. This method takes a layout string as its parameter and returns a formatted string representation of the time value.
// Create a time value
t := time.Date(2025, time.March, 19, 14, 30, 45, 0, time.UTC)
// Basic formatting
fmt.Println(t.Format("2006-01-02")) // 2025-03-19
fmt.Println(t.Format("Jan 2, 2006")) // Mar 19, 2025
fmt.Println(t.Format("15:04:05")) // 14:30:45
fmt.Println(t.Format("3:04 PM")) // 2:30 PM
You can mix and match the reference date components to create virtually any date format you need. Here are some more examples:
// More complex formats
fmt.Println(t.Format("Monday, January 2, 2006")) // Wednesday, March 19, 2025
fmt.Println(t.Format("2006/01/02 15:04:05.000")) // 2025/03/19 14:30:45.000
fmt.Println(t.Format("2006-01-02T15:04:05Z07:00")) // 2025-03-19T14:30:45Z
Go also provides some predefined layouts in the time
package for common formats:
fmt.Println(t.Format(time.RFC3339)) // 2025-03-19T14:30:45Z
fmt.Println(t.Format(time.RFC822)) // 19 Mar 25 14:30 UTC
fmt.Println(t.Format(time.Kitchen)) // 2:30PM
fmt.Println(t.Format(time.Stamp)) // Mar 19 14:30:45
The Format()
method always returns a string, so it's perfect for situations where you need to display dates to users or include them in logs, JSON responses, or any other text-based output.
One thing to keep in mind is that the Format()
method uses the time zone information stored in the time value. If your time value doesn't have the correct time zone set, your formatted output might not be what you expect. We'll cover time zones in more detail in a later section.
Parsing time strings into time.Time
(Parse()
, ParseInLocation()
)
Parsing is the inverse of formatting—it converts string representations of dates and times into time.Time
values. Go provides several functions for this purpose, with the two most common being Parse()
and ParseInLocation()
.
Basic Parsing with Parse()
The Parse()
function takes two parameters: a layout string and the time string to parse. The layout string should follow the same reference date format we discussed earlier.
// Parse a date string
timeStr := "2025-03-19 14:30:45"
layout := "2006-01-02 15:04:05"
t, err := time.Parse(layout, timeStr)
if err != nil {
log.Fatal(err)
}
fmt.Println(t) // 2025-03-19 14:30:45 +0000 UTC
One important detail to note: Parse()
returns a time.Time
value in UTC by default, unless the input string explicitly specifies a time zone. This is a common source of confusion for newcomers to Go.
Parsing with Time Zones using ParseInLocation()
If you're parsing a time string that doesn't include time zone information but you know what time zone it should be in, use ParseInLocation()
. This function takes an additional *time.Location
parameter:
// Parse a date string in a specific location (time zone)
timeStr := "2025-03-19 14:30:45"
layout := "2006-01-02 15:04:05"
loc, err := time.LoadLocation("America/New_York")
if err != nil {
log.Fatal(err)
}
t, err := time.ParseInLocation(layout, timeStr, loc)
if err != nil {
log.Fatal(err)
}
fmt.Println(t) // 2025-03-19 14:30:45 -0400 EDT
Using ParseInLocation()
ensures that the parsed time is correctly interpreted in the specified time zone, which can be crucial for applications dealing with users in different parts of the world.
Working with Unix Timestamps
For working with Unix timestamps (seconds since January 1, 1970 UTC), Go provides the time.Unix()
function:
// Convert Unix timestamp to time.Time
timestamp := int64(1679234567)
t := time.Unix(timestamp, 0)
fmt.Println(t) // 2023-03-19 16:49:27 +0000 UTC
// Get Unix timestamp from time.Time
currentTime := time.Now()
unixTime := currentTime.Unix()
fmt.Println(unixTime) // e.g., 1647606587
This is particularly useful when working with systems that represent time as Unix timestamps, such as many databases and APIs.
Remember that parsing operations can fail if the input string doesn't match the expected format. Always check the error return value from parsing functions to ensure your code handles invalid input gracefully.
Handling different formats and time zones
Time zones can be one of the most challenging aspects of working with dates and times in any programming language, and Go is no exception. Let's explore how to handle different formats and time zones effectively.
Working with Multiple Formats
In real-world applications, you often need to parse dates in various formats. Here's how you can handle multiple potential formats:
func parseAnyFormat(dateStr string) (time.Time, error) {
formats := []string{
"2006-01-02",
"01/02/2006",
"Jan 2, 2006",
"2006-01-02 15:04:05",
time.RFC3339,
}
var t time.Time
var err error
for _, format := range formats {
t, err = time.Parse(format, dateStr)
if err == nil {
return t, nil
}
}
return time.Time{}, fmt.Errorf("could not parse date: %s", dateStr)
}
This function tries each format in sequence and returns the first successful parse. Note that this is a naive implementation and might not work correctly for ambiguous date formats (e.g., "01/02/2006" vs "02/01/2006").
Understanding Time Zones
Time zones in Go are represented by the time.Location
type. There are a few ways to get a Location
:
// Get the local time zone
local := time.Local
// Get UTC
utc := time.UTC
// Load a named location
nyc, err := time.LoadLocation("America/New_York")
if err != nil {
log.Fatal(err)
}
// Fixed offset (UTC+8)
offset := time.FixedZone("UTC+8", 8*60*60)
The LoadLocation()
function loads a location by its IANA Time Zone Database name (like "America/New_York" or "Asia/Tokyo"). These names are more reliable than abbreviations like "EST" or "JST" because they account for daylight saving time changes and historical time zone shifts.
Converting Between Time Zones
Once you have a time.Time
value, you can convert it to a different time zone using the In()
method:
// Create a time in UTC
utcTime := time.Date(2025, 3, 19, 14, 30, 0, 0, time.UTC)
fmt.Println(utcTime) // 2025-03-19 14:30:00 +0000 UTC
// Convert to New York time
nyc, _ := time.LoadLocation("America/New_York")
nycTime := utcTime.In(nyc)
fmt.Println(nycTime) // 2025-03-19 10:30:00 -0400 EDT
It's important to understand that In()
doesn't change the moment in time, just how it's represented. The underlying instant (like 2:30 PM UTC on March 19, 2025) remains the same, but it's expressed in terms of a different time zone.
Handling Time Zone in Format Strings
You can include time zone information in your format strings:
t := time.Now()
fmt.Println(t.Format("2006-01-02 15:04:05 MST")) // e.g., 2025-03-19 14:30:45 EDT
fmt.Println(t.Format("2006-01-02 15:04:05 -0700")) // e.g., 2025-03-19 14:30:45 -0400
fmt.Println(t.Format("2006-01-02T15:04:05Z07:00")) // e.g., 2025-03-19T14:30:45-04:00
These formats are particularly useful when generating time strings that need to be consumed by other systems or when displaying times to users in different parts of the world.
Remember that time zone handling can be complex, especially when dealing with daylight saving time transitions, historical time changes, and user inputs. Always test your code thoroughly with edge cases to ensure it behaves as expected.
Error handling in date parsing (ParseError
)
Proper error handling is crucial when working with time parsing, as user input or external data might not always conform to your expected formats. Go's time package provides specific error types and patterns to help you deal with parsing failures gracefully.
Understanding ParseError
When a parsing operation fails, Go returns a time.ParseError
that contains detailed information about what went wrong. This struct has the following fields:
type ParseError struct {
Layout string
Value string
LayoutElem string
ValueElem string
Message string
}
These fields help you identify exactly what part of the parsing failed:
-
Layout
: The format string you provided -
Value
: The input string you tried to parse -
LayoutElem
: The specific layout element that failed to match -
ValueElem
: The corresponding part of the input string -
Message
: A human-readable error message
You can extract this information to provide more helpful feedback:
timeStr := "2025-13-45" // Invalid month and day
layout := "2006-01-02"
_, err := time.Parse(layout, timeStr)
if err != nil {
if pe, ok := err.(*time.ParseError); ok {
fmt.Printf("Error parsing time: %s\n", pe.Message)
fmt.Printf("Layout: %s, Value: %s\n", pe.Layout, pe.Value)
fmt.Printf("Layout element: %s, Value element: %s\n", pe.LayoutElem, pe.ValueElem)
} else {
fmt.Printf("Unexpected error: %v\n", err)
}
}
This might output something like:
Error parsing time: month out of range
Layout: 2006-01-02, Value: 2025-13-45
Layout element: 01, Value element: 13
Practical Error Handling Strategies
In practical applications, you'll want to implement more robust error handling patterns. Here are some strategies:
1. Validate Before Parsing
For user-facing applications, it's often better to validate the format before attempting to parse:
func validateDateFormat(date string) bool {
// Simple regex to check if the date looks like YYYY-MM-DD
matched, _ := regexp.MatchString(`^\d{4}-\d{2}-\d{2}$`, date)
return matched
}
// Usage
dateStr := "2025-03-19"
if validateDateFormat(dateStr) {
t, err := time.Parse("2006-01-02", dateStr)
if err != nil {
// Handle parsing error
}
// Use the parsed time
} else {
// Inform the user about the incorrect format
}
2. Fallback Values
When parsing dates in non-critical contexts, you might want to provide a fallback:
func parseWithFallback(dateStr, layout string, fallback time.Time) time.Time {
t, err := time.Parse(layout, dateStr)
if err != nil {
return fallback
}
return t
}
// Usage
defaultDate := time.Date(2025, 1, 1, 0, 0, 0, 0, time.UTC)
date := parseWithFallback(userInput, "2006-01-02", defaultDate)
3. Flexible Parsing
As we saw in the previous section, you can try multiple formats:
func parseFlexibleDate(dateStr string) (time.Time, error) {
formats := []string{
"2006-01-02",
"01/02/2006",
"Jan 2, 2006",
// Add more formats as needed
}
for _, fmt := range formats {
if t, err := time.Parse(fmt, dateStr); err == nil {
return t, nil
}
}
return time.Time{}, fmt.Errorf("could not parse date '%s' with any known format", dateStr)
}
4. Logging and Monitoring
In production systems, log parsing errors to help identify patterns of problematic input:
func parseTimeWithLogging(timeStr, layout string) (time.Time, error) {
t, err := time.Parse(layout, timeStr)
if err != nil {
log.Printf("Time parsing error: %v (input: '%s', layout: '%s')",
err, timeStr, layout)
// You might also want to increment a metric counter here
return time.Time{}, err
}
return t, nil
}
Zero Value Checks
After parsing, it's often a good idea to check if you got a zero value (time.Time{}
), which might indicate a parsing problem even if no error was returned:
t, err := time.Parse("2006-01-02", dateStr)
if err != nil {
// Handle explicit error
} else if t.IsZero() {
// Handle zero time (though this shouldn't happen with Parse)
}
By implementing thoughtful error handling for time parsing, you can make your applications more robust and provide better feedback to users, making the experience smoother even when things don't go exactly as planned.