Object.getOwnPropertyDescriptors and Property Management
Object.getOwnPropertyDescriptors and Property Management: A Comprehensive Guide Historical and Technical Context The JavaScript language has evolved substantially since its inception in 1995, with each iteration adding new constructs and features to facilitate more robust programming paradigms. One notable improvement in ECMAScript 5 (2011) was the introduction of property descriptors which provides developers more granular control over object properties. Property descriptors represent the characteristics of a property, such as its value, writability, enumerability, and configurability. This fine-tuned control allows developers to manage object properties with greater precision, paving the way for advanced programming techniques, such as creating non-enumerable properties or preventing properties from being deleted. The Object.getOwnPropertyDescriptors method, introduced in ECMAScript 2015 (ES6), allows developers to retrieve an object’s property descriptors in a succinct and efficient manner. It returns all key-value pairs of the own properties of an object, with each property represented by an object descriptor that includes value, writable, enumerable, and configurable. Technical Specifications According to the specifications from TC39 (the committee in charge of evolving JavaScript), Object.getOwnPropertyDescriptors provides a means to obtain all the property descriptors of the target object, allowing for deeper manipulation and introspection of properties. Syntax const descriptors = Object.getOwnPropertyDescriptors(obj); obj: The object whose own property descriptors you want to retrieve. Returns An object whose own properties are the same as obj, but the values are the corresponding property descriptors. Example Usage const obj = { name: 'John', age: 30 }; const descriptors = Object.getOwnPropertyDescriptors(obj); console.log(descriptors); /* { name: { value: 'John', writable: true, enumerable: true, configurable: true }, age: { value: 30, writable: true, enumerable: true, configurable: true } } */ In-Depth Code Examples Basic Use Case Let’s begin with a basic illustration of Object.getOwnPropertyDescriptors. const user = { name: 'Alice', age: 25 }; Object.defineProperty(user, 'id', { value: '1001', enumerable: false, configurable: true }); const userDescriptors = Object.getOwnPropertyDescriptors(user); console.log(userDescriptors); /* { name: { value: 'Alice', writable: true, enumerable: true, configurable: true }, age: { value: 25, writable: true, enumerable: true, configurable: true }, id: { value: '1001', writable: false, enumerable: false, configurable: true } } */ Property Management Using property descriptors, developers can manipulate and manage their object's properties effectively. Let's illustrate how Object.defineProperties() works alongside Object.getOwnPropertyDescriptors. Creating a Deep Clone function cloneDeep(obj) { const descriptors = Object.getOwnPropertyDescriptors(obj); return Object.create( Object.getPrototypeOf(obj), descriptors ); } const original = { name: 'Bob', age: 45 }; const clone = cloneDeep(original); clone.name = 'Charlie'; // Change clone's name console.log(original.name); // Output: Bob console.log(clone.name); // Output: Charlie Fallback for Older Environments In environments where Object.getOwnPropertyDescriptors is not natively supported (like older browsers), you can create a polyfill: if (typeof Object.getOwnPropertyDescriptors !== 'function') { Object.getOwnPropertyDescriptors = function(obj) { const result = {}; for (let prop in obj) { if (obj.hasOwnProperty(prop)) { result[prop] = Object.getOwnPropertyDescriptor(obj, prop); } } return result; }; } Advanced Scenarios Nature of writable, enumerable, and configurable The properties writable, enumerable, and configurable can lead to nuanced behaviors in JavaScript. Let’s look at what happens when you try to change these attributes after they are set. const obj = {}; Object.defineProperty(obj, 'key', { value: 'value', writable: false, enumerable: false, configurable: false }); console.log(Object.getOwnPropertyDescriptors(obj)); // Attempt to change: try { Object.defineProperty(obj, 'key', { writable: true }); } catch (e) { console.error(e); // TypeError: Cannot redefine property: key } Real-World Use Cases Frameworks & Libraries: Modern JavaScript frameworks such as Vue.js or React utilize property descriptors to enforce reactivity. They watch for changes to object properties and apply updates using getters/setters defined in the property descriptors, which optimizes performance and offers the developers a clean API. Data Models: In applications like ORMs (Object-Relational Mappers), property d
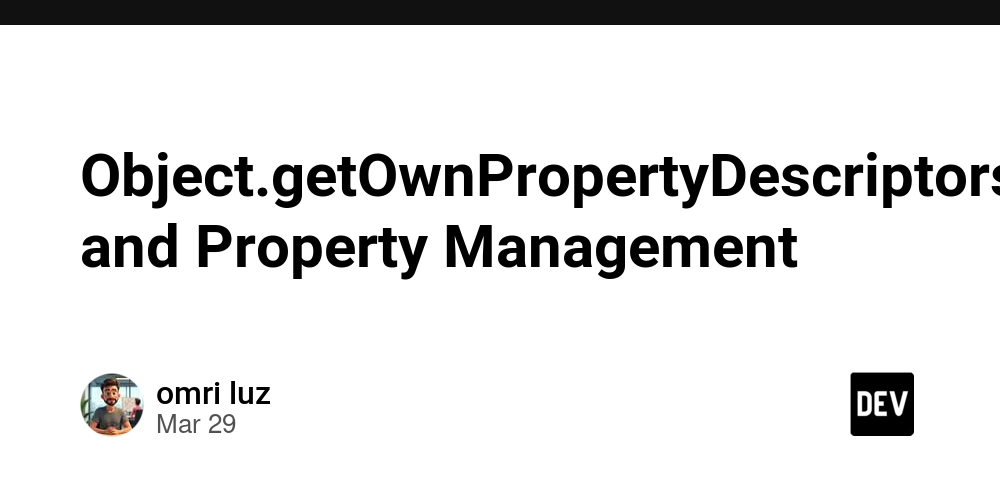
Object.getOwnPropertyDescriptors and Property Management: A Comprehensive Guide
Historical and Technical Context
The JavaScript language has evolved substantially since its inception in 1995, with each iteration adding new constructs and features to facilitate more robust programming paradigms. One notable improvement in ECMAScript 5 (2011) was the introduction of property descriptors which provides developers more granular control over object properties. Property descriptors represent the characteristics of a property, such as its value, writability, enumerability, and configurability.
This fine-tuned control allows developers to manage object properties with greater precision, paving the way for advanced programming techniques, such as creating non-enumerable properties or preventing properties from being deleted.
The Object.getOwnPropertyDescriptors
method, introduced in ECMAScript 2015 (ES6), allows developers to retrieve an object’s property descriptors in a succinct and efficient manner. It returns all key-value pairs of the own properties of an object, with each property represented by an object descriptor that includes value
, writable
, enumerable
, and configurable
.
Technical Specifications
According to the specifications from TC39 (the committee in charge of evolving JavaScript), Object.getOwnPropertyDescriptors
provides a means to obtain all the property descriptors of the target object, allowing for deeper manipulation and introspection of properties.
Syntax
const descriptors = Object.getOwnPropertyDescriptors(obj);
-
obj
: The object whose own property descriptors you want to retrieve.
Returns
An object whose own properties are the same as obj
, but the values are the corresponding property descriptors.
Example Usage
const obj = {
name: 'John',
age: 30
};
const descriptors = Object.getOwnPropertyDescriptors(obj);
console.log(descriptors);
/*
{
name: { value: 'John', writable: true, enumerable: true, configurable: true },
age: { value: 30, writable: true, enumerable: true, configurable: true }
}
*/
In-Depth Code Examples
Basic Use Case
Let’s begin with a basic illustration of Object.getOwnPropertyDescriptors
.
const user = {
name: 'Alice',
age: 25
};
Object.defineProperty(user, 'id', {
value: '1001',
enumerable: false,
configurable: true
});
const userDescriptors = Object.getOwnPropertyDescriptors(user);
console.log(userDescriptors);
/*
{
name: { value: 'Alice', writable: true, enumerable: true, configurable: true },
age: { value: 25, writable: true, enumerable: true, configurable: true },
id: { value: '1001', writable: false, enumerable: false, configurable: true }
}
*/
Property Management
Using property descriptors, developers can manipulate and manage their object's properties effectively. Let's illustrate how Object.defineProperties()
works alongside Object.getOwnPropertyDescriptors
.
Creating a Deep Clone
function cloneDeep(obj) {
const descriptors = Object.getOwnPropertyDescriptors(obj);
return Object.create(
Object.getPrototypeOf(obj),
descriptors
);
}
const original = {
name: 'Bob',
age: 45
};
const clone = cloneDeep(original);
clone.name = 'Charlie'; // Change clone's name
console.log(original.name); // Output: Bob
console.log(clone.name); // Output: Charlie
Fallback for Older Environments
In environments where Object.getOwnPropertyDescriptors
is not natively supported (like older browsers), you can create a polyfill:
if (typeof Object.getOwnPropertyDescriptors !== 'function') {
Object.getOwnPropertyDescriptors = function(obj) {
const result = {};
for (let prop in obj) {
if (obj.hasOwnProperty(prop)) {
result[prop] = Object.getOwnPropertyDescriptor(obj, prop);
}
}
return result;
};
}
Advanced Scenarios
Nature of writable
, enumerable
, and configurable
The properties writable
, enumerable
, and configurable
can lead to nuanced behaviors in JavaScript. Let’s look at what happens when you try to change these attributes after they are set.
const obj = {};
Object.defineProperty(obj, 'key', {
value: 'value',
writable: false,
enumerable: false,
configurable: false
});
console.log(Object.getOwnPropertyDescriptors(obj));
// Attempt to change:
try {
Object.defineProperty(obj, 'key', { writable: true });
} catch (e) {
console.error(e); // TypeError: Cannot redefine property: key
}
Real-World Use Cases
Frameworks & Libraries: Modern JavaScript frameworks such as Vue.js or React utilize property descriptors to enforce reactivity. They watch for changes to object properties and apply updates using getters/setters defined in the property descriptors, which optimizes performance and offers the developers a clean API.
Data Models: In applications like ORMs (Object-Relational Mappers), property descriptors are incredibly useful for defining the characteristics of data models, such as marking certain properties as read-only or defining default values.
Plugin Systems: Modular systems often use property descriptors to expose certain APIs while keeping internal functionalities encapsulated. By managing property descriptors carefully, developers can ensure that plugins operate as intended but cannot inadvertently modify core functionality.
Performance Considerations
The use of Object.getOwnPropertyDescriptors
provides a convenient way to access property information at the expense of performance, especially in scenarios involving large objects. Recent versions of JavaScript engines have optimized these methods, but the overhead of object cloning (even when using property descriptors to create copies) should be considered.
Optimization Strategies
Minimize Property Changes: Avoid excessive changes to object properties after their descriptors have been set, as this can lead to performance degradation over time.
Batch Processing: If you need to change a bulk of properties, consider collecting descriptors first and then applying all changes at once rather than modifying them property-by-property.
Use of Prototypes: By leveraging prototype inheritance, you can reduce the number of property descriptors directly set on instances, using less memory and improving cache efficiency.
Pitfalls and Advanced Debugging Techniques
Overlooking Non-Enumerability: Remember that properties defined with
enumerable: false
will not appear infor...in
loops orObject.keys()
, which can lead to confusion.Immutable Properties: Setting
configurable: false
prevents future changes to that property, including the ability to delete it or redefine its attributes. Use this with caution.Deep Cloning Complexity: While
Object.getOwnPropertyDescriptors
can be used for shallow cloning, be wary of trying to create deep clones of nested objects as they will require a more complex implementation to handle references correctly.
Advanced Debugging Using Proxies
For advanced debugging, JavaScript's Proxy can be a brilliant tool. It allows you to watch property access and changes dynamically.
const user = {
name: 'David',
age: 50
};
const handler = {
get(target, prop) {
console.log(`Getting ${prop}:`, target[prop]);
return target[prop];
},
set(target, prop, value) {
console.log(`Setting ${prop} to ${value}`);
target[prop] = value;
}
};
const proxyUser = new Proxy(user, handler);
console.log(proxyUser.name); // Log: Getting name: David
proxyUser.age = 60; // Log: Setting age to 60
References and Advanced Resources
- ECMAScript 2015 (ES6) Specification
- MDN Documentation on Object.getOwnPropertyDescriptors
- JavaScript: The Definitive Guide
- Understanding JavaScript Proxy Objects
Conclusion
In conclusion, Object.getOwnPropertyDescriptors
provides developers invaluable insight into an object's properties, allowing for sophisticated property management and control. Understanding the breadth and depth of property descriptors is essential for modern JavaScript development, particularly in frameworks that rely heavily on reactive programming. Through careful consideration of performance and potential pitfalls, advanced developers can leverage this feature fully, ensuring robust and maintainable JavaScript applications. This article aims to serve as a comprehensive resource, bringing together theoretical knowledge, practical applications, and advanced coding techniques that are essential for any senior JavaScript developer.