Nullish Coalescing and Optional Chaining Explained
Nullish Coalescing and Optional Chaining Explained: A Comprehensive Guide JavaScript is an evolving language, constantly adapting to the needs of its developer community. Among the recent additions to the language are Nullish Coalescing and Optional Chaining. These features offer elegant solutions for dealing with values that may be null or undefined, significantly improving code readability and maintainability. In this exhaustive technical exploration, we will delve into the historical context, provide in-depth code examples, examine edge cases, evaluate alternative approaches, showcase real-world use cases, discuss performance considerations, and highlight debugging techniques. Historical Context JavaScript has grappled with various concerns regarding nullish values since its inception. Prior to ECMAScript 2020 (ES11), the language used more traditional techniques for handling null and undefined. The former approaches often relied on logical operators or type checks that lacked clarity. Platforms, libraries, and frameworks often introduced custom utility functions. Pre-ES2020: The Challenges Logical OR (||): The most common strategy was to use the logical OR operator. However, this approach could introduce bugs: const value = userInput || 'default'; // Problematic if userInput is 0, '', or false Here, a falsy value (like 0) improperly yields the default. Type Checking: Developers frequently resorted to explicit type checks to determine whether a variable was null or undefined: const value = (userInput !== null && userInput !== undefined) ? userInput : 'default'; This approach, while clear, resulted in more verbose code that was challenging to maintain. Introduction of Nullish Coalescing (??) and Optional Chaining (?.) In June 2020, ECMAScript’s proposal for the Nullish Coalescing Operator (??) and Optional Chaining Operator (?.) was finalized as part of ECMAScript 2020. Nullish Coalescing Operator (??): This operator returns the right-hand operand when the left-hand operand is null or undefined, providing a more precise fallback mechanism. Optional Chaining Operator (?.): This operator allows developers to safely access deeply nested properties without having to check each level for existence explicitly. Understanding Nullish Coalescing Operator (??) Syntax The Nullish Coalescing Operator (??) is used as follows: const a = null; const defaultValue = 'default'; const value = a ?? defaultValue; // value is 'default' Detailed Use Cases and Complexity Handling Falsy Values: Unlike the logical OR operator, the nullish coalescing operator does not treat falsy values (such as 0, NaN, and '') as reasons to fallback to the default. This distinction is crucial. const input = 0; const result = input ?? 'default'; // result is 0 Combining with Optional Chaining: This operator shines in instances where objects may not be entirely defined. const user = { preferences: { theme: null } }; const theme = user.preferences?.theme ?? 'light'; // theme is 'light' Chaining: As you combine the two features, they permit cleaner access to deeply nested properties: const user = { settings: { display: { brightness: undefined } } }; const brightness = user.settings?.display?.brightness ?? 50; // brightness is 50 Edge Cases Multiple Nullish Checks: If you have multiple possible sources of values, combining ?? with other logical operators can lead to undesirable outcomes. const a = null; const b = undefined; const c = false; const result = a ?? b ?? c; // result is false, not null or undefined Advanced Implementation Techniques Default Function Parameters: You can use the Nullish Coalescing operator to define default function parameters: function calculateTotal(price, discount) { discount = discount ?? 0; // Default to 0 if undefined or null return price - discount; } Exploring Optional Chaining Operator (?.) Syntax and Behavior The Optional Chaining Operator (?.) allows you to read the value of a property deep within a nested object without having to validate each reference in the chain. const user = { address: { city: 'New York' } }; const city = user.address?.city; // 'New York' const zip = user.address?.zip; // undefined, avoids error Detailed Use Cases Deep Property Access: If a nested property doesn't exist, undefined is returned without throwing an error. const user = { name: 'Alice', contact: null }; const email = user.contact?.email; // email is undefined, rather than throwing TypeError Array and Function Invocations: You can also use optional chaining with array methods and function calls. const users
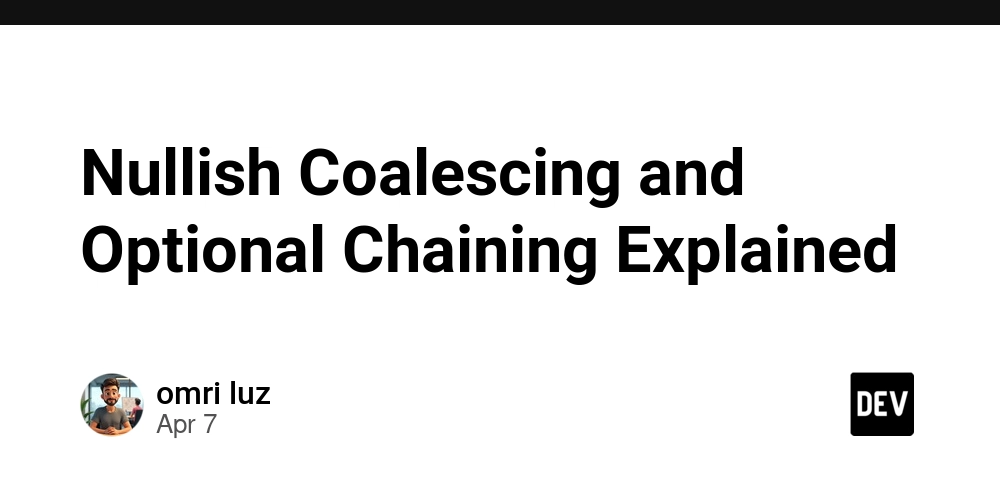
Nullish Coalescing and Optional Chaining Explained: A Comprehensive Guide
JavaScript is an evolving language, constantly adapting to the needs of its developer community. Among the recent additions to the language are Nullish Coalescing and Optional Chaining. These features offer elegant solutions for dealing with values that may be null
or undefined
, significantly improving code readability and maintainability.
In this exhaustive technical exploration, we will delve into the historical context, provide in-depth code examples, examine edge cases, evaluate alternative approaches, showcase real-world use cases, discuss performance considerations, and highlight debugging techniques.
Historical Context
JavaScript has grappled with various concerns regarding nullish values since its inception. Prior to ECMAScript 2020 (ES11), the language used more traditional techniques for handling null
and undefined
. The former approaches often relied on logical operators or type checks that lacked clarity. Platforms, libraries, and frameworks often introduced custom utility functions.
Pre-ES2020: The Challenges
-
Logical OR (
||
): The most common strategy was to use the logical OR operator. However, this approach could introduce bugs:
const value = userInput || 'default'; // Problematic if userInput is 0, '', or false
Here, a falsy value (like 0
) improperly yields the default.
-
Type Checking:
Developers frequently resorted to explicit type checks to determine whether a variable was
null
orundefined
:
const value = (userInput !== null && userInput !== undefined) ? userInput : 'default';
This approach, while clear, resulted in more verbose code that was challenging to maintain.
Introduction of Nullish Coalescing (??
) and Optional Chaining (?.
)
In June 2020, ECMAScript’s proposal for the Nullish Coalescing Operator (??
) and Optional Chaining Operator (?.
) was finalized as part of ECMAScript 2020.
Nullish Coalescing Operator (
??
): This operator returns the right-hand operand when the left-hand operand isnull
orundefined
, providing a more precise fallback mechanism.Optional Chaining Operator (
?.
): This operator allows developers to safely access deeply nested properties without having to check each level for existence explicitly.
Understanding Nullish Coalescing Operator (??
)
Syntax
The Nullish Coalescing Operator (??
) is used as follows:
const a = null;
const defaultValue = 'default';
const value = a ?? defaultValue; // value is 'default'
Detailed Use Cases and Complexity
-
Handling Falsy Values:
Unlike the logical OR operator, the nullish coalescing operator does not treat falsy values (such as
0
,NaN
, and''
) as reasons to fallback to the default. This distinction is crucial.
const input = 0;
const result = input ?? 'default'; // result is 0
- Combining with Optional Chaining:
This operator shines in instances where objects may not be entirely defined.
const user = {
preferences: {
theme: null
}
};
const theme = user.preferences?.theme ?? 'light'; // theme is 'light'
- Chaining:
As you combine the two features, they permit cleaner access to deeply nested properties:
const user = {
settings: {
display: {
brightness: undefined
}
}
};
const brightness = user.settings?.display?.brightness ?? 50; // brightness is 50
Edge Cases
- Multiple Nullish Checks:
If you have multiple possible sources of values, combining ??
with other logical operators can lead to undesirable outcomes.
const a = null;
const b = undefined;
const c = false;
const result = a ?? b ?? c; // result is false, not null or undefined
Advanced Implementation Techniques
- Default Function Parameters: You can use the Nullish Coalescing operator to define default function parameters:
function calculateTotal(price, discount) {
discount = discount ?? 0; // Default to 0 if undefined or null
return price - discount;
}
Exploring Optional Chaining Operator (?.
)
Syntax and Behavior
The Optional Chaining Operator (?.
) allows you to read the value of a property deep within a nested object without having to validate each reference in the chain.
const user = {
address: {
city: 'New York'
}
};
const city = user.address?.city; // 'New York'
const zip = user.address?.zip; // undefined, avoids error
Detailed Use Cases
- Deep Property Access:
If a nested property doesn't exist, undefined
is returned without throwing an error.
const user = { name: 'Alice', contact: null };
const email = user.contact?.email; // email is undefined, rather than throwing TypeError
- Array and Function Invocations:
You can also use optional chaining with array methods and function calls.
const users = [{ name: 'Alice', greet: () => 'Hello' }];
const greeting = users[0]?.greet?.(); // 'Hello'
const farewell = users[1]?.greet?.(); // undefined
Complex Scenario
You can effectively combine ?.
and ??
for default handling:
const state = {
user: {
profile: {
age: null
}
}
};
const userAge = state.user?.profile?.age ?? 18; // userAge is 18
Performance Considerations and Optimization Strategies
Performance Analysis
Both ??
and ?.
are compiled directly into JavaScript, thus maintaining nearly the same performance level as traditional approaches. However, nesting these operators can lead to more complex expressions that may affect the readability and debugging capacity of code.
Optimization Tips
Minimize Deep Nesting:
Proliferating deep property access using these operators can slow down execution time slightly compared to more straightforward logic checks.Evaluate Context:
Use these operators judiciously in performance-critical code paths (e.g., rendering loops in a React application) to prevent any unnecessary checks.
Comparing Alternative Approaches
Logical OR Operator (||
)
As previously noted, the logical OR operator returns the right operand if the left is falsy. This behavior can often lead to undesired results.
const result = input || 'default'; // returns 'default' for any falsy input
Ternary Operator
Using the ternary operator can be clearer when the full set of conditions must be handled explicitly:
const result = userInput !== null && userInput !== undefined ? userInput : 'default';
However, this leads to verbose syntax.
Real-World Use Cases
- Web Applications:
Modern web applications often require safe property access due to the dynamic nature of user interfaces. Implementations of optional chaining and coalescing can make code much more expressive:
const profileImage = user.profile?.image ?? 'default.png';
- API Responses:
API responses can vary dramatically, especially with optional fields. Using these features can prevent runtime errors.
const value = apiResponse.data?.value ?? 'Not Found';
- React Components:
In React, using optional chaining can simplify the rendering logic significantly, where optional properties are common.
return (
<div>
<h1>{user.name ?? 'Guest'}</h1>
<img src={user.profile?.avatar ?? 'default.png'} alt="Profile" />
</div>
);
Potential Pitfalls and Advanced Debugging Techniques
Common Pitfalls
Unexpected Return Values: Always ensure that the value returned via
??
is indeed what is expected (for instance, zero being treated as a non-default).Overuse of Optional Chaining: Though syntactically nice, it can create confusion. Overusing can lead to diminished readability.
Debugging Techniques
- Utilizing Console Logs: Use console log statements to compare values before applying the operators, ensuring that you're aware of edge states for variables.
console.log('Address:', user.address); // Check the state of address before using optional chaining
- Test Cases: Develop comprehensive unit tests that cover all potential states.
Advanced Resources
Conclusion
Nullish Coalescing and Optional Chaining provide powerful mechanisms for cleanly managing nullish values in JavaScript. By understanding their syntactical nuances along with potential pitfalls and performance considerations, developers can write more maintainable and resilient code. These features, when properly leveraged, can significantly enhance the developer experience in building modern JavaScript applications.
This article serves as a foundational resource for advanced JavaScript developers to harness these concepts effectively, drive productivity, and maintain code robustness in applications of any scale.