.NET Learning Notes: How to Add a JWTVersion Filter
At times, we use JWT and, after sending it, we want to verify its validity. To do this, we include a version in the JWT. If the version matches the one in the database, it's considered valid. However, if the version is lower than the one in the database, it's deemed invalid. To avoid checking this every where, we implement a filter to perform the check. 1.Add a Attribute to mark the method which does not need to check [AttributeUsage(AttributeTargets.Method, AllowMultiple = false)] public class NotCheckJwtVersionAttribute : System.Attribute { } 2.Add a filter to check jwtversion public class JwtVersionCheckFilter(UserManager userManager) : IAsyncActionFilter { public async Task OnActionExecutionAsync(ActionExecutingContext context, ActionExecutionDelegate next) { var actionDescriptor = context.ActionDescriptor as ControllerActionDescriptor; if (actionDescriptor == null) { await next(); return; } var has = actionDescriptor.MethodInfo .GetCustomAttributes(typeof(NotCheckJwtVersionAttribute), false).Length != 0; if (has) { await next(); return; } var claimJwtVersion = context.HttpContext.User.FindFirst("JWTVersion"); if (claimJwtVersion == null) { context.Result = new ObjectResult("JWTVersion not found"){StatusCode = 404}; return; } var clientJwtVersion = Convert.ToInt64(claimJwtVersion.Value); var user = await userManager.FindByIdAsync(context.HttpContext.User.FindFirst("UserId").Value); if (user == null) { context.Result = new ObjectResult("User not found"){StatusCode = 404}; return; } if (user.Version > clientJwtVersion) { context.Result = new ObjectResult("JWTVersion expire"){StatusCode = 400}; return; } await next(); } } 3.Add filter in the program.cs builder.Services.AddControllers(options => { options.Filters.Add(order: 1); options.Filters.Add(order: 2); }); Note:In headers, we need to add Authorization Bearer Token
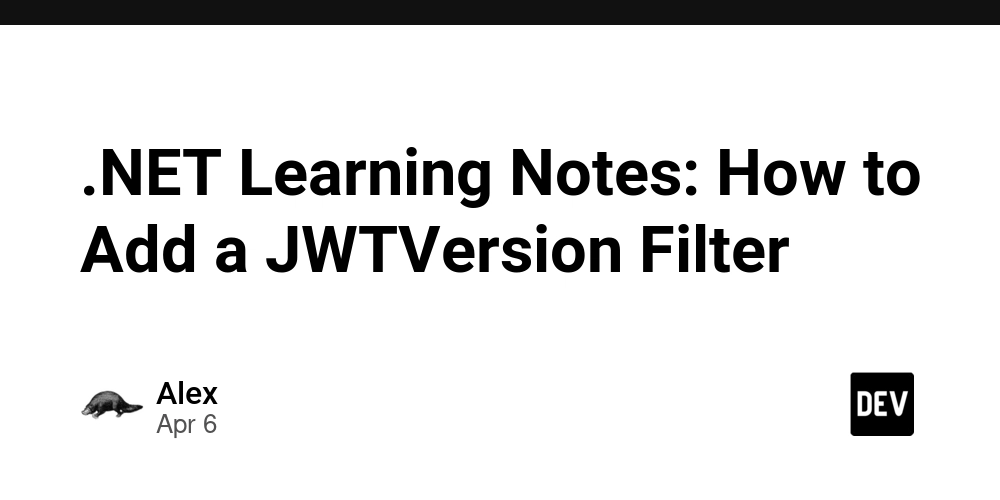
At times, we use JWT and, after sending it, we want to verify its validity. To do this, we include a version in the JWT. If the version matches the one in the database, it's considered valid. However, if the version is lower than the one in the database, it's deemed invalid. To avoid checking this every where, we implement a filter to perform the check.
1.Add a Attribute to mark the method which does not need to check
[AttributeUsage(AttributeTargets.Method, AllowMultiple = false)]
public class NotCheckJwtVersionAttribute : System.Attribute
{
}
2.Add a filter to check jwtversion
public class JwtVersionCheckFilter(UserManager userManager) : IAsyncActionFilter
{
public async Task OnActionExecutionAsync(ActionExecutingContext context, ActionExecutionDelegate next)
{
var actionDescriptor = context.ActionDescriptor as ControllerActionDescriptor;
if (actionDescriptor == null)
{
await next();
return;
}
var has = actionDescriptor.MethodInfo
.GetCustomAttributes(typeof(NotCheckJwtVersionAttribute), false).Length != 0;
if (has)
{
await next();
return;
}
var claimJwtVersion = context.HttpContext.User.FindFirst("JWTVersion");
if (claimJwtVersion == null)
{
context.Result = new ObjectResult("JWTVersion not found"){StatusCode = 404};
return;
}
var clientJwtVersion = Convert.ToInt64(claimJwtVersion.Value);
var user = await userManager.FindByIdAsync(context.HttpContext.User.FindFirst("UserId").Value);
if (user == null)
{
context.Result = new ObjectResult("User not found"){StatusCode = 404};
return;
}
if (user.Version > clientJwtVersion)
{
context.Result = new ObjectResult("JWTVersion expire"){StatusCode = 400};
return;
}
await next();
}
}
3.Add filter in the program.cs
builder.Services.AddControllers(options =>
{
options.Filters.Add(order: 1);
options.Filters.Add(order: 2);
});
Note:In headers, we need to add Authorization Bearer Token